PRISMA: A Comprehensive Guide
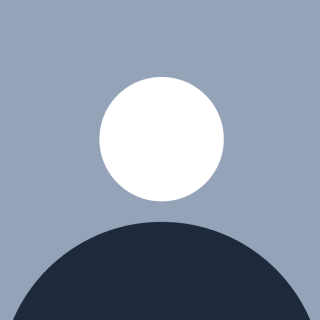
Getting Started with Prisma
Here’s a step-by-step guide to get started with Prisma:
Set Up a New Project
Firstly, create a new project and navigate to the project directory:
mkdir my-prisma-app
cd my-prisma-app
npm init -y
Install Prisma and Dependencies
npm install prisma typescript ts-node @types/node --save-dev
npx tsc --init
#in tsconfig.json
# Change `rootDit` to `src`
# Change `outDir` to `dist`
Initialize Prisma in project:
npx prisma init
This command creates a prisma
directory with a schema.prisma
file
Define Your Data Model
Edit the prisma/schema.prisma
file to define your data models. Here’s an example:
datasource db {
provider = "sqlite"
url = env("DATABASE_URL")
}
generator client {
provider = "prisma-client-js"
}
model User {
id Int @id @default(autoincrement())
firstName String
lastName String
email String @unique
}
Add the DATABASE_URL
in .env file
DATABASE_URL = "your_database_url"
Run Migrations
Generate and apply the migration to create the database tables:
npx prisma migrate dev --name <migration_name>#addTodo
This command creates the necessary tables in your database based on your schema.
Note: Rollback a Migration
To undo a migration, we can use the
migrate reset
command to reset your database:npx prisma migrate reset
Exploring database
If you have psql
installed locally, try to explore the tables that prisma
created for you.
psql -h localhost -d postgres -U postgres
How to Use Prisma Client for Database Management
Generate Prisma Client
Generate the Prisma Client based on your schema:
npx prisma generate
Use Prisma Client in Your Application
You can now use the Prisma Client to interact with your database. Create a file named index.ts
(if using TypeScript)
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
interface UserParams {
firstName:string,
lastName: string
}
async function createUser(email:string, {firstName, lastName}: UserParams ) {
const res = await prisma.user.create({
data:{
email,
firstName,
lastName
}
})
return res
}
createUser("example@gmail.com", {
firstName:"abc",
lastName:"abv"
})
Finally, run the project after completing all the steps above. This will generate the database tables based on your schema.
To visually browse the database
npx prisma studio
Subscribe to my newsletter
Read articles from Pawan S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Pawan S
Pawan S
Understanding technology.