States and Hooks

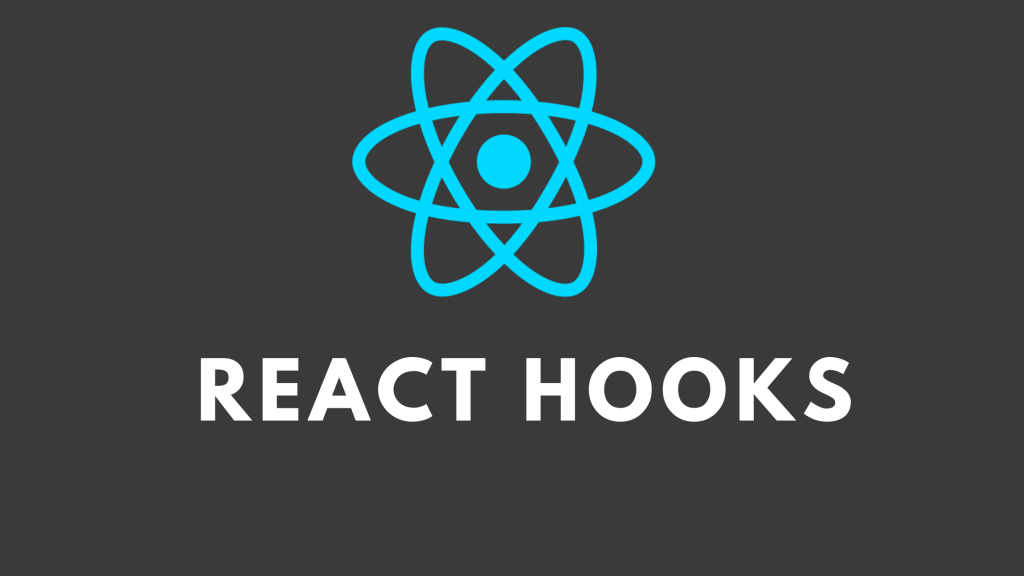
State in react:
State are used to create dynamic data on the UI.
State are mutable (Its value can change).
State are local, states belong to one particular component.
We cannot share the state between component like props.
By default FBC(Functional Based Component) are stateless it means it don't have inbuilt state objects in FBC.
But we can make FBC statefull by using a advance feature called Hooks and the Hooks which are used to make FBC statefull is "useState".
Hooks:
Hook are like inbuilt method in react.
Hooks always starts from prefix word "use".
whenever we wanted to use hooks we have to import it from react library
NOTE: "import" statement are mandatory.
Hooks are many Hooks in react these are the few below hooks
useState
useEffect
useContext
useReducer
useRef
useMemo
useCallback
Custom Hooks
useState:
It is used to add the state variable in your component
Example:
Variable Declaration: it can change it as per your demand of your code let, const.
Identifier /Current state: The current state of this variable, initially set to the initial state provider.
Method to change/Set Function: The set Function that lets you change it to any other value in response to interaction.
initial value if data: call use state at the top level of your component to declare at state variable.
Q. Write a program for passing a String inside state?
State.jsx:
import React from 'react'
import { useState } from 'react'
const State = () => {
let[data, setData]=useState("Hungry")
let eat =()=>{ setData("My Stomatch is full ")}
console.log(data);
<div>
{data}
<button onClick={eat}>Food</button>
</div>
}
export default State
Q. Write a program for passing an array inside state?
Statearr.jsx:
import React, { useState } from 'react'
const Statearr = () => {
let[data,setData]=useState(["hi","hello", "bye"])
return (
<div>
<h1>{data[0]}</h1>
<h1>{data[1]}</h1>
<h1>{data[2]}</h1>
</div>
)
}
export default Statearr
Q. Write a Program for passing an object inside state?
Stateob.jsx:
import React, { useState } from 'react'
const Stateob = () => {
let[obj,setObj]=useState({name:"John Dev"})
return (
<div>
<h1>{obj.name}</h1>
</div>
)
}
export default Stateob
Q. Write a program for increment, decrement, reset?
Task.jsx:
import React, { useState } from 'react'
const Task = () => {
let[count,setCount] =useState(0)
let incre=()=>{
setCount(count+1)
}
let decre=()=>{
setCount(count-1)
}
let reset=()=>{
setCount(0)
}
return (
<div>
<h1>{count}</h1>
<button onClick={incre}>Increment</button>
<button onClick={decre}>Decrement</button>
<button onClick={reset}>Reset</button>
</div>
)
}
export default Task
List in React:
to display data in an ordered format.
the map() function is used for traversing the list.
Create a file name userData.json and store the value provided
{
"employees":[
{
"userName":"Frontend",
"jobTitleName":"Frontend Developer",
"employeeCode":"E1",
"techstack":[
"React",
"javascript",
"html",
"css"
],
"emailAddress":"fe@gmail.com",
"image": "https://trio.dev/static/46a74b0f7c9b47353ea207a29731bc51/263a75529a1752b75d64f9f21fd07c92.jpg"
},
{
"userName":"Backend",
"jobTitleName":"Backend Developer",
"employeeCode":"E2",
"techstack":[
"React",
"Node",
"php"
],
"emailAddress":"be@gmail.com",
"image": "https://s3.studytonight.com/curious/uploads/pictures/1631204081-106730.png"
},
{
"firstName":"Fullstack",
"lastName":"Developer",
"employeeCode":"E3",
"techstack":[
"React",
"javascript",
"node",
"php",
"html",
"css"
],
"emailAddress":"fs@gmail.com",
"image": "https://www.reachfirst.com/wp-content/uploads/2018/08/Web-Development.jpg"
}
]
}
FetchData.jsx:
import React, { useState } from 'react';
import context from '../userData.json';
const FetchData = () => {
const [user, setUser] = useState(context.employees);
return (
<div>
{user && user.map((x, index) => (
<div key={index}>
<h1>{x.employeeCode}</h1>
<h1>{x.userName}</h1>
<img src={x.image} alt={x.userName} />
</div>
))}
</div>
);
}
export default FetchData;
useEffect():
The useEffect()
Hook allows you to perform side effects in your components.
Some examples of side effects are: fetching data, directly updating the DOM, and timers.
useEffect
accepts two arguments. The second argument is optional.
useEffect(<function>, <dependency>)
Task.jsx:
import React from "react";
import { useState, useEffect } from "react";
const Task4 = () => {
const [count, setCount] = useState(0);
useEffect(() => {
setTimeout(() => {
setCount((count) => count + 1);
},[]);
});
return (
<div>
<h1>I've rendered {count} times!</h1>
</div>
)
}
export default Task4
Variation of use Effect:
Without dependency
Empty dependency
Passing a dependency array
Without dependency:
It runs with first render and also run on any thing changes in that component.
useEffect(() => {
// ...
}); // Always runs again
Empty dependency:
It runs only on first render. Useful for Fetching data.
useEffect(() => {
// ...
}, []); // Does not run again (except once in development)
Passing a dependency array:
It runs on first render and then any variable that we pass in dependency change it will run.
useEffect(() => {
// ...
}, [a, b]); // Runs again if a or b are different
Clean-up Function in useEffect:
If we are setting state using setInterval and that side effect is not cleaned up, when our component unmounts or we're no longer using it, the state is destroyed with the component – but the setInterval function will keep running. and that’s make our application slow and low in performance.
To use clean-up function we need to run return function in useEffect. Timeouts, subscriptions, event listeners, and other effects that are no longer needed should be disposed with the help of cleanup function.
its Example:
import React, { useEffect, useState } from "react";
const App = () => {
const [count, setCount] = useState(0);
useEffect(() => {
console.log("Run useEffect", count);
return () => { // clean-up function
console.log("Clean up", count);
};
}, [count]);
return (
<div>
<h3>Count {count}</h3>
<button onClick={() => setCount(count + 1)}>Increase</button>
</div>
);
};
export default App;
useContext:
useContext is a way to manage the state globally.
if we have a nested components and want to pass a data from parent component to nested child component, then we have to send that data to all the child component which would be unnecessary for other component.
to solve this problem we use useContext hook which directly send the data from parent to whatever child component needed that data.
How to create useContext?
There are 3 steps to create useContext:
Creating the context
Providing the context
Consuming the context
App.jsx:
import React, { createContext } from "react"; import MainComponent from "./component/MainComponent"; export const LoginContext = createContext(); function App() { return ( <LoginContext.Provider value={true}> <div className="App"> <MainComponent /> </div> </LoginContext.Provider> ); } export default App;
MainComponent.jsx:
import React from 'react' import SinglePost from './SinglePost' const MainComponent = () => { return ( <div> <SinglePost/> </div> ) } export default MainComponent
SinglePost.jsx:
import React, { useContext } from 'react' import { LoginContext } from '../App'; const SinglePost = () => { const login=useContext(LoginContext); console.log(login); return ( <div>SinglePost</div> ) } export default SinglePost
I have transferred the "value={true}" from App.jsx to SinglePost.jsx using useContext.
For Creating the context in App.jsx I have created LoginContext.
For Providing the Context we have to use :
<LoginContext.Provider value={true}></LoginContext.Provider>
as I have usedFor Consuming the context in SinglePost.jsx I have used this:
const login=useContext(LoginContext);
If you want to pass the data just for the child component, then you can use props
.
Don't use this most of the time to call the data from parent to child using useContext hook.
Subscribe to my newsletter
Read articles from Nitin Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
