Understanding Prop Validation in React.js
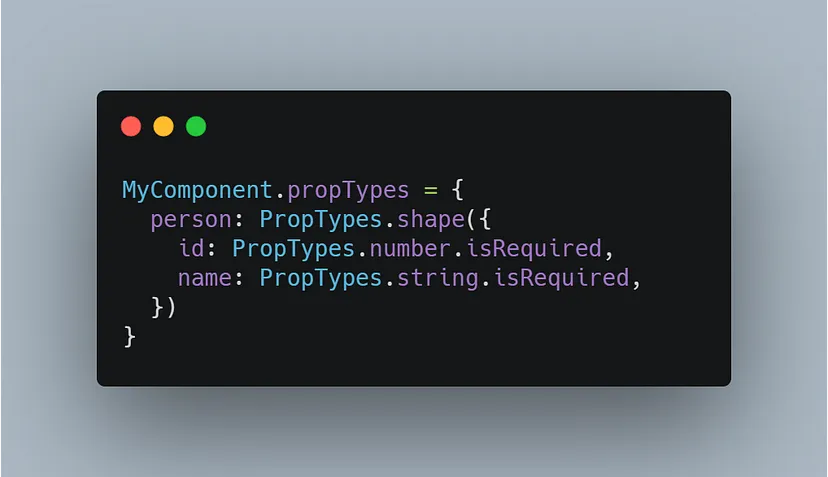
Prop validation in React.js is a powerful tool that ensure that components receive props of the correct type. This is especially useful in large applications where components can be reused extensively. By validating props, catching bugs becomes easy, and also improve code readability.
And their is one library to rescue PropTypes
.
Lets Understand
What are Props in React?
Props, short for properties, are used to pass data from one component to another in React. They enable components to be dynamic and reusable.
Why Validate Props?
Prop validation offers several benefits:
Error Prevention: Catch potential issues early in development.
Improved Readability: Makes the code more understandable by clearly defining expected prop types.
Documentation: Acts as a form of self-documentation for the component's API.
Maintainability: Helps in maintaining the integrity of the component as it evolves.
Introducing PropTypes
React provides a built-in library called PropTypes
for prop validation. It allows developers to specify the type and shape of props that a component should receive.
To use PropTypes
, you need to import it from the prop-types
package. If it's not already installed in your project, you can add it using npm or yarn
npm install prop-types
or
yarn add prop-types
Basic Usage of PropTypes
Here's a simple example to demonstrate how to use PropTypes
in a React component:
import React from 'react';
import PropTypes from 'prop-types';
function Greeting({ name, age }) {
return (
<div>
<h1>Hello, {name}!</h1>
<p>You are {age} years old.</p>
</div>
);
}
Greeting.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
export default Greeting;
In this example:
name
is expected to be a string and is marked as required.age
is expected to be a number and is also marked as required.
If the Greeting
component receives props of the wrong type or if any required props are missing, React will log a warning in the console.
Common PropTypes
Here are some common prop types provided by the PropTypes
library:
PropTypes.any
: The prop can be of any data type.PropTypes.bool
: The prop should be a boolean.PropTypes.number
: The prop should be a number.PropTypes.string
: The prop should be a string.PropTypes.func
: The prop should be a function.PropTypes.array
: The prop should be an array.PropTypes.object
: The prop should be an object.PropTypes.node
: The prop can be any renderable content (numbers, strings, elements, or an array containing these types).PropTypes.element
: The prop should be a React element.PropTypes.instanceOf
: The prop should be an instance of a specified class.PropTypes.oneOf
: The prop should be one of the specified values.PropTypes.oneOfType
: The prop should be one of the specified types.PropTypes.arrayOf
: The prop should be an array of a specified type.PropTypes.objectOf
: The prop should be an object with values of a specified type.PropTypes.shape
: The prop should be an object with a specific shape.
Advanced Prop Validation
Let's consider a more complex example with nested props and custom validation:
import React from 'react';
import PropTypes from 'prop-types';
function UserProfile({ user }) {
return (
<div>
<h2>{user.name}</h2>
<p>Age: {user.age}</p>
<p>Email: {user.email}</p>
<p>Address: {user.address.city}, {user.address.country}</p>
</div>
);
}
UserProfile.propTypes = {
user: PropTypes.shape({
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
email: PropTypes.string.isRequired,
address: PropTypes.shape({
city: PropTypes.string.isRequired,
country: PropTypes.string.isRequired,
}).isRequired,
}).isRequired,
};
export default UserProfile;
In this example:
The
user
prop is an object with specific properties (name
,age
,email
, andaddress
).The
address
prop itself is an object withcity
andcountry
properties.
Custom Prop Validation
Sometimes, you may need custom validation logic. You can define a custom prop type validator function to achieve this:
import React from 'react';
import PropTypes from 'prop-types';
function CustomComponent({ customProp }) {
return <div>{customProp}</div>;
}
function customPropValidator(props, propName, componentName) {
if (props[propName] !== 'validValue') {
return new Error(
`Invalid prop \`${propName}\` supplied to \`${componentName}\`. Validation failed.`
);
}
}
CustomComponent.propTypes = {
customProp: customPropValidator,
};
export default CustomComponent;
In this example, customPropValidator
checks if the customProp
prop equals 'validValue'. If not, it returns an error.
Conclusion
To stand out the quality start using PropTypes
.
Subscribe to my newsletter
Read articles from Vaishnavi Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by