Adding Debounce to Search Bar in Ionic: A Step-by-Step Guide

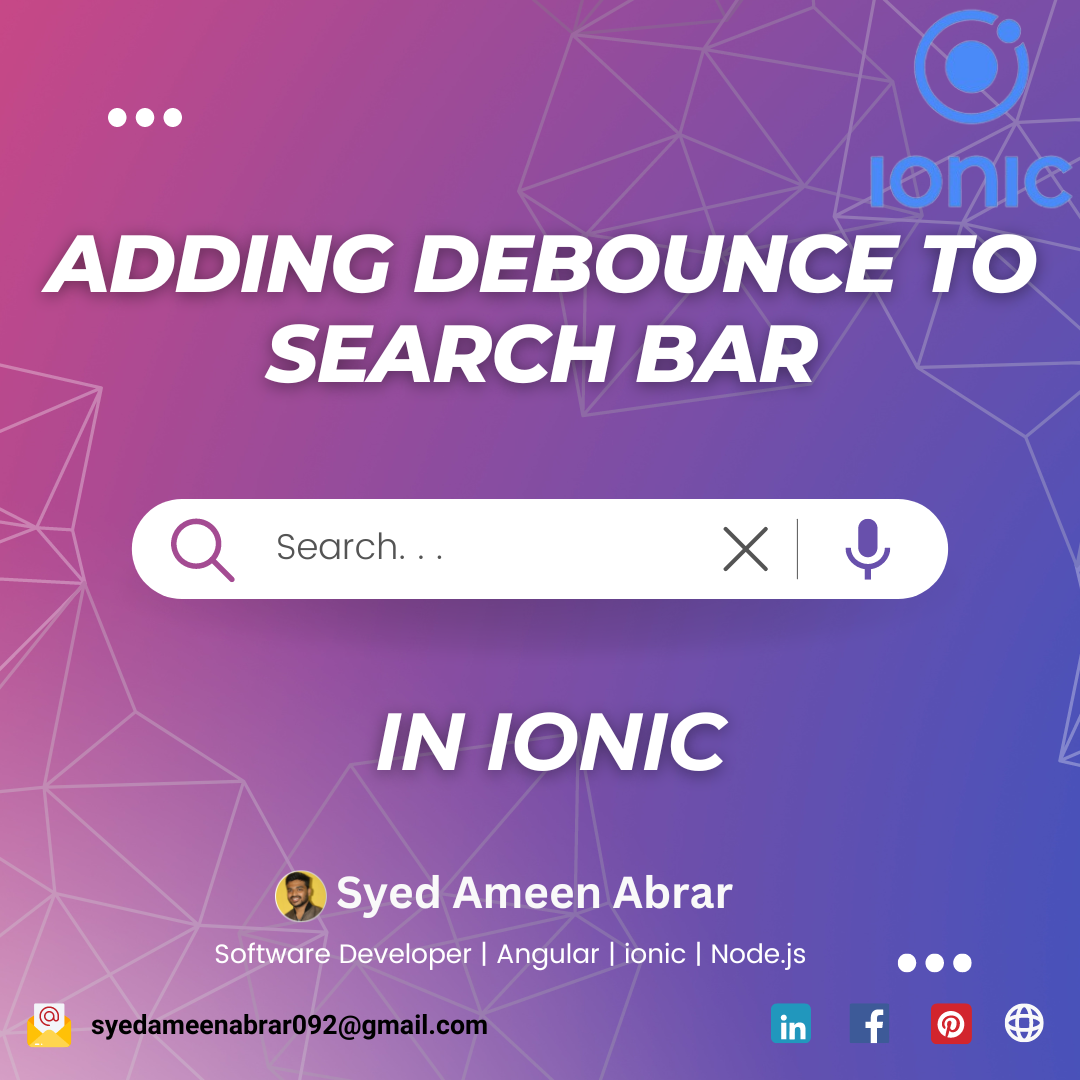
Introduction:
In Ionic applications, implementing a search functionality is a common requirement. However, performing a search operation with every keystroke can be resource-intensive and may lead to performance issues, especially in large datasets. To optimize this, we can introduce a debounce mechanism, which triggers the search function only after a certain period of inactivity. In this step-by-step guide, we'll learn how to add debounce to a search bar in an Ionic application.
Prerequisites:
Basic understanding of Ionic framework.
Ionic CLI installed.
A basic Ionic project set up.
Step 1: Create an Ionic Project If you haven't already set up an Ionic project, create one using the Ionic CLI with the following command:
ionic start myApp blank
Step 2: Add a Search Bar Component In your Ionic project, navigate to the page or component where you want to add the search functionality. Replace the existing code with the following snippet:
<ion-col size="12"> <ion-searchbar class="srchBar" placeholder="Search your {{listType}} here" [debounce]="1000" (ionInput)="handleInput($event)"></ion-searchbar> </ion-col>
Step 3: Implement the HandleInput Function
In the corresponding TypeScript file (e.g., home.page
.ts
), implement the handleInput
function as follows:
import { Component } from '@angular/core'; @Component({ selector: 'app-home',
templateUrl: '
home.page
.html',
styleUrls: ['
home.page
.scss'],})
export class HomePage { searchTerm: string = ''; constructor() {} handleInput(event: any) {
this.searchTerm =
event.target
.value; // Call your search function here this.getSearchData();
} getSearchData() {
// Implement your search logic here
console.log('Searching for:', this.searchTerm);
}
}
Step 4: Explanation
In the HTML template, we've added an
ion-searchbar
component with thedebounce
attribute set to1000
milliseconds. This means that thehandleInput
function will be triggered only if the user stops typing for 1 second.The
(ionInput)
event is bound to thehandleInput
function, which captures the input event and updates thesearchTerm
property accordingly.In the TypeScript file, the
handleInput
function updates thesearchTerm
property and calls thegetSearchData
function, which represents the actual search operation.
Step 5: Test Your Implementation
Run your Ionic application using the following command:
ionic serve
Open your application in a browser and test the search functionality. You should observe that the search function is triggered only after a brief pause in typing, thanks to the debounce mechanism.
Conclusion:
In this tutorial, we've learned how to add debounce to a search bar in an Ionic application. By implementing debounce, we ensure that the search function is invoked efficiently, optimizing performance and user experience. This technique is particularly useful when dealing with large datasets or resource-intensive operations.
Fallow me for more such content,
Email me at : syedameenabrar092@gmail.com,
Website : https://syedameenabrar.blogspot.com/p/home.html
Blogs : https://syedameenabrar.hashnode.dev/
Instagram : https://www.instagram.com/syed_ameen_abrar/
Linkedin: https://www.linkedin.com/in/syed-ameen-abrar-61a67217a/
Facebook : https://www.facebook.com/syedameenabrar92/
Subscribe to my newsletter
Read articles from Syed Ameen Abrar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
