Callback Function and Callback Return

Table of contents
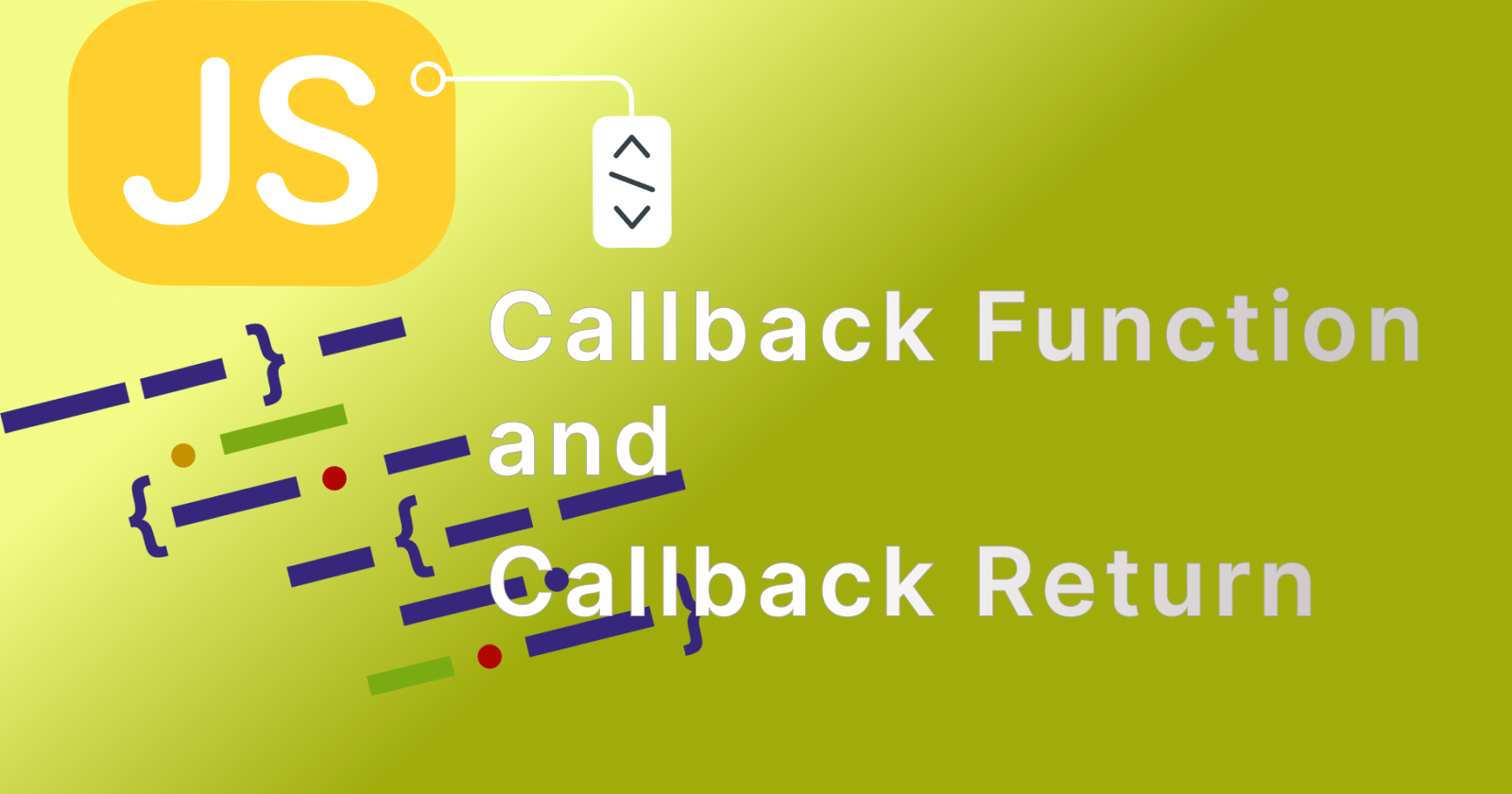
Callback Function
A function passed as an argument to another function, which is then executed inside that function.
Callbacks are commonly used in asynchronous programming, where operations (like I/O tasks) are completed without blocking the main thread.
Example:
function fetchData(callback) {
// Simulate an asynchronous operation using setTimeout
setTimeout(() => {
// Simulated data retrieval
const data = "Here is your data!";
// Invoke the callback with the data
callback(data);
}, 2000);
}
function processData(data) {
console.log("Processing data:", data);
}
// Call fetchData with processData as the callback
fetchData(processData);
In this example:
fetchData
is a function that accepts a callback function as an argument.processData
is the callback function that is called once the asynchronous operation (simulated bysetTimeout
) is complete.Output :-
Processing data: Here is your data!
Callback Return
When a callback function is invoked, it can also return a value. However, in the context of asynchronous operations, the return value of a callback function is typically not used because the main function's execution flow has already moved on.
Example:
function add(a, b, callback) {
const result = a + b;
return callback(result);
}
function displayResult(result) {
console.log("The result is:", result);
return result; // Return value of the callback
}
const sum = add(5, 3, displayResult);
console.log("Returned value:", sum); // This logs the returned value from displayResult
In this example:
add
is a function that takes two numbers and a callback function as arguments.displayResult
is the callback function, which logs the result and returns it.Output:-
The result is: 8
Returned value: 8
The key points about callbacks and their return values:
Execution Context: The return value of a callback is only relevant within the context of the callback itself. The calling function (
add
in the example) can use this return value if needed.Asynchronous Context: In asynchronous functions, the main function often does not wait for the callback to complete, so returning a value from the callback may not affect the main function's execution. This is why callbacks in asynchronous operations typically don't return values intended to affect the main function's flow.
Summary
Callback Function: A function passed as an argument to another function, executed later.
Callback Return: The value returned by a callback function, usually not used in asynchronous contexts.
Callbacks are fundamental in JavaScript, especially in handling asynchronous operations such as API calls, file reading, and more.
We are committed to delivering content that informs, inspires, and resonates with you. Your comments, and shared insights fuel our passion to continue creating valuable content.
As we move forward, we invite you to stay connected with us. Feel free to share your thoughts in the comments.
Once again, thank you for being a part of our community. We look forward to continuing this journey together.
Subscribe to my newsletter
Read articles from Harshal Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harshal Yadav
Harshal Yadav
I am Harshal, a passionate and creative developer from ๐ฎ๐ณ with a strong interest in MERN and DevOps. Full stack developer with experience in developing and managing web applications. Reactjs, Nextjs, Nodejs, Expressjs, CSS, HTML, JavaScript, MongoDB, Docker, and AWS .