Step-by-Step Tutorial: DynamoDB CRUD Operations with Python AWS SDKs ๐ ๏ธ๐
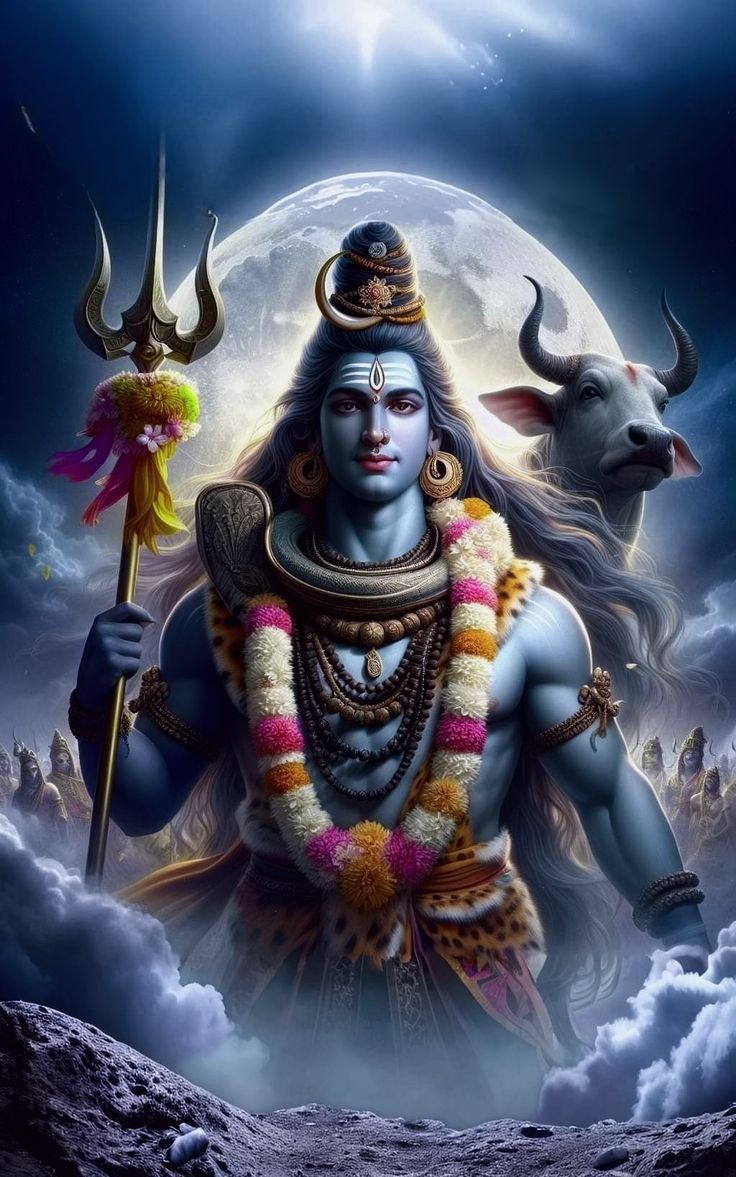
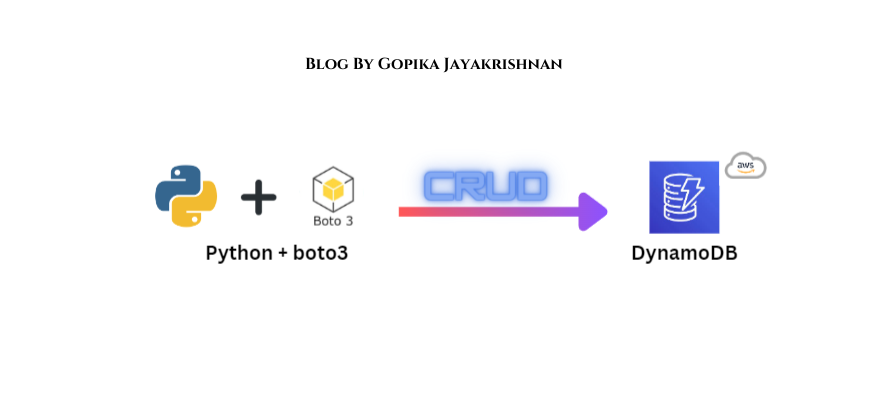
Introduction
In the fast-paced world of cloud computing, managing databases efficiently is crucial. That's where DynamoDB, Amazon's fully managed NoSQL database service, comes in, offering seamless and scalable data storage. To interact with DynamoDB, you'll need a strong programming interface, and Boto3, the official AWS SDK for Python, fits the bill perfectly. In this blog, weโll explore how to perform DynamoDB CRUD operations using Boto3, unlocking the potential of these tools for efficient data management in the AWS cloud.
Unlocking the Power of Boto3: Your Gateway to AWS Services
Boto3 makes it easy to integrate your Python application, library, or script with AWS services like Amazon S3, Amazon EC2, Amazon DynamoDB, and more. Think of Boto3 as your reliable bridge between your Python code and the expansive AWS ecosystem. It offers pre-built functions and classes to interact with various AWS services, including DynamoDB. With Boto3 handling the low-level communication, you can focus on your application logic without having to reinvent the wheel.
Mastering CRUD Operations in DynamoDB with Boto3
First, create a Lambda function in the AWS Lambda Console with the necessary permissions to handle CRUD operations on a DynamoDB table. For this demo, we'll use DynamoDB Full Access permission, but in practice, you should follow the principle of least privilege. Replace the boilerplate code with the provided code, which performs CRUD actions based on events in the DynamoDB table, and then attach the necessary IAM permissions to the function.
import json
import boto3
dynamodb = boto3.resource('dynamodb')
table_name = 'ContactBookApp' # update the table name here.
table = dynamodb.Table(table_name)
def lambda_handler(event, context):
operation = event['operation']
if operation == 'create':
return create_item(event)
elif operation == 'read':
return read_item(event)
elif operation == 'list':
return list_items(event)
elif operation == 'update':
return update_item(event)
elif operation == 'delete':
return delete_item(event)
else:
return {
'statusCode': 400,
'body': json.dumps('Invalid operation')
}
def create_item(event):
item_data = event['item']
# everything is a string until you parse it.
# Ensure the 'id' field is cast to an integer
item_data['id'] = int(item_data['id'])
# we need to use put_item() boto3 api to create item in DDB Table
response = table.put_item(Item=item_data)
return {
'statusCode': 200,
'body': json.dumps(response)
}
def read_item(event):
item_id = int(event['item_id']) # Convert 'item_id' to an integer
# we need to use get_item() boto3 api to read item from DDB Table
response = table.get_item(Key={'id': item_id})
item = response.get('Item')
return {
'statusCode': 200,
'body': json.dumps(str(item)) # Convert 'item_id' to string
}
def list_items(event):
# we need to use scan() boto3 api to list all items in DDB Table
response = table.scan()
items = response.get('Items', [])
return {
'statusCode': 200,
'body': json.dumps(str(items)) # Convert 'item_id' to string
}
def update_item(event):
item_id = int(event['item_id']) # Convert 'item_id' to an integer
updated_data = event['updated_data']
# Prepare the update expression and attribute values dynamically
update_expression = 'SET '
expression_attribute_values = {}
for key, value in updated_data.items():
update_expression += f'#{key} = :{key}, '
expression_attribute_values[f':{key}'] = value
# Remove the trailing comma and space
update_expression = update_expression[:-2]
# we need to use update_item() boto3 api to update item in DDB Table
response = table.update_item(
Key={'id': item_id},
UpdateExpression=update_expression,
ExpressionAttributeNames={f'#{key}': key for key in updated_data.keys()},
ExpressionAttributeValues=expression_attribute_values
)
return {
'statusCode': 200,
'body': json.dumps(response)
}
def delete_item(event):
item_id = int(event['item_id']) # Convert 'item_id' to an integer
response = table.delete_item(Key={'id': item_id})
return {
'statusCode': 200,
'body': json.dumps(response)
}
Below is the DynamoDB table which does not have any items for now and as soon as we invoke the lambda function based on the particular events as per CRUD, the item will get created, read, listed, updated, and deleted to/from the DynamoDB table.
Events created as per DDB CRUD Operations
DynamoDB (DDB) which is a NoSQL database, aligns with standard CRUD operations - Create, Read, Update, and Delete and each operation is tailored to address distinct data manipulation requirements. Let's delve into each of the following events one by one.
Create Event (Adding data to DynamoDB)
The Create operation involves adding new items or records to a DynamoDB table. Using Boto3, this is achieved by providing the necessary attributes for the new item as a dictionary and calling the put_item
method.
{
"operation": "create",
"item": {
"id": 7777,
"name": "Ronnie",
"email": "ronnie@gmail.com",
"contactNo": "87090993",
"designation": "Manager",
"project": "CVB",
"newHire": "Yes"
}
}
We will get the following response after invoking the lambda function with the create event.
Now we can see the item created in the DynamoDB table.
Read Event (Retrieving data from DynamoDB)
The Read operation retrieves data from DynamoDB based on specified criteria. Boto3 offers the get_item
method for this purpose.
{
"operation": "read",
"item_id": 7777
}
We will get the following response after invoking the lambda function with the read event.
List Event (Retrieving all data from DynamoDB)
The List operation lists/retrieves all the data from DynamoDB. Boto3 offers the scan
method for this purpose, and we don't need to provide extra parameters in this case.
{
"operation": "list"
}
We will get the following response after invoking the lambda function with the list event.
Update Event (Modifying existing data or add new data into DynamoDB table)
The Update operation is used to modify existing data in DDB table items or add new data. Boto3 offers the update_item
method for this purpose.
{
"operation": "update",
"item_id": 7777,
"updated_data": {
"designation": "Senior Engg Manager",
"project": "QWE",
"awards": "yes"
}
}
This is the existing table item created as per the create event and now we are going to modify this item as per the update event as seen above.
We will get the following response after invoking the lambda function with the update event.
Now we can see that the item has been updated in the DynamoDB table as well.
Delete Event (Removing data from DynamoDB table)
The Delete operation involves removing items from the DynamoDB table. Boto3 provides the delete_item
method for this purpose.
{
"operation": "delete",
"item_id": 7777
}
We will get the following response after invoking the lambda function with the delete event.
Now we can see that the item has been deleted from the DynamoDB table.
References
Getting Started with Lambda Function
Conclusion
This blog provides detailed instructions on programmatically performing CRUD operations within a DynamoDB table. You can customize these procedures to fit your specific table structures and needs.
Thank you for taking the time to read my blog! ๐ I hope you found it helpful and insightful. If you did, please give it a ๐ and consider ๐ subscribing to my newsletter for more content like this.
I'm always looking for ways to improve, so your comments and suggestions are welcome ๐ฌ.
Thanks again for your continued support! ๐
Subscribe to my newsletter
Read articles from Gopika Jayakrishnan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
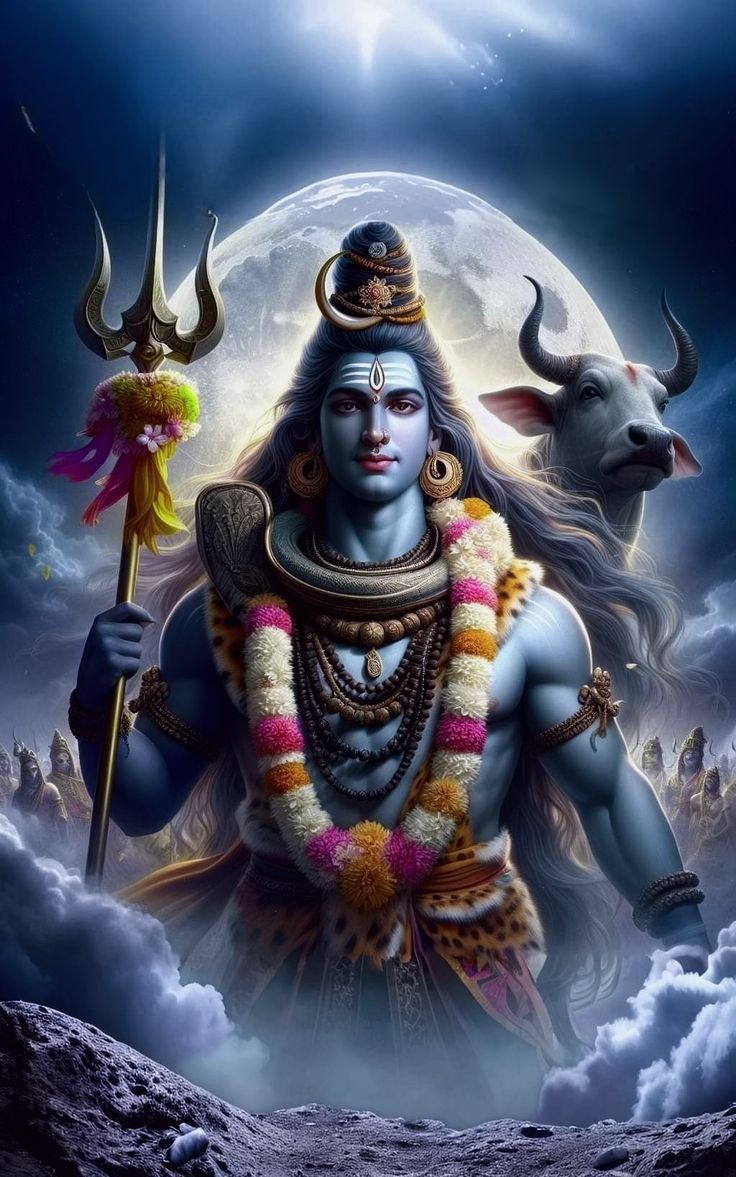
Gopika Jayakrishnan
Gopika Jayakrishnan
๐ I'm a Cloud DevOps engineer โ๏ธ. I develop, deploy, and maintain software applications in cloud environments, ensuring they are scalable, reliable, and secure ๐. My expertise lies in programming languages and cloud platforms, and I work collaboratively to deliver innovative solutions that meet business needs ๐.