How to Implement African's Talking SMS API
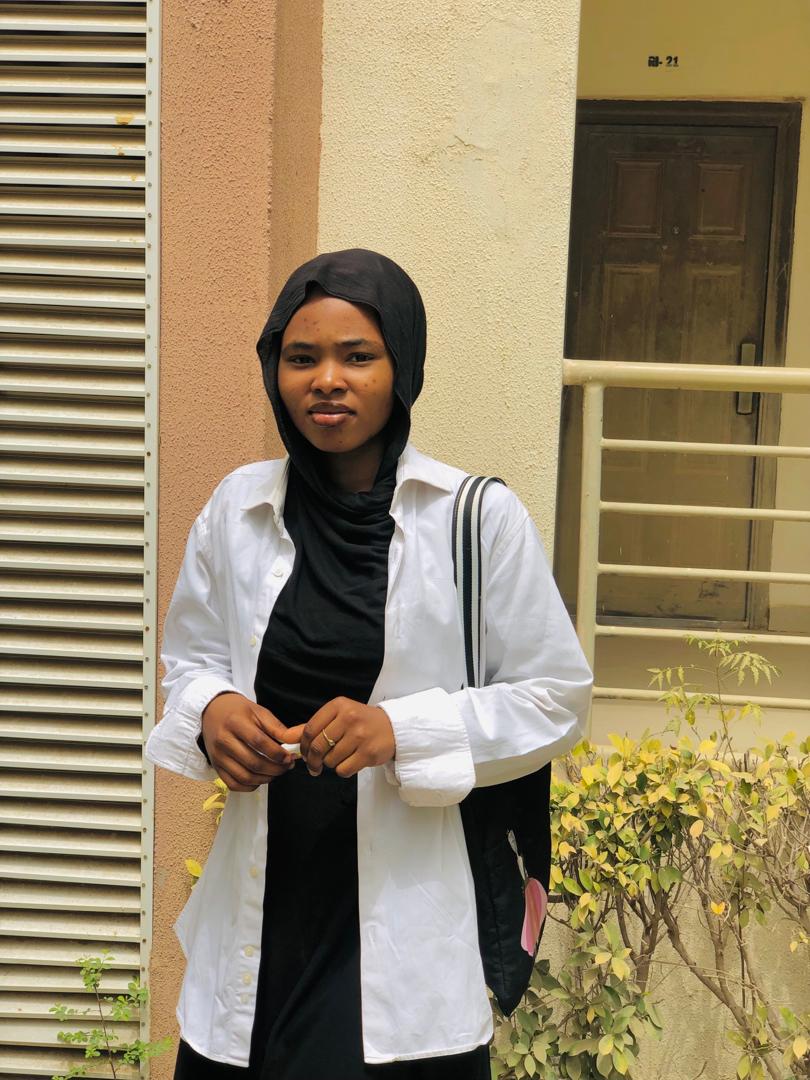
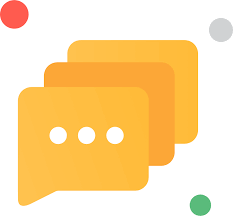
Introduction
Hello Devs,
Hope you're doing well. I recently participated in a hackathon event hosted by Africa's Talking. It was my first time participating in a hackathon, and I'm proud to have seen it through. The hackathon focused on AgriTech, and one of the criteria for winning was to include at least one of Africa's Talking APIs in the solution.
For our solution, we decided to use the Africa's Talking SMS API. Integrating this API was quite a challenge, but I learned a lot from the experience. To help others, I decided to create a simple and easy-to-understand article on how to implement the Africa's Talking API as a front-end developer with little to no back-end knowledge.
This article will guide you through implementing the Africa's Talking API from a front-end developer's perspective.
Setting Up Your Backend
As a front-end developer, I assume you already have your interface up and running—whether it's a form or just a single input field. Now, you're ready to call the API, right? Well, not quite. Unfortunately, you can't directly interact with the API from your front-end React or Vue application. We'll need to set up a backend.
Create a Backend Directory: In your React project folder, create a new directory for the backend at the root level. You can name it
server
or whatever you prefer.Initialize NPM: Open your terminal, navigate to the backend directory, and type
npm init
. This will initialize NPM. After that, install the necessary dependencies:npm install express africastalking dotenv body-parser
Dependencies:
express
: A web application framework for Node.js.africastalking
: The Africa's Talking Node.js SDK.dotenv
: A zero-dependency module that loads environment variables from a .env file.body-parser
: Middleware to parse incoming request bodies in a middleware before your handlers.
Create sendSms.js: Create a file named
sendSms.js
(or whatever you prefer) in your backend directory.Set Up Your Server: Add the following code to
sendSms.js
to set up your server and handle POST requests:import express from 'express'; import bodyParser from 'body-parser'; import AfricasTalking from 'africastalking'; import dotenv from 'dotenv'; dotenv.config(); const app = express(); const port = process.env.PORT || 3000; const africasTalking = AfricasTalking({ apiKey: process.env.AFRICASTALKING_API_KEY, username: process.env.AFRICASTALKING_USERNAME, }); app.use(bodyParser.json()); // Middleware for handling CORS app.use((req, res, next) => { res.header('Access-Control-Allow-Origin', '*'); res.header('Access-Control-Allow-Methods', 'GET, POST, OPTIONS'); res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization'); if (req.method === 'OPTIONS') { return res.sendStatus(200); } next(); }); app.post('/send-sms', async (req, res) => { const { to, message } = req.body; try { const result = await africasTalking.SMS.send({ to, message, from: process.env.SENDER_ID, }); res.status(200).json({ status: 'success', data: result }); console.log("success", res.status); } catch (error) { console.log("error:", error); res.status(500).json({ status: 'error', message: error.message }); } }); app.listen(port, () => { console.log(`Server running on port ${port}`); });
Explanation:
dotenv.config(): This line loads environment variables from a
.env
file intoprocess.env
. This is useful for storing sensitive information like API keys securely.africasTalking: This initializes the Africa's Talking SDK with your API key and username, which are stored in environment variables. This SDK will be used to send SMS messages.
CORS Middleware: This middleware handles Cross-Origin Resource Sharing (CORS), which is crucial when your front-end application (running on a different domain or port) needs to communicate with your backend server. It allows your backend to accept requests from any origin (
'*'
)./send-sms Route: This route handles POST requests to send SMS messages using the Africa's Talking API. When a request is received at this endpoint, it extracts the
to
(recipient's phone number) andmessage
from the request body, sends an SMS using Africa's Talking SDK, and returns the result.app.listen(port, () => { ... }): This line starts the Express server and makes it listen for incoming requests on the specified port. The
port
is either taken from an environment variable or defaults to3000
if not provided. The callback function insideapp.listen
is executed once the server starts successfully, logging a message to the console.
By including app.listen
, you ensure that your backend server is actively listening for incoming HTTP requests, ready to handle them as defined by your routes (like the /send-sms
route).
Logging into Africa's Talking Dashboard
Create an Account: Go to Africa's Talking and create an account or log in.
Accessing the Sandbox Environment
- Go to the Sandbox App: After logging in, you will see the dashboard. Click on the "Go To Sandbox App" button.
Getting Your API Key
Navigate to the Settings
- In the sandbox dashboard, go to the "Settings" section on the left sidebar.
Generate Your API Key
- In the settings section, find the API key area. Click on "Generate New API Key" to get your unique API key. Copy this key as you'll need it for authentication in your application.
Launching the Simulator
Accessing the Simulator
In the sandbox dashboard, find the "Launch Simulator" option in the left sidebar and click on it.
Using the Simulator
- The simulator allows you to test sending and receiving messages. You can simulate sending SMS, voice, and USSD requests. Use the API key generated in the previous step to authenticate your requests.
Creating/Getting Shortcodes
Navigate to the SMS Section
- In the sandbox dashboard, click on the "SMS" section from the left sidebar.
View or Create Shortcodes
In the SMS section, you can see existing shortcodes or request a new one. Click on "Create New Shortcode" if you need a new one
.
Fill Out the Shortcode Request Form
Fill out the required information for the shortcode request, such as the desired shortcode, country, and any additional details. Submit the form and wait for approval
Connecting Front-End to Back-End
Now, let's connect your front-end application to the backend using Axios or another HTTP client. Here's an example using Axios:
import axios from 'axios';
import { useState } from 'react';
const SendMessage = () => {
const [phoneNumber, setPhoneNumber] = useState('');
const [message, setMessage] = useState('');
const sendMessage = async () => {
try {
const response = await axios.post('http://localhost:3000/send-sms', {
to: phoneNumber,
message,
});
console.log('SMS sent successfully:', response.data);
} catch (error) {
console.error('Error sending SMS:', error);
}
};
return (
<div>
<input
type="text"
placeholder="Phone Number"
value={phoneNumber}
onChange={(e) => setPhoneNumber(e.target.value)}
/>
<textarea
placeholder="Message"
value={message}
onChange={(e) => setMessage(e.target.value)}
/>
<button onClick={sendMessage}>Send SMS</button>
</div>
);
};
export default SendMessage;
Testing Your Application
Run the Backend Server: Make sure your backend server is running on port 3000 or whichever port you've set.
Launch the Simulator: Use Africa's Talking simulator and add the number you want to test with.
Run Tests: Ensure the routes and the
sendSms.js
file are working correctly. You can use tools like Postman to test the endpoints.
That's it! You've successfully integrated Africa's Talking API as a front-end developer. With this setup, you can send SMS messages from your application. Happy coding!
I hope this guide was helpful. If you have any questions or need further clarification, feel free to leave a comment. Keep coding and stay awesome! By the way, I was the first runner-up, so you know this advice is coming from someone who almost won!
Subscribe to my newsletter
Read articles from Hassana Abdullahi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
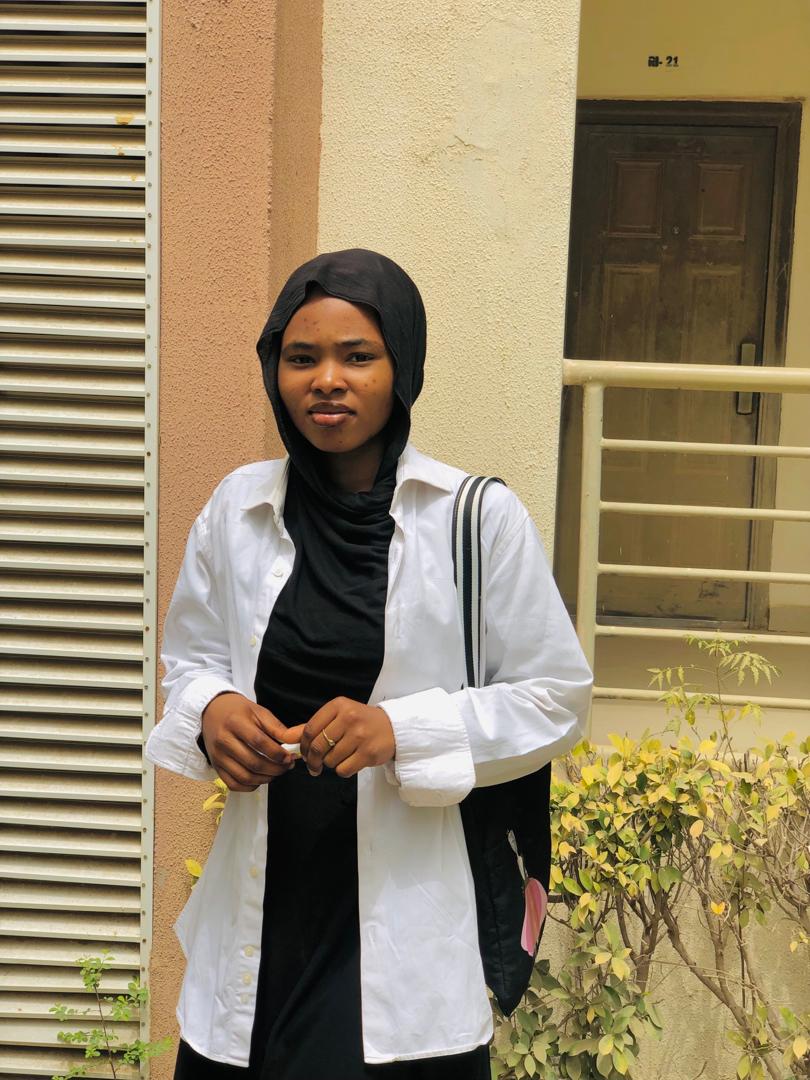
Hassana Abdullahi
Hassana Abdullahi
Hey everyone, I'm Hassana Abdullahi, a recently graduate of computer science and a passionate front-end developer with a case of app-building wanderlust! While I love the world of web development, i also like the world of Mobile development. let's just say, somethings wouldn't fly on a browser 😂. And I also enjoy writing sometimes, I am not consistent but hey, don't judge. P.S. I am also a proud hijabi techie lady who believes style and code can go hand-in-hand. Stay tuned for some insights on juggling the tech world with a touch of flair! ✨