Why You Should Avoid Storing Authentication Tokens in Local Storage π«π
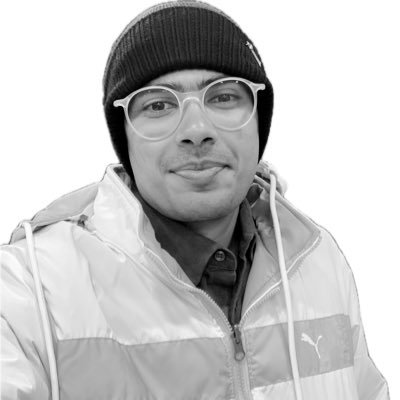
Table of contents
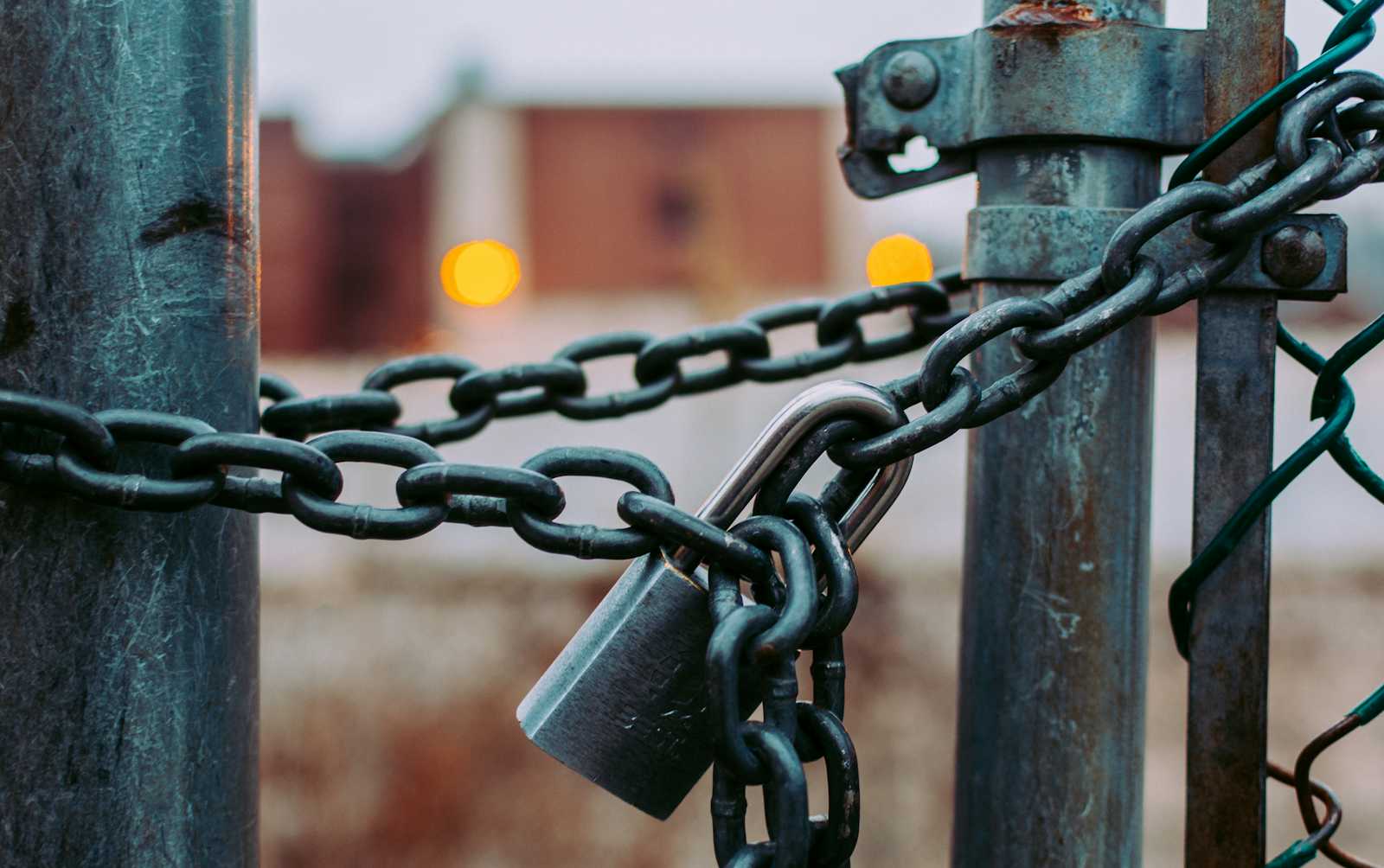
In the realm of web development, securely managing authentication tokens is crucial for protecting user data and maintaining application integrity. A common approach for storing these tokens is using localStorage
due to its simplicity and ease of use. However, this method comes with significant security risks. In this blog post, we'll explore why you should avoid storing authentication tokens in localStorage
and provide safer alternatives with practical examples in a Next.js application using the app router.
Understanding Authentication Tokens
Authentication tokens, such as JWT (JSON Web Tokens), are used to verify the identity of a user and grant access to protected resources. Storing these tokens securely is essential to prevent unauthorized access and protect user data.
Risks of Storing Tokens in localStorage
1. Vulnerability to Cross-Site Scripting (XSS) Attacks β οΈ
Cross-Site Scripting (XSS) is a common security vulnerability where attackers inject malicious scripts into web pages viewed by other users. Since localStorage
is accessible through JavaScript, an XSS attack can easily retrieve stored tokens and compromise user sessions.
// Example of accessing localStorage
localStorage.setItem('token', 'your-auth-token');
// Malicious script can access the token
const token = localStorage.getItem('token');
2. Lack of Secure Transmission π«
Tokens stored in localStorage
are not automatically transmitted with HTTP requests. Developers often need to manually add them to request headers, increasing the risk of exposing tokens if not handled correctly.
// Adding token to request headers
fetch('/api/protected', {
method: 'GET',
headers: {
'Authorization': `Bearer ${localStorage.getItem('token')}`
}
});
3. Persistent Storage π
Tokens in localStorage
persist until explicitly removed. If a user forgets to log out on a shared or public computer, their token remains accessible, posing a security risk.
Safer Alternatives for Storing Tokens
1. HttpOnly Cookies πͺ
HttpOnly cookies are a more secure alternative for storing tokens. These cookies are not accessible via JavaScript, significantly reducing the risk of XSS attacks.
Setting HttpOnly Cookies in Next.js with App Router
- Install
cookie
package
npm install cookie
- Create API Route to Set HttpOnly Cookie
// app/api/login/route.js
import { serialize } from 'cookie';
export async function POST(request) {
const { username, password } = await request.json();
// Authenticate user and get token (simplified)
const token = 'your-auth-token';
const cookie = serialize('token', token, {
httpOnly: true,
secure: process.env.NODE_ENV === 'production',
maxAge: 60 * 60 * 24, // 1 day
path: '/',
});
return new Response(JSON.stringify({ success: true }), {
status: 200,
headers: { 'Set-Cookie': cookie },
});
}
- Create API Route to Access Protected Resource
// app/api/protected/route.js
import { parse } from 'cookie';
export async function GET(request) {
const cookies = parse(request.headers.get('cookie') || '');
const token = cookies.token;
if (token === 'your-auth-token') {
return new Response(JSON.stringify({ message: 'Access granted' }), { status: 200 });
}
return new Response(JSON.stringify({ message: 'Access denied' }), { status: 401 });
}
2. Secure Session Management π‘οΈ
Using session management on the server side is another secure method. This approach stores session data on the server, ensuring that tokens are never exposed to the client.
Setting Up Secure Sessions in Next.js with Next-Auth
- Install
next-auth
package
npm install next-auth
- Configure Next-Auth
// app/api/auth/[...nextauth]/route.js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Credentials({
name: 'Credentials',
authorize: async (credentials) => {
// Authenticate user and return token (simplified)
if (credentials.username === 'user' && credentials.password === 'password') {
return { id: 1, name: 'User', email: 'user@example.com' };
}
return null;
},
}),
],
session: {
jwt: true,
},
callbacks: {
jwt: async (token, user) => {
if (user) {
token.id = user.id;
}
return token;
},
session: async (session, token) => {
session.user.id = token.id;
return session;
},
},
});
- Protecting a Page
// app/page.js
'use client';
import { useSession, signIn, signOut } from 'next-auth/react';
export default function HomePage() {
const { data: session } = useSession();
if (session) {
return (
<div>
<h1>Welcome, {session.user.name}</h1>
<button onClick={() => signOut()}>Sign out</button>
</div>
);
}
return (
<div>
<h1>Please sign in</h1>
<button onClick={() => signIn()}>Sign in</button>
</div>
);
}
Storing authentication tokens in localStorage
poses significant security risks. By opting for HttpOnly cookies or secure session management, you can enhance the security of your application and protect user data. As developers, itβs our responsibility to implement best practices and ensure that our applications are not only functional but also secure.
Happy coding! πππ
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
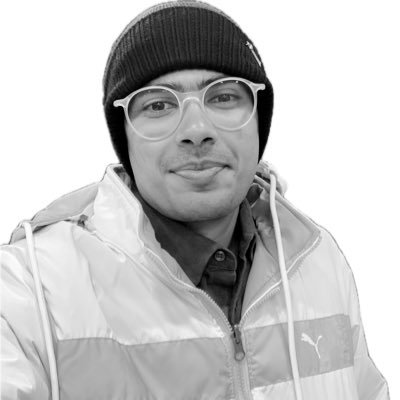
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on β¨οΈ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix π¨π»βπ»