Building Resilient Infrastructure: Deploying EC2 Instances Across Multiple Availability Zones using Terraform
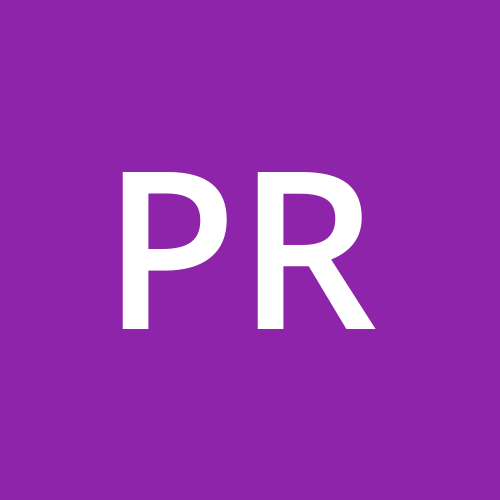
Table of contents
- Introduction
- Prerequisites
- Before we begin, make sure you have the following:
- Step 1: Set Up Your Terraform Project
- Step 2: Define the Provider and Variables
- Step 3: Create a Security Group
- Step 4: Launch EC2 Instances
- Step 5: Create a Load Balancer
- Set up an Elastic Load Balancer (ELB) to distribute traffic among your EC2 instances.
- Step 6: Apply the Configuration
- Conclusion
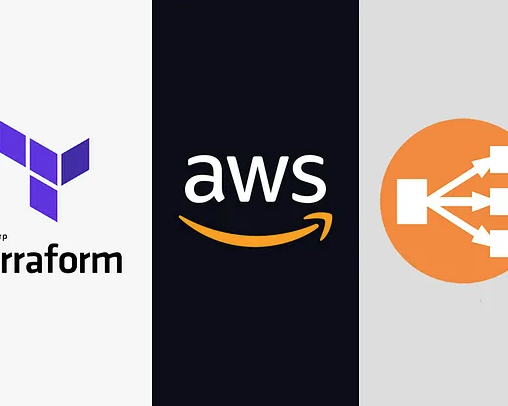
Introduction
Deploying EC2 Instances in Multiple Availability Zones with a Load Balancer Using Terraform
Deploying EC2 instances across multiple Availability Zones (AZs) is a key practice for ensuring high availability and fault tolerance of your applications on AWS. Using Terraform, an infrastructure-as-code (IaC) tool, you can automate this process and manage your infrastructure efficiently. In this blog, we will walk you through the steps to deploy EC2 instances in multiple AZs and set up a load balancer to distribute traffic among them.
Prerequisites
Before we begin, make sure you have the following:
1.AWS Account: An active AWS account.
2.Terraform: Installed on your local machine. You can download it from here.
3.AWS CLI: Installed and configured with your AWS credentials. You can download it from here.
Step 1: Set Up Your Terraform Project
First, create a directory for your Terraform project. Inside this directory, create a new file called main.tf
.
mkdir terraform-ec2-multi-az
cd terraform-ec2-multi-az
touch main.tf
Step 2: Define the Provider and Variables
In your main.tf
, start by defining the AWS provider and necessary variables.
provider "aws" {
region = "us-west-2" # Replace with your preferred region
}
variable "instance_count" {
description = "Number of EC2 instances"
default = 2
}
variable "instance_type" {
description = "Type of EC2 instance"
default = "t2.micro"
}
variable "vpc_id" {
description = "VPC ID where instances will be deployed"
}
variable "subnet_ids" {
description = "List of Subnet IDs in different AZs"
type = list(string)
}
variable "ami_id" {
description = "AMI ID for the EC2 instances"
}
Step 3: Create a Security Group
Create a security group to allow necessary traffic to your EC2 instances.
resource "aws_security_group" "web_sg" {
name = "web_sg"
description = "Allow web traffic"
vpc_id = var.vpc_id
ingress {
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
Step 4: Launch EC2 Instances
Use a for_each
loop to deploy EC2 instances across multiple AZs.
resource "aws_instance" "web" {
count = var.instance_count
ami = var.ami_id
instance_type = var.instance_type
subnet_id = element(var.subnet_ids, count.index)
security_groups = [aws_security_group.web_sg.name]
tags = {
Name = "web-instance-${count.index + 1}"
}
}
Step 5: Create a Load Balancer
Set up an Elastic Load Balancer (ELB) to distribute traffic among your EC2 instances.
resource "aws_elb" "web_elb" {
name = "web-load-balancer"
availability_zones = var.subnet_ids
listener {
instance_port = 80
instance_protocol = "HTTP"
lb_port = 80
lb_protocol = "HTTP"
}
health_check {
target = "HTTP:80/"
interval = 30
timeout = 5
healthy_threshold = 2
unhealthy_threshold = 2
}
instances = [for instance in aws_instance.web : instance.id]
tags = {
Name = "web-elb"
}
}
Step 6: Apply the Configuration
Initialize Terraform, review the execution plan, and apply the configuration to create your resources.
terraform init
terraform plan
terraform apply
Conclusion
By following these steps, you have successfully deployed EC2 instances in multiple Availability Zones and set up a load balancer using Terraform. This setup ensures high availability and fault tolerance for your application, providing a resilient architecture on AWS.
Terraform makes infrastructure management more predictable and less error-prone by allowing you to define your infrastructure as code. Keep exploring Terraform’s capabilities to manage your AWS infrastructure more efficiently.
Feel free to expand this setup with additional features like auto-scaling, advanced monitoring, and integrating with other AWS services.
-Hope you like this blog! Happy Learning!
Subscribe to my newsletter
Read articles from Prasanna directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
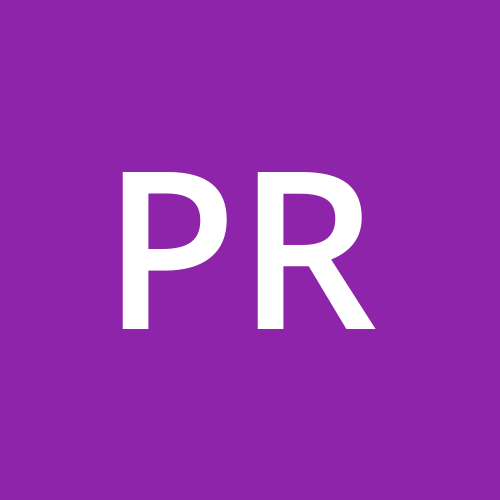