Beware: They Might Be Using Your Camera Without You Noticing via WebAssembly

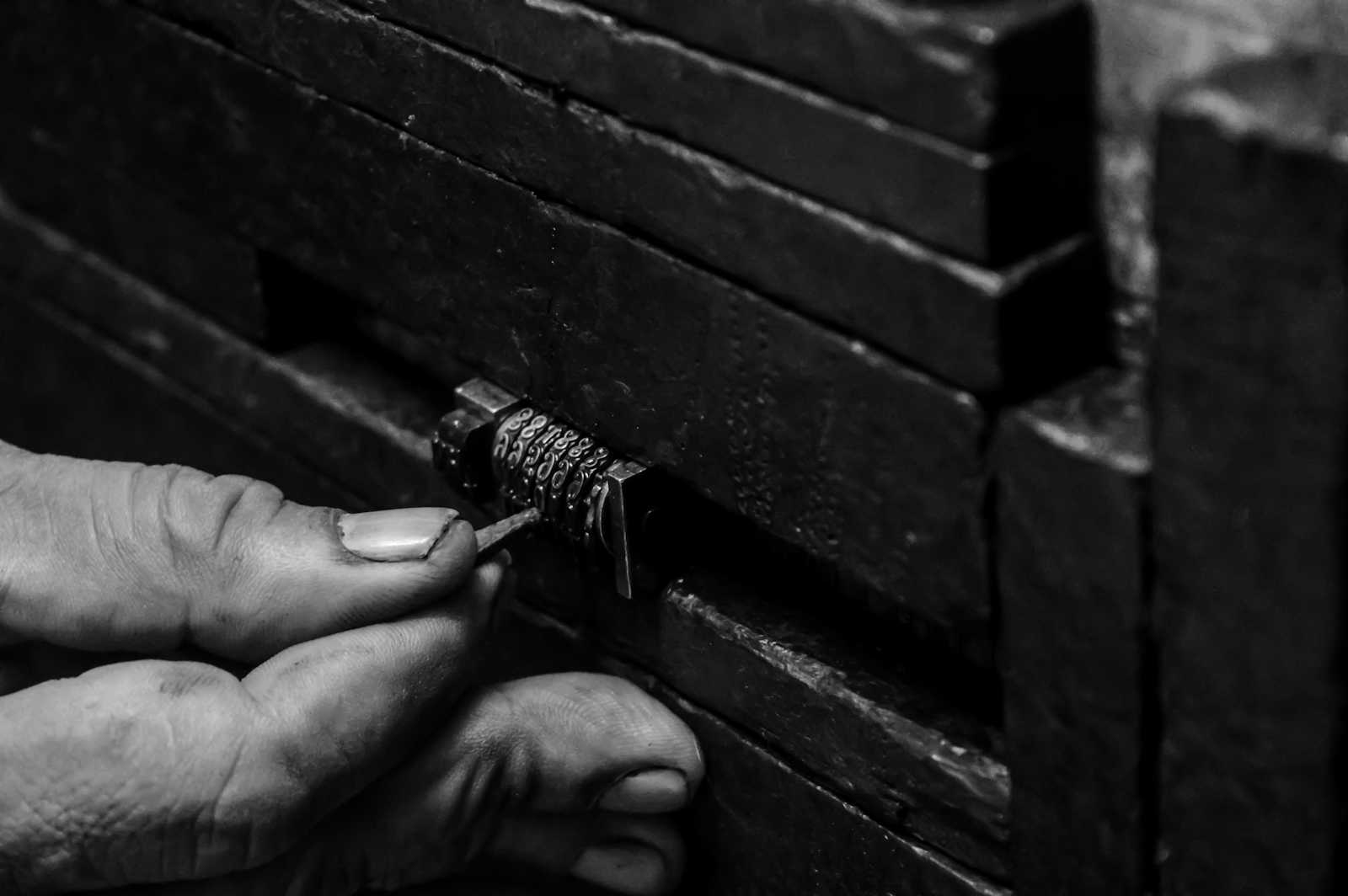
Welcome to the thrilling, techy world where WebAssembly (Wasm) reigns supreme! It's like the superhero of the web, offering speed and efficiency that can make your web apps zoom faster than a caffeinated squirrel. But with great power comes the potential for great mischief—yes, we're talking about your camera. Buckle up, because we're about to dive into how WebAssembly could turn your webcam into a secret spy cam without you even realizing it.
WebAssembly: The Speedster with a Secret
WebAssembly is like a turbo boost for your web apps. Imagine if your browser could bench-press code like a bodybuilder—this is WebAssembly in action. It allows you to run compiled code with near-native speed, meaning complex tasks like video processing and real-time analysis can be performed at breakneck speeds. But don’t be fooled by its superpowers; this also means it can be used for more nefarious purposes if the wrong hands get hold of it.
How WebAssembly Can Sneak a Peek at Your Camera
Bypassing Browser Permissions: Normally, browsers are pretty good about asking you for explicit permission before using your camera. But with WebAssembly, there’s a chance that some sneaky devs could find ways to sidestep these permissions, making your camera a passive observer without your knowledge.
Hidden WebAssembly Modules: WebAssembly code can be like a stealthy ninja in your JavaScript, silently sneaking around without a peep. Malicious WebAssembly code could be loaded and executed in the background, accessing your camera while you’re none the wiser.
High-Speed Data Processing: Once they’ve got access, WebAssembly can process video data at such high speeds that you’d need a magnifying glass to spot it. This could be used for everything from unauthorized surveillance to extracting juicy data you never meant to share.
Example Exploits: How Your Camera Could Be Secretly Hijacked
Let’s pull back the curtain and see some examples of what a malicious WebAssembly module could do. Don’t worry; we’re not giving you a how-to guide for evil, just a peek into how the bad guys might think.
1. Covert Camera Access
Step 1: Crafting the Malicious WebAssembly Module
Here's a simple example of how a nefarious actor might write a WebAssembly module to access your camera:
// malicious_camera.c
#include <stdio.h>
void access_camera() {
// Placeholder for malicious camera access code
printf("Accessing camera... (Shh!)\n");
}
Step 2: Compiling the WebAssembly Module
Compile this code to WebAssembly using Emscripten, the wizard’s wand for turning C/C++ code into web magic:
emcc malicious_camera.c -o malicious_camera.js -s WASM=1
Step 3: Sneaky Execution
Here’s how a bad actor might load and execute this module:
<!DOCTYPE html>
<html>
<head>
<title>Malicious WebAssembly Example</title>
</head>
<body>
<h1>Malicious WebAssembly Example</h1>
<script>
// Sneaky WebAssembly load
WebAssembly.instantiateStreaming(fetch('malicious_camera.wasm'))
.then(obj => {
// Execute the covert operation
obj.instance.exports.access_camera();
// More sneaky stuff could be happening here
});
</script>
</body>
</html>
2. Unauthorized Data Extraction
Step 1: Writing WebAssembly Code for Sneaky Data Processing
Here’s a Rust example that processes video data, potentially extracting sensitive info:
// src/lib.rs
use wasm_bindgen::prelude::*;
#[wasm_bindgen]
pub fn process_video_frame(frame_data: &[u8]) {
// Placeholder for frame processing
console_log!("Processing video frame... (Spying)");
}
Step 2: Compiling to WebAssembly
Build it with wasm-pack
, turning Rust code into web wizardry:
wasm-pack build
Step 3: WebAssembly Meets JavaScript
Here’s how JavaScript might interact with this WebAssembly code:
<!DOCTYPE html>
<html>
<head>
<title>Data Extraction Example</title>
</head>
<body>
<h1>Data Extraction Example</h1>
<script type="module">
import init, { process_video_frame } from './pkg/your_project_name.js';
async function setup() {
await init();
// Assume we have a video feed
const videoElement = document.querySelector('video');
videoElement.addEventListener('play', () => {
setInterval(() => {
// Capture and process video frame
const frameData = captureFrame(videoElement);
process_video_frame(frameData);
}, 1000);
});
}
function captureFrame(video) {
// Example frame data
return new Uint8Array(640 * 480 * 4);
}
setup();
</script>
</body>
</html>
3. Covert File System Access
Step 1: Writing WebAssembly Code for File Access
Here’s a C++ example that might be used to read files:
// file_access.cpp
#include <iostream>
#include <fstream>
extern "C" {
void read_file(const char* filename) {
std::ifstream file(filename);
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
}
}
Step 2: Compiling to WebAssembly
Compile the C++ code:
emcc file_access.cpp -o file_access.js -s WASM=1
Step 3: File Access via JavaScript
Load and use the WebAssembly module in a webpage:
<!DOCTYPE html>
<html>
<head>
<title>File Access Example</title>
</head>
<body>
<h1>File Access Example</h1>
<script>
WebAssembly.instantiateStreaming(fetch('file_access.wasm'))
.then(obj => {
// Execute the function to read a file
obj.instance.exports.read_file('path/to/your/file.txt');
});
</script>
</body>
</html>
How to Protect Yourself
1. Stay Vigilant with Permissions
When a website asks to use your camera, think twice before granting access. Always verify that the request is coming from a trustworthy source. And remember, just because a site asks nicely doesn’t mean it’s trustworthy.
2. Use Browser Extensions
Enhance your browser's security with extensions that keep an eye out for suspicious activities:
Privacy Badger: Automatically slams the door on trackers trying to sneak in.
NoScript: Gives you the power to control which scripts and WebAssembly modules can run, like a bouncer for your browser.
3. Regularly Update Your Browser
Keep your browser as updated as your smartphone. Security patches and updates often come with fixes for vulnerabilities that could be exploited by rogue WebAssembly code.
4. Be Cautious with Unknown Sites
Avoid wandering into the shady corners of the web. Stick to reputable sites and applications, especially those asking for access to sensitive permissions. Remember, if a site feels sketchy, it probably is.
5. Monitor WebAssembly Execution
Your browser’s developer tools can act like a security camera for your code. Keep an eye on WebAssembly modules to spot any unexpected or suspicious activity.
What Developers Should Know
For developers, it’s crucial to wield the power of WebAssembly responsibly. Here are some best practices:
Transparency: Be upfront with users about the use of WebAssembly and any permissions required. Honesty goes a long way.
Sandboxing: Isolate WebAssembly modules like you’d isolate a secret agent. This helps prevent them from accessing sensitive resources without explicit user consent.
Auditing and Testing: Regularly audit and test your WebAssembly code. Think of it as checking your code’s passport for any unauthorized entries.
Wrapping Up
WebAssembly is a technological marvel that can turn your web applications into high-speed powerhouses. But with its powers come potential risks, particularly concerning your privacy. By staying vigilant, using protective tools, and following best practices, you can enjoy the benefits of WebAssembly without sacrificing your privacy.
In the digital age, keeping your camera and data secure is no laughing matter. Stay informed, stay cautious, and remember: even in the world of web security, it’s not paranoia if they’re really out to get your data!
If your code isn't working or you need help debugging, feel free to reach out. Let's tackle it together!
Via Email: scansioquielom@gmail.com
WhatsApp: +2349074395694
LinkedIn: https://www.linkedin.com/in/scansio/
Follow, subscribe, and stay tuned for my next publication!
Subscribe to my newsletter
Read articles from Emmanuel Paul Elom directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emmanuel Paul Elom
Emmanuel Paul Elom
Experienced Full Stack Developer with expertise in JAVA, JavaScript, TypeScript, NodeJS, Rust, ExpressJS, and PHP. Passionate about open-source contributions and creating scalable solutions. Seeking a challenging role to leverage my skills in both front-end and back-end development to drive innovation and efficiency.