Mastering Git: A Comprehensive Guide for Beginners
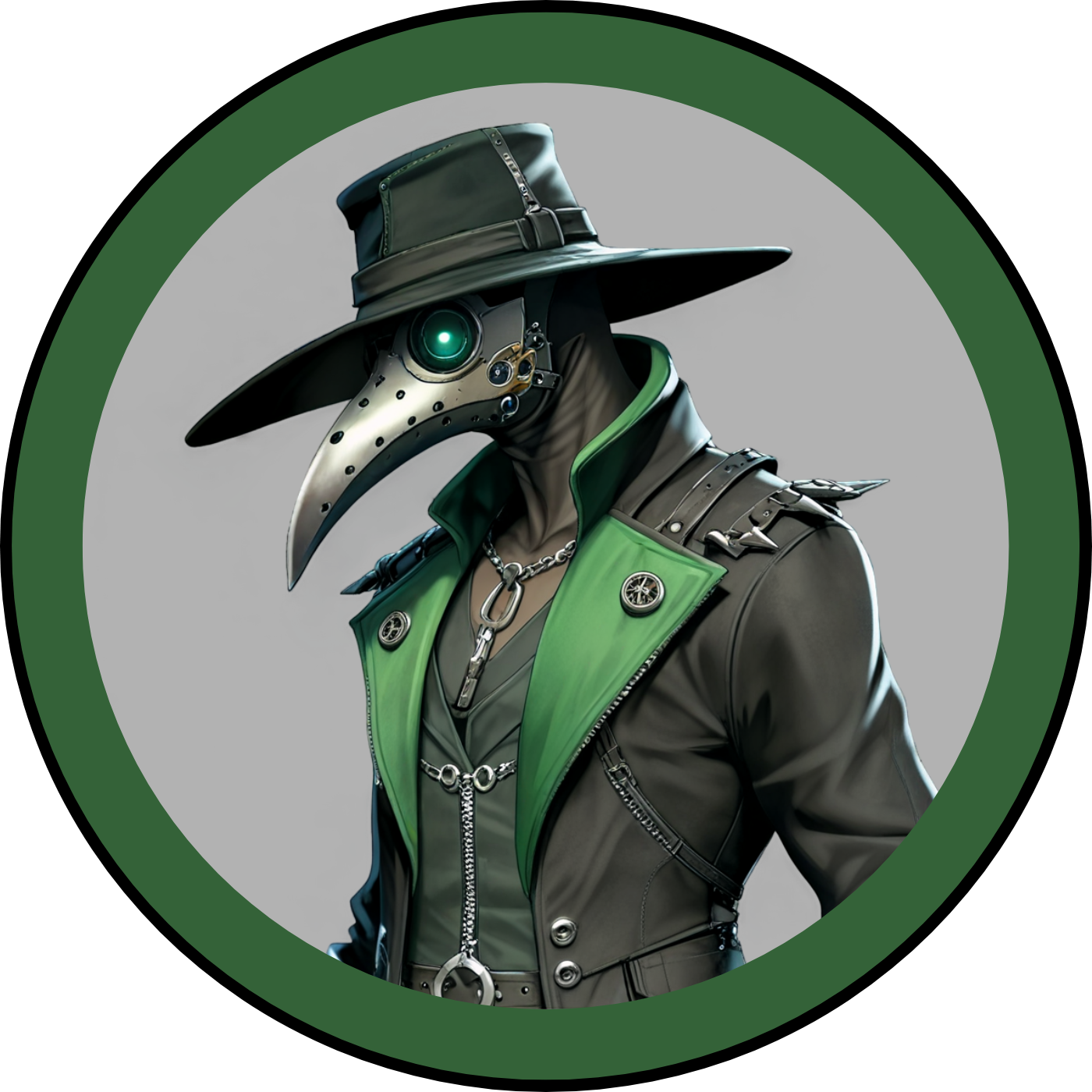

Git, a distributed version control system, has revolutionized software development, emerging as a preferred choice over alternatives like SVN and Mercurial due to its robust features and efficiency. This guide aims to provide a thorough understanding of Git, from basic commands to advanced techniques, helping you master this essential tool.
Understanding Git: The Basics
Since its inception by Linus Torvalds in 2005 for Linux kernel development, Git has significantly evolved, becoming a cornerstone in modern software development practices. It is designed to handle projects with remarkable speed and efficiency, ensuring data integrity and facilitating distributed, non-linear workflows, which means multiple developers can work on different features simultaneously without disrupting the main codebase.
Why Use Git?
Track Changes
Git meticulously tracks every modification in the codebase, allowing developers to follow the evolution of a project with precision. For instance, in a small project, this can help in swiftly pinpointing when a particular function was introduced or modified. This is invaluable during debugging, as it allows developers to identify and revert to previous states of the codebase if necessary.
Collaboration
With Git, multiple developers can work on the same project simultaneously, using tools like Slack or Microsoft Teams for communication without disrupting each other's progress. This decentralized approach to version control means that teams can collaborate more effectively, making it easier to manage large-scale projects and ensuring that everyone is on the same page.
Branching and Merging
Git's branching feature enables developers to diverge from the main line of development and experiment without risks. Branching allows developers to work on features or fixes in isolation, which can then be merged back into the main branch once they are complete and tested. This strategy not only keeps the main codebase stable but also encourages innovation and experimentation.
Branching Out
Branching is one of Git’s most powerful tools, providing a way to veer off the main project without disrupting it. Here’s how branching works in practice:
Feature Branches
Creating a new branch for each feature you're working on is a common strategy. This keeps your main branch clean and ensures that incomplete features don't affect the main codebase. For example, if you are adding a new authentication feature, you can create a branch named feature/authentication
and develop the feature independently of the main codebase.
Hotfix Branches
Hotfix branches are used for urgent fixes that need to be addressed immediately. These branches can be merged back into the main branch and the release branch to ensure the fix is included in the next release. For example, if a critical bug is found in production, a hotfix/critical-bug
branch can be created to address the issue quickly.
Branching Strategies
Different projects might adopt various branching strategies depending on their needs. Some common strategies include:
- Git Flow: A popular branching model that defines a strict branching strategy with specific branch types (e.g., feature, release, hotfix).
- GitHub Flow: A simpler model that uses a single main branch with feature branches created for new work. Merging into the main branch triggers a deployment.
- Trunk-Based Development: Developers commit to the main branch frequently, with feature branches being short-lived.
Merging and Conflicts
Merging combines changes from one branch into another, which can sometimes lead to conflicts. Here’s a step-by-step guide to resolving a merge conflict, ensuring smooth integration of changes:
- Identify the Conflict: Git will notify you of any conflicts that need to be resolved before the merge can be completed.
- Open the Files: Look for the conflict markers (
<<<<<<<
,=======
,>>>>>>>
) in the files. - Resolve the Conflict: Edit the files to resolve the differences between the conflicting changes.
- Mark as Resolved: Use
git add
to mark the conflict as resolved. - Commit the Merge: Complete the merge by committing the changes.
Conflicts are a natural part of collaborative development, and resolving them effectively ensures that all contributions are integrated smoothly. It's essential to communicate with your team during this process to understand the changes and avoid overriding important updates.
Advanced Git: Beyond the Basics
Git Rebase
Used for reordering or squashing commits, rebase can simplify a complex history. However, it should be used cautiously to avoid altering public history. Rebase is particularly useful when you want to maintain a linear project history. For example, if you have several small commits that could be combined into a single commit for clarity, you can use git rebase -i
to interactively rebase and squash those commits.
Git Stash
Stashing is handy for saving uncommitted changes temporarily. For example, if you need to switch branches but aren’t ready to commit, git stash
will save your work until you can return to it. This allows you to work on multiple features simultaneously without losing any progress. To stash your changes, simply use git stash
, and to apply them later, use git stash apply
.
Git Cherry-Pick
This command is useful for applying changes from one branch to another without merging the full branch. For instance, if a specific bug fix in one branch needs to be applied to another, cherry-picking allows you to select that particular commit. This is particularly useful in situations where you need to backport fixes to earlier versions of the software. Use git cherry-pick <commit-hash>
to apply the changes from a specific commit.
Git Bisect
Git Bisect is a powerful tool for finding bugs. It uses a binary search algorithm to identify the commit that introduced a bug. This can be incredibly useful when you have a large codebase and need to pinpoint the exact commit that caused an issue. To start a bisect session, use git bisect start
, mark the bad and good commits with git bisect bad
and git bisect good
, and Git will guide you through the process of testing and narrowing down the problematic commit.
Git Submodules
Git Submodules allow you to include external repositories within your repository. This is useful for managing dependencies and ensuring that specific versions of external projects are included in your project. For example, if your project depends on a library that is also under version control, you can add it as a submodule using git submodule add <repository-url>
and manage it within your main repository.
Git Hooks
Git Hooks are scripts that run automatically at specific points in the Git workflow. They can be used to enforce policies, automate tasks, and improve the development process. For example, you can use a pre-commit hook to run tests and linters before allowing a commit, ensuring that only high-quality code is committed. Hooks are stored in the .git/hooks
directory and can be customized to fit your workflow.
Best Practices for Using Git
Commit Often
Small, frequent commits can reduce conflicts and make it easier to locate problems. Each commit should represent a logical unit of work, making it easier to understand the history of changes. Frequent commits also make it easier to use tools like Git Bisect to track down bugs.
Write Meaningful Commit Messages
A good commit message should explain what the commit achieves and why, not just what has changed. For example, instead of saying "Fixed bug," a better message would be "Fixed null pointer exception in user login process." This provides context and helps other developers understand the purpose of the change. A well-written commit message typically consists of a short summary followed by a detailed explanation if necessary.
Use Branches Effectively
Branches should be used to isolate work on new features, bug fixes, or experiments. This keeps the main branch stable and allows you to develop and test changes in isolation before merging them into the main branch. Following a branching strategy that suits your project can help maintain a clean and organized codebase.
Regularly Sync with the Main Branch
To avoid large, complex merges, regularly sync your feature branches with the main branch. This helps keep your branch up-to-date with the latest changes and reduces the likelihood of conflicts. Use git fetch
and git rebase
or git merge
to incorporate changes from the main branch into your feature branch.
Clean Up After Yourself
Once a branch has been merged and is no longer needed, delete it to keep your repository clean and organized. This prevents clutter and makes it easier to navigate the repository. Use git branch -d <branch-name>
to delete a branch locally and git push origin --delete <branch-name>
to delete it remotely.
Using Git in Teams
Implementing a workflow that includes code reviews and regular communication is crucial for team projects. This ensures that changes are vetted and that the team remains aligned. Here are some tips for using Git effectively in a team:
Code Reviews
Regularly review code to ensure quality and consistency. Tools like GitHub and GitLab offer integrated code review features that make it easy to comment on and approve changes before they are merged. Code reviews help catch potential issues early, improve code quality, and facilitate knowledge sharing among team members.
Continuous Integration
Set up continuous integration (CI) pipelines to automatically test and build your code. This helps catch issues early and ensures that the main branch is always in a deployable state. CI tools like Jenkins, Travis CI, and GitLab CI/CD can be integrated with your Git repository to automate the testing and deployment process.
Branch Protection
Use branch protection rules to prevent accidental merges into the main branch without code review and passing tests. This ensures that all changes are thoroughly reviewed and tested before being integrated into the main branch. Branch protection rules can be configured in platforms like GitHub, GitLab, and Bitbucket.
Communication
Effective communication is key to successful collaboration. Use tools like Slack, Microsoft Teams, or Discord to stay in touch with your team, discuss changes, and resolve conflicts. Regular meetings and updates can help keep everyone aligned and ensure that the project is progressing smoothly.
Documentation
Maintain comprehensive documentation for your project, including coding standards, branching strategies, and workflow guidelines. This helps new team members get up to speed quickly and ensures that everyone follows the same practices. Use tools like Markdown for easy-to-read and maintainable documentation.
Tools That Enhance Git
Numerous tools integrate with Git to streamline workflow. For example, continuous integration tools can automate tests and builds, enhancing code quality. Here are some popular tools that can enhance your Git workflow:
- GitHub: A platform that offers Git repository hosting along with additional features like pull requests, issue tracking, and project management tools. GitHub is widely used in the open-source community and provides a robust set of features for managing repositories and collaborating on code.
- GitLab: Similar to GitHub, GitLab provides Git repository hosting along with CI/CD pipelines, making it a powerful tool for DevOps. GitLab offers a comprehensive suite of tools for managing the entire software development lifecycle, from planning and development to testing and deployment.
- Bitbucket: Another Git repository hosting service that integrates well with Jira for project tracking and management. Bitbucket offers features like pull requests, branch permissions, and built-in CI/CD pipelines, making it a popular choice for teams using the Atlassian suite of tools.
- Sourcetree: A graphical user interface (GUI) for Git that simplifies complex Git operations, making it easier for beginners to get started with Git. Sourcetree provides a visual representation of your repository, branches, and commits, making it easier to understand the state of your project and perform common Git tasks.
- VS Code: Visual Studio Code (VS Code) is a popular code editor with built-in Git integration. It allows you to manage your Git repositories directly from the editor, making it easy to stage changes, commit, and push updates. VS Code also offers a wide range of extensions that enhance Git functionality, such as GitLens for detailed commit history and code authorship.
- Jenkins: A widely used CI tool that can be integrated with Git to automate the build and testing process. Jenkins supports a wide range of plugins and can be configured to trigger builds based on changes in your Git repository, ensuring that your code is always tested and ready for deployment.
- Travis CI: A cloud-based CI service that integrates seamlessly with GitHub repositories. Travis CI automates the testing and deployment process, making it easy to set up and maintain a CI pipeline for your projects. With Travis CI, you can define your build process in a
.travis.yml
file and let Travis handle the rest. - GitKraken: A powerful Git GUI client that offers a visually appealing interface and a range of features to simplify Git workflows. GitKraken provides tools for managing branches, resolving conflicts, and visualizing commit history, making it a popular choice for developers looking for a user-friendly Git client.
Conclusion
Mastering Git is a rewarding journey that enhances your capabilities as a developer. Continuously seek out new learning opportunities and engage with community forums to further your understanding. Git's versatility and power make it an indispensable tool for modern software development, and becoming proficient with Git will significantly improve your development workflow.
def engage_with_blog(reaction):
if reaction == 'happy':
leave_comment()
elif reaction == 'loved':
share_with_friends()
elif reaction == 'amazed':
react_with_emoji('😲')
else:
print('Thanks for reading!')
Subscribe to my newsletter
Read articles from Surgical directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
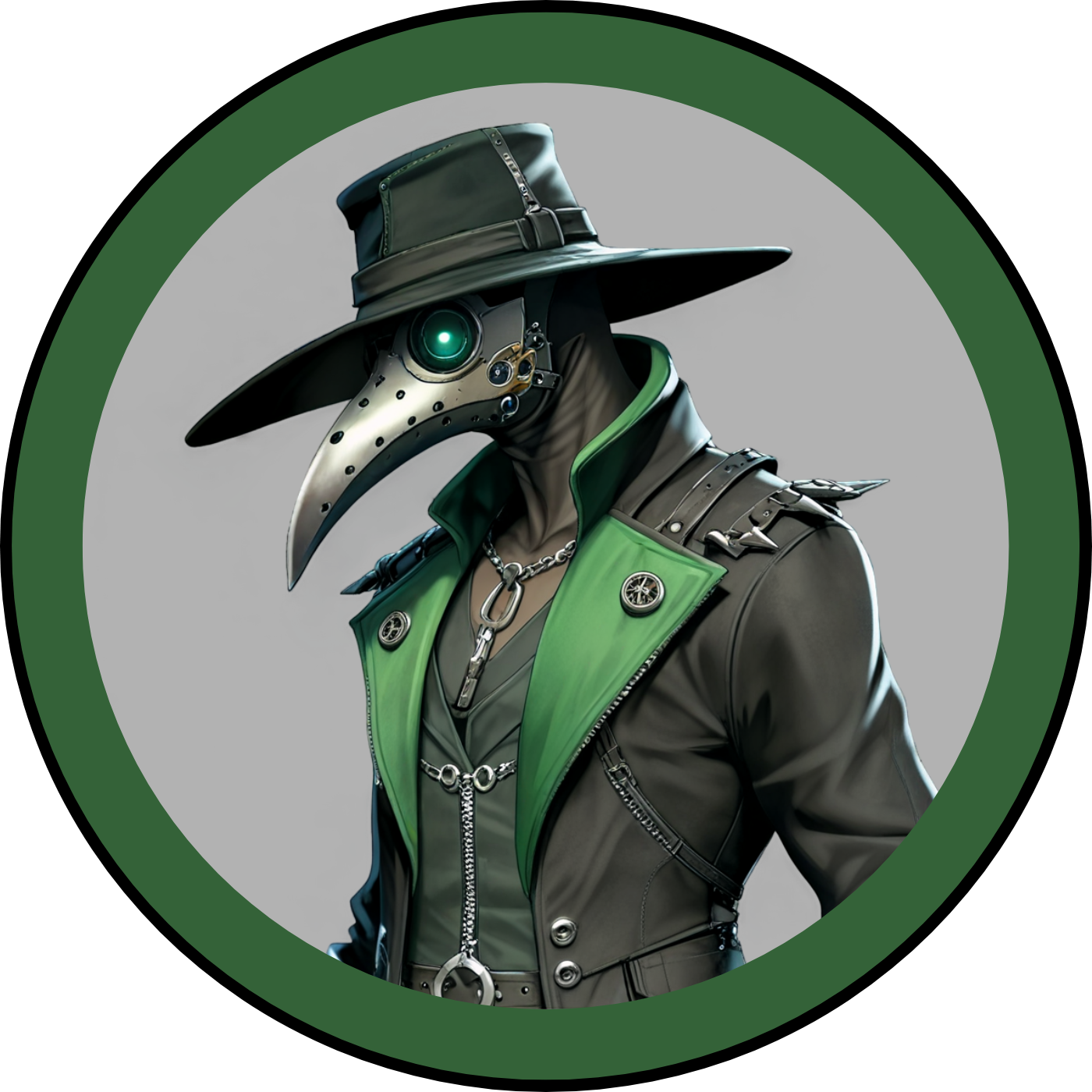
Surgical
Surgical
a Multifaceted professional specializing in IT Support, Web Design, System Management, Software Development, and Hosting Solutions. With a deep-rooted passion for technology and its transformative potential, I have dedicated myself to crafting beautiful, functional, and user-centric digital experiences.