Strings Manipulation -ch-02
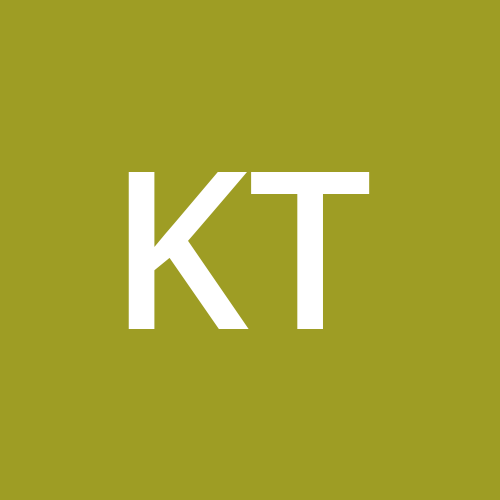
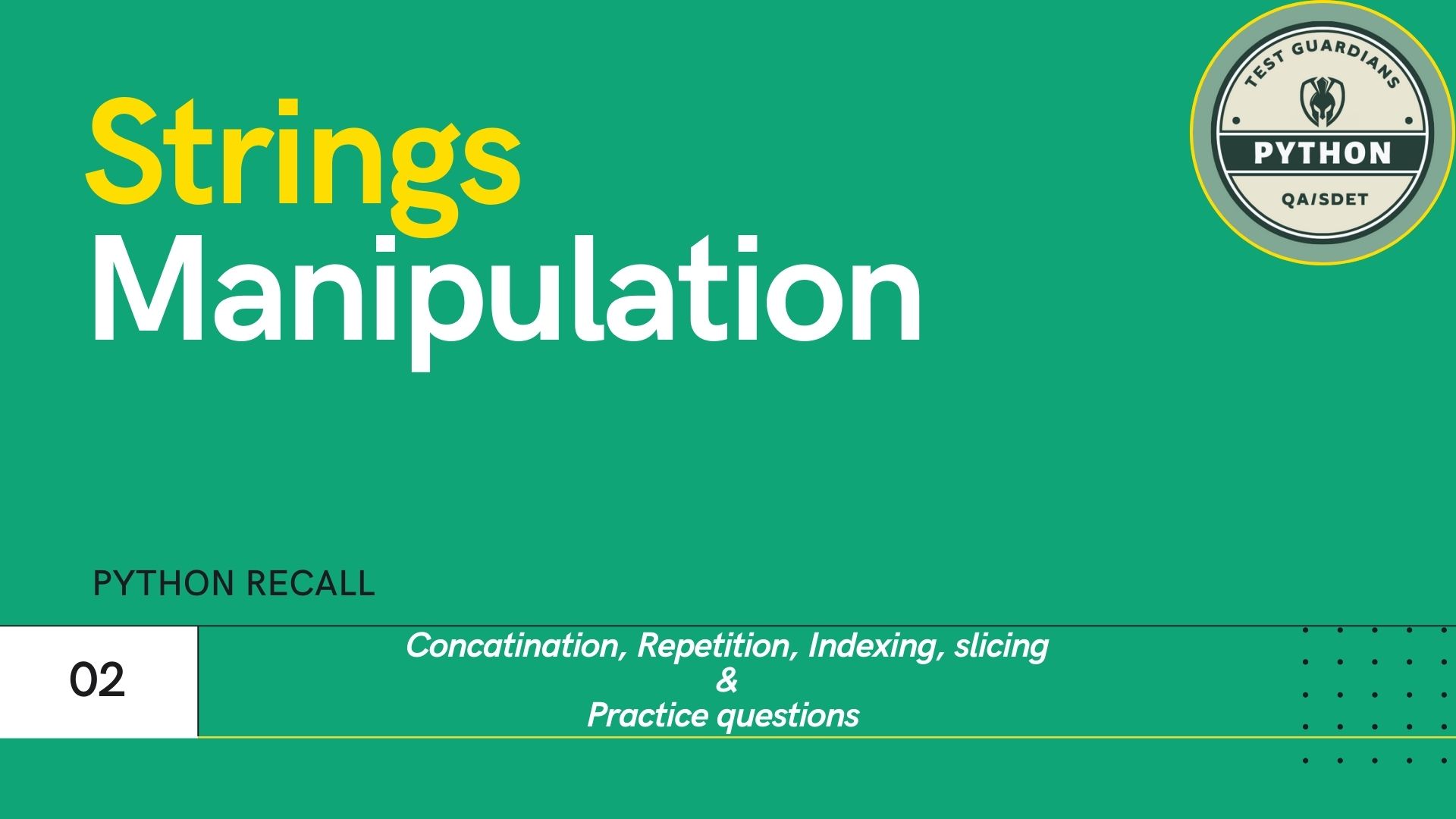
Concatenation :
concatenate using '+' operator
prefix = "al"
suffix = "pha"
print(prefix + suffix) # output : alpha
f-String:
helpful to print message with variable values.
syntax: f" your message {var_a} and print {var_b} "
prefix = "al"
suffix = "pha"
print(f"This is prefix: {prefix} and this is suffix: {suffix}")
#output: This is prefix: al and this is suffix: pha
Repetition:
Repeat string x no of times using '*' operator
prefix = "al"
suffix = "pha"
print((prefix + suffix)*2) # output : alphaalpha
Indexing:
starts from 0, access element using index value
prefix = "al"
suffix = "pha"
print(prefix[0]) # output : a
print(prefix[1]) # output: h
Slicing:
slice(stop)
blog_name = "python recall" sliced_string = blog_name[slice(4)] print(sliced_string) # output: pyth
slice(start, stop, step)
start at given index, then stop at given index while jumping by step value.
Step is optional parameter if don't want to jump
blog_name = "python recall" sliced_string = blog_name[slice(2,12,2)] print(sliced_string) # output: to ea
[:]
up to given index[:], while excluding given value.
eg: index[3] , will return 0,1,2 and excludes 3
blog_name = "python recall" sliced_string = blog_name[:3] print(sliced_string) # output: pyt
[-i]
starts from last index towards 0.
e.g: -1 is last index value of string
blog_name = "python recall" sliced_string = blog_name[-3] print(sliced_string) # output: a
Reverse [::-1]
Reverse the string and return
blog_name = "python recall" sliced_string = blog_name[::-1] print(sliced_string) # output: llacer nohtyp
String Functions
There is huge list of string functions available but these are few we should be able to keep top of mind.
capitalize, count, endswith, find, index, isalnum, isalpha, isdecimal, isdigit, isidentifier, islower, isnumeric, isspace, istitle, isupper, join, lower, partition, replace, split, startswith, strip, title, upper, sorted
We are going to use this code to learn all these functions.
book_name = "art of intrusion Version"
version = "v02"
price = "25"
discount="3.95"
publication_house = "pyhouse"
Function | code | Output | Note |
capitalize | book_name.capitalize() | Art of intrusion version | First letter is capitalize |
count | book_name.count(i) | 3 | |
endswith | book_name.endswith('ion') | True | If string ends with given value |
find | book_name.find('ion') | 13 | if not found, return -1 |
index | book_name.index('ion') | 13 | if does not exist, error |
isalnum | book_name.isalnum() | False | only a-z & numbers No-space, No-characters |
isalpha | book_name.isalpha() | False | a-z, A-Z, no space, no characters |
isdecimal | price.isdecimal() | True | only numbers with in 0-9 , no space, valid(254) invalid(456.32) |
isdigit | price.isdigit() | True | T if all numbers(124) F(Hello123, 2.52, ' ', 1/2) |
islower | book_name.islower() | False | T/F all lower? |
isnumeric | price.isnumeric() | True | all #, no space/char/float |
isspace | book_name.isspace() | False | all whitespace ? |
istitle | book_name.istitle() | False | all string titlecase ? |
isupper | book_name.isupper() | False | all char uppercase ? |
join | ' '.join( ['hello','python'] ) | hello python | join and return 1 string |
partition | book_name.partition('rus') | ('art of int', 'rus', 'ion Version') | partition at given value , first occurrence''' |
replace | book_name.replace('art','Art') | Art of intrusion Version | |
startswith | book_name.startswith('ar') | True | return if string starts with given value |
strip | book_name.strip() | art of intrusion Version | remove leading, trailing space |
upper | book_name.upper() | ART OF INTRUSION VERSION | all uppercase |
title | book_name.title() | Art Of Intrusion Version | all words title case, first char capital only |
sorted | sorted(publication_house) | ['e', 'h', 'o', 'p', 's', 'u', 'y'] | sorted list return |
sorted(publication_house, reverse=True) | ['y', 'u', 's', 'p', 'o', 'h', 'e'] | reverse sort |
you may find isalnum, isalpha, isdigit confusing, just to revise.
isalnum() e.g: abc123 isalpha() e.g: abc isdigit() e.g: 123
Subscribe to my newsletter
Read articles from Kunaal Thanik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
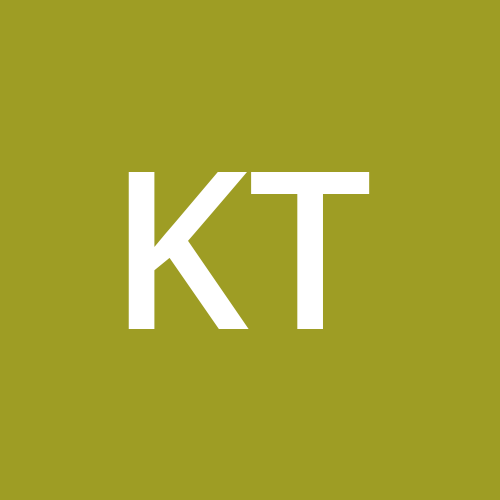