A Simple Guide to Writing Modular Python Code

Table of contents
- What is Modular Programming, Anyway?
- The Golden Rules of Modularity
- Structuring a Modular Python Project
- Building Blocks of Modular Code
- Dependency Injection: The Secret Sauce
- AI Chatbot Example: Putting It All Together
- Advanced Techniques for the Modularity Ninjas
- Testing Modular Code: Because "Hope Is Not a Testing Strategy"
- Avoiding the Dark Side: Common Pitfalls
- Ensuring Your Code Survives the Test of Time
- Conclusion: Your Future Self Will Thank You
- References
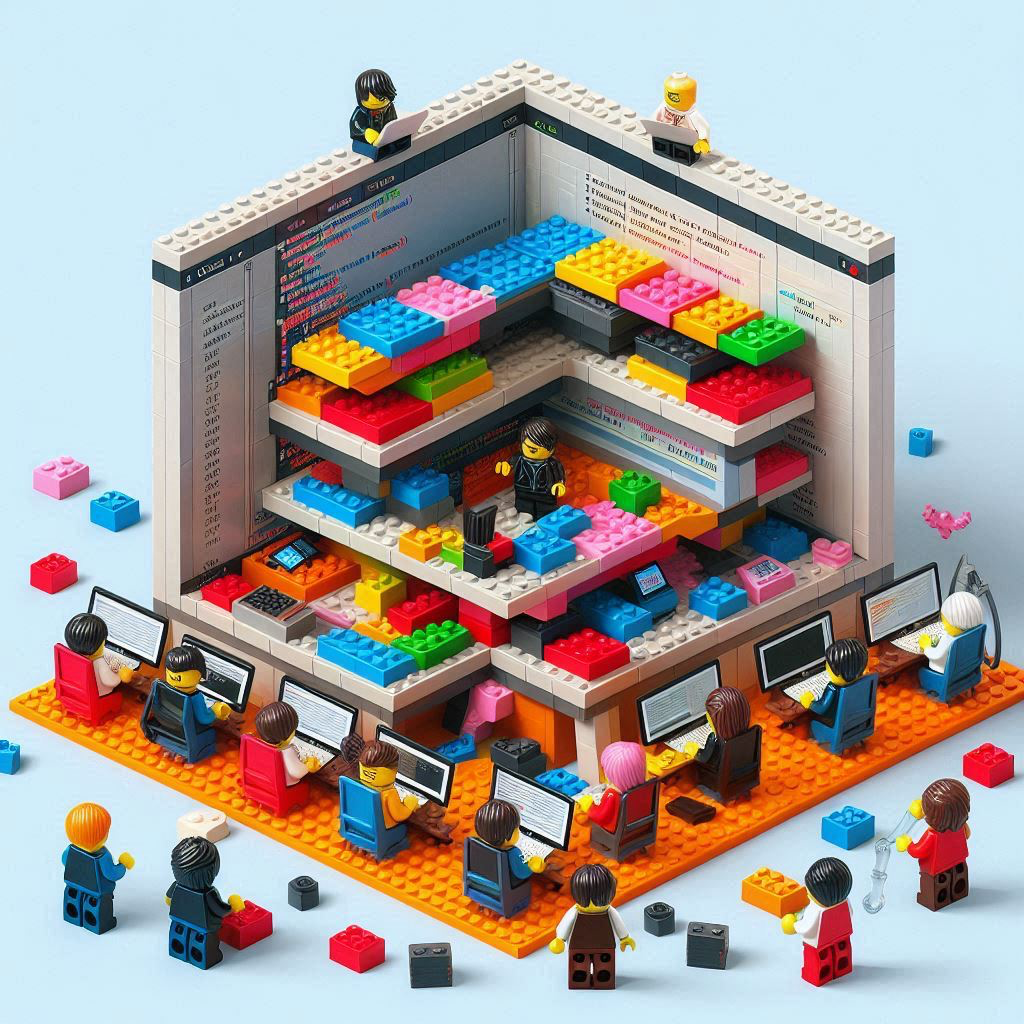
Welcome to the simple guide on writing modular Python code that won't make your future self want to travel back in time to delete your GitHub account! If you've ever inherited a 2,000-line Python script that looks like it was written by a caffeinated octopus during a keyboard shortage, this guide is your salvation.
What is Modular Programming, Anyway?
Modular programming is like organizing your kitchen cupboards. Instead of throwing all your ingredients, utensils, and that mysterious gadget your aunt gave you last Christmas into one giant drawer, you categorize and separate them into logical sections.
In code terms, modularity means breaking down your program into separate, independent modules that each handle a specific functionality. Think of modules as LEGO blocks - each has a specific purpose, can be built and tested independently, and can be combined with others to create something amazing (without stepping on them barefoot at 2 AM).
The benefits? Oh, let me count the ways:
Maintainability: When bugs inevitably appear (like uninvited relatives during holidays), you only need to fix the specific module.
Reusability: Write once, use everywhere! Good modules can be reused across projects.
Readability: Clean, well-organized code is easier to understand for both your future self and other developers.
Testability: Smaller, isolated modules are much easier to test thoroughly.
Collaboration: Multiple developers can work on different modules simultaneously without stepping on each other's toes (or code).
As one wise developer once said, "The best code is the code you don't have to write." By creating reusable modules, you're saving yourself from writing the same functionality over and over again - it's like a programmer version of Groundhog Day, but with more coffee.
The Golden Rules of Modularity
Before we dive into the implementation details, let's establish some golden rules of modularity. Think of these as the "commandments of clean code" that will guide you on your journey from spaghetti code to modular magnificence:
1. Separate Files Are Your Friends
Keep each module in its own file, and make sure it's easy to find the right one. This might seem obvious, but you'd be surprised how many developers try to cram everything into a single file like it's the last lifeboat on the Titanic.
2. Interfaces are Key
Define clear interfaces between modules, so they can communicate effectively without knowing too much about each other's internal workings. It's like how you don't need to know how your coffee machine works internally to make it produce your morning brew.
3. Dependency Injection is a Must
Pass dependencies into a module instead of hardcoding them inside. This makes your modules more flexible and easier to test, like being able to swap out ingredients in a recipe without having to rewrite the entire cookbook.
4. Testing is Essential
Test each module thoroughly before integrating it with others. This way, when something breaks (and something will always break), you'll know exactly where to look.
Structuring a Modular Python Project
The foundation of any well-organized Python project is its directory structure. A good structure is like a good map – it helps you and others navigate the codebase without getting lost in the wilderness of nested directories.
Basic Structure for a Medium-Sized Project
Here's a typical layout for a small-sized Python project:
my_awesome_project/
├── my_awesome_project/ # Main package directory
│ ├── __init__.py # Makes the directory a package
│ ├── main.py # Entry point
│ ├── module1/ # Subpackage for related functionality
│ │ ├── __init__.py
│ │ └── functionality.py
│ ├── module2/ # Another subpackage
│ │ ├── __init__.py
│ │ └── another_functionality.py
│ └── utils/ # Utility functions used across modules
│ ├── __init__.py
│ └── helpers.py
├── tests/ # All your tests
│ ├── __init__.py
│ ├── test_module1.py
│ └── test_module2.py
├── docs/ # Documentation
├── README.md # Project overview
├── requirements.txt # Dependencies
└── setup.py # Installation script
This structure follows the "package of packages" approach, which works well for most projects. Each module or group of related modules gets its own directory, making it easy to locate specific functionality.
Building Blocks of Modular Code
Now that we have our structure in place, let's look at the building blocks of modular Python code:
Functions: The Atomic Units
Functions are the smallest unit of modularity in Python. A well-designed function should:
Do one thing and do it well (like that one friend who makes amazing pancakes but can't boil water)
Have a clear name that describes what it does
Have appropriate docstrings explaining its purpose, parameters, and return values
Handle errors gracefully
Be as pure as possible (minimize side effects)
Let's see an example of a well-designed function for our chatbot:
def parse_user_message(message: str) -> dict:
"""
Parse a user message into a structured format.
Args:
message: The raw message string from the user
Returns:
A dictionary containing the parsed message with keys:
- intent: The detected intent of the message
- entities: A list of entities extracted from the message
Raises:
ValueError: If the message is empty or not a string
"""
if not isinstance(message, str) or not message.strip():
raise ValueError("Message must be a non-empty string")
# Parsing logic here...
parsed_result = {
"intent": "greeting", # This would be determined by actual NLP
"entities": [] # Entities would be extracted from the message
}
return parsed_result
This function has a clear purpose, descriptive name, comprehensive docstring, input validation, and returns a well-defined structure.
Classes: Encapsulating Related Functionality
Classes allow you to group related functions and data together. In a modular system, classes should:
Have a single responsibility
Expose a clear interface
Hide implementation details
Manage their own state
Here's how we might create a class for handling chatbot responses:
class ResponseGenerator:
"""Generates appropriate responses based on parsed user messages."""
def __init__(self, response_templates: dict, default_response: str = "I'm not sure how to respond to that."):
"""
Initialize the response generator.
Args:
response_templates: A dictionary mapping intents to response templates
default_response: The response to use when no matching intent is found
"""
self.templates = response_templates
self.default = default_response
def generate_response(self, parsed_message: dict) -> str:
"""
Generate a response based on the parsed message.
Args:
parsed_message: A dictionary containing the parsed user message
Returns:
A string response to send back to the user
"""
intent = parsed_message.get("intent")
if intent in self.templates:
template = self.templates[intent]
return template
return self.default
This class has a single responsibility (generating responses), a clear interface (generate_response
), and manages its own state (the templates).
Dependency Injection: The Secret Sauce
Dependency injection is like bringing your own ingredients to a cooking class instead of being stuck with whatever they give you. It gives you flexibility, control, and makes testing a breeze.
What is Dependency Injection?
Dependency injection means passing dependencies to a module rather than having the module create or find them itself. It's a fancy term for a simple concept: don't hardcode your dependencies, pass them in!
Let's see how we can apply dependency injection to our chatbot:
# Without dependency injection (not great)
class Chatbot:
def __init__(self):
self.parser = SimpleParser() # Hardcoded dependency
self.response_generator = TemplateResponseGenerator() # Hardcoded dependency
def process_message(self, message):
parsed = self.parser.parse(message)
return self.response_generator.generate_response(parsed)
# With dependency injection (much better!)
class Chatbot:
def __init__(self, parser, response_generator):
self.parser = parser # Injected dependency
self.response_generator = response_generator # Injected dependency
def process_message(self, message):
parsed = self.parser.parse(message)
return self.response_generator.generate_response(parsed)
Now we can easily swap out different implementations of the parser and response generator without changing the Chatbot
class. Want to use a fancy AI model instead of templates? No problem! Just inject a different response generator.
AI Chatbot Example: Putting It All Together
Let's build a modular ChatGPT-style chatbot to see all these principles in action. We'll create a simple but extensible chatbot that can use either templates or an AI model for responses.
First, let's define our interfaces:
from abc import ABC, abstractmethod
from typing import Dict, Any
class MessageParser(ABC):
"""Interface for parsing user messages."""
@abstractmethod
def parse(self, message: str) -> Dict[str, Any]:
"""Parse a user message into a structured format."""
pass
class ResponseGenerator(ABC):
"""Interface for generating responses."""
@abstractmethod
def generate_response(self, parsed_message: Dict[str, Any]) -> str:
"""Generate a response based on a parsed message."""
pass
class AIModel(ABC):
"""Interface for AI model integration."""
@abstractmethod
def generate_text(self, prompt: str) -> str:
"""Generate text based on a prompt."""
pass
Next, let's implement a simple parser:
class SimpleParser(MessageParser):
"""A simple implementation of a message parser."""
def parse(self, message: str) -> Dict[str, Any]:
"""
Parse a user message into intent and entities.
This is a simple implementation that just looks for keywords.
A real implementation might use NLP or machine learning.
"""
message = message.lower()
# Simple intent detection
if any(word in message for word in ["hello", "hi", "hey"]):
intent = "greeting"
elif any(word in message for word in ["bye", "goodbye", "see you"]):
intent = "farewell"
else:
intent = "unknown"
# Simple entity extraction (just a placeholder)
entities = []
return {
"intent": intent,
"entities": entities,
"raw_message": message
}
Now, let's implement our response generators:
class TemplateResponseGenerator(ResponseGenerator):
"""Generates responses based on templates."""
def __init__(self, templates: Dict[str, str], default: str = "I'm not sure how to respond to that."):
"""
Initialize with response templates.
Args:
templates: A dictionary mapping intents to response templates
default: The default response for unknown intents
"""
self.templates = templates
self.default = default
def generate_response(self, parsed_message: Dict[str, Any]) -> str:
"""Generate a response based on the parsed message intent."""
intent = parsed_message.get("intent", "unknown")
return self.templates.get(intent, self.default)
class AIResponseGenerator(ResponseGenerator):
"""Generates responses using an AI model."""
def __init__(self, ai_model: AIModel):
"""
Initialize with an AI model.
Args:
ai_model: An implementation of the AIModel interface
"""
self.ai_model = ai_model
def generate_response(self, parsed_message: Dict[str, Any]) -> str:
"""Generate a response using the AI model."""
raw_message = parsed_message.get("raw_message", "")
prompt = f"User said: '{raw_message}'. Respond in a helpful and friendly way:"
return self.ai_model.generate_text(prompt)
Let's implement an OpenAI model integration:
import openai
class OpenAIModel(AIModel):
"""Integration with OpenAI's GPT API."""
def __init__(self, api_key: str, model: str = "gpt-3.5-turbo"):
"""
Initialize with API key and model.
Args:
api_key: The OpenAI API key
model: The model to use
"""
openai.api_key = api_key
self.model = model
def generate_text(self, prompt: str) -> str:
"""Generate text using the OpenAI API."""
try:
response = openai.ChatCompletion.create(
model=self.model,
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": prompt}
]
)
return response.choices[0].message.content
except Exception as e:
# In a real application, we would handle this more gracefully
return f"Sorry, I couldn't generate a response. Error: {str(e)}"
Finally, let's put it all together:
def main():
"""Run an interactive chat session."""
print("Welcome to the Modular Chatbot!")
print("Type 'exit' to end the conversation.")
# Set up our templates
templates = {
"greeting": "Hello there! How can I help you today?",
"farewell": "Goodbye! Have a great day!",
"unknown": "I'm not sure I understand. Could you rephrase that?"
}
# Choose which chatbot to use
use_ai = input("Use AI for responses? (y/n): ").lower() == 'y'
# Create the appropriate components using dependency injection
parser = SimpleParser()
if use_ai:
api_key = input("Enter your OpenAI API key: ")
ai_model = OpenAIModel(api_key)
generator = AIResponseGenerator(ai_model)
else:
generator = TemplateResponseGenerator(templates)
# Create the chatbot with injected dependencies
chatbot = Chatbot(parser, generator)
# Chat loop
while True:
user_input = input("\nYou: ")
if user_input.lower() == 'exit':
print("Goodbye!")
break
response = chatbot.process_message(user_input)
print(f"Bot: {response}")
if __name__ == "__main__":
main()
This example demonstrates:
Clear interfaces that define the contracts between components
Dependency injection to provide different implementations
Modular organization with related functionality grouped together
Flexibility to swap out components (e.g., using a template-based or AI-based response generator)
Advanced Techniques for the Modularity Ninjas
Ready to level up your modularity game? Let's explore some advanced techniques that will make your code so elegant, other developers might shed a tear of joy:
Magic Methods for Clean Interfaces
Python's magic methods (those with double underscores like __init__
) let you create intuitive interfaces for your classes:
class ChatHistory:
def __init__(self):
self.messages = []
def add_message(self, role, content):
self.messages.append({"role": role, "content": content})
# Make the class behave like a list
def __len__(self):
return len(self.messages)
def __getitem__(self, index):
return self.messages[index]
# String representation for debugging
def __repr__(self):
return f"ChatHistory({len(self)} messages)"
Now you can use ChatHistory
like a regular list: chat_history
, len(chat_history)
, etc., while still having specialized methods.
Context Managers for Resource Management
Context managers (using the with
statement) are excellent for ensuring resources are properly managed:
class ChatSession:
"""Manages a chat session with proper setup and teardown."""
def __init__(self, user_id: str):
self.user_id = user_id
self.session_id = None
def __enter__(self):
"""Set up the chat session."""
self.session_id = f"session_{self.user_id}_{int(time.time())}"
print(f"Starting chat session {self.session_id}")
return self
def __exit__(self, exc_type, exc_val, exc_tb):
"""Clean up the chat session."""
print(f"Ending chat session {self.session_id}")
# Save session history, clean up resources, etc.
Now you can use it with the with
statement:
with ChatSession(user_id="12345") as session:
# Do chat stuff
pass # When this block exits, cleanup happens automatically
Itertools for Elegant Data Handling
The itertools
module provides powerful tools for working with iterators efficiently:
import itertools
# Group chat messages by sender
messages = [
{"sender": "user", "content": "Hello"},
{"sender": "bot", "content": "Hi there!"},
{"sender": "user", "content": "How are you?"},
{"sender": "bot", "content": "I'm good, thanks!"}
]
# Group messages by sender
for sender, group in itertools.groupby(messages, key=lambda x: x["sender"]):
print(f"Messages from {sender}:")
for message in group:
print(f" - {message['content']}")
Collections for Specialized Data Structures
The collections
module offers specialized container datatypes that can make your code more elegant:
from collections import defaultdict, Counter, namedtuple
# Count word frequencies in user messages
word_counts = Counter()
for message in user_messages:
word_counts.update(message.lower().split())
# Track conversation stats by user
stats = defaultdict(lambda: {"message_count": 0, "avg_length": 0})
# Create a structured message type
Message = namedtuple("Message", ["sender", "content", "timestamp"])
msg = Message(sender="user", content="Hello", timestamp=1617293847)
Testing Modular Code: Because "Hope Is Not a Testing Strategy"
One of the major benefits of modular code is that it's much easier to test. Let's look at how we might test some of our chatbot components:
import unittest
from unittest.mock import MagicMock
class TestSimpleParser(unittest.TestCase):
def setUp(self):
self.parser = SimpleParser()
def test_greeting_intent(self):
message = "Hello there!"
result = self.parser.parse(message)
self.assertEqual(result["intent"], "greeting")
def test_farewell_intent(self):
message = "Goodbye for now"
result = self.parser.parse(message)
self.assertEqual(result["intent"], "farewell")
def test_unknown_intent(self):
message = "What's the weather like today?"
result = self.parser.parse(message)
self.assertEqual(result["intent"], "unknown")
class TestAIResponseGenerator(unittest.TestCase):
def setUp(self):
# Create a mock AI model that returns predefined responses
self.ai_model = MagicMock()
self.ai_model.generate_text.return_value = "AI generated response"
self.generator = AIResponseGenerator(self.ai_model)
def test_generate_response(self):
parsed_message = {"raw_message": "Hello AI"}
response = self.generator.generate_response(parsed_message)
self.assertEqual(response, "AI generated response")
# Verify the AI model was called with the right prompt
self.ai_model.generate_text.assert_called_once()
Notice that:
We can test each component in isolation
We use mocks to avoid dependencies on external services
We test specific behaviors rather than implementation details
Avoiding the Dark Side: Common Pitfalls
Even with the best intentions, it's easy to fall into some common pitfalls when working on modular code. Here are some to watch out for:
Circular Dependencies
Problem: Module A imports from module B, which imports from module A. This creates a dependency loop that's harder to maintain than your New Year's resolutions.
Solution:
Restructure your modules to break the cycle
Move shared functionality to a separate module
Use dependency injection instead of direct imports
Too Fine-Grained Modules
Problem: Creating too many tiny modules that don't really stand on their own. This is like slicing bread so thin you can see through it.
Solution:
Find the right balance for your project
Group closely related functionality together
Consider whether a module could reasonably be reused elsewhere
Leaky Abstractions
Problem: Implementation details leak through your interfaces, making them less useful as abstractions. It's like a submarine with screen doors.
Solution:
Design interfaces around use cases, not implementations
Hide implementation details behind clean APIs
Regularly review interfaces from the perspective of a consumer
Ensuring Your Code Survives the Test of Time
Creating modular code is one thing, but ensuring it stays maintainable over time is another challenge. Here are some strategies:
Comprehensive Documentation
Documentation is crucial for long-term maintainability. Make sure to document:
The purpose of each module
The interfaces between modules
Any non-obvious design decisions
How to extend the system for common use cases
Example of good module documentation:
"""
Message Parsing Module
=====================
This module handles the parsing of user messages into structured data
that can be processed by the chatbot.
Classes:
SimpleParser: A basic parser that uses keyword matching
NLPParser: An advanced parser that uses natural language processing
Usage:
parser = SimpleParser()
parsed_message = parser.parse("Hello, chatbot!")
# Returns: {"intent": "greeting", "entities": [], "raw_message": "hello, chatbot!"}
Extension:
To add support for new intents, update the keyword lists in the
respective parser implementation.
"""
Regular Refactoring
Code tends to degrade over time if not actively maintained, like that salad you forgot in the back of your fridge. Schedule regular refactoring sessions to:
Remove duplicated code
Simplify complex functions
Ensure module boundaries remain clear
Update documentation to reflect the current state
As Martin Fowler wisely said, "If it hurts, do it more often." The more you refactor, the less painful each refactoring session becomes.
Conclusion: Your Future Self Will Thank You
Writing modular Python code is not just about following a set of mechanical rules—it's about embracing a mindset that values clarity, reusability, and maintainability. By breaking down complex problems into manageable, well-defined modules, you not only make your code easier to understand and test but also set the stage for collaboration and future extensions.
As we've seen with our chatbot example, modularity allows you to swap out components, test in isolation, and adapt to changing requirements with minimal disruption. Whether you're building AI-powered applications or any other type of software, these principles will help you create code that stands the test of time.
Remember, the future maintainer of your code might be you six months from now, with no recollection of why you made certain decisions. Be kind to that future you (and your colleagues) by writing modular, well-documented, and clean code today. Your future self will thank you when they don't have to untangle a Gordian knot of spaghetti code at 3 AM during a production emergency!
Now, go forth and modularize! And may your dependencies always be injected, your interfaces always be clear, and your code reviews always be painless.
References
[1] Dagster. (2024, February 27). Best Practices in Structuring Python Projects. Retrieved from https://dagster.io/blog/python-project-best-practices
[2] Gyansetu. (2024, June 15). Advanced Python Methods and Techniques. Retrieved from https://www.gyansetu.in/blog/advanced-python-methods-and-techniques/
[3] Real Python. (2023, February 24). ChatterBot: Build a Chatbot With Python. Retrieved from https://realpython.com/build-a-chatbot-python-chatterbot/
[4] Pawamoy. (2022, September 22). Somewhat-modern Python. Retrieved from https://pawamoy.github.io/posts/somewhat-modern-python-development/
[5] Inedo. (2025, March 31). How to Escape Python Script Hell with Modules & Packages. Retrieved from https://blog.inedo.com/python/modularization-and-packages/
[6] Python Course EU. (2023, November 8). Modular Programming and Modules. Retrieved from https://python-course.eu/python-tutorial/modules-and-modular-programming.php
[7] Tech With Tim. (2023, October 9). Create a Python GPT Chatbot - In Under 4 Minutes [Video]. YouTube. Retrieved from https://www.youtube.com/watch?v=q5HiD5PNuck
Subscribe to my newsletter
Read articles from Derek Armstrong directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Derek Armstrong
Derek Armstrong
I share my thoughts on software development and systems engineering, along with practical soft skills and friendly advice. My goal is to inspire others, spark ideas, and discover new passions.