Efficient Thread Management and API Call Handling in Backend Servers
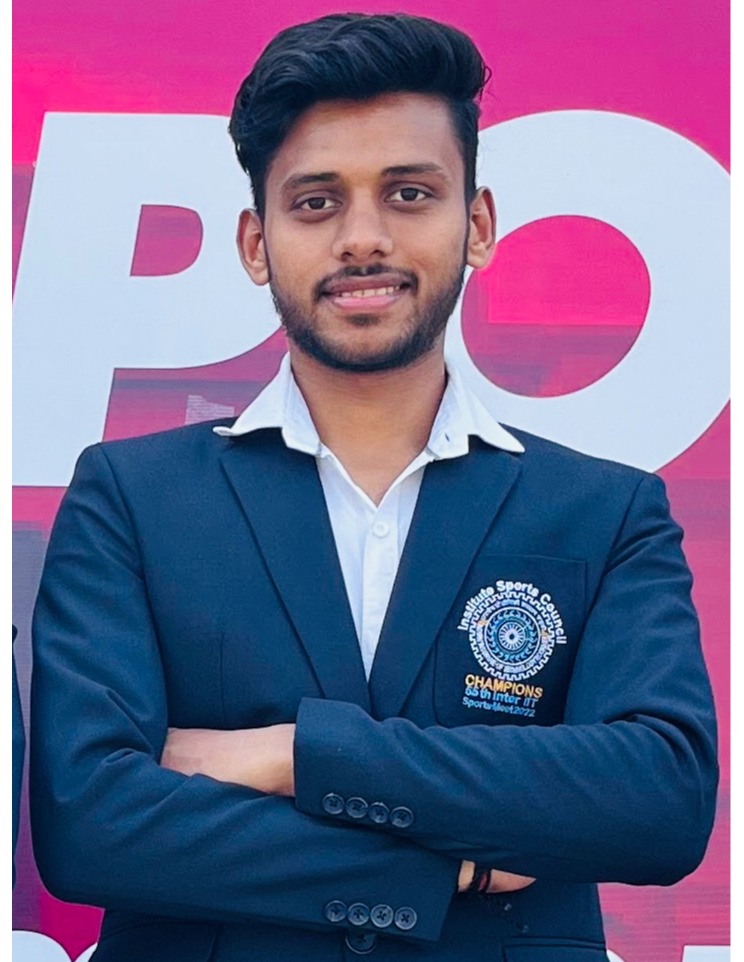
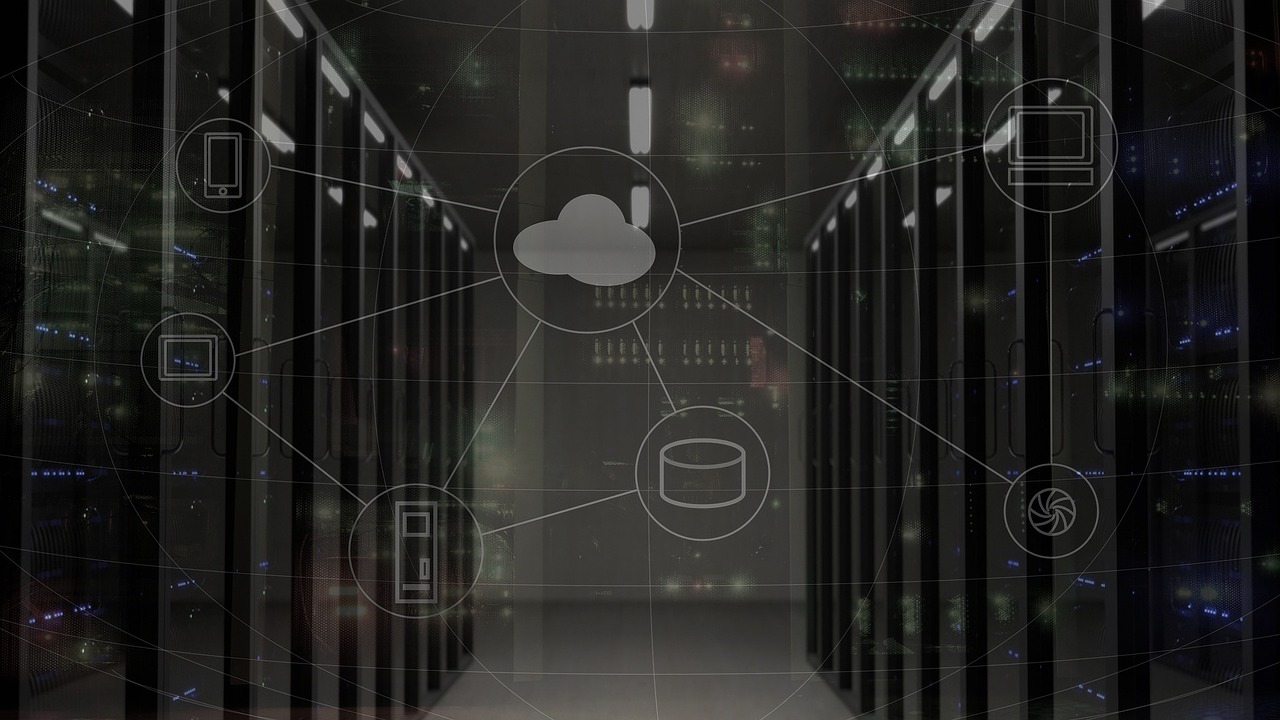
Outline:
Introduction
Fundamental Concepts
Thread Management and API Call Handling
Single-Threaded Environment (Node.js)
Multi-Threaded Environment (Java)
Factors Affecting API Call Capacity
Estimating API Call Capacity
Conclusion
Efficient thread management and API call handling are crucial for the performance and responsiveness of backend systems. This article explores the fundamental concepts of tasks, processes, and threads, and discusses how different server environments handle threading and API calls.
Introduction
Backend servers need to manage multiple concurrent tasks efficiently to provide fast and reliable services. Understanding how threading and API call handling works can help developers optimize server performance.
Fundamental Concepts
Task: A unit of work that the system needs to perform, such as executing a program, processing data, or performing an input/output operation.
Process: An instance of a program being executed, containing the program code and its current activity. Each process has its own memory space.
Thread: The smallest unit of execution within a process. Threads within the same process share memory but execute independently, allowing for concurrent task execution.
Multitasking: The ability of an OS to handle multiple tasks at the same time through time-sharing or true parallel execution.
Parallel Processing: Simultaneous execution of multiple processes or threads, often on multiple CPUs or cores, to perform tasks concurrently.
Thread Management and API Call Handling
The number of threads that can be created and the capacity to handle API calls depend on system resources, JVM settings, OS limits, and application design. Efficient thread management is essential to avoid performance degradation.
Single-Threaded Environment (Node.js)
Node.js uses a single-threaded event loop to handle tasks asynchronously. This non-blocking I/O model allows it to handle multiple requests concurrently despite being single-threaded.
Example: API Call in Node.js
const express = require('express');
const app = express();
const axios = require('axios');
const db = require('./db');
app.get('/data', async (req, res) => {
try {
const dbData = await db.query('SELECT * FROM my_table');
const apiData = await axios.get('https://api.thirdparty.com/data');
res.json({ dbData, apiData: apiData.data });
} catch (error) {
res.status(500).send('Error occurred');
}
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Multi-Threaded Environment
In multi-threaded environments, servers can handle concurrent tasks using multiple threads, each managing separate tasks like API calls and database interactions.
Example: Thread Pool in Java
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
private static final int MAX_THREADS = 100;
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(MAX_THREADS);
for (int i = 0; i < 1000; i++) {
executorService.submit(() -> {
handleApiCall();
});
}
executorService.shutdown();
}
private static void handleApiCall() {
// Implementation of API call handling logic
}
}
Factors Affecting API Call Capacity
Hardware Specifications: More powerful servers can handle more concurrent connections.
Application Design: Efficient code and optimized resource management improve the server's capacity.
Thread Pool Configuration: Proper thread pooling avoids overwhelming system resources.
Load Balancing: Distributing requests across multiple servers increases overall capacity.
Estimating API Call Capacity
To estimate the number of API calls a server can handle:
Benchmarking and Load Testing: Tools like Apache JMeter or Gatling can simulate high traffic and measure server response.
Monitoring and Profiling: Tools like Prometheus and Grafana can help monitor CPU, memory, and thread usage, while profiling tools identify inefficiencies.
Conclusion
Efficient thread management and API call handling are critical for backend server performance. By understanding the differences between single-threaded and multi-threaded environments and optimizing resource management, developers can build responsive and scalable applications. This knowledge is essential for ensuring that backend systems can handle high volumes of requests efficiently and reliably.
Subscribe to my newsletter
Read articles from anand ranjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
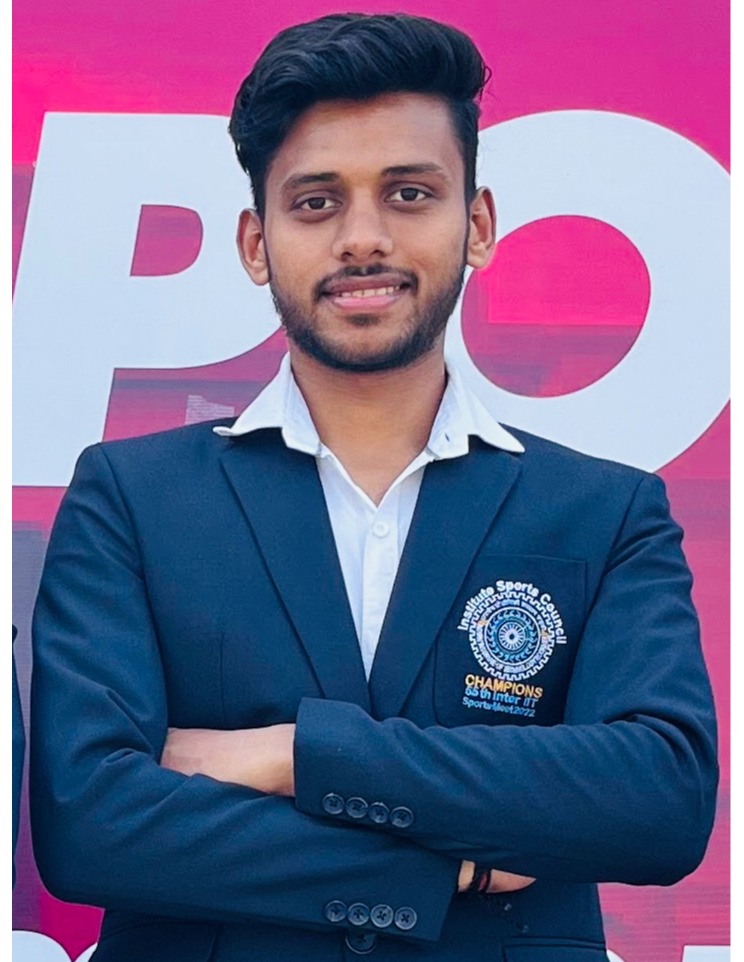
anand ranjan
anand ranjan
Full-stack developer with more than one year of experience in Node.js and Next.js stack-based application development, with extended knowledge of cloud computing and production deployment.