Understanding return 1, return -1, and exit (1) in C Programming
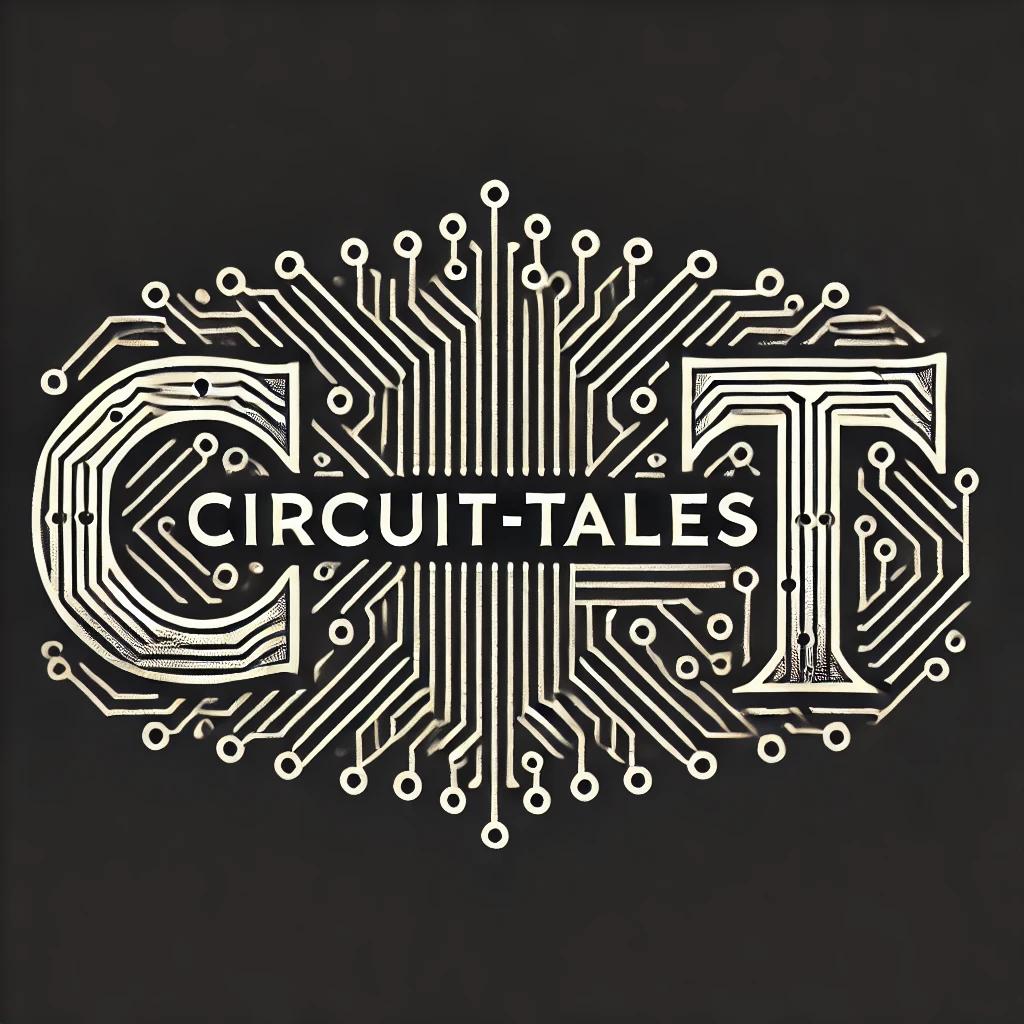
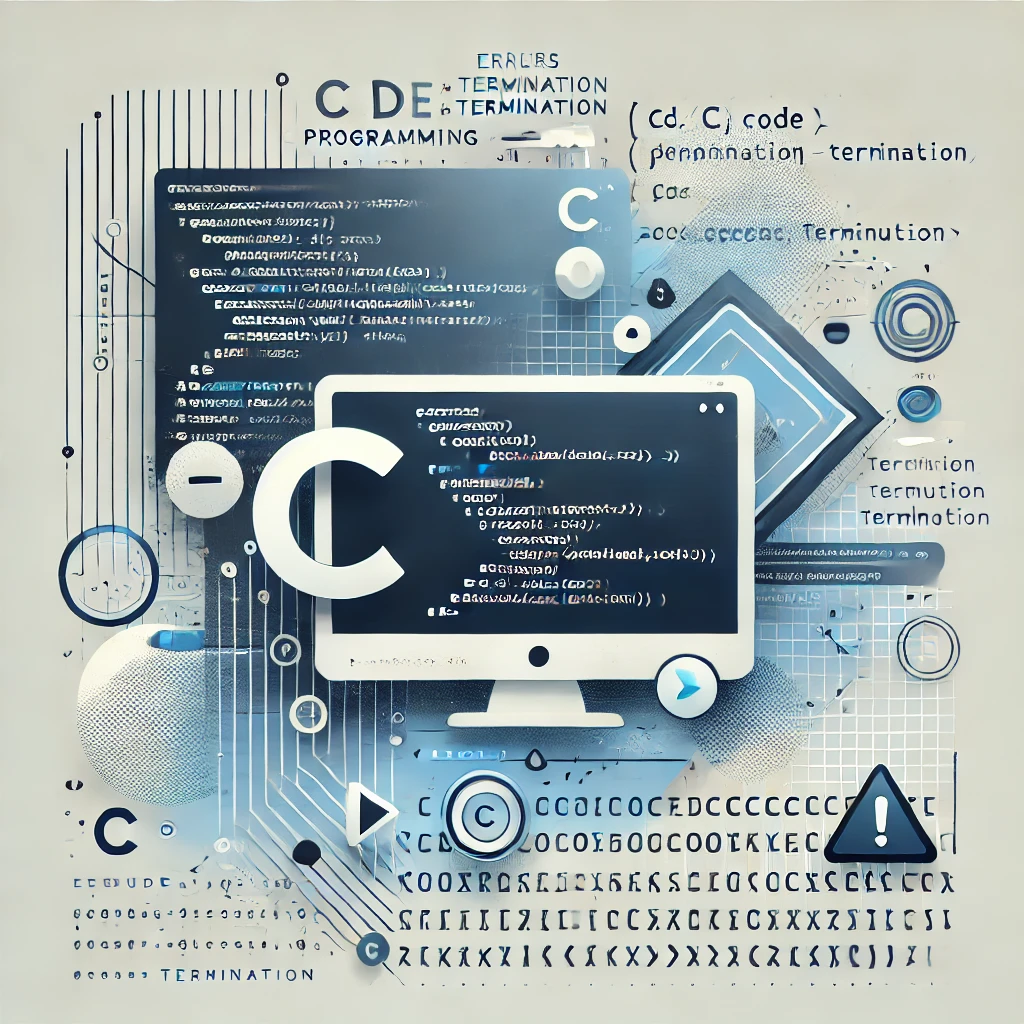
1. return 1
;
Usage: This is usually used to return from the
main
function in a C program.Meaning: Returning
1
frommain
typically indicates an error or abnormal termination of the program.
Example
#include <stdio.h>
int main() {
// Some condition that causes an error
if (error_condition) {
return 1; // Indicates an error
}
printf("Program completed successfully.\n");
return 0; // Indicates success
}
Explanation: In the above example, if error_condition
is true, the program will return 1
to indicate an error. If not, it will print a message and return 0
to indicate success.
2. return -1
Usage: Returning
-1
from themain
function or other functions is a non-standard way to indicate an error. It's often used in function definitions to return a value to the caller indicating something went wrong.Meaning: It signals an error or an exceptional condition.
Example
#include <stdio.h> int check_value(int value) { if (value < 0) { return -1; // Indicates an error } return 0; // Indicates success } int main() { int result = check_value(-5); if (result == -1) { printf("Error: Value is negative.\n"); return -1; // Indicates an error in main } printf("All values are valid.\n"); return 0; // Indicates success }
Explanation: In this example, the function
check_value
returns-1
if the input value is negative. Themain
function also returns-1
to indicate an error ifcheck_value
returns-1
.3.
exit (1)
Usage: The
exit
function terminates the program immediately and can be called from anywhere in the code. It passes an exit status to the operating system.Meaning:
exit(1)
indicates an error or abnormal termination, whileexit(0)
indicates successful completionExample
#include <stdio.h> #include <stdlib.h> int main() { // Some condition that causes an error if (error_condition) { printf("Encountered an error. Exiting...\n"); exit(1); // Indicates an error and terminates the program } printf("Program completed successfully.\n"); exit(0); // Indicates success and terminates the program }
Explanation: In this example, if
error_condition
is true, the program prints an error message and callsexit(1)
to terminate the program with an error status. If not, it prints a success message and callsexit(0)
to terminate the program successfully.
Summary
return 1
: Used in themain
function to indicate an error. The value1
is passed to the operating system.return -1
: Often used in functions to indicate an error. It's a non-standard way to return an error value to the caller.exit(1)
: Immediately terminates the program and passes an exit status to the operating system.exit(1)
indicates an error, whileexit(0)
indicates success.
By understanding these different ways to handle errors and terminate a program, you can write more robust and predictable C code.
Subscribe to my newsletter
Read articles from Circuit Tales directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
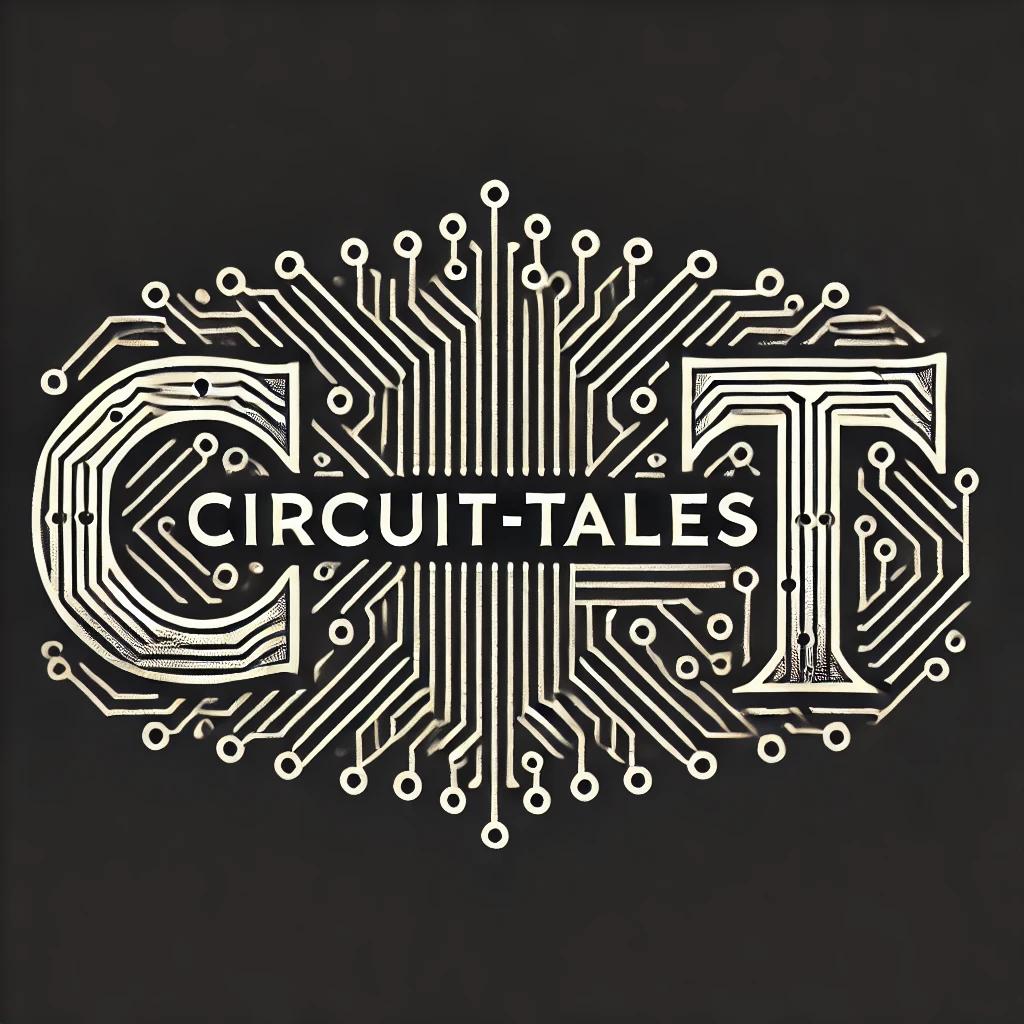
Circuit Tales
Circuit Tales
I am Ritesh K, a Software Embedded Engineer from India with a passion for developing IoT solutions and embedded systems. With extensive experience in C programming and a keen interest in the latest technology trends, I enjoy sharing my knowledge and insights through detailed tutorials and guides. Follow my blog, Circuit Tales, for regular updates on embedded systems, IoT, and programming tips.