Containerizing Applications using Docker Compose, Dockerfile, and Docker Commit
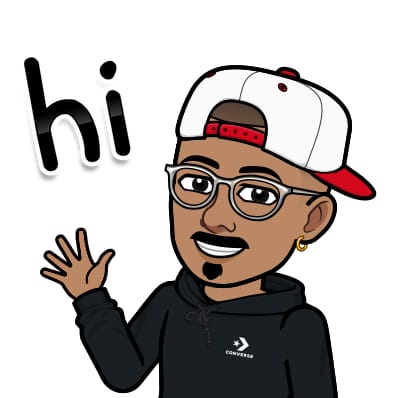
Table of contents
- Introduction
- Prerequisites
- Step-by-Step Guide
- 1. Create a Simple Flask Application
- 2. Dockerize the Flask Application Using Dockerfile
- 3. Build and Run the Docker Container
- 4. Dockerize Using Docker Compose
- 5. Dockerize Using Docker Commit
- 6. Other Methods to Containerize Applications
- Best Practices
- Conclusion

We'll walk through the process of containerizing a simple Flask application using Docker Compose, Dockerfile, and Docker commit. We'll also touch on other methods to containerize applications.
Introduction
In today's fast-paced development world, containerization has become a cornerstone for deploying applications efficiently and reliably. Docker, a leading containerization platform, offers a suite of tools to streamline this process. Let's delve into three key components: Docker Compose, Dockerfile, and Docker Commit.
Prerequisites
Basic knowledge of Flask and Python
Docker installed on your machine
Docker Compose installed on your machine
Step-by-Step Guide
1. Create a Simple Flask Application
First, let's create a simple Flask application.
Create a directory for your project:
mkdir flask_app
cd flask_app
Create a Python virtual environment:
python3 -m venv venv
source venv/bin/activate
Install Flask:
pip install Flask
Create an app.py
file with the following content:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Docker!"
if __name__ == "__main__":
app.run(host='0.0.0.0', port=5000)
Create a requirements.txt
file:
pip freeze > requirements.txt
Your project structure should look like this:
flask_app/
│
├── app.py
├── requirements.txt
└── venv/
2. Dockerize the Flask Application Using Dockerfile
Dockerfile: The Blueprint
A Dockerfile is essentially a text document containing a set of instructions on how to build a Docker image. It defines the base image, necessary dependencies, configuration settings, and commands to run when the container starts.
Create a Dockerfile
in the root of your project directory:
# Use an official Python runtime as a parent image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy the requirements file into the container
COPY requirements.txt ./
# Install the required packages
RUN pip install --no-cache-dir -r requirements.txt
# Copy the rest of the application code into the container
COPY . .
# Make port 5000 available to the world outside this container
EXPOSE 5000
# Define the command to run the application
CMD ["python", "app.py"]
3. Build and Run the Docker Container
Build the Docker image:
docker build -t flask-app .
Run the Docker container:
docker run -p 5000:5000 flask-app
Visit http://localhost:5000
in your browser, and you should see "Hello, Docker!".
4. Dockerize Using Docker Compose
Docker Compose: Orchestrating Multiple Containers
Docker Compose is a tool for defining and running multi-container Docker applications. It uses a YAML file to configure the services, networks, and volumes.
Create a docker-compose.yml
file in the root of your project directory:
version: '3.8'
services:
web:
image: nginx:latest
ports:
- "80:80"
volumes:
- ./html:/usr/share/nginx/html
networks:
- webnet
db:
image: mysql:5.7
environment:
MYSQL_ROOT_PASSWORD: example
MYSQL_DATABASE: mydb
MYSQL_USER: user
MYSQL_PASSWORD: password
volumes:
- db_data:/var/lib/mysql
networks:
- webnet
volumes:
db_data:
networks:
webnet:
Build and run the application using Docker Compose:
docker-compose up
This will build the Docker image (if not already built) and start the container.
5. Dockerize Using Docker Commit
Docker Commit: Capturing Container Changes
Docker Commit creates a new image from an existing container. It's useful for saving changes made to a running container, but it's generally discouraged for production environments due to its potential for creating image bloat.
Docker commit is useful for creating a new image from a container's changes.
Start a container from a base image:
docker run -it python:3.9-slim /bin/bash
Inside the container, install Flask and your application:
apt-get update && apt-get install -y python3-pip pip install Flask
Copy your application files to the container (assuming you have them locally):
docker cp app.py <container_id>:/usr/src/app/app.py docker cp requirements.txt <container_id>:/usr/src/app/requirements.txt
Exit the container:
exit
Commit the changes to create a new image:
docker commit <container_id> flask-app-committed
Run the new image:
docker run -p 5000:5000 flask-app-committed
6. Other Methods to Containerize Applications
Docker Swarm: A native clustering and orchestration tool for Docker. It lets you manage a group of Docker nodes as one virtual system.
Kubernetes: An open-source system for automating deployment, scaling, and management of containerized applications.
Podman: A daemonless container engine for developing, managing, and running OCI containers (OCI (Open Container Initiative) containers are a set of open standards for container formats and runtimes.) on your Linux system.
Best Practices
Utilize Dockerfiles for consistent and reproducible images.
Use Docker Compose to efficiently manage multi-container applications.
Avoid Docker Commit in production to ensure reproducibility and prevent image bloat.
Optimize image layers for size and performance.
Employ Docker volumes for persistent data management.
Keep container images updated for security and stability.
Conclusion
Docker Compose, Dockerfile, and Docker Commit are key tools for containerizing applications. Knowing their roles and best practices helps you build efficient containerized systems. Using these tools together can greatly streamline your development and deployment processes. Dockerfile and Docker Compose are popular for building and managing containers, while Docker commit is good for quick prototyping and debugging.
🎉 Happy containerizing! 🐳
Subscribe to my newsletter
Read articles from Jasai Hansda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
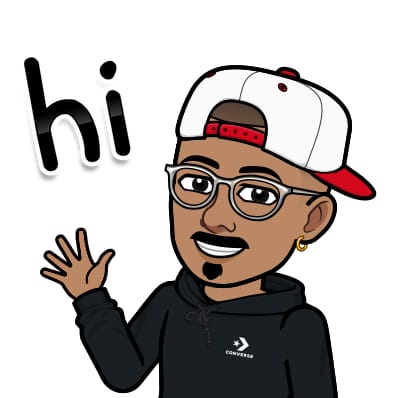
Jasai Hansda
Jasai Hansda
Software Engineer (2 years) | In-transition to DevOps. Passionate about building and deploying software efficiently. Eager to leverage my development background in the DevOps and cloud computing world. Open to new opportunities!