How to Deploy and Host a React App using Netlify
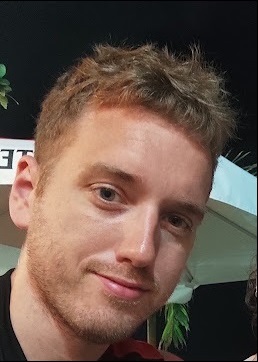

In this article, we will deploy the final part of our full-stack application to the cloud: the front-end website. This will communicate with the REST API we deployed in the previous blog. We will discuss why we are using Netlify to host our React app instead of AWS Amplify (although I will cover deploying to AWS Amplify in a future blog outside of this series) or another provider. Once this is done, our full-stack application will be live on the web for public use. However, there are still more articles ahead in the series to improve security and make optimizations.
As always, the video below offers a visual demo of everything covered in this blog for your reference. Continue reading for the step-by-step guide.
Why use Netlify
A problem we will face when deploying our frontend app is making requests to an HTTP address (our AWS Elastic Beanstalk REST API) from an HTTPS source (our React website), which will cause exceptions. The proper solution is to secure our REST API so that requests are HTTPS to HTTPS. However, this requires purchasing a domain name and SSL from a DNS provider like Namecheap or GoDaddy. I will cover this in a future blog post. Since this is our first app and we are learning, we will use a free option that is still straightforward. Netlify offers a solution to this problem, which AWS Amplify does not, as we will see now.
React Front-end proxy configurations for REST API communication
In this section, we will explain how to connect your React website to the REST API, both locally and in the cloud. If you are using the sample web app from this series you will need to update some values, we will go through the files of interest now.
ApiRequests.js
For reusability, you will likely want to centralize API requests. In the sample web app, this class is called ApiRequests.js. The important thing to note here is the URLs. For example, in the getUser function, the URL is /api/user?id=${userId}
.
export function getUser(userId, onSuccess) {
try {
fetch(`/api/user?id=${userId}`)
.then(function (response) {
return response.json();
})
.then(function (data) {
onSuccess(data);
});
} catch (error) {
console.error("Failed to get user:", error);
}
}
All my URLs in this class begin with /api
This prefix will be set up to be replaced (or redirected) with our REST API's base URL. This approach allows us to specify the base URL only once in our codebase, rather than for every request. If we need to change it in the future, it will cause minimal disruption. It also helps us solve our HTTPS to HTTP problem, so follow this pattern.
package.json
In our package.json file, we need to specify the proxy here:
"proxy": "http://mynotes-api-env.eba-wmh9t6sz.us-east-1.elasticbeanstalk.com"
Replace the value with your AWS Elastic Beanstalk endpoints value.
setupProxy.js
This file is important for running your React app locally against your AWS Elastic Beanstalk REST API instance. It is not mandatory for deployment, but it is useful for local testing. Add this file (with the exact name "setupProxy.js") under your /src folder, and you can copy these contents:
const { createProxyMiddleware } = require('http-proxy-middleware');
module.exports = function(app) {
app.use(
'/api',
createProxyMiddleware({
target: 'http://mynotes-api-env.eba-wmh9t6sz.us-east-1.elasticbeanstalk.com',
changeOrigin: true,
pathRewrite: {
'^/api': '',
},
})
);
};
The only value you need to update is the "target" value. Replace it with your AWS Elastic Beanstalk endpoint.
If you are using a different prefix for your API requests (i.e., "/api"), update the pathRewrite value to match your prefix. Also note you must have the http-proxy-middleware installed, so if you haven't already added it, run this command:
npm install http-proxy-middleware
netlify.toml
The netlify.toml file is the final configuration needed to get your deployed React app to communicate with your AWS Elastic Beanstalk REST API. This file is used exclusively by Netlify, so if you are using a different platform, it will be ignored. Place this file under the /src folder, and it should contain the following contents:
[[redirects]]
from = "/*"
to = "/index.html"
status = 200
[[headers]]
for = "/*"
[headers.values]
Access-Control-Allow-Origin="*"
Access-Control-Allow-Methods="GET, POST, DELETE, OPTIONS"
Access-Control-Allow-Headers="Content-Type"
_redirects
One more file to ensure “/api” is redirected properly is the _redirects file which lives under the “public” folder, rather than the “src” folder like the other changes. Create this file if it doesn’t exist, and copy the below contents, making sure to change http://mynotes-api-env.eba-wmh9t6sz.us-east-1.elasticbeanstalk.com
with your Elastic beanstalk endpoint url.
/api/* http://mynotes-api-env.eba-wmh9t6sz.us-east-1.elasticbeanstalk.com/:splat 200
/* /index.html 200
Deploy to Netlify
Your Git/Bitbucket repository should now be ready to deploy to Netlify.
Go to Netlify and sign up or log in by linking to your source control.
On the Netlify dashboard, choose "Add new site" and select "Import an existing project" from the dropdown.
You will see a list of your projects under source control. Select your React app.
On the next screen, fill in the following fields:
Site name: This will be your domain name. Enter your desired name and if it is available, proceed or try again.
Build command: This is the command Netlify will use to build your React app. I use
npm run build
, which works for standard React apps.Publish directory: This is usually the
/build
folder. Check your repo directory and enter the correct value.
There is an option to add environment variables here (which can also be added later). If your app has important environment variables (usually found in your .env file), add them here. Note that the key format should be uppercase letters and underscores only. For example, if you have a key
my.test.key
in your .env file, you must enterMY_TEST_KEY
on Netlify. Don't worry, it will still work with your code; you don't need to change the casing or add underscores in your code.Click the Deploy button. Your app will be deployed, which usually takes a few minutes. On the overview screen, scroll down to see the progress under Production deploys. Once published, you will see the URL at the top of this page. Click it to view your live site.
Summary
Our web app is now live and pulling data from our deployed AWS REST API. We configured a proxy to let Netlify bypass HTTPS to HTTP issues. In the next article, we will add a login screen to our app and manage users with AWS Cognito!
Subscribe to my newsletter
Read articles from Bryan Barrett directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
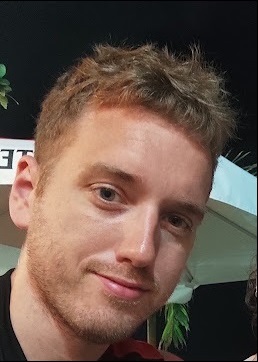