Exploring React 19: Comprehensive Overview of Major Updates and Features

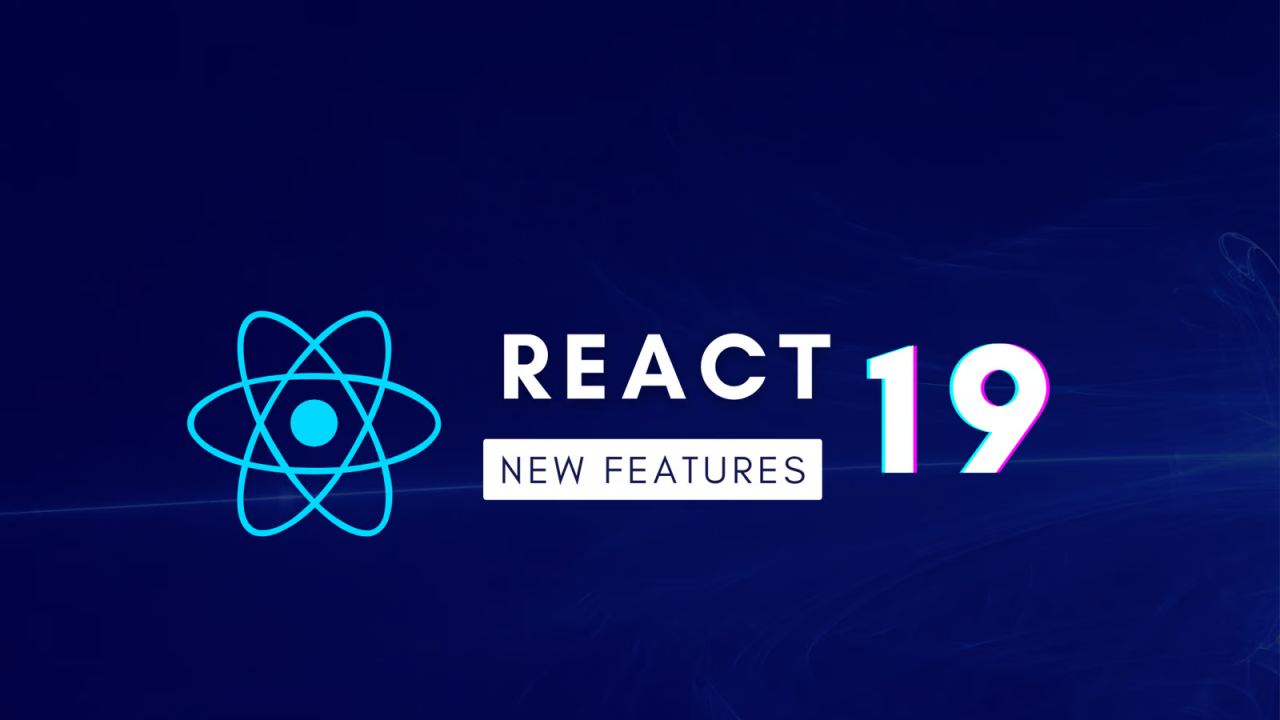
React 19 brings a host of new features and enhancements designed to streamline development, improve performance, and enhance the user experience. Here's a detailed breakdown of what's new in React 19:
1. React Server Components
Description
React Server Components allow components to be rendered on the server, providing several benefits such as faster page load times, better SEO optimization, and overall improved performance.
Key Points
Server components are defined using
“use server”
at the beginning of the component.They are similar to the server components used in Next.js.
This feature improves application performance by handling certain tasks server-side.
"use server";
export async function getData() {
const res = await fetch('https://jsonplaceholder.typicode.com/posts');
return res.json();
}
Benefits
Faster initial page load times.
Improved SEO since content is pre-rendered on the server.
Enhanced performance for complex applications.
2. Actions API
Description
The Actions API introduces a more efficient way to handle state and data mutations, especially useful in scenarios involving forms.
Key Points
Utilizes the
useActionState
hook to manage state changes, errors, and pending states during data fetching.Simplifies handling of form submissions and state updates.
Example
function ChangeEmail({ email, setEmail }) {
const [state, submitAction, isPending, error] = useActionState(
async (previousState, formData) => {
const error = await updateEmail(formData.get("email"));
if (error) {
return error;
}
redirect("/path");
return null;
},
email,
null
);
return (
<form onSubmit={submitAction}>
<input type="email" name="email" defaultValue={state} />
<button type="submit" disabled={isPending}>
{isPending ? "Updating..." : "Update"}
</button>
{error && <p>{error}</p>}
</form>
);
}
Benefits
Streamlined state management.
Automatic handling of form states (e.g., pending, error).
Improved code readability and maintainability.
3. Document Metadata Management
Description
React 19 simplifies managing document metadata, such as titles and meta tags, directly within components.
Key Points
Eliminates the need for external packages like
react-helmet
.Enhances SEO and accessibility with less boilerplate code.
Example
const HomePage = () => {
return (
<>
<title>Pieces for Developers</title>
<meta name="description" content="Your Workflow Assistant" />
// Page content
</>
);
};
4. Background Asset Loading
Description
This feature allows React to load images and other assets in the background while users interact with the current page, reducing load times for subsequent pages.
Key Points
Improves performance by preloading assets.
Enhances user experience with faster navigation.
Benefits
Reduced wait times when navigating between pages.
Smoother user experience.
5. Enhanced Hooks
Description
React 19 introduces new hooks and improves existing ones, giving developers more control and flexibility.
Key Hooks
use()
hook: Simplifies handling asynchronous functions and state management.useFormStatus
hook: Allows child components to access form status from parent components.useOptimistic
hook: Facilitates optimistic UI updates during data fetching.useSignal
hook: Enhances reactivity and state management by allowing components to react to signals or events.
Example (useOptimistic)
const [optimisticState, setOptimisticState] = useOptimistic(initialState, newState);
Benefits
More concise and readable code.
Improved handling of asynchronous tasks and form statuses.
Enhanced user experience with optimistic updates.
6. Web Components Integration
Description
React 19 offers better support for Web Components, making it easier to integrate React with other web technologies.
Key Points
Improved compatibility with Web Components.
Facilitates the use of React in diverse web projects.
Benefits
Greater flexibility in combining different web technologies.
Enhanced ability to integrate React into existing projects.
7. Concurrent Rendering
Description
Concurrent rendering improves the responsiveness of applications by preparing multiple versions of the UI and presenting the most optimal one.
Benefits
Faster time-to-interactive for users.
Smoother transitions and better handling of heavy computations.
8. Automatic Server-Side Rendering (SSR)
Description
React 19 enhances server-side rendering capabilities, making it easier to render pages on the server for better SEO and performance.
Benefits
Improved search engine rankings.
Faster initial page loads for users.
9. Global State Management
Description
React 19 includes improved tools for managing global state, making it easier to handle data across the entire application.
Benefits
Reduced boilerplate code for state management.
Faster development and deployment of new features.
Conclusion
React 19 is a significant update that brings numerous improvements aimed at enhancing both developer and user experiences. From better server-side rendering and enhanced hooks to improved asset loading and metadata management, React 19 makes building modern web applications more efficient and enjoyable. By upgrading to React 19, developers can leverage these new features to create faster, more responsive, and SEO-friendly applications.
Let's Talk about all this in detail in upcoming blogs.
Subscribe to my newsletter
Read articles from NITYOM TIKHE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NITYOM TIKHE
NITYOM TIKHE
I am a dedicated Frontend Engineer with a strong focus on building responsive and innovative web applications. Proficient in React, Vite, and Framer Motion, I specialize in creating dynamic user experiences. With a background in both web development and algorithmic problem-solving, I bring technical expertise and creativity to every project. Let's connect and collaborate on exciting tech ventures!