Building High-Performance Mobile Apps with React Native
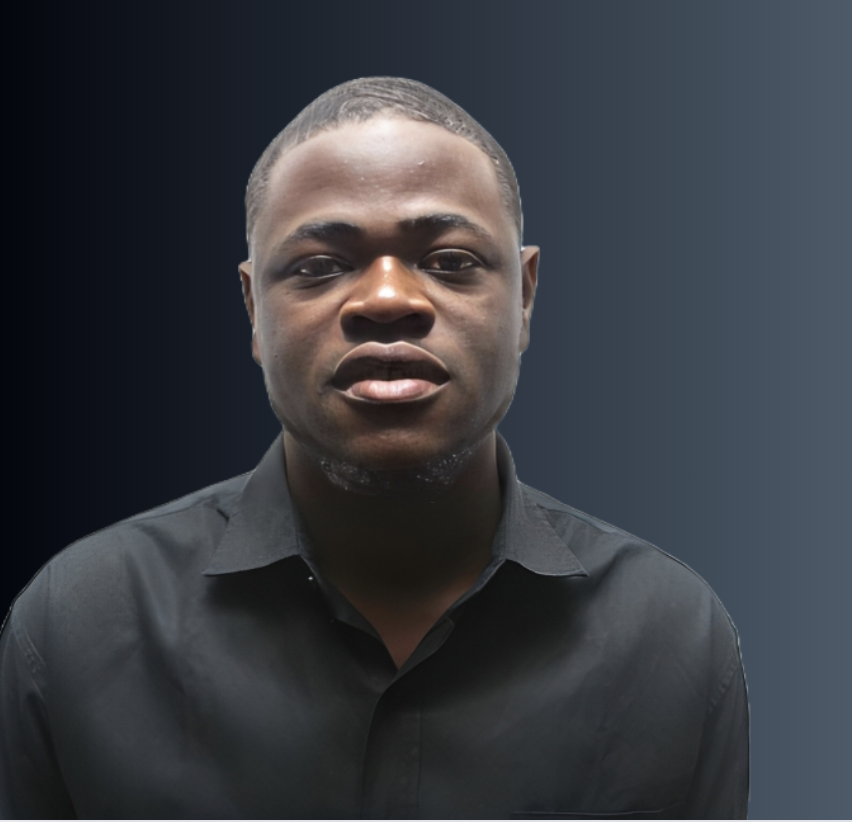
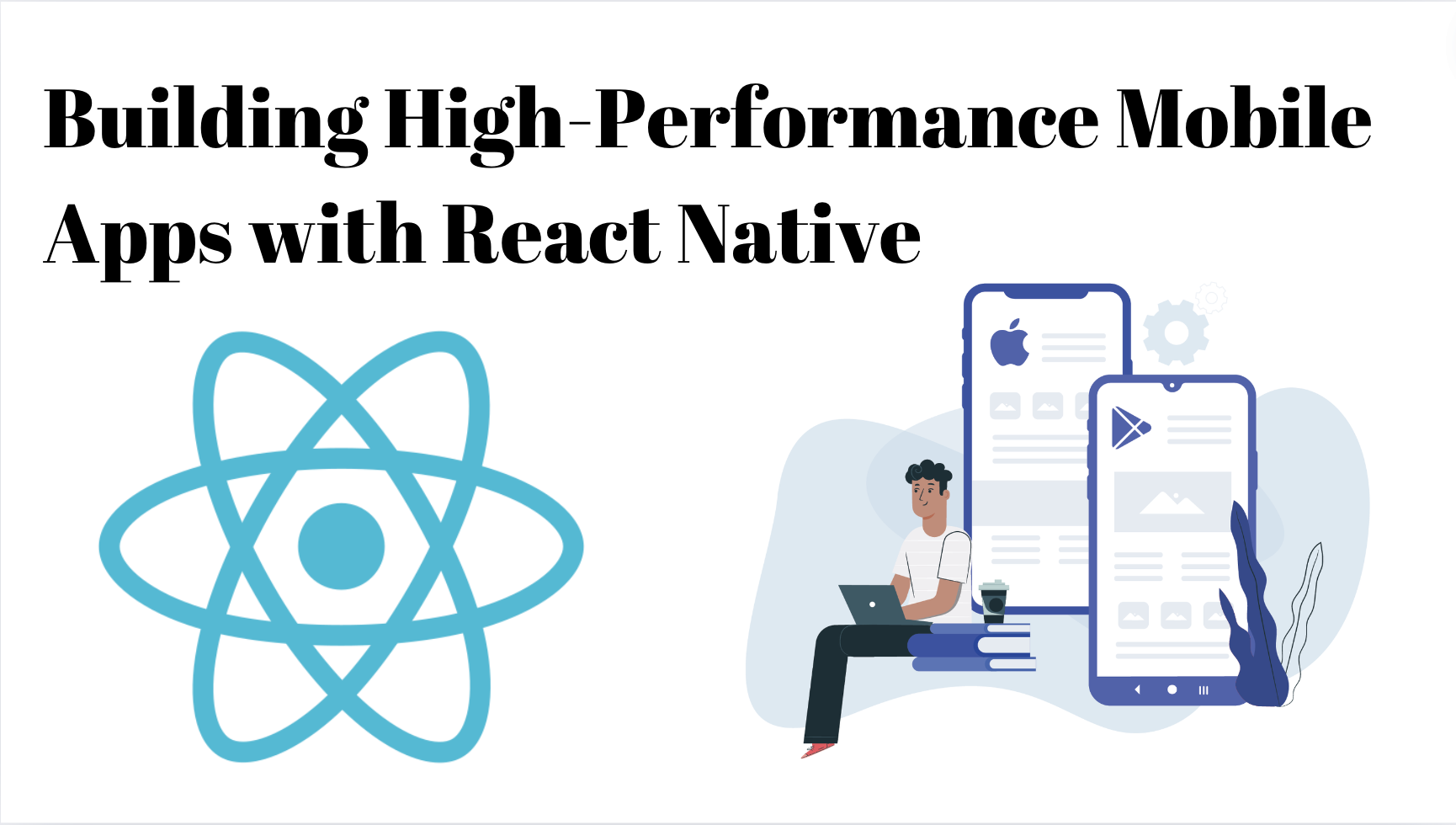
React Native has become a major player in cross-platform mobile development. It promises to create experiences similar to native apps while using a single codebase. But to build React Native apps that perform well, you need to understand the framework's complexities and best practices. Let's explore the methods that make outstanding React Native apps stand out.
Performance Foundations: Building Better
Component Optimisation:
Memoization with
React.memo
:React.memo
stops components from redrawing when they don't need to. Be careful with dependency arrays to avoid too much memoization.useMemo
and useCallback: These hooks store costly calculations and functions to cut down on processing time.
State Management:
Context API (for simple scenarios): Leverage Context to share state efficiently across component trees without prop drilling.
Redux (for complex apps): When managing intricate state interactions, Redux provides a centralized and predictable state management solution.
Redux Toolkit: Streamline Redux development with this official library, offering simplified setup and a standardized pattern.
Navigation:
React Navigation: The go-to navigation library for React Native. Optimize performance by using lazy loading and minimizing unnecessary re-renders of navigation stacks.
Deep Linking: Implement deep linking to allow seamless navigation from external sources into specific parts of your app.
Rendering Mastery: Smooth & Responsive UI
FlatList
andSectionList
:Virtualization: These components render only the items visible on the screen, drastically improving performance for large lists.
Optimized Rendering: Use
getItemLayout
orkeyExtractor
to provide the layout information upfront, preventing layout calculations during scrolling.
Animations:
useNativeDriver
: Enable this prop whenever possible for smoother animations that run on the native UI thread, independent of JavaScript.Animated
API: Leverage theAnimated
API for declarative and performant animations. UseAnimated.timing
,Animated.spring
, andAnimated.decay
for different animation effects.Lottie: Consider Lottie for complex animations, as it uses a pre-rendered format for efficient playback.
Advanced Performance Techniques
Hermes Engine:
- Faster JavaScript Execution: Enable Hermes, a JavaScript engine optimized for React Native, which significantly improves startup time and runtime performance.
Code Profiling:
React Native Performance Monitor: Use this tool to identify bottlenecks in your app's performance. Track frame rates, memory usage, and component rendering times.
Chrome DevTools Profiler: Profile your JavaScript code to identify performance hotspots and optimise them.
Image Optimization:
Image Caching: Implement image caching to prevent unnecessary network requests and speed up image loading.
Image Resizing: Resize images on the server-side to serve appropriately sized images to different devices, reducing download times.
Expert Tips & Tricks
Lazy Loading: Defer loading modules and components until they really are needed.
Batched Bridge Optimisation: Reduce the number of calls from JavaScript to native code and back by batching them together.
Native Modules: Especially in areas where high performance is required, one can consider writing native modules in Java/Kotlin (for Android) or Objective-C/Swift (for iOS).
Continuous Monitoring: Keep checking the metrics of your app in production for regressions to ensure that the performance doesn't degrade with time.
Conclusion
Building high-performance React Native apps is inherently complex, but now, armed with knowledge of these techniques and staying current on the most relevant best practices, you will be well on your way to rendering mobile experiences as responsive and smooth as those natively built. Remember that performance optimisation is a process—keep on analysing, refining, and stretching the limits of what's possible with React Native!
Subscribe to my newsletter
Read articles from Omotayo Alimi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
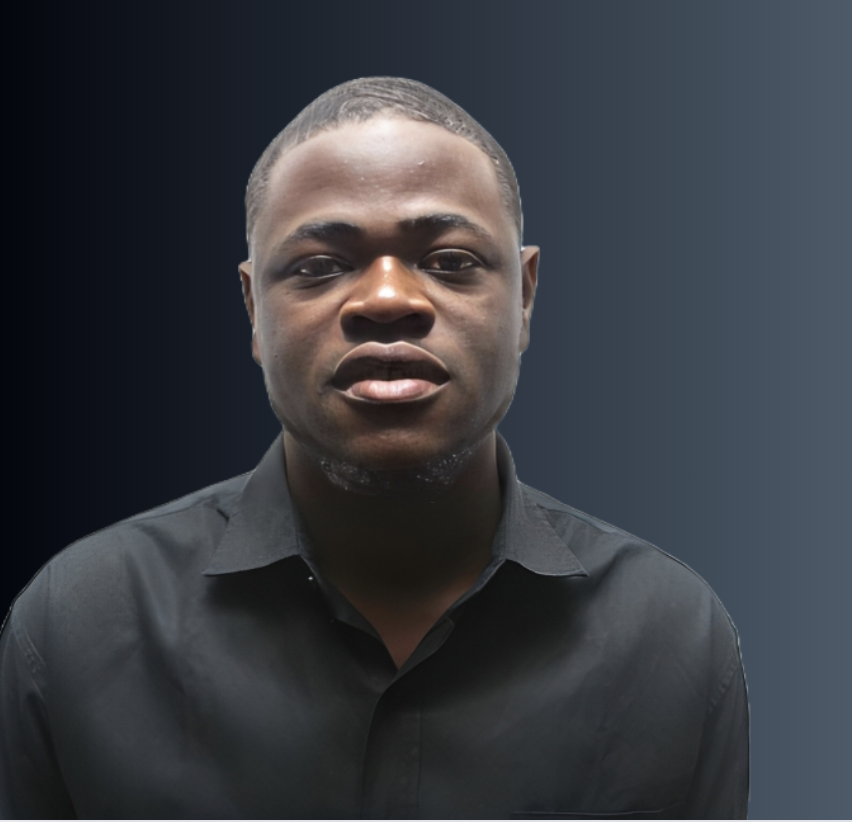
Omotayo Alimi
Omotayo Alimi
Senior Software Engineer