How to Design a Sign-Up Form with HTML and CSS
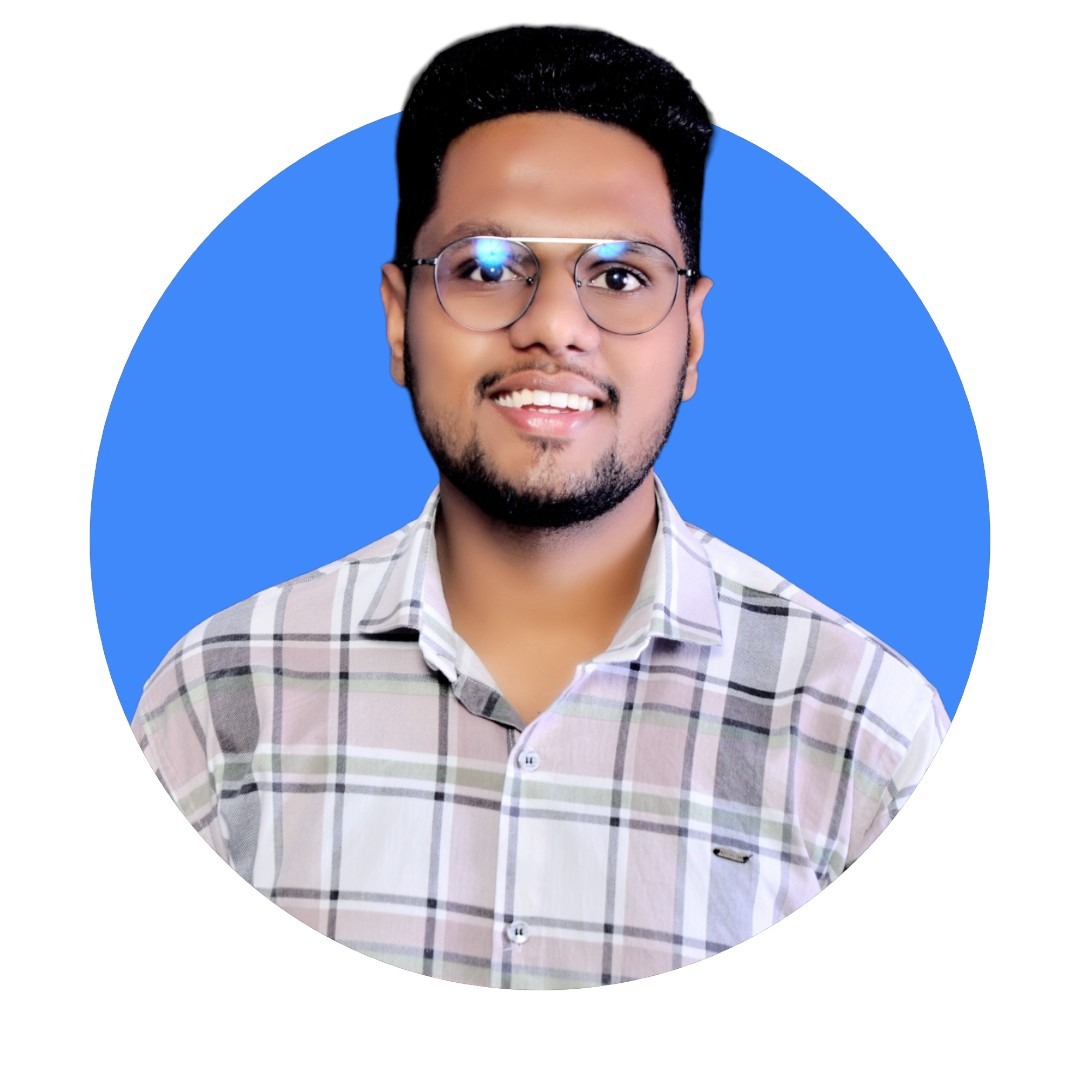
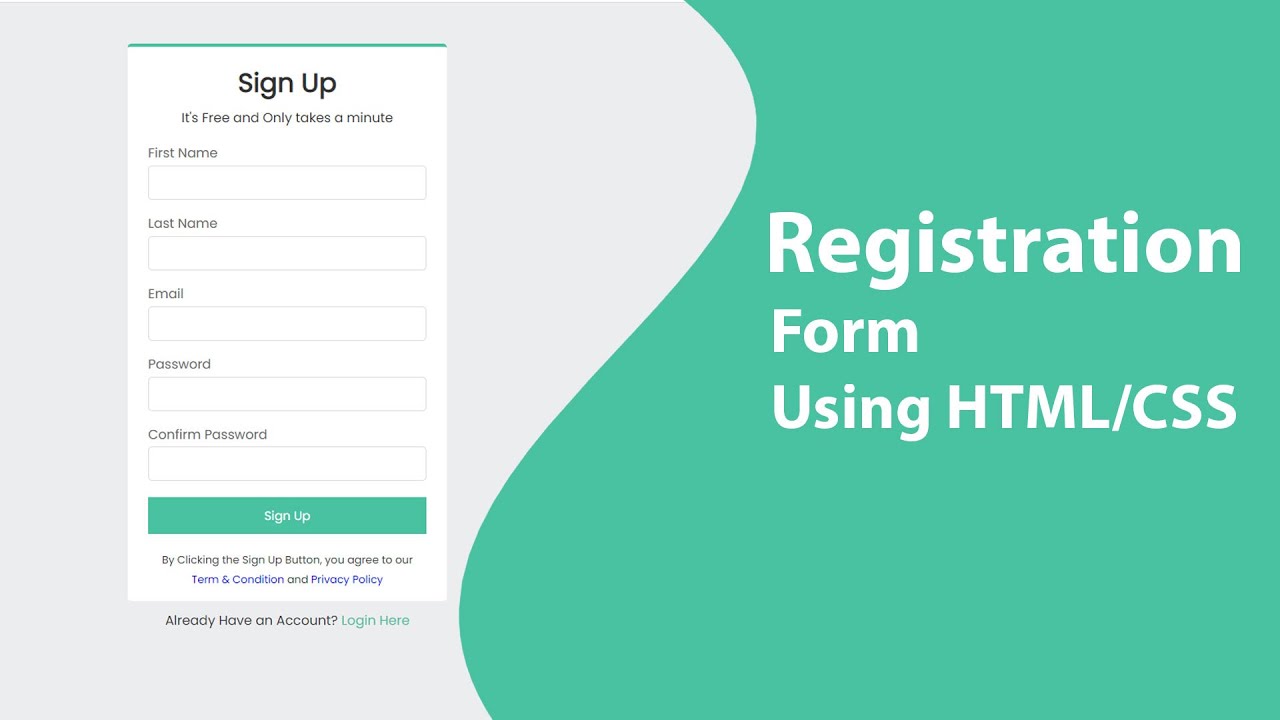
HTML Registration /Sign Up Form :
The HTML registration form is a user interface component comprising input fields for capturing personal information such as name, email, password typically styled with CSS for presentation and validation.
Approach
HTML document with a registration form containing input fields for first name, last name, email, password, etc.
CSS styles are applied to elements for layout, spacing, colors, borders, and alignment to enhance visual appeal.
Utilizes flexbox for centering the form vertically and horizontally within the viewport for better presentation.
Required attribute added to input fields for basic form validation to ensure mandatory fields are filled before submission.
Clickable button styled with CSS for submitting the form data to the server upon completion.
Responsive Signup Form in HTML and CSS :
When you will create a website Registration Form is very important to register the user inside your site. So, I’ve made the tutorial on How to create Registration From Design in HTML and CSS with Code. You can check it Now on below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="css/style.css" />
<title>Signup Form in HTML and CSS3</title>
</head>
<body>
<!-- Main Container -->
<div class="container">
<!-- Form Container -->
<div class="form-wrap">
<h1>Signup Form</h1>
<div class="signup-box">
<div class="left-box">
<form action="#">
<input type="text" placeholder=" Full Name " class="form-control" />
<input type="text" placeholder=" User Name " class="form-control" />
<input type="email" placeholder=" Email " class="form-control" />
<input type="password" placeholder=" Password " class="form-control" />
<input type="password" placeholder=" Confirm Password " class="form-control" />
<a href="#">Signup Now</a>
</form>
</div>
<div class="right-box">
<img src="img/img.svg" alt="" />
</div>
</div>
</div>
<p>
By Clicking the SIgnup Button You Agree to Our <a href="#">Terms and Condition </a> and
<a href="#">Privacy Policy</a>
</p>
</div>
</body>
</html>
Once you did that, then you need to use CSS to design it, I’ve mentioned the CSS code below you can check it now.
@import url("https://fonts.googleapis.com/css2?family=Poppins&family=Roboto&display=swap");
* {
box-sizing: 0;
padding: 0;
margin: 0;
font-family: "poppins", sans-serif;
}
.container {
width: 100%;
min-height: 100vh;
background: #273746;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.form-wrap {
background: #fff;
max-width: 800px;
padding: 2rem 0px;
border-right: 0.5rem solid #6c63ff;
border-radius: 1rem;
}
.form-wrap h1 {
font-size: 2rem;
color: #333;
margin-bottom: 30px;
text-align: center;
}
.signup-box {
display: flex;
justify-content: center;
align-items: center;
flex-wrap: wrap;
}
.left-box {
flex-basis: 50%;
flex: 1;
}
.right-box {
flex-basis: 50%;
flex: 1;
}
.right-box img {
display: block;
width: 80%;
margin: auto;
}
.form-control {
width: 90%;
padding: 0.5rem;
margin: 0.2rem 1rem;
outline: none;
border: 1px solid #273746;
font-size: 1rem;
border-radius: 04px;
}
.left-box a {
text-decoration: none;
background: #273746;
color: #fff;
margin: 1rem 1rem;
padding: 0.6rem 0.5rem;
display: block;
text-align: center;
width: 91%;
transition: all 0.5s;
border-radius: 03px;
}
.left-box a:hover {
background: #6c63ff;
color: #fff;
transition: 0.5s;
}
.container {
color: #fff;
text-align: center;
}
.container p {
padding: 1rem 0px;
}
.container a {
color: #ddd;
}
@media (max-width: 768px) {
.signup-box {
flex-direction: column;
}
}
Output:
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
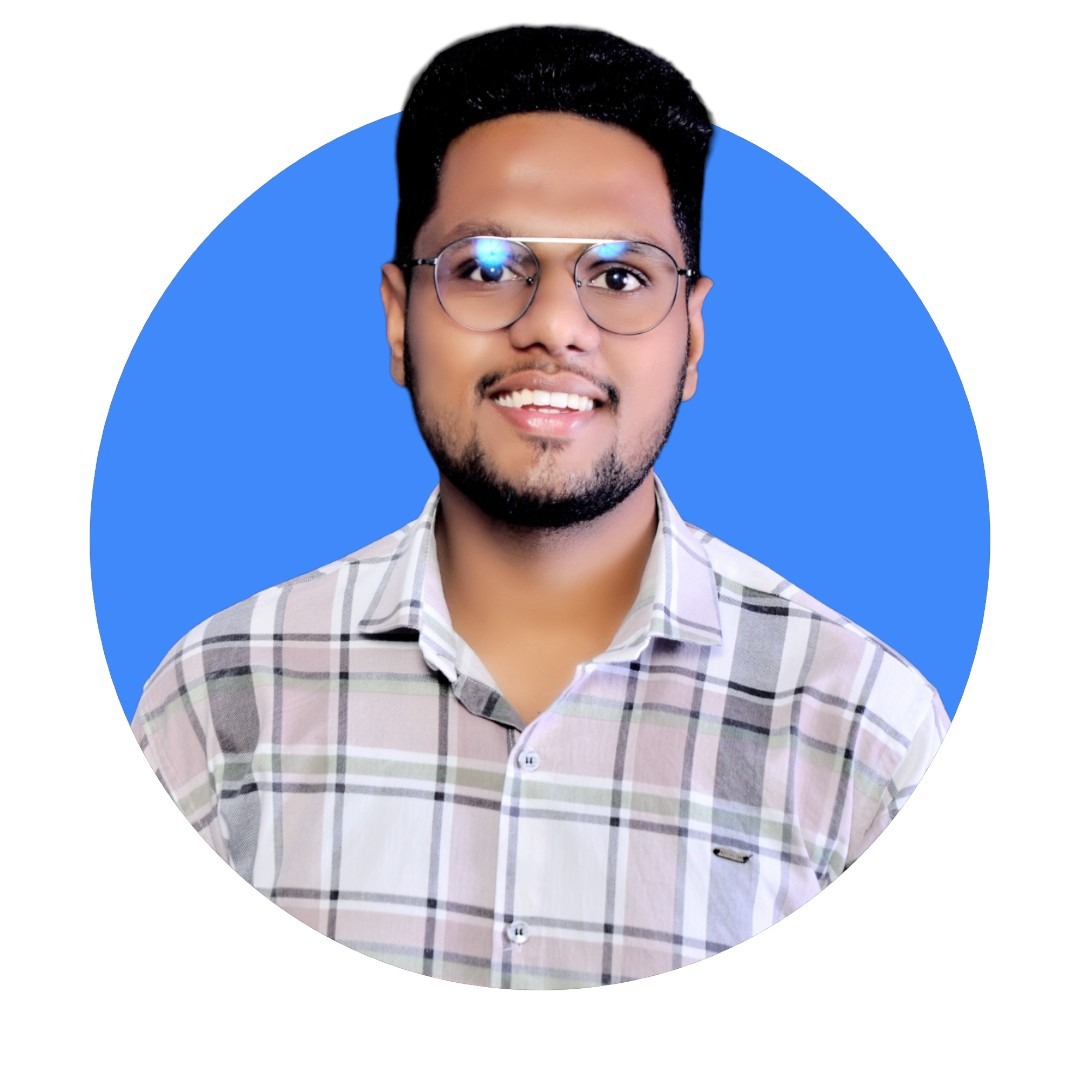
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.