How to Build Your First AI Model: A Comprehensive Guide π€π
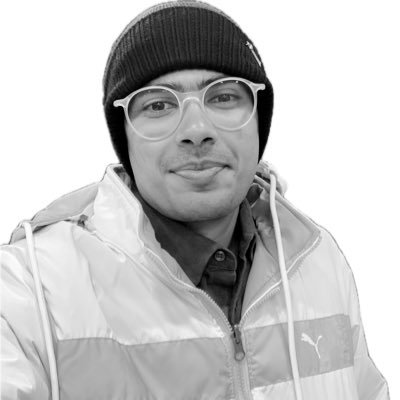

Artificial Intelligence (AI) is transforming industries and redefining the way we interact with technology. Building your first AI model can seem like a daunting task, but with the right guidance and tools, you can embark on this exciting journey. In this blog post, we'll walk through the steps to build your first AI model, from understanding the basics to deploying your model. Whether you're a beginner or an experienced developer, this guide will help you get started with AI. π
Understanding the Basics of AI π§
Before diving into building an AI model, it's essential to understand some fundamental concepts.
What is AI? π€
Artificial Intelligence (AI) refers to the simulation of human intelligence in machines that are programmed to think and learn like humans. AI can be classified into two types:
Narrow AI: AI systems designed for specific tasks, such as image recognition or language translation.
General AI: AI systems with generalized human cognitive abilities (still largely theoretical).
Machine Learning and Deep Learning π§¬
Machine Learning (ML) is a subset of AI that involves training algorithms to learn from data and make predictions. Deep Learning (DL), a subset of ML, uses neural networks with many layers to analyze various factors of data.
Steps to Build Your First AI Model π οΈ
1. Define the Problem π§©
The first step in building an AI model is to define the problem you want to solve. This could be anything from predicting stock prices to recognizing objects in images. Clearly define your objectives and the type of predictions you need.
2. Gather and Prepare Data π
Data is the backbone of any AI model. Collect relevant data that will help your model learn and make predictions. Data can be sourced from publicly available datasets, company databases, or by generating synthetic data.
Example of loading data using Python:
import pandas as pd
# Load dataset
data = pd.read_csv('your_dataset.csv')
# Explore the data
print(data.head())
3. Choose a Model and Algorithm π§
Select a machine learning algorithm that suits your problem. Common algorithms include linear regression, decision trees, and neural networks. For beginners, starting with a simple algorithm like linear regression or decision trees is advisable.
Example of choosing a model using Scikit-Learn:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Split the data into training and testing sets
X = data[['feature1', 'feature2']]
y = data['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize the model
model = LinearRegression()
# Train the model
model.fit(X_train, y_train)
4. Train the Model ποΈββοΈ
Training the model involves feeding it with data and allowing it to learn the patterns. The training process will differ based on the chosen algorithm and the complexity of the model.
5. Evaluate the Model π
After training, evaluate the model's performance using metrics such as accuracy, precision, recall, or F1 score. This step helps you understand how well your model is performing and whether it needs further tuning.
Example of evaluating a model:
from sklearn.metrics import mean_squared_error
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
6. Tune the Model π§
Tuning the model involves adjusting hyperparameters to improve performance. This step might require multiple iterations to find the best combination of parameters.
7. Deploy the Model π
Once satisfied with the model's performance, deploy it to a production environment where it can make real-time predictions. This can be done using various platforms like AWS, Google Cloud, or even custom APIs.
Example of deploying a model using FastAPI:
from fastapi import FastAPI, Request
import joblib
from pydantic import BaseModel
app = FastAPI()
# Load the trained model
model = joblib.load('model.pkl')
class Features(BaseModel):
feature1: float
feature2: float
@app.post('/predict')
async def predict(features: Features):
data = [[features.feature1, features.feature2]]
prediction = model.predict(data)
return {'prediction': prediction.tolist()}
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, host='0.0.0.0', port=8000)
Building your first AI model is an exciting and rewarding experience. By following these stepsβdefining the problem, gathering and preparing data, choosing and training a model, evaluating and tuning it, and finally deploying itβyou can create an AI model that solves real-world problems. Remember, the key to success in AI is continuous learning and experimentation. So, dive in, experiment, and have fun with AI! π€β¨
Happy coding! ππ
Feel free to share your experiences and any additional tips in the comments below! π¬
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
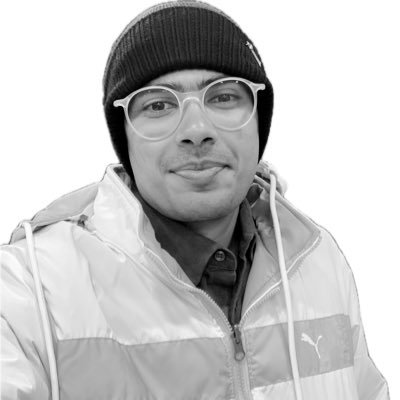
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on β¨οΈ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix π¨π»βπ»