How to Implement Pagination in Your Flask Applications for Better User Experience
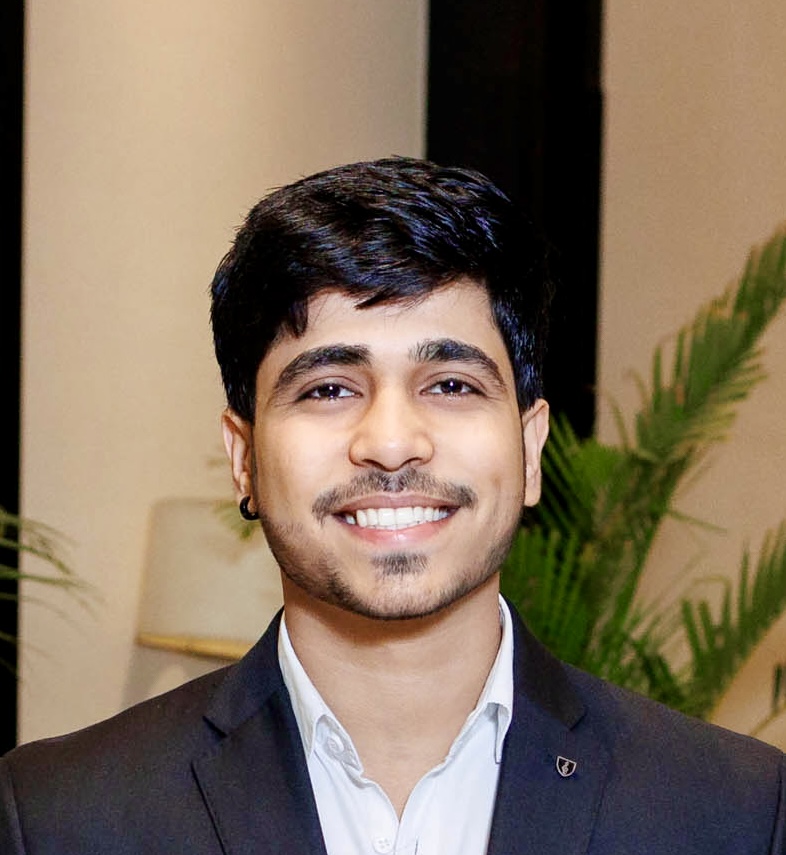
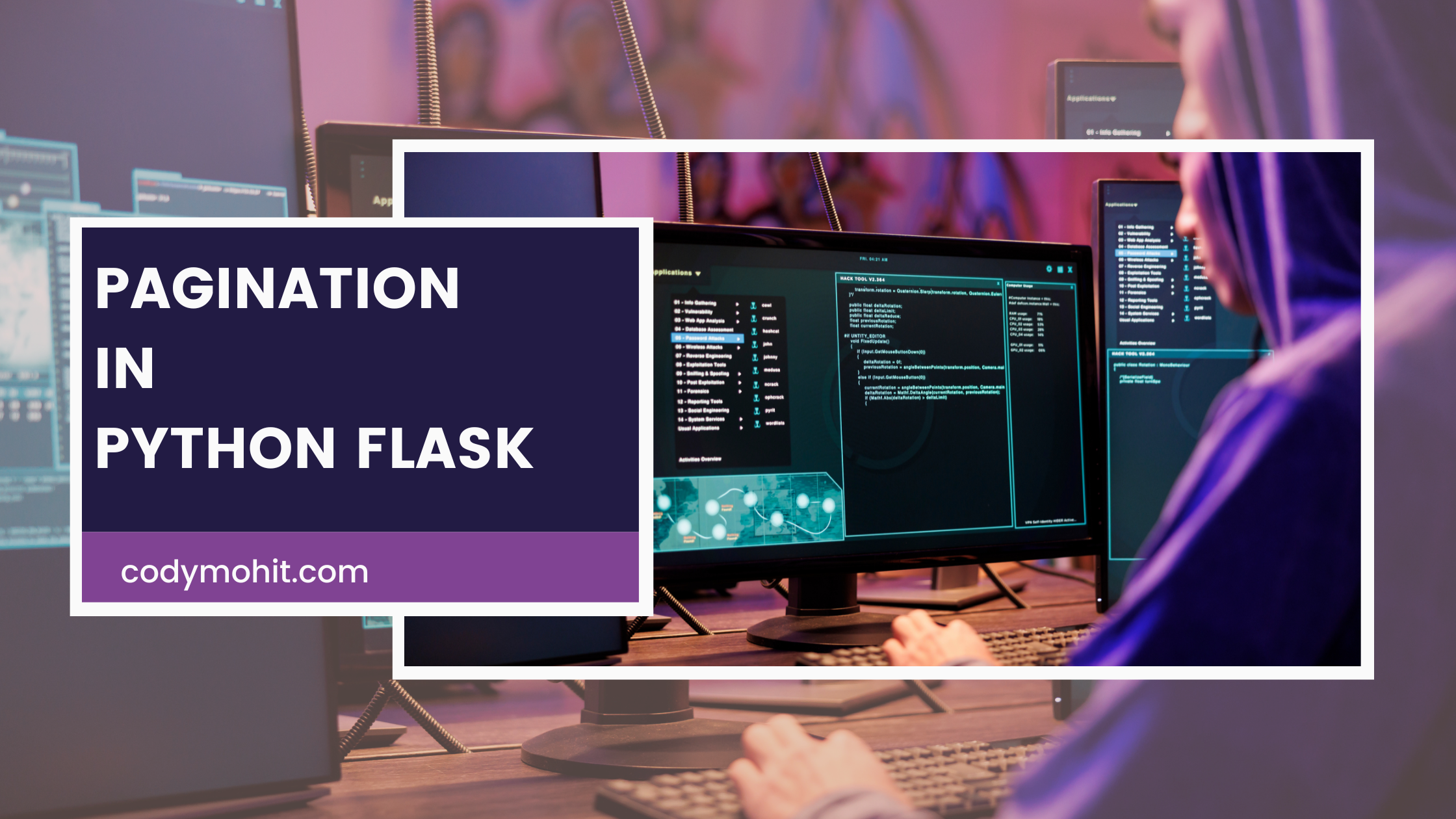
Are your Flask applications feeling a bit sluggish? If you've ever wrestled with endless lists of data, you know that too much information on one page can be overwhelming and slow. 😓 But fear not! Implementing pagination in your Flask app isn't just a technical tweak—it's a game-changer for enhancing user experience and boosting performance. 🚀
In this blog, we'll dive into the nitty-gritty of pagination—that magical technique that breaks down large sets of data into bite-sized, manageable chunks. From understanding why pagination is crucial for user experience to step-by-step instructions on setting it up in Flask, we’ve got you covered. So, buckle up and get ready to transform your app’s performance and user interaction!
Introduction to Pagination in Web Applications
Pagination is a technique used in web applications to divide a large set of data into smaller, manageable chunks. Instead of displaying all data at once, which can be overwhelming and slow, pagination breaks the data into pages. This way, users can navigate through the pages to view different portions of the data.
Definition and Importance
Pagination is essentially the process of splitting a large dataset into pages or sections. This technique is crucial for several reasons:
Performance: Loading a massive amount of data all at once can significantly slow down your web application. Pagination helps to reduce the amount of data loaded at any given time, which improves the performance and speed of the application.
Usability: Pagination makes it easier for users to find and view the information they’re looking for. Without pagination, users might have to scroll endlessly, which can be frustrating and inefficient.
Resource Management: By loading only a subset of data at a time, pagination helps in managing server resources more efficiently. This reduces the load on your server and network, leading to a smoother user experience.
Impact on User Experience
The impact of pagination on user experience is significant:
Faster Load Times: Users can access the information faster since they’re not waiting for the entire dataset to load. This is especially important for mobile users who may have slower internet connections.
Enhanced Navigation: Pagination provides a clear structure for users to follow. They can easily navigate between pages using previous and next buttons or page numbers, which makes the browsing experience more intuitive.
Reduced Overwhelm: By breaking down information into smaller chunks, users are less likely to feel overwhelmed by a wall of text or data. This makes the content easier to digest and interact with.
Example Code for Pagination
Here’s a simple example of how pagination might be implemented in a Flask application using SQLAlchemy. This example assumes you have a model named Item
and you want to paginate a list of items.
from flask import Flask, request, render_template
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db'
db = SQLAlchemy(app)
class Item(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50), nullable=False)
@app.route('/items')
def items():
# Get the page number from the URL query parameters, default to 1
page = request.args.get('page', 1, type=int)
# Set the number of items per page
per_page = 10
# Query the items and paginate
paginated_items = Item.query.paginate(page, per_page, error_out=False)
return render_template('items.html', items=paginated_items.items, pagination=paginated_items)
if __name__ == '__main__':
app.run(debug=True)
In this code:
paginate()
method is used to split the query results into pages.page
andper_page
are parameters used to control the pagination.
Note: This example assumes you have a basic HTML template (items.html
) to render the paginated items. You would need to create pagination controls in your HTML to allow users to navigate between pages.
Why Pagination Matters
Pagination is a technique used in web applications to divide large sets of data into smaller, manageable chunks. This approach isn’t just about making your content look neat; it has a significant impact on user experience and performance.
Enhancing User Experience
Reducing Load Time:
Loading a huge list of items all at once can slow down your page and make it feel sluggish. By using pagination, you only load a small portion of data at a time, which helps in reducing the initial load time. Imagine trying to load a giant book in one go versus flipping through it page by page—pagination is like flipping pages, making it faster and less overwhelming.# Example in Flask using SQLAlchemy @app.route('/items') def items(): page = request.args.get('page', 1, type=int) per_page = 10 items = Item.query.paginate(page, per_page, False) return render_template('items.html', items=items)
Simplifying Navigation:
Pagination makes it easier for users to navigate through large datasets. Instead of scrolling endlessly, users can jump between pages or use next/previous buttons. It’s like having a table of contents in a book that helps you find the section you want without flipping through every single page.
Improving Performance
Efficient Data Handling:
Handling data in chunks rather than all at once is much more efficient. When you paginate, the server only processes and sends the necessary data for each page. This way, you reduce the amount of data that needs to be processed and transferred at any one time, leading to a smoother experience.# Example of pagination handling in SQLAlchemy @app.route('/items') def items(): page = request.args.get('page', 1, type=int) per_page = 10 items = Item.query.offset((page - 1) * per_page).limit(per_page).all() return render_template('items.html', items=items)
Decreasing Server Load:
Loading and serving smaller chunks of data reduces the load on the server. When a page request only involves a subset of the entire dataset, the server can handle the request more efficiently, freeing up resources for other tasks. Think of it like managing a warehouse: it’s easier to handle and organize small boxes than a massive shipment all at once.
Basic Concepts of Pagination
Pagination vs. Infinite Scrolling
Pagination: This method divides content into distinct pages. Users navigate through these pages using "Next" and "Previous" buttons or page numbers. It's often seen in search results or large lists.
Example: Search results on Google where you see page numbers at the bottom to jump between different sets of results.
Infinite Scrolling: In this approach, new content loads automatically as the user scrolls down. There are no distinct pages; instead, more data is fetched and displayed as needed. It's commonly used in social media feeds or image galleries.
Example: Facebook or Twitter feeds where new posts appear as you scroll down.
How Pagination Works
Pagination Logic
Determine Total Number of Items: First, you need to know how many items you have. This helps you figure out how many pages you'll need.
Define Page Size: Decide how many items you want to show per page (e.g., 10 items per page).
Calculate Total Pages: Divide the total number of items by the page size to get the number of pages. If you have 100 items and show 10 per page, you'll have 10 pages.
Fetch Data for Current Page: Based on the user's current page, fetch and display the relevant data. For example, if the user is on page 3, you show items 21-30.
Common Pagination Techniques
Offset-Based Pagination: This technique uses an offset to skip a certain number of items and retrieve a subset. For example, to get page 3 with 10 items per page, the offset would be 20 (since 2 pages * 10 items = 20).
SQL Example:
SELECT * FROM items LIMIT 10 OFFSET 20;
Cursor-Based Pagination: Also known as keyset pagination, this technique uses a unique identifier (like a timestamp or ID) to fetch the next set of items. It's often more efficient for large datasets.
SQL Example:
SELECT * FROM items WHERE id > last_seen_id ORDER BY id ASC LIMIT 10;
Page Number-Based Pagination: This method uses a page number to fetch data. It's simple but can be less efficient for very large datasets as it might involve scanning many rows.
SQL Example:
SELECT * FROM items LIMIT 10 OFFSET (page_number - 1) * 10;
Code Example for Basic Pagination
Here's a basic example in Python using Flask and SQLAlchemy to implement pagination:
from flask import Flask, request, jsonify
from models import db, Item # Assuming you have a SQLAlchemy model called Item
app = Flask(__name__)
@app.route('/items', methods=['GET'])
def get_items():
page = request.args.get('page', 1, type=int)
per_page = request.args.get('per_page', 10, type=int)
items_query = Item.query.paginate(page, per_page, False)
items = [item.to_dict() for item in items_query.items] # Assuming you have a to_dict method in your model
return jsonify({
'items': items,
'total': items_query.total,
'pages': items_query.pages,
'current_page': items_query.page
})
if __name__ == '__main__':
app.run(debug=True)
In this example, we use Flask to handle HTTP requests and SQLAlchemy to manage database queries. The paginate
method handles the pagination logic, making it easier to retrieve only the items needed for the current page.
Setting Up Pagination in Flask
Pagination is a technique used in web applications to divide large sets of data into smaller, more manageable chunks. It improves user experience by ensuring that users don't have to load an overwhelming amount of data all at once. In Flask, setting up pagination involves a few key steps. Let's walk through how to set it up, starting from scratch.
Prerequisites
Flask Installation: Make sure Flask is installed in your environment. You can install it using pip if it's not already there:
pip install flask
Basic Knowledge of Flask Routing: Knowing how to set up routes in Flask is essential since pagination will be implemented within these routes.
Basic Flask Setup
Creating a New Flask Project
First, create a new directory for your Flask project and navigate into it. Create a new Python file, for instance,
app.py
.mkdir flask_pagination cd flask_pagination touch app.py
Setting Up Flask Routes
In
app.py
, start by setting up a basic Flask application with a route to display data. For this example, let's assume we have a list of items that we want to paginate.from flask import Flask, request, render_template app = Flask(__name__) # Sample data - in real applications, this would come from a database ITEMS = [f'Item {i}' for i in range(1, 101)] # 100 items @app.route('/') def index(): # Get the page number from query parameters; default is 1 page = request.args.get('page', 1, type=int) per_page = 10 # Number of items per page start = (page - 1) * per_page end = start + per_page # Slice the list to get the items for the current page paginated_items = ITEMS[start:end] # Calculate the total number of pages total_pages = (len(ITEMS) + per_page - 1) // per_page return render_template('index.html', items=paginated_items, page=page, total_pages=total_pages) if __name__ == '__main__': app.run(debug=True)
In this setup:
We use
request.args.get('page', 1, type=int)
to get the page number from the query string. It defaults to page 1 if not specified.per_page
is the number of items displayed per page.We calculate the start and end indices for slicing the list of items.
We also calculate the total number of pages to help with pagination navigation.
Creating the Template
Create a template named index.html
in a templates
folder. This file will render the paginated items and provide links to navigate between pages.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Pagination Example</title>
</head>
<body>
<h1>Items</h1>
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
<div>
{% if page > 1 %}
<a href="?page={{ page - 1 }}">Previous</a>
{% endif %}
{% if page < total_pages %}
<a href="?page={{ page + 1 }}">Next</a>
{% endif %}
</div>
</body>
</html>
In this template:
We list all items for the current page.
We provide "Previous" and "Next" links to navigate through the pages. These links adjust the
page
parameter in the URL.
Implementing Pagination in Flask
Using Flask-SQLAlchemy for Pagination
Flask-SQLAlchemy is an extension for Flask that simplifies working with SQLAlchemy, a powerful SQL toolkit and Object Relational Mapper (ORM). It makes integrating SQLAlchemy with Flask a breeze, providing a high-level API for database operations.
Example of Pagination with SQLAlchemy
To implement pagination with Flask-SQLAlchemy, you can use the paginate
method provided by SQLAlchemy. Here’s how to do it:
Setup Flask and Flask-SQLAlchemy:
First, you need to have Flask and Flask-SQLAlchemy installed. If you haven’t done so, you can install them using pip:
pip install Flask Flask-SQLAlchemy
Define your Flask application and SQLAlchemy setup:
from flask import Flask, request, jsonify from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db' db = SQLAlchemy(app) class Item(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(80), unique=True, nullable=False) @app.route('/items', methods=['GET']) def get_items(): page = request.args.get('page', 1, type=int) per_page = request.args.get('per_page', 10, type=int) pagination = Item.query.paginate(page, per_page, error_out=False) items = pagination.items return jsonify({'items': [item.name for item in items], 'total': pagination.total})
In this example:
paginate(page, per_page, error_out=False)
fetches a specific page of items from the database.page
andper_page
are obtained from the query parameters.
Manual Pagination with SQL Queries
Sometimes, you may want more control over your pagination, or you may not be using an ORM. In such cases, you can write custom SQL queries.
Example Code for Manual Pagination
Here’s how you can implement manual pagination:
Setup Flask and SQLite:
from flask import Flask, request, jsonify import sqlite3 app = Flask(__name__) def get_db_connection(): conn = sqlite3.connect('example.db') conn.row_factory = sqlite3.Row return conn @app.route('/items', methods=['GET']) def get_items(): page = request.args.get('page', 1, type=int) per_page = request.args.get('per_page', 10, type=int) offset = (page - 1) * per_page conn = get_db_connection() items = conn.execute('SELECT * FROM item LIMIT ? OFFSET ?', (per_page, offset)).fetchall() conn.close() return jsonify({'items': [dict(item) for item in items]})
In this example:
LIMIT ? OFFSET ?
is used to fetch a specific subset of results.offset
is calculated based on the current page and items per page.
Conclusion
Pagination is essential for efficiently managing large datasets and improving user experience. Whether you use Flask-SQLAlchemy for a more integrated approach or write custom SQL queries for flexibility, both methods have their use cases. By implementing pagination, you ensure your Flask application remains performant and user-friendly.
Pagination Techniques and Best Practices
Pagination is a technique used in web applications to split large sets of data into smaller, more manageable chunks. This not only improves the user experience but also helps in optimizing performance. Here’s a guide on pagination techniques, best practices, and how to handle large datasets.
Page Number vs. Offset-Based Pagination
Page Number Pagination
This technique divides your data into pages. Each page has a specific number, and users can navigate to different pages by clicking on page numbers.
Example:
def get_page_data(page_number, page_size):
offset = (page_number - 1) * page_size
query = "SELECT * FROM items LIMIT %s OFFSET %s"
return db.execute(query, (page_size, offset))
When to Use Page Number Pagination:
User-Friendly: It’s intuitive for users as they can jump to a specific page easily.
Small to Medium Datasets: It’s good for datasets that don’t change often and aren’t too large.
Offset-Based Pagination
Offset-based pagination also uses limits but relies on an offset value to fetch data. This is usually used with an offset parameter to fetch the next chunk of data.
Example:
def get_offset_data(offset, limit):
query = "SELECT * FROM items LIMIT %s OFFSET %s"
return db.execute(query, (limit, offset))
When to Use Offset-Based Pagination:
Dynamic Datasets: Better for situations where the dataset can change frequently.
Efficient for Smaller Pages: Suitable for smaller pages where data doesn’t change much between page loads.
Comparing Both:
Page Number Pagination is generally easier for users to navigate but can become less efficient with very large datasets due to increased computation as the page number grows.
Offset-Based Pagination can be more efficient for large datasets but may become less intuitive for users who need to navigate to specific pages.
Handling Large Datasets
Strategies for Efficient Pagination
Cursor-Based Pagination: Instead of using offset, use a unique identifier (like an ID) to keep track of the last item. This is often more efficient for large datasets.
Example:
def get_cursor_data(last_id, limit): query = "SELECT * FROM items WHERE id > %s LIMIT %s" return db.execute(query, (last_id, limit))
Keyset Pagination: This is similar to cursor-based but uses a more complex key to handle pagination, especially in composite scenarios.
Performance Optimization Tips
Indexes: Make sure your database tables are properly indexed on the columns used in pagination queries. For example, indexing the
id
column if you’re using cursor-based pagination.Avoid Large Offsets: Large offsets can degrade performance as the database has to scan through many rows. Cursor-based or keyset pagination helps avoid this issue.
Database Caching: Implement caching strategies to store frequently accessed pages. This can reduce the load on the database and improve response times.
Limit Data Fetching: Always fetch only the necessary columns and rows. For instance, avoid using
SELECT *
in queries. Instead, select only the columns you need.
Summary
Page Number Pagination is user-friendly but can be less efficient for large datasets.
Offset-Based Pagination is more adaptable for dynamic data but less intuitive.
Cursor-Based Pagination is efficient for large datasets and ensures better performance.
Implement indexes, avoid large offsets, use caching, and limit data fetching to optimize performance.
Remember, choosing the right pagination technique depends on your specific use case, dataset size, and performance requirements. Happy paginating!
Integrating Pagination with Flask Templates
Pagination helps users navigate through large sets of data by breaking them into manageable chunks. When integrating pagination with Flask templates, you create navigation controls to allow users to move between pages easily. Let’s break down how to achieve this, including designing navigation links and styling them.
Creating Pagination Controls
Designing Navigation Links
To create pagination controls in your Flask application, you need to provide navigation links in your HTML templates. These links will typically include options to go to the previous page, next page, and specific pages (like 1, 2, 3, etc.).
Here’s a basic example of how you might set up your pagination controls using HTML and Jinja2 in a Flask template:
<nav aria-label="Page navigation"> <ul class="pagination"> {% if pagination.has_prev %} <li class="page-item"> <a class="page-link" href="{{ url_for('your_view', page=pagination.prev_num) }}" aria-label="Previous"> <span aria-hidden="true">«</span> </a> </li> {% endif %} {% for page_num in pagination.iter_pages() %} {% if page_num %} {% if page_num == pagination.page %} <li class="page-item active" aria-current="page"> <span class="page-link">{{ page_num }}</span> </li> {% else %} <li class="page-item"> <a class="page-link" href="{{ url_for('your_view', page=page_num) }}">{{ page_num }}</a> </li> {% endif %} {% endif %} {% endfor %} {% if pagination.has_next %} <li class="page-item"> <a class="page-link" href="{{ url_for('your_view', page=pagination.next_num) }}" aria-label="Next"> <span aria-hidden="true">»</span> </a> </li> {% endif %} </ul> </nav>
In this code:
pagination.has_prev
andpagination.has_next
check if there are previous or next pages available.pagination.iter_pages()
generates a list of page numbers for the pagination controls.url_for('your_view', page=page_num)
generates URLs for each page.
Example HTML and Jinja2 Code
Let's assume you have a
paginate
object passed to your template. Here’s a simplified example of how you might use this object to create pagination controls:<nav> <ul class="pagination"> {% if paginate.has_prev %} <li class="page-item"> <a class="page-link" href="{{ url_for('index', page=paginate.prev_num) }}">Previous</a> </li> {% endif %} {% for page in paginate.iter_pages() %} {% if page %} {% if page == paginate.page %} <li class="page-item active"><span class="page-link">{{ page }}</span></li> {% else %} <li class="page-item"><a class="page-link" href="{{ url_for('index', page=page) }}">{{ page }}</a></li> {% endif %} {% endif %} {% endfor %} {% if paginate.has_next %} <li class="page-item"> <a class="page-link" href="{{ url_for('index', page=paginate.next_num) }}">Next</a> </li> {% endif %} </ul> </nav>
Styling Pagination Controls
CSS Tips for Pagination
Styling your pagination controls helps make them more visually appealing and user-friendly. Here are some tips:
Consistency: Use consistent colors and sizes that match your site’s theme.
Visibility: Ensure the links are easily clickable and have enough padding.
Feedback: Highlight the current page to make it clear to users where they are.
Example CSS to style the pagination controls:
.pagination {
display: flex;
list-style: none;
padding: 0;
margin: 0;
}
.pagination .page-item {
margin: 0 5px;
}
.pagination .page-link {
display: block;
padding: 10px 15px;
border: 1px solid #ddd;
text-decoration: none;
color: #007bff;
}
.pagination .page-item.active .page-link {
background-color: #007bff;
color: white;
border-color: #007bff;
}
.pagination .page-link:hover {
background-color: #e9ecef;
}
Examples of Pagination Styles
Here are a couple of examples to visualize different styles of pagination:
Basic Style: Simple borders and padding for a clean look.
Modern Style: Use of shadows and rounded corners for a more contemporary feel.
Example of a basic style:
<style>
.pagination {
display: inline-block;
}
.pagination li {
display: inline;
margin: 0 5px;
}
.pagination a, .pagination span {
display: inline-block;
padding: 10px 15px;
border: 1px solid #ddd;
color: #007bff;
text-decoration: none;
}
.pagination .active {
background-color: #007bff;
color: white;
border-color: #007bff;
}
.pagination a:hover {
background-color: #e9ecef;
}
</style>
Example of a modern style:
<style>
.pagination {
display: flex;
justify-content: center;
padding: 0;
}
.pagination .page-item {
margin: 0 2px;
}
.pagination .page-link {
padding: 10px 20px;
border-radius: 50%;
border: 1px solid #ddd;
color: #007bff;
text-decoration: none;
background-color: #f8f9fa;
}
.pagination .active .page-link {
background-color: #007bff;
color: white;
border-color: #007bff;
}
.pagination .page-link:hover {
background-color: #e9ecef;
}
</style>
By integrating pagination with Flask templates and applying these styling tips, you can create an intuitive and visually appealing navigation experience for users browsing through paginated data.
Error Handling and Edge Cases
When working with pagination in web applications, it's important to handle errors and edge cases gracefully to ensure a smooth user experience. Here are some key considerations:
Handling Empty Results
Displaying Messages for No Data
Sometimes, a query might return no results. Instead of showing a blank page or confusing the user, it's best to display a friendly message indicating that no data is available. This improves the user experience and provides clear feedback.
Example Code:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/items/<int:page>')
def items(page):
items_per_page = 10
all_items = get_all_items() # Function to retrieve all items from the database
total_items = len(all_items)
# Calculate the starting and ending indices for the current page
start = (page - 1) * items_per_page
end = start + items_per_page
# Slice the list to get items for the current page
current_page_items = all_items[start:end]
# Check if there are no items on the current page
if not current_page_items:
return render_template('no_data.html') # Render a template with a "No data available" message
return render_template('items.html', items=current_page_items)
def get_all_items():
# This function should interact with your database to fetch items
return []
if __name__ == '__main__':
app.run()
Explanation:
get_all_items()
is a placeholder for fetching items from your database.The
items
function calculates which items to show based on the page number and items per page.If
current_page_items
is empty, it renders a templateno_data.html
to show a message like "No data available."
Validating Page Numbers
Ensuring Page Numbers are Within Range
To avoid errors when users request a page number that's out of range (like -1 or 1000), you need to validate the page number and handle invalid requests appropriately. This prevents errors and improves reliability.
Example Code:
from flask import Flask, render_template, abort
app = Flask(__name__)
@app.route('/items/<int:page>')
def items(page):
items_per_page = 10
all_items = get_all_items() # Function to retrieve all items from the database
total_items = len(all_items)
# Calculate total number of pages
total_pages = (total_items + items_per_page - 1) // items_per_page
# Validate page number
if page < 1 or page > total_pages:
abort(404) # Return a 404 error for invalid page numbers
# Calculate the starting and ending indices for the current page
start = (page - 1) * items_per_page
end = start + items_per_page
# Slice the list to get items for the current page
current_page_items = all_items[start:end]
# Check if there are no items on the current page
if not current_page_items:
return render_template('no_data.html') # Render a template with a "No data available" message
return render_template('items.html', items=current_page_items)
def get_all_items():
# This function should interact with your database to fetch items
return []
if __name__ == '__main__':
app.run()
Explanation:
We calculate the total number of pages by dividing the total number of items by the number of items per page.
We then check if the requested page number is within the valid range. If not, we use
abort(404)
to display a 404 error page.
FREQUENTLY ASKED QUESTIONS:
What is the difference between page number and offset-based pagination?
Page number pagination involves requesting data based on a specific page number and page size. For example, if you request page 2 with a page size of 10, you'll get items 11-20. Offset-based pagination, on the other hand, uses an offset to determine which records to fetch. If you request an offset of 10 with a limit of 10, you get items 11-20. The main difference is that page number pagination is generally easier to understand, while offset-based pagination can be more flexible for scenarios like infinite scrolling.
How can I optimize pagination for very large datasets?
For very large datasets, consider using cursor-based pagination instead of traditional page number or offset-based methods. Cursor-based pagination uses a unique identifier (like a database ID) to fetch records relative to a certain point. This method is efficient because it avoids the performance hit of counting rows or skipping large offsets. Indexing relevant columns in your database can also help improve performance and reduce query time.
What libraries can assist with pagination in Flask?
In Flask, you can use libraries like Flask-Paginate
or Flask-SQLAlchemy
(with its built-in pagination methods). Flask-Paginate
provides an easy way to create pagination controls in your templates, while Flask-SQLAlchemy
offers pagination methods directly on query objects. Both libraries simplify implementing pagination and managing page controls in your web applications.
How do I handle pagination for API responses in Flask?
To handle pagination for API responses in Flask, you can use query parameters to specify pagination details, such as page
and per_page
. For example, your API endpoint might accept ?page=2&per_page=10
to return the second page with ten items per page. You can then calculate the pagination offset and limit in your view function and return the paginated results along with metadata like total items and total pages.
What are common performance issues with pagination?
Common performance issues with pagination include slow queries due to large offsets or inefficient indexing, and excessive memory usage if the dataset is very large. Additionally, page number pagination can become inefficient if users frequently jump to high-numbered pages, as it may require scanning a lot of rows. Optimizing queries, using proper indexing, and employing cursor-based pagination can help mitigate these issues.
How can I customize pagination controls in Flask templates?
To customize pagination controls in Flask templates, you can use Flask-Paginate
or manually create pagination controls in your HTML. For Flask-Paginate
, you can override the default template by providing your own template files that define how pagination links should be displayed. For manual customization, create pagination controls with HTML and Jinja2, and style them with CSS to match your application's design.
What are some best practices for designing pagination controls?
Best practices for designing pagination controls include ensuring that the controls are intuitive and easy to use, providing clear navigation options like "Next," "Previous," and page numbers. It’s also useful to display a summary of results, such as "Showing 1-10 of 50 items." Make sure to handle edge cases, such as displaying disabled controls when there are no more pages. Accessibility considerations, like keyboard navigation and screen reader support, are also important.
How do I test pagination functionality in Flask applications?
To test pagination functionality in Flask applications, write unit tests and integration tests that check various scenarios, such as valid page numbers, edge cases (like the first and last pages), and invalid requests (e.g., negative page numbers). Ensure that your tests cover both the API endpoints and the UI components (if applicable). Use testing frameworks like pytest
along with Flask’s testing utilities to simulate requests and verify the correctness of pagination logic.
What should I do if pagination links are not working correctly?
If pagination links are not working correctly, first check the logic used to generate links and ensure that the URLs are being constructed with the correct parameters. Verify that the backend is handling pagination parameters properly and returning the expected results. Also, inspect your templates to ensure that pagination controls are correctly wired to the backend logic. Debugging tools and logging can help identify where the issue might be occurring.
How can I implement dynamic pagination with asynchronous loading?
To implement dynamic pagination with asynchronous loading, use JavaScript (e.g., with libraries like Axios or Fetch API) to load more data without refreshing the entire page. When a user scrolls to the bottom or clicks a "Load More" button, send an asynchronous request to fetch the next set of items. Update the page content dynamically based on the response. This approach provides a smoother user experience by loading data incrementally and avoiding full-page reloads.
Subscribe to my newsletter
Read articles from Mohit Bhatt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
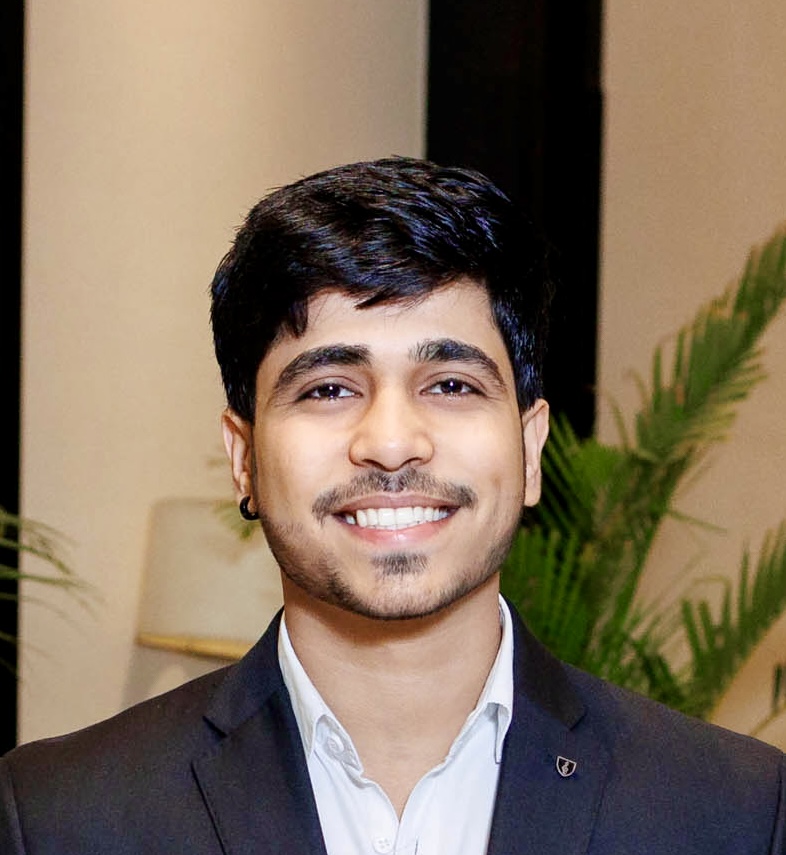
Mohit Bhatt
Mohit Bhatt
As a dedicated and skilled Python developer, I bring a robust background in software development and a passion for creating efficient, scalable, and maintainable code. With extensive experience in web development, Rest APIs., I have a proven track record of delivering high-quality solutions that meet client needs and drive business success. My expertise spans various frameworks and libraries, like Flask allowing me to tackle diverse challenges and contribute to innovative projects. Committed to continuous learning and staying current with industry trends, I thrive in collaborative environments where I can leverage my technical skills to build impactful software.