Node.js Made Easy: A Basic Introduction for New Learnersđź‘¶
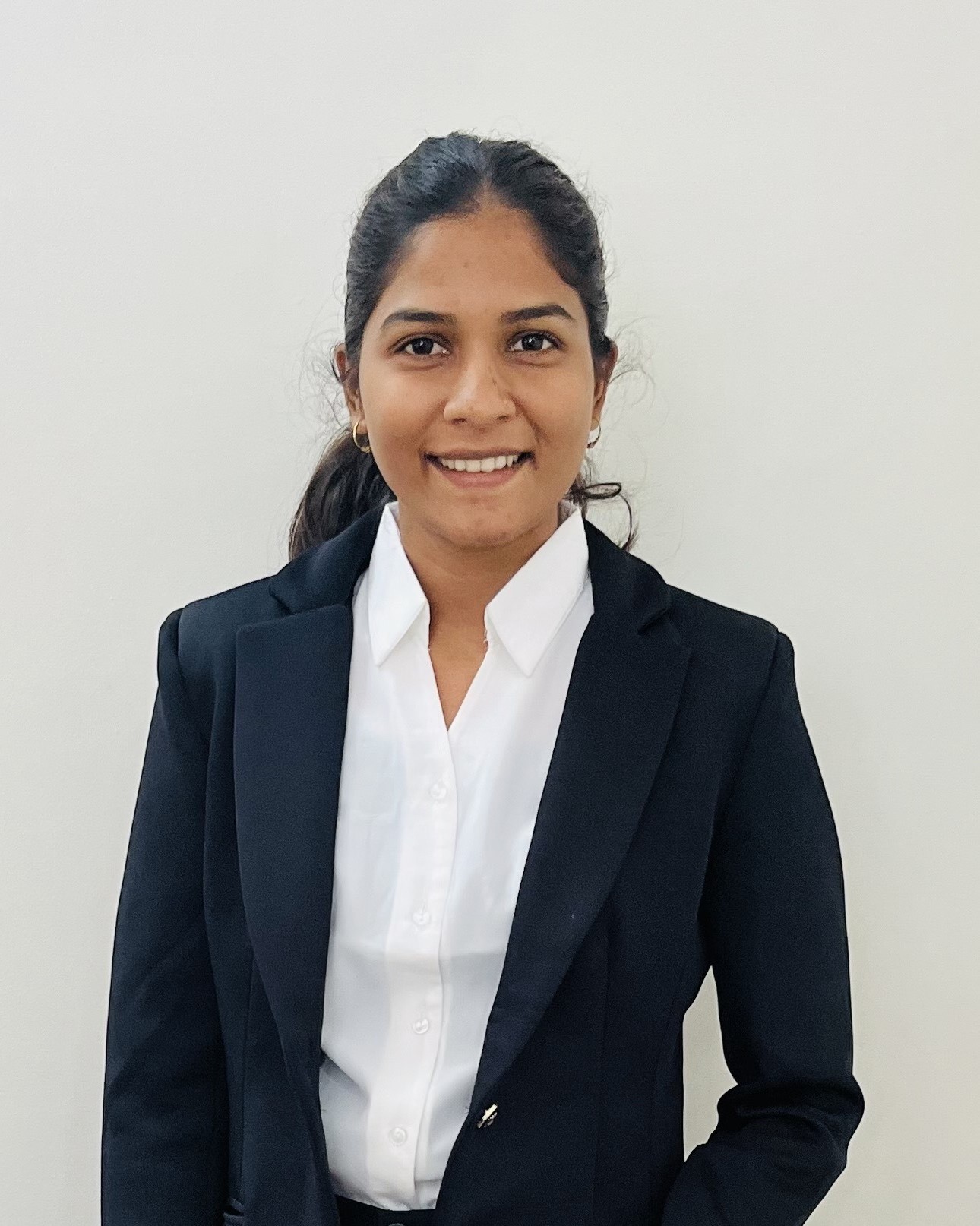
Table of contents
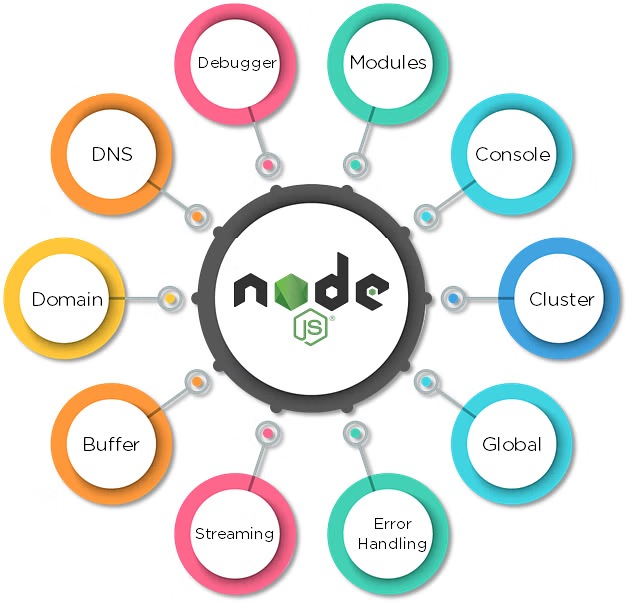
Here's a comprehensive guide to Node.js that covers its key concepts, architecture, modules, common use cases, and more. This will serve as an extensive resource for you to delve deeper into Node.js development.
1. Introduction to Node.js
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment built on Chrome's V8 JavaScript engine.
It allows JavaScript to be used for server-side scripting, enabling developers to produce dynamic web page content before the page is sent to the user's web browser.
Why Use Node.js?
Non-blocking, Event-Driven Architecture: Ideal for I/O-heavy applications like APIs, chat apps, real-time collaboration tools, etc.
Single Programming Language: Full-stack JavaScript, enabling code reuse between client and server.
Rich Ecosystem: npm (Node Package Manager) provides thousands of open-source libraries to accelerate development.
2. Node.js Architecture
Single-Threaded Event Loop:
Node.js operates on a single-threaded event loop model, which handles multiple concurrent requests without creating multiple threads.
It uses non-blocking I/O operations, meaning it doesn’t wait for an operation to complete before moving on to the next one.
Event Loop Mechanism:
Call Stack: Handles function calls.
Event Queue: Stores callback functions that are waiting to be executed.
Event Loop: Continuously checks the call stack and event queue to handle events asynchronously.
3. Core Modules
Node.js provides several built-in modules, which handle various functionalities:
Common Core Modules:
http
: Creates an HTTP server to handle requests and responses.fs
: Interacts with the file system, including reading and writing files.path
: Provides utilities for handling and transforming file paths.url
: Provides utilities for URL resolution and parsing.os
: Provides information about the operating system.crypto
: Provides cryptographic functionalities like hashing, encryption, etc.events
: Provides an EventEmitter class for managing events.
Example of Using Core Modules:
const http = require('http');
const fs = require('fs');
const server = http.createServer((req, res) => {
fs.readFile('index.html', (err, data) => {
if (err) {
res.writeHead(404, { 'Content-Type': 'text/html' });
res.write("File not found");
} else {
res.writeHead(200, { 'Content-Type': 'text/html' });
res.write(data);
}
res.end();
});
});
server.listen(3000, () => {
console.log("Server is listening on port 3000");
});
4. Asynchronous Programming
Callbacks:
- Functions passed as arguments to other functions, to be executed once an operation is complete.
fs.readFile('file.txt', (err, data) => {
if (err) throw err;
console.log(data.toString());
});
Promises:
- An object representing the eventual completion (or failure) of an asynchronous operation, allowing chaining of asynchronous operations.
const readFilePromise = (path) => {
return new Promise((resolve, reject) => {
fs.readFile(path, (err, data) => {
if (err) reject(err);
resolve(data.toString());
});
});
};
readFilePromise('file.txt')
.then(data => console.log(data))
.catch(err => console.error(err));
Async/Await:
- Syntactic sugar for promises, allowing asynchronous code to be written in a synchronous style.
const readFileAsync = async (path) => {
try {
const data = await readFilePromise(path);
console.log(data);
} catch (err) {
console.error(err);
}
};
readFileAsync('file.txt');
5. Express.js – A Popular Web Framework
What is Express.js?
A minimal and flexible Node.js web application framework that provides robust features for web and mobile applications.
Simplifies routing, middleware, and request/response handling.
Setting up an Express Server:
npm install express
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}/`);
});
6. Working with Databases
MongoDB:
- NoSQL database, often used with Node.js for building scalable applications.
Mongoose:
- An ODM (Object Data Modeling) library for MongoDB and Node.js.
npm install mongoose
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true });
const Schema = mongoose.Schema;
const userSchema = new Schema({
name: String,
age: Number,
});
const User = mongoose.model('User', userSchema);
const newUser = new User({ name: 'Alice', age: 25 });
newUser.save().then(() => console.log('User saved.'));
7. Security Best Practices
Environment Variables:
- Use
.env
files to store sensitive information like API keys, database credentials, etc.
npm install dotenv
require('dotenv').config();
const apiKey = process.env.API_KEY;
Input Validation and Sanitization:
Always validate and sanitize user inputs to prevent SQL Injection, XSS, etc.
Libraries like
validator
andjoi
can help with this.
8. Real-Time Applications
- Enables real-time, bi-directional communication between web clients and servers.
npm install socket.io
const http = require('http').createServer();
const io = require('socket.io')(http);
io.on('connection', (socket) => {
console.log('a user connected');
socket.on('chat message', (msg) => {
io.emit('chat message', msg);
});
});
http.listen(3000, () => {
console.log('listening on *:3000');
});
9. Deployment
Popular Hosting Platforms:
Heroku: Easy to set up and scale.
AWS: Flexible and robust, with services like EC2, Elastic Beanstalk.
Vercel: Optimized for frontend frameworks and static sites, but also supports Node.js.
Dockerizing a Node.js App:
- Containerizing your Node.js application using Docker for consistent environments.
# Use the official Node.js image
FROM node:14
# Set the working directory
WORKDIR /app
# Copy the project files
COPY . .
# Install dependencies
RUN npm install
# Expose the application port
EXPOSE 3000
# Start the application
CMD ["node", "server.js"]
10. Testing
Mocha and Chai:
- Mocha is a feature-rich JavaScript test framework, and Chai is an assertion library for Node.js.
npm install mocha chai
const chai = require('chai');
const expect = chai.expect;
describe('Array', () => {
it('should return -1 when the value is not present', () => {
expect([1, 2, 3].indexOf(4)).to.equal(-1);
});
});
11. Common Use Cases
Building REST APIs:
- With Express and libraries like
body-parser
andcors
.
Microservices:
- Node.js is ideal for microservices architecture due to its lightweight nature and efficient I/O.
Real-Time Applications:
- Node.js is commonly used in real-time applications like chat applications, live updates, and collaborative tools.
Command-Line Tools:
- You can create CLI tools using Node.js, utilizing packages like
commander
andinquirer
.
12. Best Practices
Modular Code: Break your application into smaller, reusable modules.
Error Handling: Implement robust error handling mechanisms.
Logging: Use logging libraries like
winston
to track application behavior.Monitoring: Use tools like
pm2
for process management and monitoring.Performance Optimization: Use asynchronous patterns and consider caching strategies.
13. Learning Resources
Documentation: Node.js Documentation
Books:
"Node.js Design Patterns" by Mario Casciaro
"You Don’t Know JS" by Kyle Simpson
Online Courses: Platforms like Udemy, Coursera, and freeCodeCamp offer comprehensive Node.js courses.
best mentors: Hitesh Choudhary ,codewithharry,harkirat singh,sanket singh,anil sidhhu.
This guide should serve as a solid foundation for your journey into Node.js. As you continue to explore, you'll discover even more capabilities and patterns to leverage in building scalable, high-performance applications.
Subscribe to my newsletter
Read articles from priyanka chaudhari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
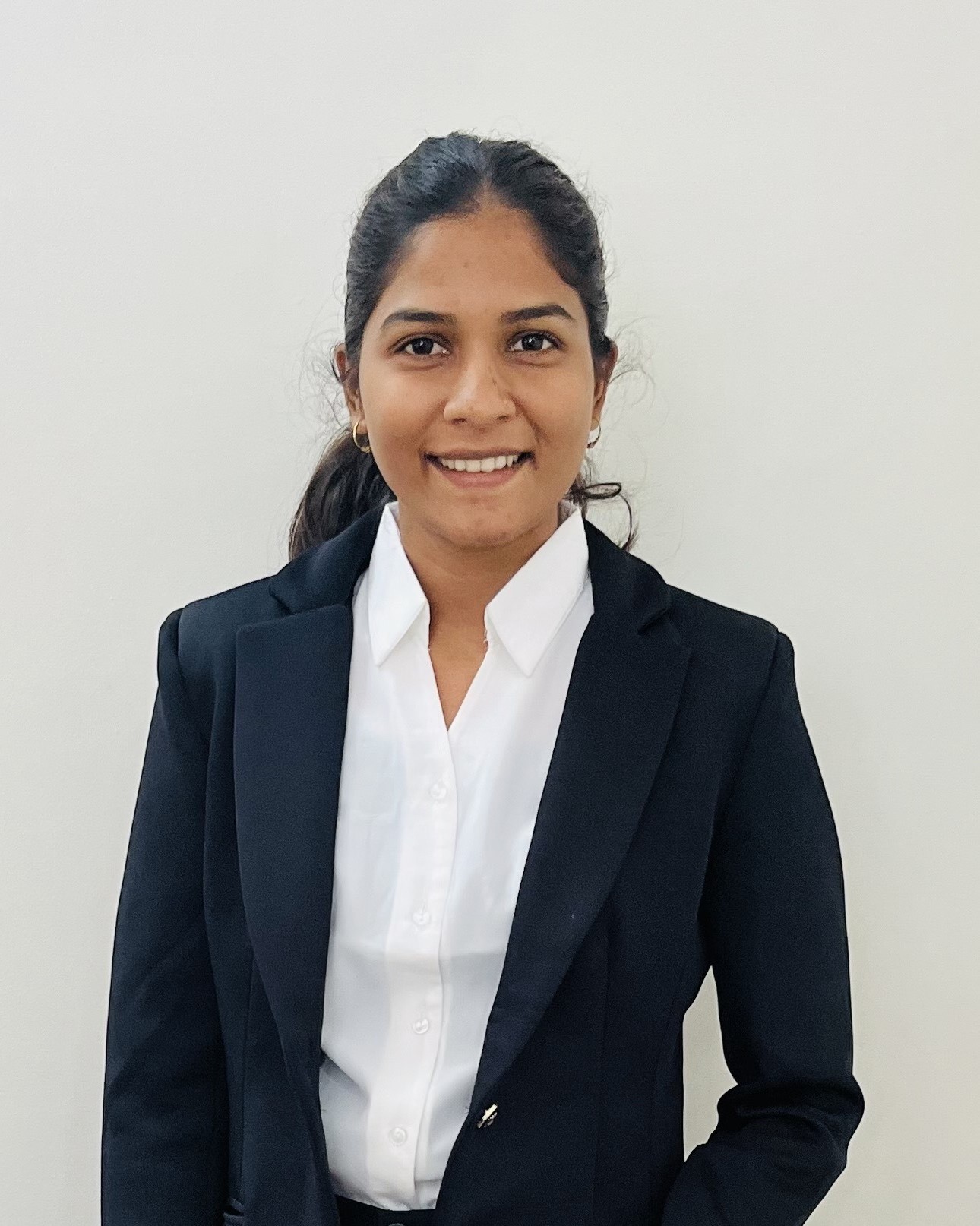
priyanka chaudhari
priyanka chaudhari
i am a full stack web developer and i write code....