Recurrent Neural Networks: Mastering Sequence Prediction

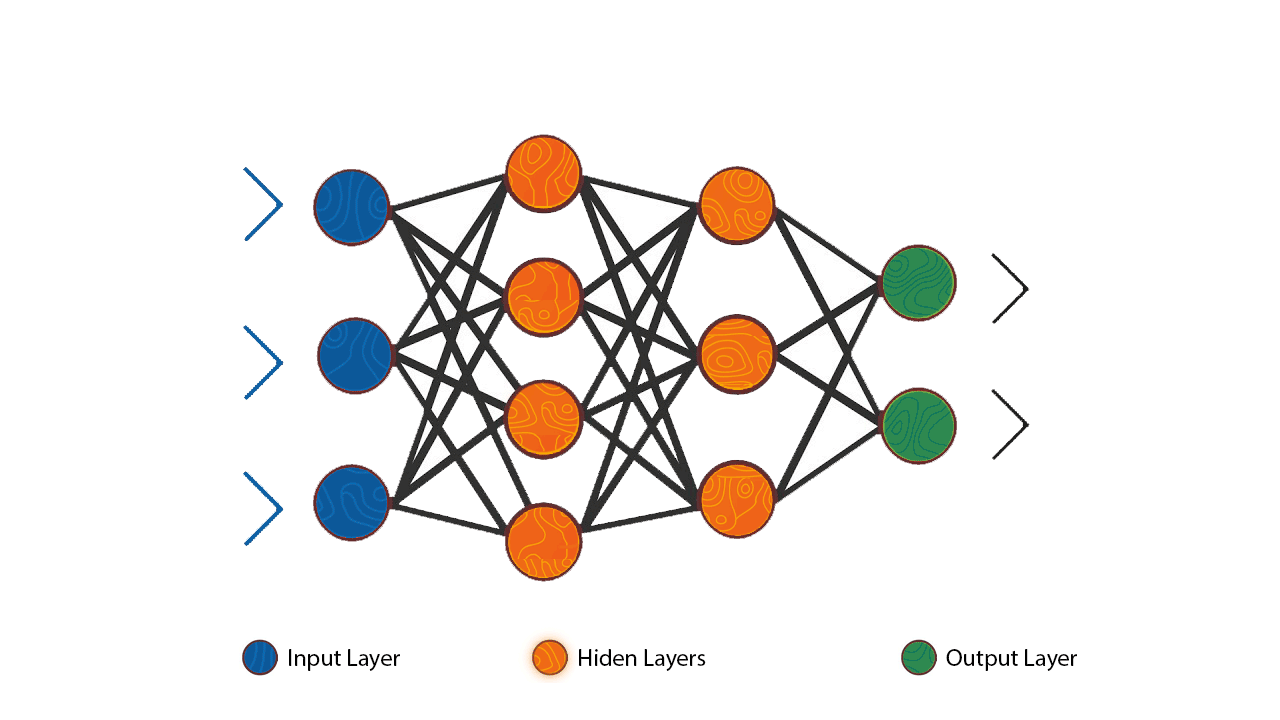
Introduction:
Imagine you're trying to predict the next word in a sentence, the next note in a melody, or the next value in a stock price series. These tasks involve understanding sequences, and that's where Recurrent Neural Networks (RNNs) shine. RNNs are a class of artificial neural networks designed to recognize patterns in sequences of data, making them ideal for tasks like language modeling, speech recognition, and time series forecasting.
The Magic of RNNs
Unlike traditional neural networks, RNNs have a unique ability to remember previous inputs thanks to their internal state, or "memory." This allows them to process sequences of variable length and maintain context over time. Think of it as having a conversation where each sentence builds on the previous one.
Key Concepts
RNN Layers: RNNs can be built using various layers like
nn.RNN
,nn.LSTM
, andnn.GRU
. These layers can be stacked to create deep RNNs.Hidden States: The hidden state is the network's memory, enabling it to process sequences of data.
Sequence Batching: For efficient training, sequences are often batched together. Care must be taken to pad or truncate sequences to the same length within a batch.
A Simple RNN Example in PyTorch
Let's dive into a simple example of an RNN for sequence prediction using PyTorch. We'll predict the next value in a sine wave given previous values.
import torch
import torch.nn as nn
import numpy as np
class SimpleRNN(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(SimpleRNN, self).__init__()
self.hidden_size = hidden_size
self.rnn = nn.RNN(input_size, hidden_size)
self.linear = nn.Linear(hidden_size, output_size)
def forward(self, input_seq, hidden_state):
rnn_out, hidden_state = self.rnn(input_seq.view(len(input_seq), 1, -1), hidden_state)
predictions = self.linear(rnn_out.view(len(input_seq), -1))
return predictions[-1], hidden_state
def init_hidden(self):
return torch.zeros(1, 1, self.hidden_size)
# Parameters
input_size = 1
hidden_size = 20
output_size = 1
seq_length = 30
epochs = 600
lr = 0.01
# Generate dummy data: a simple sine wave
time_steps = np.linspace(0, np.pi, seq_length + 1)
data = np.sin(time_steps)
data.resize((seq_length + 1, 1)) # size becomes (seq_length+1, 1), adds an input_size dimension
targets = data[1:] # all but the first piece of data
data = data[:-1] # all but the last piece of data (labels)
# Convert to tensors
inputs = torch.Tensor(data).unsqueeze(0)
targets = torch.Tensor(targets)
# Instantiate the model
criterion = nn.MSELoss()
rnn = SimpleRNN(input_size, hidden_size, output_size)
optimizer = torch.optim.Adam(rnn.parameters(), lr=lr)
h_state = rnn.init_hidden()
# Training loop
for i in range(epochs):
optimizer.zero_grad()
h_state.detach_()
h_state = h_state.data
output, h_state = rnn(inputs, h_state)
loss = criterion(output.squeeze(), targets)
loss.backward()
optimizer.step()
if i % 100 == 0:
print('Epoch', i, 'loss:', loss.item())
This example illustrates how you can set up a basic RNN in PyTorch to perform sequence prediction. We define our dataset as a sine wave for simplicity.
Scaling Up
To scale up this simple example into more complex tasks such as language modeling or stock price prediction:
Data Preprocessing: Input sequences would need proper preprocessing such as tokenization for text or feature scaling for numerical data.
Hyperparameter Tuning: Optimize layer sizes, learning rate, and other parameters for better performance.
Model Complexity: Add more layers or switch to LSTM or GRU layers which can handle longer dependencies and reduce issues like vanishing gradients.
Further Reading and Resources
Videos and Tutorials
By mastering RNNs, you can unlock the potential to predict and understand sequences in a wide range of applications, from natural language processing to financial forecasting.
Happy coding !!
Happy Coding Inferno !!
Happy Learning !!
Subscribe to my newsletter
Read articles from Sujit Nirmal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sujit Nirmal
Sujit Nirmal
๐ Hi there! I'm Sujit Nirmal, a AI /ML Developer with a passion for creating intelligent, seamless ML applications. With a strong foundation in both machine learning and Deep Learning I thrive at the intersection of data and technology.