Beginner's Guide to Using Props in React
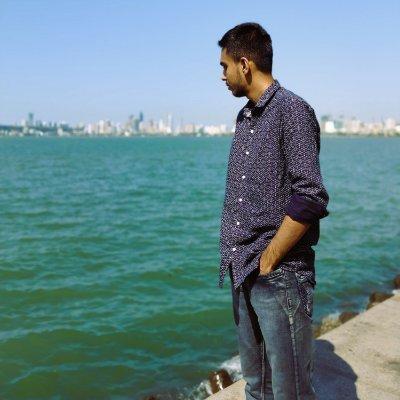
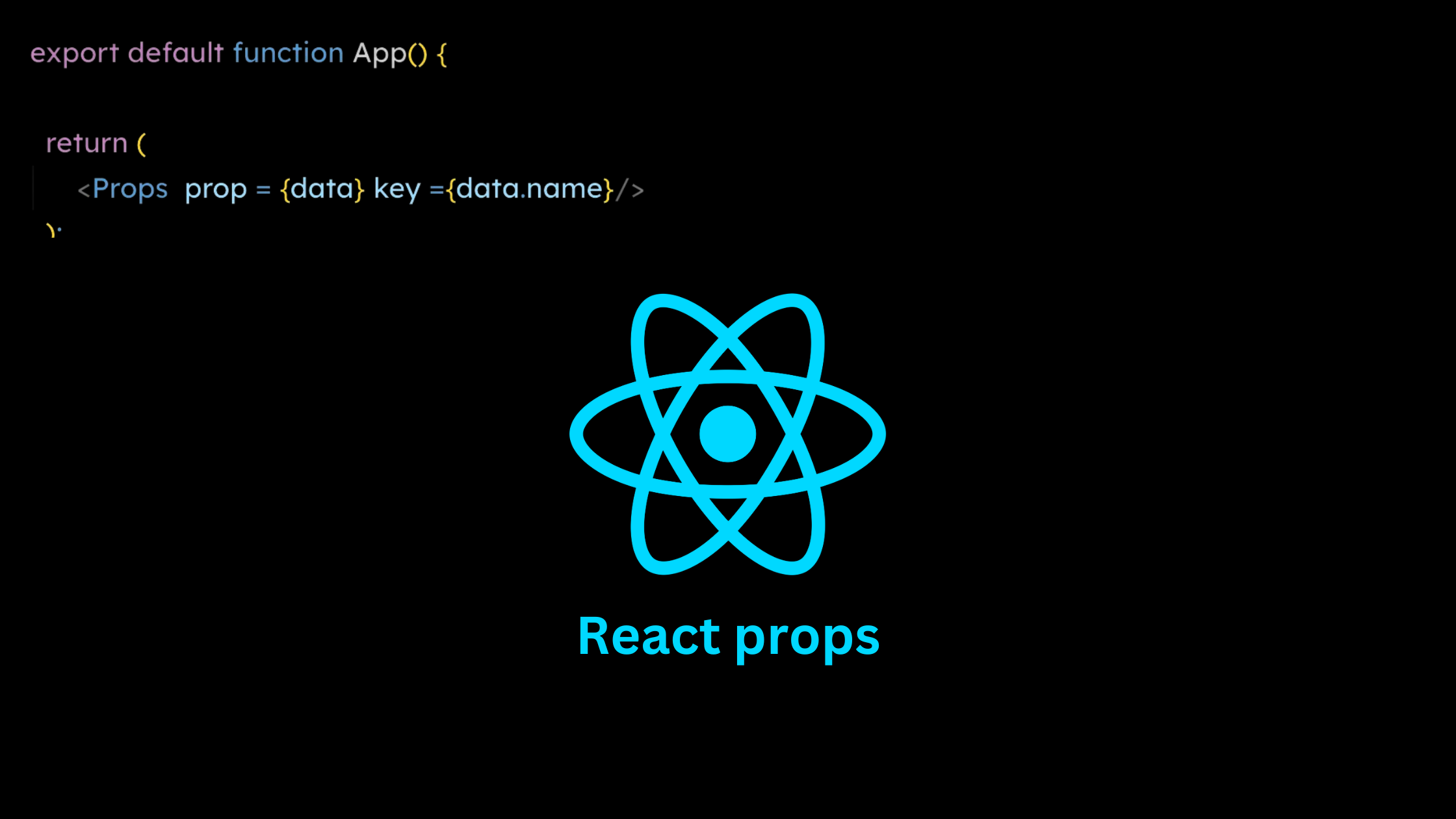
What are props-
Props, short for properties, are a fundamental concept in React that enable components to communicate with each other. They act like channels, allowing data to flow from a parent component to its child components in a unidirectional manner. This flow of data is essential for building dynamic and interactive user interfaces. Props not only allow you to pass data but can also be used to pass functions. This means you can trigger functions in a parent component from a child component, which is particularly useful for handling events and updating state.
How to use props-
Props in React can be passed from a parent component to a child component. In the child component, the prop has to be accepted, and then it can be used wherever you want in the function. This is a key feature of React that allows for dynamic and reusable components.
const data =
{
name: "i am a raect prop",
}
export default function App() {
return (
<Props prop = {data} key ={data.name}/>
);
}
function Props ({prop}) {
return (
<h1>{prop.name}</h1>
);
}
In this example, we start by creating a data object with a name property that has the value "i am a react prop". Next, we define the main App component and use export default to make it available for use in other files. Inside the App component, we render the Props component and pass the data object to it as a prop named prop. The Props component receives this prop object as an argument, and it displays the name property of the prop object inside an h1 element. This demonstrates how data is passed from a parent component (App) to a child component (Props) using props in React.
Immutability of props-
Props in React are immutable. This means that a prop cannot be manipulated or changed by the child component that receives it. The child component can only read and use the props but cannot modify them. This immutability ensures that data flow between components remains predictable and unidirectional, which is a core principle of React.
Passing elements as prop-
In React, props aren't limited to simple data types like strings, numbers, or objects. You can also pass elements as props, which can be incredibly powerful for creating dynamic and reusable components. This allows you to pass JSX elements from a parent component to a child component, enabling the child component to render those elements as part of its output.
const data =
{
name: "i am a react prop",
element: "i am element prop"
}
export default function App() {
return (
<Props prop = {data} elements={<Elementprop element={data}/>} key ={data.name}/>
);
}
function Props ({prop, elements}) {
return (
<>
<h1>{prop.name}</h1>
{elements}
</>
);
}
function Elementprop({element}) {
return <h1>{element.element}</h1>
}
In the example, the parent component (App) passes a data object and an Elementprop component instance as props to the Props component. The Props component receives these props, displaying the name property from the data object and rendering the Elementprop component using the elements prop. The Elementprop component, in turn, receives the data object and displays its element property. This demonstrates how you can pass elements as props in React, allowing for dynamic and reusable components by rendering JSX elements passed from parent to child components.
Children prop-
The children prop in React is a special prop, automatically passed to every component. It allows you to pass reusable components as children, which can be used multiple times throughout your application. This is particularly useful for maintaining consistency and reducing redundancy. For example, consider a button component used in various places within your app. By using the children prop, you can ensure all buttons have the same look and feel, making your UI more consistent and easier to manage.
const data =
{
name: "i am a react prop",
};
const className = {
backgroundColor:"#fcc419",
width: "130px",
height:"30px",
};
function Button({children}) {
return <button style={className}> {children}</button>
}
export default function App() {
return (
<>
<Props prop = {data} key ={data.name}/>
<Button>Children prop 1</Button>
</>
);
}
function Props ({prop}) {
return (
<>
<h1>{prop.name}</h1>
<Button>Children prop 2</Button>
</>
);
}
In the example, the Button component uses the children prop to render whatever content is passed between its opening and closing tags. The App component passes "Children prop 1" as the button's content, while the Props component passes "Children prop 2". Both buttons share the same styling defined in the className object. This demonstrates how the children prop allows for reusable components with consistent styling and behavior across different parts of the application. The Props component also receives a data object as a prop, displaying its name property, showcasing how props can be used to pass data and elements between components in React.
Conclusion-
Working with different types of props is very important for React developers to create good UIs. Understanding how to use props, like passing data, elements, and using the children prop, helps build reusable and maintainable components. This blog aims to introduce React props to beginners in React development. By learning the basics of props, you can make your application more flexible and consistent. Props are the main way to pass data and settings between components, making them essential in React's component-based system.
Subscribe to my newsletter
Read articles from Satyam Kale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
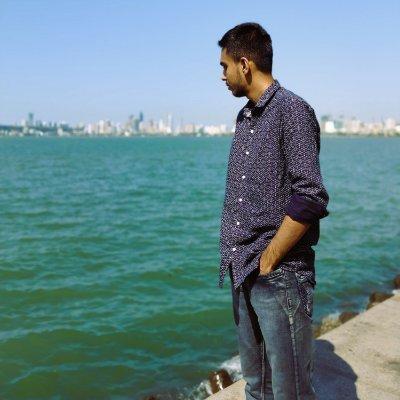
Satyam Kale
Satyam Kale
i am student who is interested in webdev, devops and open source contributions and i love to write about it.