Exploring JSON: The Backbone of Data Exchange
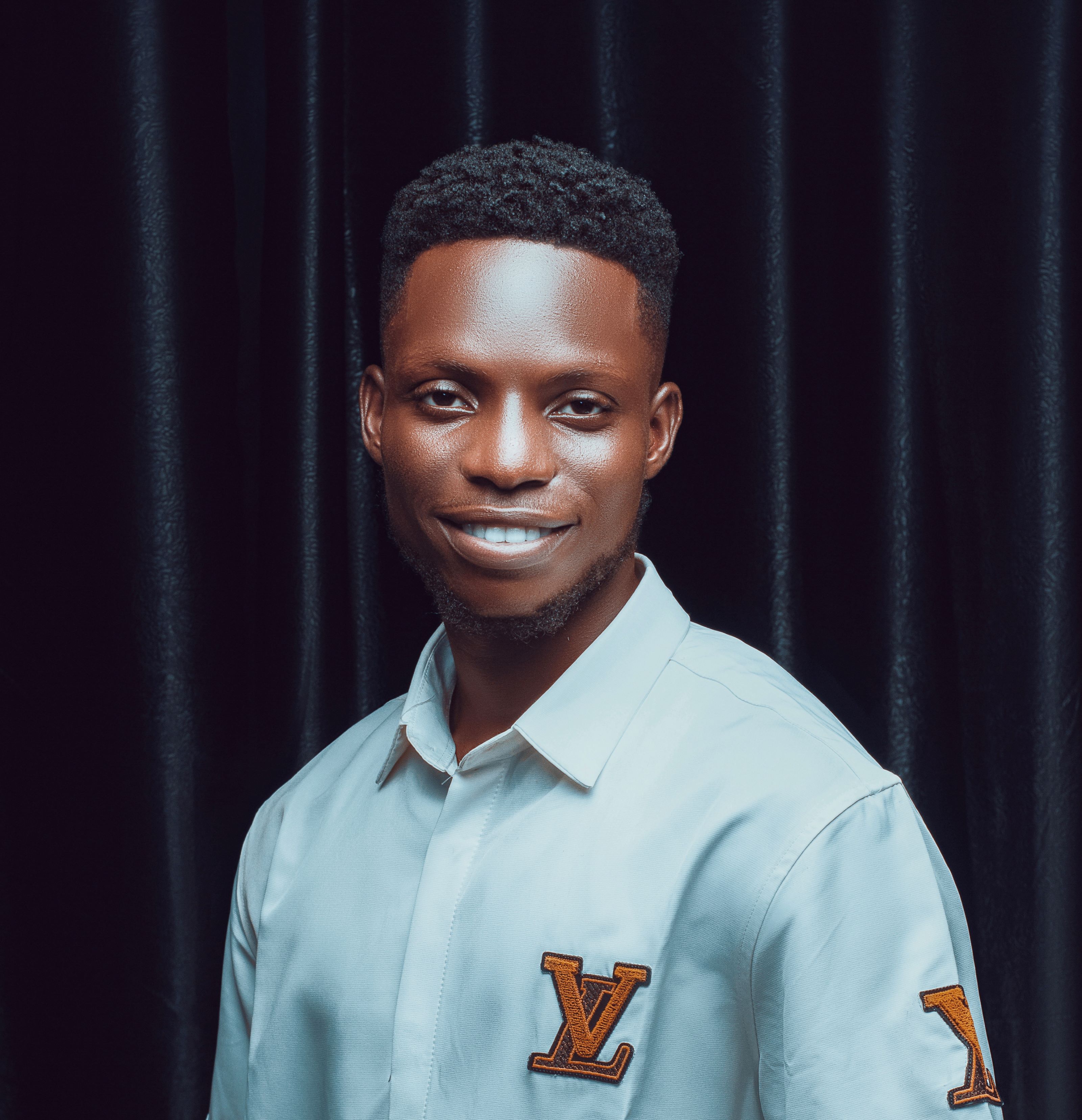
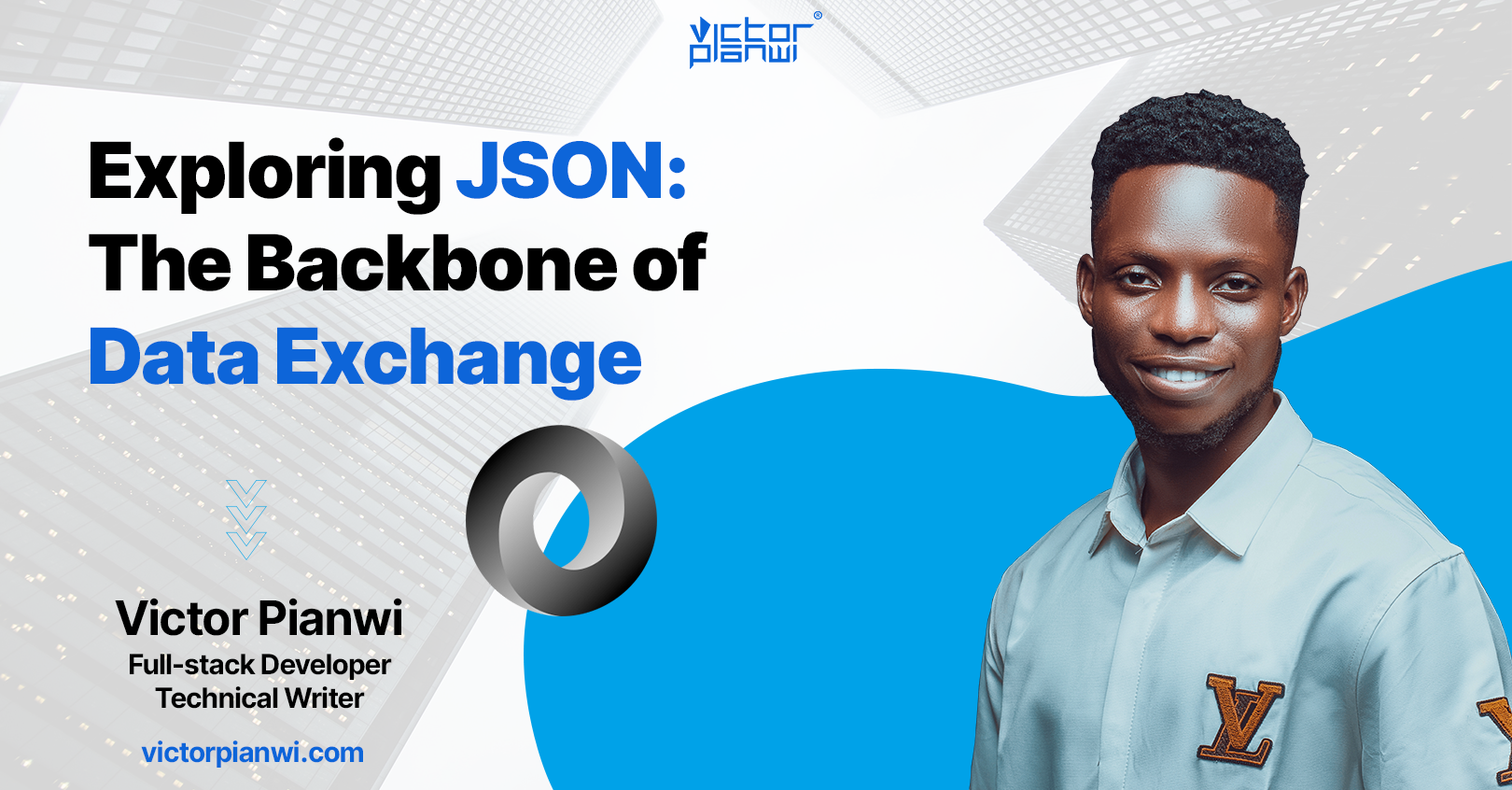
JavaScript Object Notation (JSON) is a key part of modern web development, acting as a lightweight and efficient data interchange format. From APIs to configuration files, JSON is everywhere because it's simple and easy to read. Let's look at what JSON is, why it's so popular, and how it's used in different situations.
What is JSON?
JSON, short for JavaScript Object Notation, is a lightweight data-interchange format that's easy for humans to read and write, and easy for machines to parse and generate. Despite its name, JSON is a language-independent format. It is based on a subset of the JavaScript Programming Language Standard ECMA-262 3rd Edition - December 1999. JSON is a text format that is completely language-independent but uses conventions familiar to programmers of the C family of languages, which includes C, C++, C#, Java, JavaScript, Perl, Python, and many others.
JSON Syntax
JSON's syntax is simple and comprises key-value pairs. Here are the basic rules:
Objects: Enclosed in curly braces
{}
and contain key-value pairs. Keys are strings, and values can be strings, numbers, arrays, objects, booleans, ornull
.{ "name": "John Doe", "age": 30, "isStudent": false, "courses": ["Math", "Science"], "address": { "street": "123 Main St", "city": "Anytown" } }
Arrays: Enclosed in square brackets
[]
and contain a list of values.["Apple", "Banana", "Cherry"]
Values: Must be one of the following data types: string, number, object, array, true, false, or null.
{ "stringExample": "Hello, World!", "numberExample": 42, "booleanExample": true, "nullExample": null, "arrayExample": [1, 2, 3], "objectExample": {"key": "value"} }
The Importance of JSON in Development
Data Interchange: JSON is the backbone of data exchange between a server and a web application. APIs (Application Programming Interfaces) often use JSON to format the data sent and received. Its lightweight nature ensures quick transmission and efficient processing, making it ideal for web applications.
Ease of Use: JSON's syntax is easy to understand, reducing the learning curve for developers. This simplicity allows developers to parse and manipulate JSON data, enhancing productivity quickly.
Compatibility: JSON is language-agnostic, meaning it can be used with almost any programming language. This compatibility makes it a versatile choice for various applications, from web development to data storage and configuration files.
Readability: JSON is human-readable, which aids in debugging and understanding the structure of the data. This readability also makes it easier to document and maintain code.
Lightweight: Compared to XML, another popular data interchange format, JSON is more lightweight. This means it uses less bandwidth, which is crucial for web applications that need to be fast and efficient.
Practical Applications of JSON
APIs and Web Services: JSON is the standard format for RESTful APIs. When a client requests data from a server, the server responds with JSON, making it easy to work with in JavaScript and other languages. For example, a weather API might return the following JSON response:
{ "location": "New York", "temperature": 22, "conditions": "Cloudy" }
Configuration Files: JSON is commonly used for configuration files in many software applications due to its simplicity and ease of parsing. For example, configuration files in web frameworks like Angular and React often use JSON. A configuration file might look like this:
{ "appName": "MyApp", "version": "1.0.0", "settings": { "theme": "dark", "language": "en" } }
Data Storage: JSON is used in databases like MongoDB, which stores data in a JSON-like format called BSON (Binary JSON). This NoSQL database allows for flexible, schema-less data structures, making it ideal for applications with evolving data requirements.
Front-End Development: JSON is widely used in front-end development to manage application state, pass data between components, and interact with APIs. JavaScript's native support for JSON makes it seamless to integrate with front-end frameworks like React, Vue, and Angular.
Server-Side Processing: Server-side languages like Node.js, Python, and Ruby can easily parse JSON data, making it an excellent choice for server-side processing and handling client requests.
Working with JSON
Parsing JSON: In JavaScript, you can parse JSON using
JSON.parse()
. For example:const jsonString = '{"name": "John", "age": 30}'; const jsonObject = JSON.parse(jsonString); console.log(jsonObject.name); // Outputs: John
Stringifying JSON: To convert a JavaScript object into a JSON string, use
JSON.stringify()
:const jsonObject = { name: "John", age: 30 }; const jsonString = JSON.stringify(jsonObject); console.log(jsonString); // Outputs: {"name":"John","age":30}
Best Practices for Working with JSON
Validate JSON: Always validate JSON data to ensure it is correctly formatted. Tools and libraries are available to help with validation, such as JSONLint and AJV.
Handle Errors Gracefully: Implement error handling for JSON parsing and serialization to prevent application crashes and provide meaningful error messages to users.
Minimize JSON Payloads: To optimize performance, minimize the size of JSON payloads by removing unnecessary data and using shorter key names when possible.
Secure Your Data: Be mindful of security when working with JSON data. Sanitize inputs and outputs to prevent injection attacks and other vulnerabilities.
Use JSON Schema: JSON Schema is a powerful tool for validating the structure of JSON data. It helps ensure data consistency and integrity by defining the expected format and types for JSON objects.
Conclusion
JSON is now a crucial part of modern web development, allowing for efficient data exchange, configuration management, and storage solutions. Its simplicity, readability, and compatibility with many programming languages make it essential for developers. By understanding and using JSON effectively, you can create robust, scalable, and maintainable web applications that meet today's digital needs. Whether you're working on front-end development, server-side processing, or database management, JSON is key to unlocking the full potential of modern web development.
Subscribe to my newsletter
Read articles from Victor Pianwi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
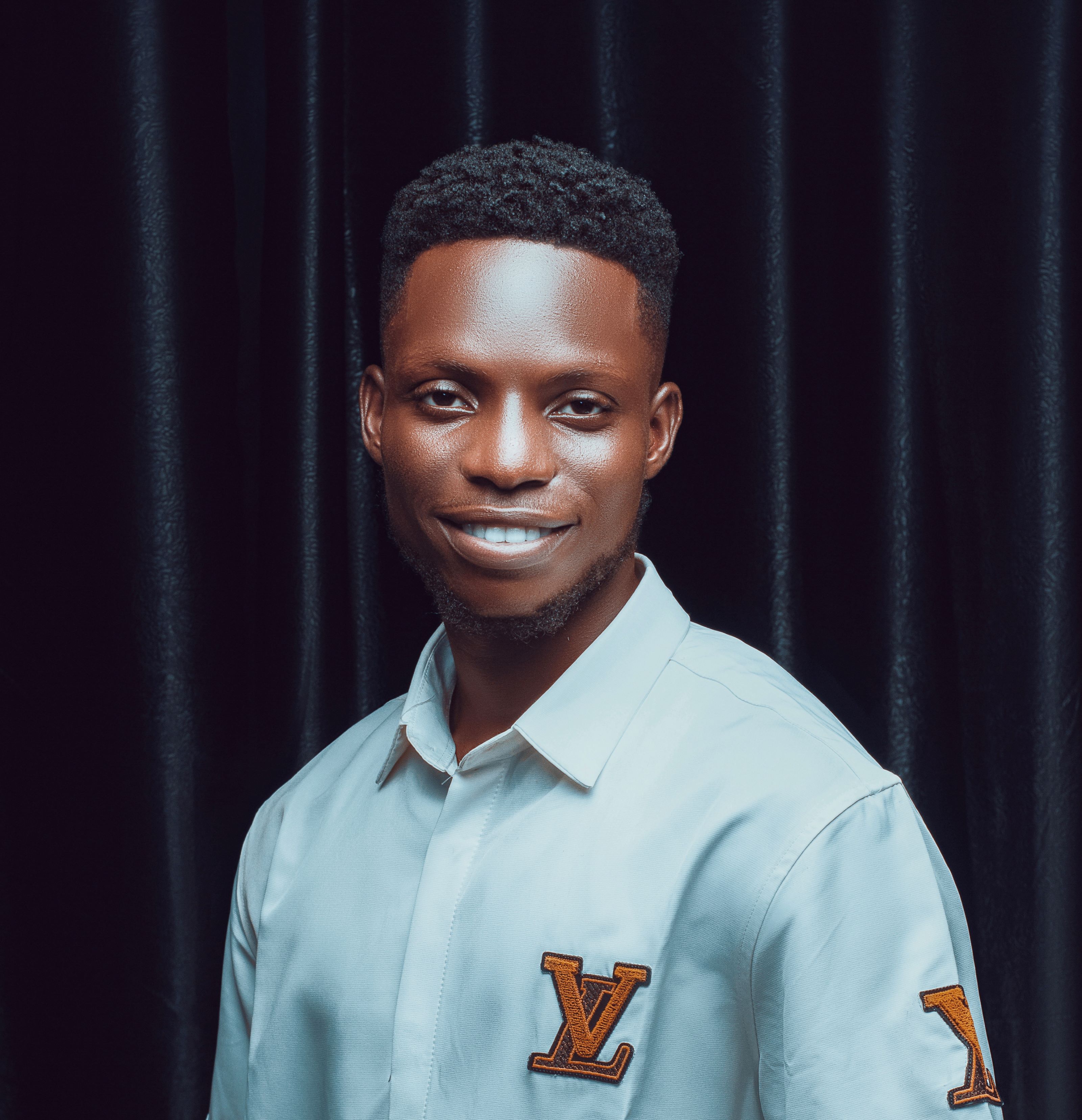
Victor Pianwi
Victor Pianwi
I am a passionate full-stack developer with two years of hands-on experience crafting and maintaining dynamic and responsive solutions, with expertise in web, app and smart contract development, a technical writer and a community manager focused on empowering the community and individuals. I write well-detailed technical articles on self-growth, development(website, application, decentralized application and smart contract) and blockchain.