FORMS in HTML, REACT and NEXT
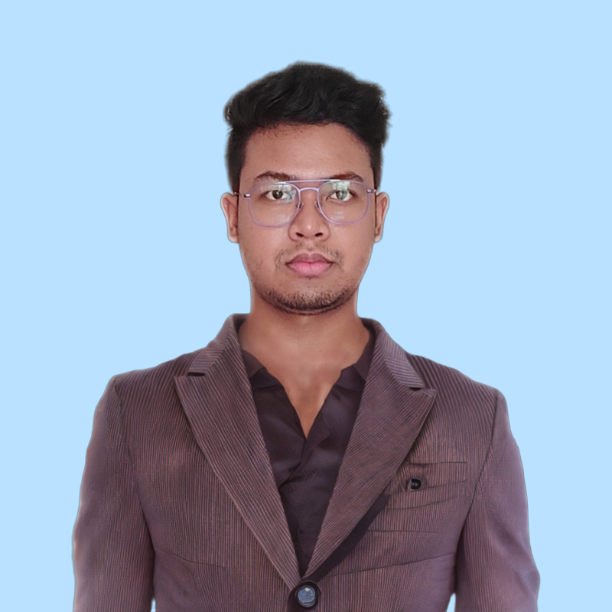
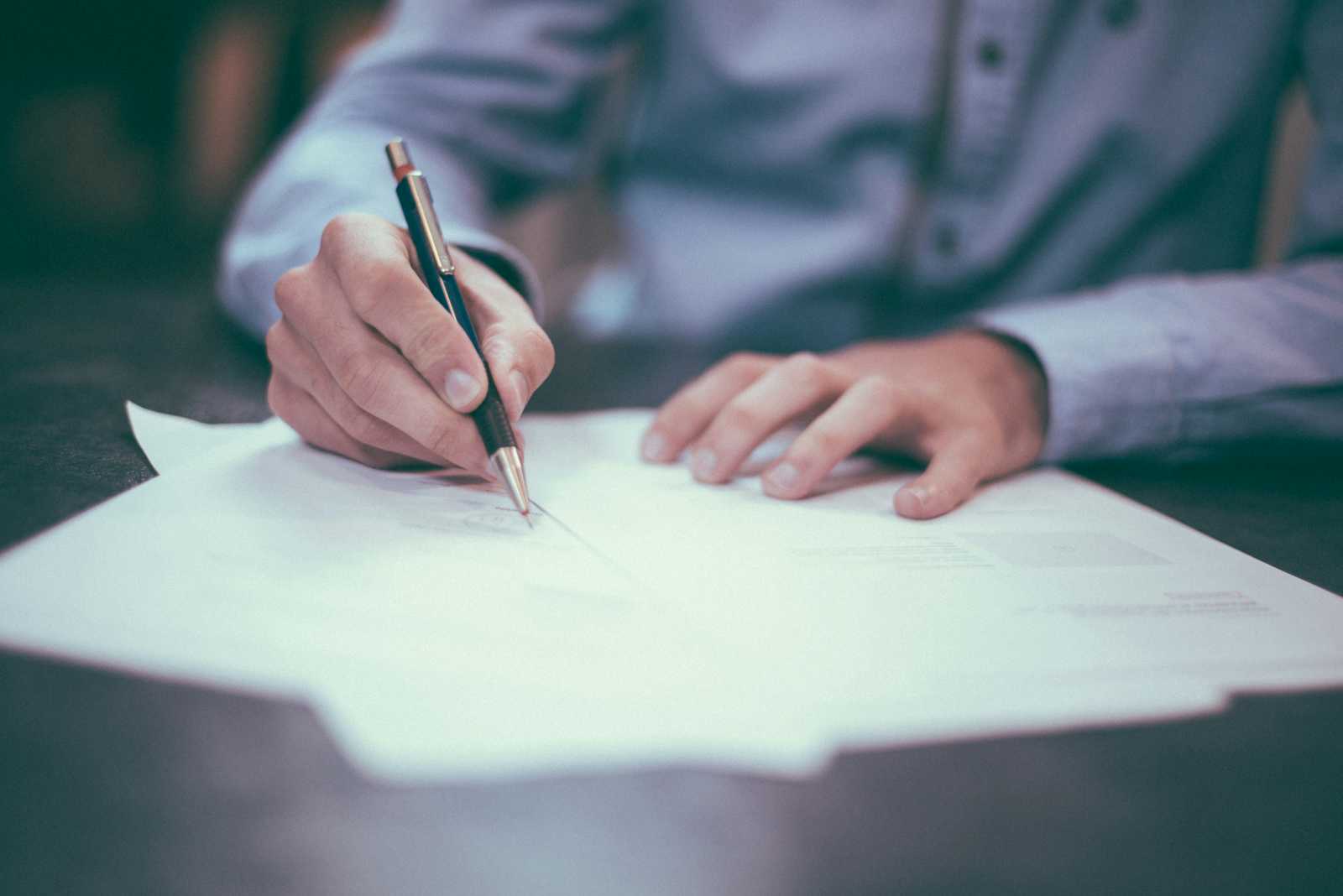
Writing forms in plain HTML, React, and Next.js involves different approaches and levels of complexity. Below, we'll compare these three methods, discuss best practices, and touch on industry preferences.
Comparison of Writing Forms
Plain HTML
Pros:
Simplicity: Easy to understand and quick to implement.
No dependencies: Doesn't require additional libraries or frameworks.
Direct integration: Forms are directly written in the HTML file.
Cons:
Limited interactivity: Adding complex interactivity requires JavaScript.
State management: Handling form state and validation can become cumbersome.
Scalability: Difficult to manage in large applications.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Plain HTML Form</title>
</head>
<body>
<form action="/submit" method="POST">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br><br>
<button type="submit">Submit</button>
</form>
</body>
</html>
React
Pros:
Component-based: Forms can be broken down into reusable components.
State management: Easier state handling with hooks (e.g.,
useState
,useContext
).Interactivity: Easy to add complex interactivity and dynamic form behaviors.
Validation: Can use libraries like Formik or react-hook-form for validation.
Cons:
Complexity: Requires understanding of React concepts.
Dependencies: Additional libraries may be needed for complete functionality.
Example:
import React, { useState } from 'react';
function MyForm() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
console.log({ name, email });
};
return (
<form onSubmit={handleSubmit}>
<label htmlFor="name">Name:</label>
<input
type="text"
id="name"
value={name}
onChange={(e) => setName(e.target.value)}
required
/><br /><br />
<label htmlFor="email">Email:</label>
<input
type="email"
id="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
required
/><br /><br />
<button type="submit">Submit</button>
</form>
);
}
export default MyForm;
Next.js
Pros:
Server-side rendering (SSR): Can pre-render forms for better performance and SEO.
API routes: Integrated backend routes for form submission handling.
React-based: Inherits all benefits of React.
Static site generation (SSG): Can statically generate forms.
Cons:
Learning curve: Requires understanding of Next.js features and configuration.
Overhead: Might be overkill for simple forms.
Example:
// pages/index.js
import { useState } from 'react';
export default function Home() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleSubmit = async (event) => {
event.preventDefault();
const res = await fetch('/api/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ name, email }),
});
const data = await res.json();
console.log(data);
};
return (
<form onSubmit={handleSubmit}>
<label htmlFor="name">Name:</label>
<input
type="text"
id="name"
value={name}
onChange={(e) => setName(e.target.value)}
required
/><br /><br />
<label htmlFor="email">Email:</label>
<input
type="email"
id="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
required
/><br /><br />
<button type="submit">Submit</button>
</form>
);
}
// pages/api/submit.js
export default function handler(req, res) {
const { name, email } = req.body;
res.status(200).json({ message: `Received name: ${name}, email: ${email}` });
}
Best Practices
General Best Practices
Accessibility: Ensure forms are accessible with proper labels,
aria
attributes, and keyboard navigation.Validation: Implement both client-side and server-side validation.
Security: Protect against common vulnerabilities like CSRF, XSS, and injection attacks.
Responsive Design: Ensure forms are responsive and usable on different devices.
React/Next.js Specific
State Management: Use appropriate hooks or state management libraries for form state.
Reusable Components: Create reusable form components to avoid code duplication.
Form Libraries: Utilize libraries like Formik, react-hook-form, or Yup for form handling and validation.
Performance: Optimize form rendering and avoid unnecessary re-renders.
Industry Preference
Plain HTML: Suitable for simple static websites or when working within environments where JavaScript frameworks are not an option.
React: Preferred for modern web applications due to its component-based architecture, ease of state management, and large ecosystem of libraries and tools.
Next.js: Gaining popularity for its features like SSR, SSG, and API routes. Preferred for projects requiring improved performance, SEO, and scalability.
Summary
Plain HTML: Best for simplicity and quick prototyping.
React: Ideal for interactive, dynamic forms in complex web applications.
Next.js: Suitable for SEO-friendly, high-performance web applications with server-side capabilities.
By following best practices and choosing the right tool for the job, you can create robust, user-friendly forms that meet the needs of your project and industry standards.
Subscribe to my newsletter
Read articles from Nirban Roy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
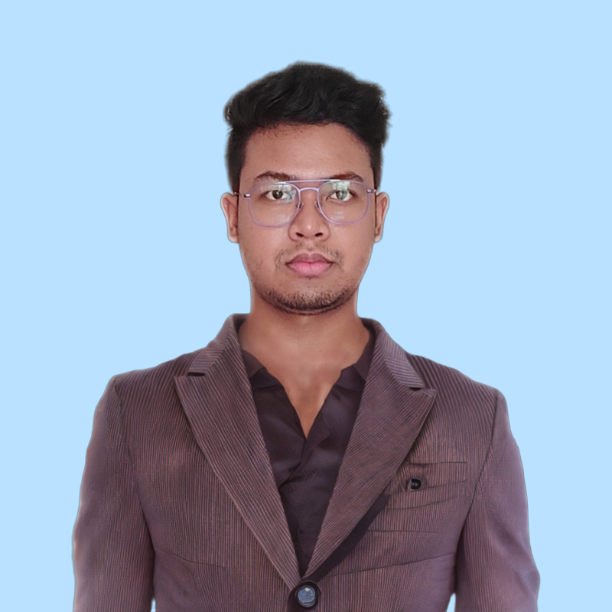
Nirban Roy
Nirban Roy
As a software engineer, ๐งโ๐ป I write guides ๐ on the technologies ๐ I have used to make them simpler for the new developers. ๐