Spring AOP: A Practical Approach to Logging and Auditing
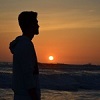
Aspect-Oriented Programming (AOP) complements Object-Oriented Programming (OOP) by allowing developers to separate cross-cutting concerns from the business logic. In the context of the Spring Framework, AOP proves particularly useful for logging and auditing, enabling developers to add functionality across various parts of an application without cluttering the core logic. This article explores how to use Spring AOP effectively for logging and auditing, providing practical insights into its implementation and benefits.
Understanding AOP Concepts
Before diving into practical applications, it’s essential to grasp some core AOP concepts:
Aspect
An aspect is a module that encapsulates a cross-cutting concern. For example, a logging aspect defines how logging should occur throughout an application.
Join Point
A join point is a specific point in the execution of the program, such as method execution or exception handling, where an aspect can be applied.
Advice
Advice refers to the action taken by an aspect at a particular join point. There are several types of advice:
Before: Executed before a join point.
After: Executed after a join point, regardless of the outcome.
After Returning: Executed only if a join point completes successfully.
After Throwing: Executed if a join point throws an exception.
Around: Wraps a join point, allowing code to execute both before and after the join point.
Pointcut
A pointcut is an expression that defines a set of join points where advice should be applied. It acts as a filter for join points.
Weaving
Weaving is the process of linking aspects with the main codebase, which can occur at compile time, load time, or runtime.
Benefits of Using Spring AOP for Logging and Auditing
Using Spring AOP for logging and auditing offers several advantages:
Separation of Concerns: This approach keeps logging and auditing logic separate from business logic, resulting in cleaner and more maintainable code.
Reusability: Once a developer creates an aspect, they can reuse it across different parts of the application.
Configurability: Spring AOP allows for easy configuration and modification of aspects without affecting the core application code.
Granular Control: Developers can target specific methods or classes for logging and auditing, offering precise control over what gets logged.
For those seeking to enhance their understanding of Java and AOP, java training classes in Gurgaon, Delhi, Pune and other parts of India can provide valuable insights into these concepts, along with practical experience.
Practical Implementation of Spring AOP for Logging
Let’s discuss how to implement logging using Spring AOP. The steps outlined below represent a typical approach:
1. Add the Spring AOP Dependency
To use Spring AOP, include the Spring AOP dependency in your project. If you’re using Maven, add the following to your pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
2. Create a Logging Aspect
Next, create a logging aspect that contains the advice specifying what to log and when to log it.
java
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
private static final Logger logger = LoggerFactory.getLogger(LoggingAspect.class);
@Before("execution(* com.example.service.*.*(..))")
public void logBeforeMethod() {
logger.info("A method in the service layer is about to be executed.");
}
}
3. Define Pointcuts
In the example above, the pointcut expression execution(* com.example.service.*.*(..)) targets all methods within classes in the com.example.service package. You can customize this expression to target specific classes or methods based on your needs.
4. Apply Additional Advice
In addition to @Before, consider adding other types of advice to enhance your logging:
Use @After to log after method execution.
Use @AfterReturning to log the return value of methods.
Use @AfterThrowing to log exceptions thrown by methods.
5. Test the Aspect
To ensure that the logging aspect functions correctly, invoke a method from the targeted service classes and observe the logs. The messages should print to the console or your log file, depending on your logging configuration.
Auditing with Spring AOP
Auditing works similarly to logging but focuses on tracking changes to data and actions taken by users. Here’s how to implement auditing using Spring AOP:
1. Create an Auditing Aspect
Create a separate aspect for auditing actions such as creating, updating, or deleting records:
java
@Aspect
@Component
public class AuditingAspect {
private static final Logger logger = LoggerFactory.getLogger(AuditingAspect.class);
@AfterReturning("execution(* com.example.repository.*.save(..))")
public void auditAfterSave(JoinPoint joinPoint) {
logger.info("Saved entity: " + joinPoint.getArgs()[0]);
}
}
2. Customise Auditing Logic
Adjust the pointcuts and advice based on your auditing requirements. Consider including user information, timestamps, and other relevant data to create a comprehensive audit trail.
3. Configure Auditing
If you are using Spring Data JPA, leverage its built-in auditing features. This allows for the automatic population of fields like "created by," "created date," "modified by," and "modified date."
Conclusion
Spring AOP provides a powerful mechanism for logging and auditing in Java applications. By separating these concerns from core business logic, developers can create cleaner, more maintainable code. With the ability to define aspects, pointcuts, and advice, you gain fine-grained control over how and when logging and auditing occurs. Whether tracking method execution or auditing data changes, Spring AOP enhances the quality and maintainability of your application.
Subscribe to my newsletter
Read articles from Sanjeet Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
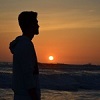
Sanjeet Singh
Sanjeet Singh
I work as a professional in Digital Marketing and specialize in both technical and non-technical writing. My enthusiasm for continuous learning has driven me to explore diverse areas such as lifestyle, education, and technology. That's what led me to discover Uncodemy, a platform offering a wide array of IT courses, including Python, Java, and data analytics. Uncodemy also stands out for providing the java training course in Mohali locations across India, including Faridabad and Jabalpur. It's a great place to enhance one's skills and knowledge in the ever-evolving world of technology.