Understanding Data Transfer Objects (DTOs) in NestJS: A Comprehensive Guide
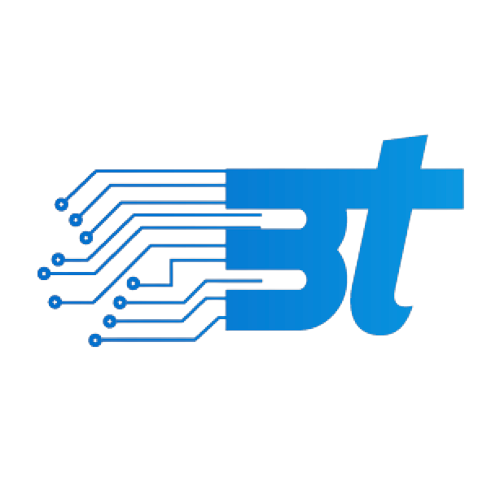
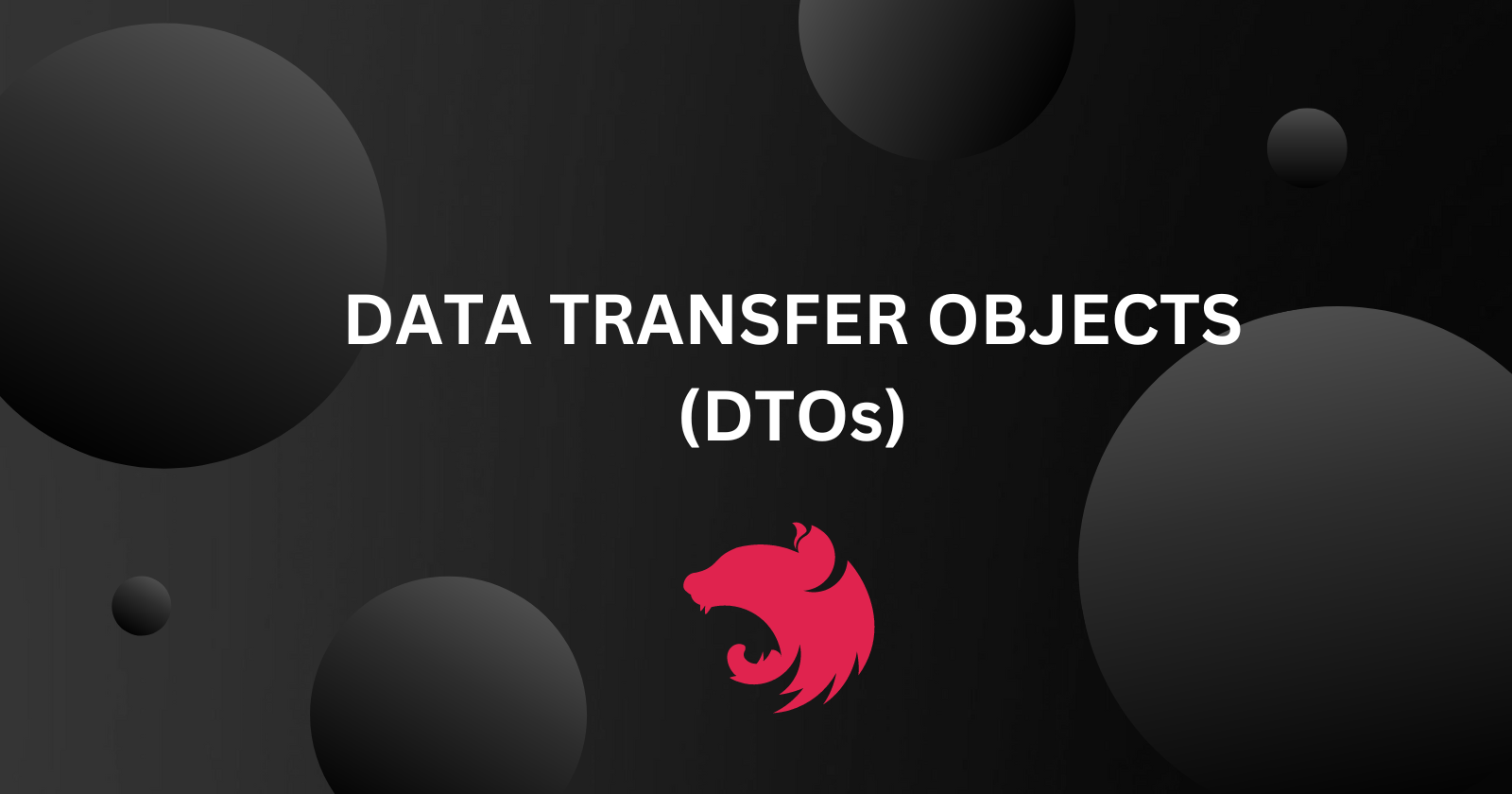
In the ever-evolving world of web development, efficiency and clarity are paramount. As developers, we strive to build applications that are not only functional but also maintainable and scalable. One of the key practices that help achieve these goals is the use of Data Transfer Objects (DTOs). In this blog, we’ll dive deep into DTOs within the context of NestJS, exploring their importance, benefits, and practical implementation with engaging examples.
What is a Data Transfer Object (DTO)?
A Data Transfer Object (DTO) is a design pattern used to transfer data between software application subsystems. Essentially, a DTO is an object that carries data between processes, reducing the number of method calls. This pattern is particularly useful in web development, where it helps streamline communication between the server and the client, ensuring that only the necessary data is sent over the network.
Why Use DTOs in NestJS?
NestJS is a progressive Node.js framework that builds on top of Express, providing an out-of-the-box application architecture. It’s designed to facilitate the creation of highly testable, scalable, loosely coupled, and easily maintainable applications. DTOs play a crucial role in achieving these goals by:
Validation: Ensuring that incoming data is correctly formatted and meets specific criteria before it reaches the business logic.
Type Safety: Leveraging TypeScript’s strong typing to catch errors at compile time, leading to more robust code.
Separation of Concerns: Decoupling the data representation from the business logic, making the codebase cleaner and easier to maintain.
Implementing DTOs in NestJS
Let's walk through a practical example to illustrate how DTOs work in NestJS.
Scenario: You are building a simple CRUD (Create, Read, Update, Delete) application for managing a list of books. Each book has a title, author, and publication year.
Setting Up the Project
First, create a new NestJS project:
nest new books-api cd books-api
Generate a module, controller, and service for books:
nest generate module books nest generate controller books nest generate service books
Creating the DTO
DTOs in NestJS are typically created using TypeScript classes. These classes define the shape of the data and can be decorated with validation rules using the
class-validator
package.Install the required packages:
npm install class-validator class-transformer
Create a
create-book.dto.ts
file in thebooks
directory:import { IsString, IsInt, IsOptional } from 'class-validator'; export class CreateBookDto { @IsString() title: string; @IsString() author: string; @IsInt() publicationYear: number; } export class UpdateBookDto { @IsOptional() @IsString() title?: string; @IsOptional() @IsString() author?: string; @IsOptional() @IsInt() publicationYear?: number; }
Using the DTO in the Controller
Now, use the DTO in the books controller to validate incoming requests.
Update
books.controller.ts
:import { Controller, Post, Body, Get, Param, Put, Delete } from '@nestjs/common'; import { BooksService } from './books.service'; import { CreateBookDto, UpdateBookDto } from './dto/create-book.dto'; @Controller('books') export class BooksController { constructor(private readonly booksService: BooksService) {} @Post() create(@Body() createBookDto: CreateBookDto) { return this.booksService.create(createBookDto); } @Get(':id') findOne(@Param('id') id: string) { return this.booksService.findOne(id); } @Put(':id') update(@Param('id') id: string, @Body() updateBookDto: UpdateBookDto) { return this.booksService.update(id, updateBookDto); } @Delete(':id') remove(@Param('id') id: string) { return this.booksService.remove(id); } }
Handling Data in the Service
In the service, you handle the actual business logic. The DTO ensures that the data received here is already validated and correctly typed.
Update
books.service.ts
:import { Injectable, NotFoundException } from '@nestjs/common'; import { CreateBookDto, UpdateBookDto } from './dto/create-book.dto'; @Injectable() export class BooksService { private books = []; create(createBookDto: CreateBookDto) { const newBook = { id: Date.now().toString(), ...createBookDto }; this.books.push(newBook); return newBook; } findOne(id: string) { const book = this.books.find(book => book.id === id); if (!book) { throw new NotFoundException(`Book with ID ${id} not found`); } return book; } update(id: string, updateBookDto: UpdateBookDto) { const bookIndex = this.books.findIndex(book => book.id === id); if (bookIndex === -1) { throw new NotFoundException(`Book with ID ${id} not found`); } const updatedBook = { ...this.books[bookIndex], ...updateBookDto }; this.books[bookIndex] = updatedBook; return updatedBook; } remove(id: string) { const bookIndex = this.books.findIndex(book => book.id === id); if (bookIndex === -1) { throw new NotFoundException(`Book with ID ${id} not found`); } const removedBook = this.books.splice(bookIndex, 1); return removedBook; } }
Testing the Application
With the DTOs in place, your application is now more robust. You can test the endpoints using a tool like Postman or by writing integration tests.
// Example test for the create endpoint using Jest import { Test, TestingModule } from '@nestjs/testing'; import { BooksController } from './books.controller'; import { BooksService } from './books.service'; import { CreateBookDto } from './dto/create-book.dto'; describe('BooksController', () => { let booksController: BooksController; let booksService: BooksService; beforeEach(async () => { const module: TestingModule = await Test.createTestingModule({ controllers: [BooksController], providers: [BooksService], }).compile(); booksController = module.get<BooksController>(BooksController); booksService = module.get<BooksService>(BooksService); }); describe('create', () => { it('should create a book', async () => { const createBookDto: CreateBookDto = { title: 'Test Book', author: 'Test Author', publicationYear: 2021 }; jest.spyOn(booksService, 'create').mockImplementation(() => createBookDto); expect(await booksController.create(createBookDto)).toBe(createBookDto); }); }); });
Conclusion
With this guide, you should have a solid understanding of how to implement and use DTOs in your NestJS applications. Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
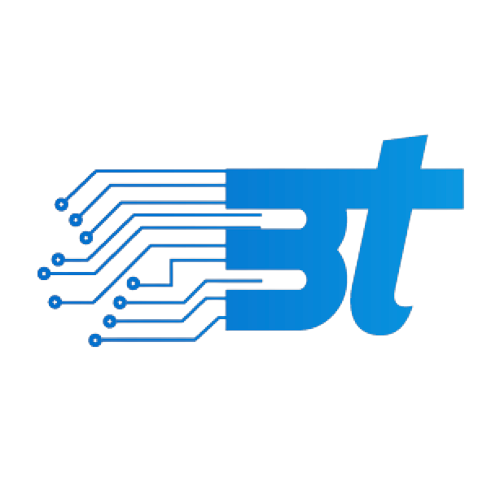
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.