Optimizing Performance in React and Next.js Applications ๐
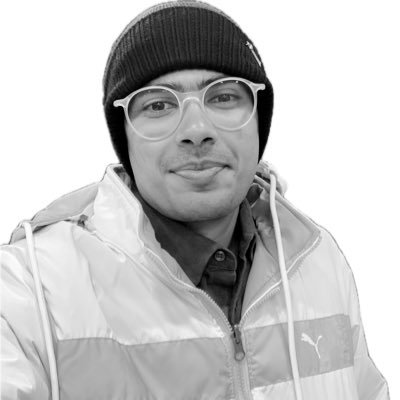
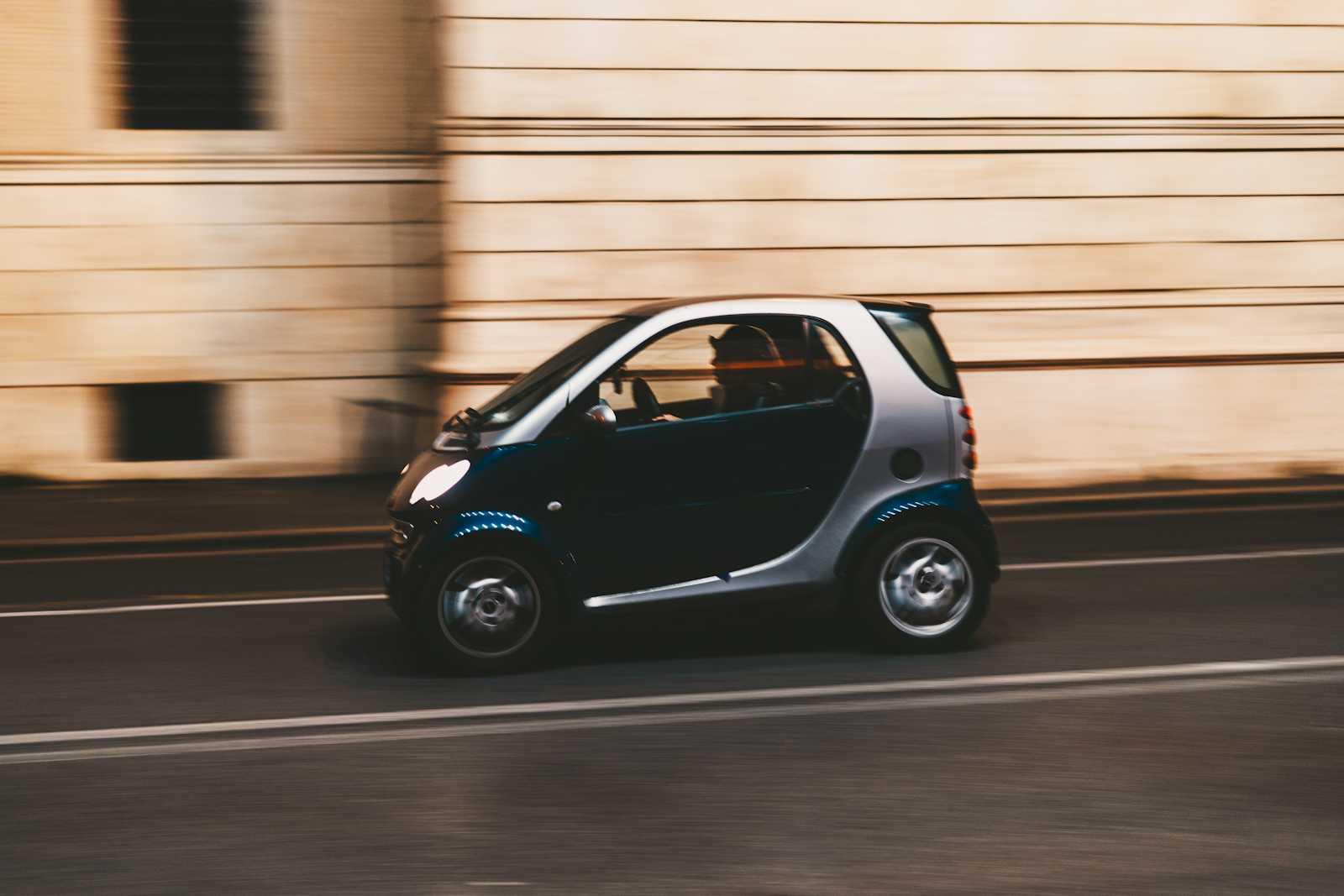
In the competitive landscape of web development, performance is crucial. A fast, responsive application not only enhances user experience but also boosts SEO and conversion rates. React and Next.js are powerful tools for building modern web applications, but optimizing their performance requires thoughtful implementation of best practices. In this blog post, we'll explore tips and techniques for improving the performance of React and Next.js apps, including code splitting, lazy loading, and efficient state management.
Code Splitting for Better Load Times ๐๏ธ
Code splitting is a technique that allows you to break down your application into smaller chunks, which can be loaded on demand. This reduces the initial load time and improves the overall performance of your app.
Implementing Code Splitting in React ๐ก
React provides a built-in way to split code using React.lazy
and Suspense
.
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
Code Splitting in Next.js ๐
Next.js automatically splits code by page, which means that each page only loads the necessary code for that particular view. This reduces the initial load time and improves navigation between pages.
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('../components/DynamicComponent'), {
loading: () => <p>Loading...</p>,
ssr: false,
});
function Home() {
return (
<div>
<DynamicComponent />
</div>
);
}
export default Home;
Lazy Loading for Efficient Resource Management โณ
Lazy loading is a technique that delays the loading of non-critical resources until they are needed. This can significantly improve the initial load time of your application.
Implementing Lazy Loading in React ๐ ๏ธ
React's React.lazy
can also be used for lazy loading components.
const LazyImage = React.lazy(() => import('./LazyImage'));
function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyImage />
</Suspense>
</div>
);
}
export default App;
Lazy Loading Images in Next.js ๐ผ๏ธ
Next.js provides an Image
component that supports lazy loading out of the box.
import Image from 'next/image';
function Home() {
return (
<div>
<Image
src="/path/to/image.jpg"
alt="Picture of something"
width={500}
height={300}
loading="lazy"
/>
</div>
);
}
export default Home;
Efficient State Management ๐ง
Efficient state management is essential for maintaining performance, especially in large applications. Using the right tools and strategies can prevent unnecessary re-renders and improve the overall responsiveness of your app.
Using React Context for State Management ๐
React Context is a powerful tool for managing state globally without prop drilling.
import React, { createContext, useContext, useState } from 'react';
const MyContext = createContext();
function MyProvider({ children }) {
const [state, setState] = useState('default value');
return (
<MyContext.Provider value={{ state, setState }}>
{children}
</MyContext.Provider>
);
}
function MyComponent() {
const { state, setState } = useContext(MyContext);
return (
<div>
<p>{state}</p>
<button onClick={() => setState('new value')}>Change State</button>
</div>
);
}
function App() {
return (
<MyProvider>
<MyComponent />
</MyProvider>
);
}
export default App;
Optimizing with Redux ๐๏ธ
For more complex state management, Redux is a popular choice. Use the redux-thunk
middleware for handling asynchronous actions.
import { createStore, applyMiddleware } from 'redux';
import { Provider } from 'react-redux';
import thunk from 'redux-thunk';
import rootReducer from './reducers';
const store = createStore(rootReducer, applyMiddleware(thunk));
function App() {
return (
<Provider store={store}>
{/* Your components */}
</Provider>
);
}
export default App;
Additional Performance Optimization Techniques ๐ก
Minimize Re-renders ๐
Use
React.memo
to memoize functional components and prevent unnecessary re-renders.Utilize the
useMemo
anduseCallback
hooks to memoize expensive calculations and functions.
Optimize Bundle Size ๐ฆ
Remove unused dependencies and optimize imports.
Use tools like Webpack Bundle Analyzer to identify large modules and optimize them.
Server-Side Rendering (SSR) ๐ฅ๏ธ
- Next.js supports SSR, which can improve performance by rendering pages on the server and sending fully rendered HTML to the client.
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return { props: { data } };
}
function Home({ data }) {
return (
<div>
<h1>{data.title}</h1>
<p>{data.description}</p>
</div>
);
}
export default Home;
Optimizing the performance of React and Next.js applications involves a combination of techniques, including code splitting, lazy loading, and efficient state management. By implementing these best practices, you can create fast, responsive, and user-friendly web applications.
Happy coding! ๐๐
For more detailed information, check out the official documentation for React and Next.js.
Feel free to share your thoughts and additional tips in the comments below! ๐ฌ
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
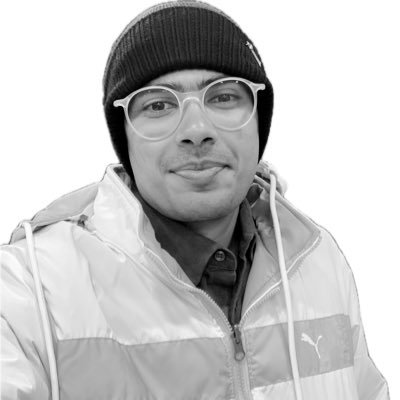
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on โจ๏ธ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix ๐จ๐ปโ๐ป