Mastering Java Streams for Efficient Data Processing
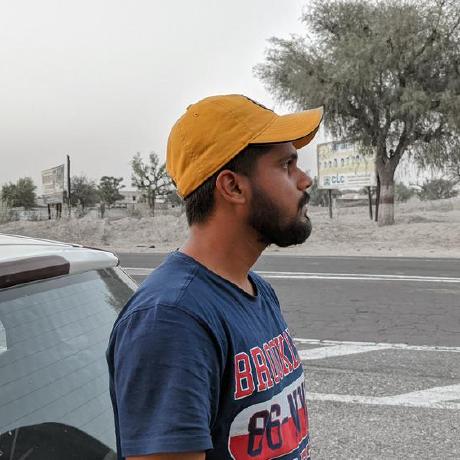
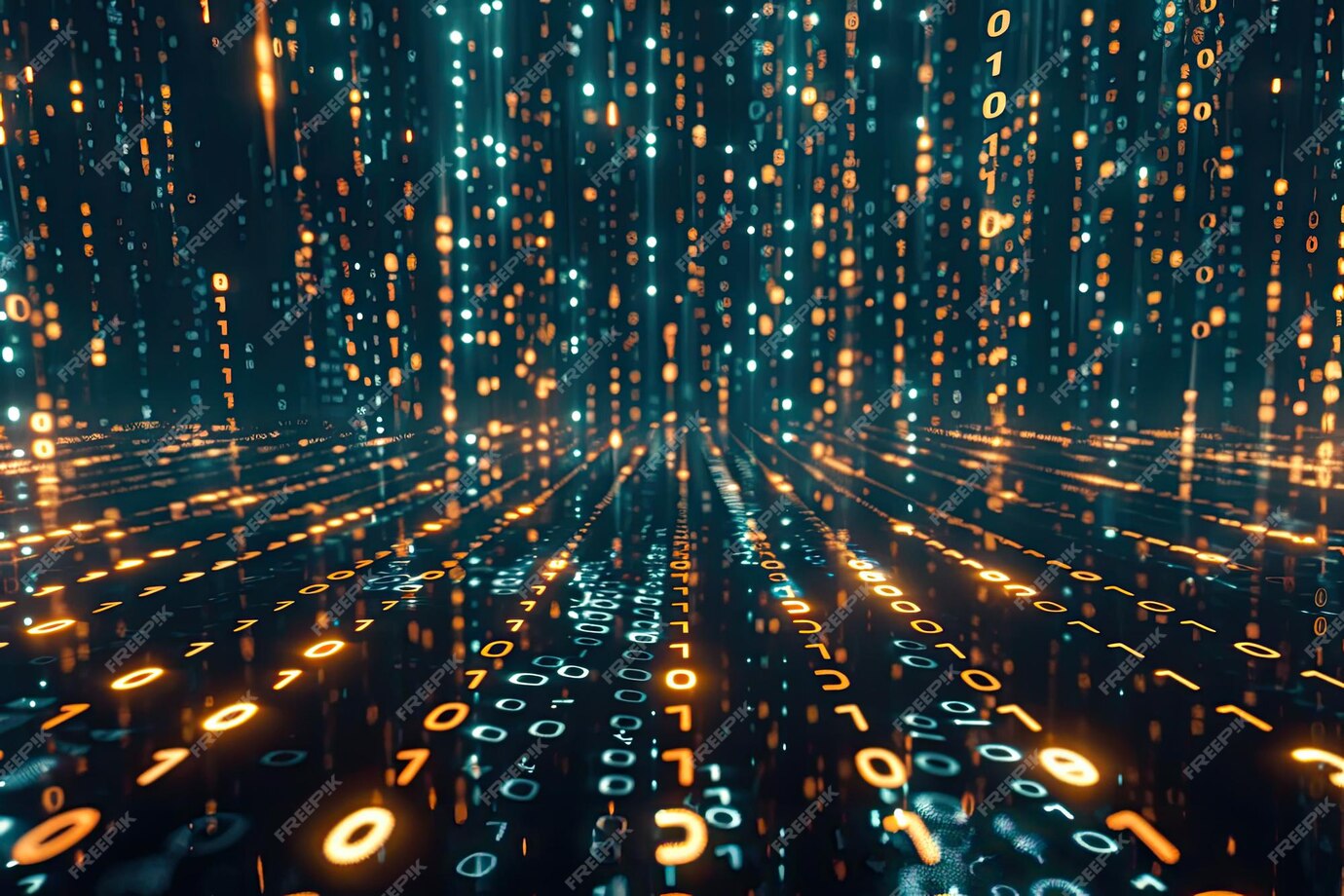
Hey there, Java enthusiast! Ready to dive into the magical world of Java Streams? If you’ve ever felt overwhelmed by looping through collections or manipulating data, you’re in for a treat. Java Streams will change how you think about data processing – and dare I say, make it fun!
Introduction
Imagine this: You’re at a huge buffet with countless dishes. Instead of walking around and picking up food yourself (which can be tedious and repetitive), you have a conveyor belt that brings you exactly what you want, prepared just the way you like it. That’s what Java Streams do for data processing – they bring the data to you in a smooth, efficient manner.
Streams, introduced in Java 8, offer a sleek way to work with collections of data. They let you filter, transform, and reduce data with ease, all while writing code that’s more readable and less error-prone. So, let’s get started on this delightful journey!
Getting Started with Streams
What is a Stream?
Think of a stream as a pipeline through which data flows. Unlike collections, streams don’t store data. Instead, they process data as it passes through. It’s like a river flowing through various checkpoints, each performing a different task – filtering, mapping, and so on.
Creating Streams
Streams can be created from collections, arrays, or even directly from specified values. Here’s how you do it:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Stream;
public class StreamExample {
public static void main(String[] args) {
// Stream from a collection
List<String> list = Arrays.asList("apple", "banana", "cherry");
Stream<String> streamFromList = list.stream();
// Stream from an array
String[] array = {"dog", "cat", "mouse"};
Stream<String> streamFromArray = Arrays.stream(array);
// Stream from specified values
Stream<String> streamFromValues = Stream.of("red", "green", "blue");
// Stream using generate method
Stream<Double> randomNumbers = Stream.generate(Math::random).limit(5);
// Stream using iterate method
Stream<Integer> evenNumbers = Stream.iterate(0, n -> n + 2).limit(5);
}
}
Cool, right? Now you have streams flowing from lists, arrays, and even out of thin air (well, almost)!
Intermediate and Terminal Operations in Depth
Intermediate Operations:
These operations transform a stream into another stream, allowing for a sequence of processing steps.
filter()
The filter()
method retains only those elements that match a given condition (predicate).
List<String> names = Arrays.asList("John", "Jane", "Jack", "Doe");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [John, Jane, Jack]
Insight: Use filter()
to zero in on the data you care about. It’s like having a sieve that catches the nuggets and lets the rest flow away.
map()
The map()
method transforms each element of the stream into another object via the provided function.
List<String> names = Arrays.asList("John", "Jane", "Jack");
List<Integer> nameLengths = names.stream()
.map(String::length)
.collect(Collectors.toList());
System.out.println(nameLengths); // Output: [4, 4, 4]
Insight: Think of map()
as a magic wand that turns apples into oranges. It’s perfect for transforming data from one type to another.
sorted()
The sorted()
method sorts the elements of the stream based on natural order or a provided comparator.
List<Integer> numbers = Arrays.asList(5, 3, 8, 1, 6);
List<Integer> sortedNumbers = numbers.stream()
.sorted()
.collect(Collectors.toList());
System.out.println(sortedNumbers); // Output: [1, 3, 5, 6, 8]
Insight: Use sorted()
to put things in order – be it ascending, descending, or any custom order you like. It’s like tidying up a messy room.
Terminal Operations:
These operations produce a result or a side-effect and mark the end of the stream pipeline.
forEach()
The forEach()
method performs an action for each element of the stream.
List<String> names = Arrays.asList("John", "Jane", "Jack");
names.stream()
.forEach(System.out::println);
Insight: forEach()
is your go-to for performing actions like printing or logging. It’s like a loop but more elegant.
collect()
The collect()
method transforms the stream elements into a different form, typically a collection.
List<String> names = Arrays.asList("John", "Jane", "Jack");
Set<String> namesSet = names.stream()
.collect(Collectors.toSet());
System.out.println(namesSet); // Output: [John, Jane, Jack]
Insight: collect()
is the ultimate gatherer. It’s like collecting flowers into a bouquet – transforming a stream into a list, set, map, or even a custom collection.
reduce()
The reduce()
method combines the elements of the stream into a single result.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.reduce(0, Integer::sum);
System.out.println("Sum: " + sum); // Output: Sum: 15
Insight: Think of reduce()
as the ultimate aggregator. Whether it’s summing numbers, concatenating strings, or finding the max value, reduce()
brings everything together.
Advanced Stream Operations:
Parallel Streams
Parallel streams split the workload across multiple threads, potentially speeding up processing.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.parallelStream()
.reduce(0, Integer::sum);
System.out.println("Sum: " + sum); // Output: Sum: 15
Insight: Parallel streams can give your processing a turbo boost, but be cautious – they’re best for CPU-intensive tasks and large datasets.
Custom Collectors
Custom collectors allow for more control over the collection process. Here’s how to join strings with a delimiter:
List<String> names = Arrays.asList("John", "Jane", "Jack");
String result = names.stream()
.collect(Collectors.joining(", "));
System.out.println(result); // Output: John, Jane, Jack
Insight: Custom collectors are like having a custom-built machine for assembling your data exactly the way you want it.
Handling Exceptions in Streams
Handling exceptions in streams can be streamlined using helper methods.
List<String> numbers = Arrays.asList("1", "2", "three", "4");
List<Integer> result = numbers.stream()
.map(StreamExample::safeParseInt)
.filter(Optional::isPresent)
.map(Optional::get)
.collect(Collectors.toList());
System.out.println(result); // Output: [1, 2, 4]
private static Optional<Integer> safeParseInt(String str) {
try {
return Optional.of(Integer.parseInt(str));
} catch (NumberFormatException e) {
return Optional.empty();
}
}
Insight: Exception handling in streams can be elegant and functional. Wrapping operations in Optional
helps manage errors gracefully.
Best Practices and Performance Considerations
Avoid Stateful Operations: Be cautious with operations like
limit()
,skip()
, andsorted()
as they can introduce performance bottlenecks, especially with parallel streams.Minimize Stream Pipelines: Combine operations into a single pipeline when possible to reduce overhead.
Use the Right Data Structures: Choose the most efficient data structures for your use case. For example,
ArrayList
for frequent access andLinkedList
for frequent inserts and deletes.Be Mindful of Side Effects: Ensure that stream operations are free of side effects to maintain predictability and reliability.
Mastering Java Streams is like unlocking a treasure chest of tools for efficient and elegant data processing. With streams, your code becomes more readable, less error-prone, and fun to write. So go forth, experiment, and let the power of streams transform your Java programming experience. Happy coding!
Subscribe to my newsletter
Read articles from Vivek Tak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
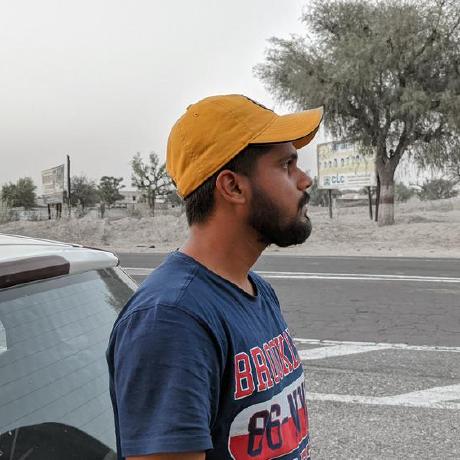
Vivek Tak
Vivek Tak
I am a developer from India. I like to learn everyday about new technologies. Interest Areas: AI, FinTech I am actively looking for learning Golang and DevOps