Integrating PHP Backend with React Native: Handling Date Range Selection
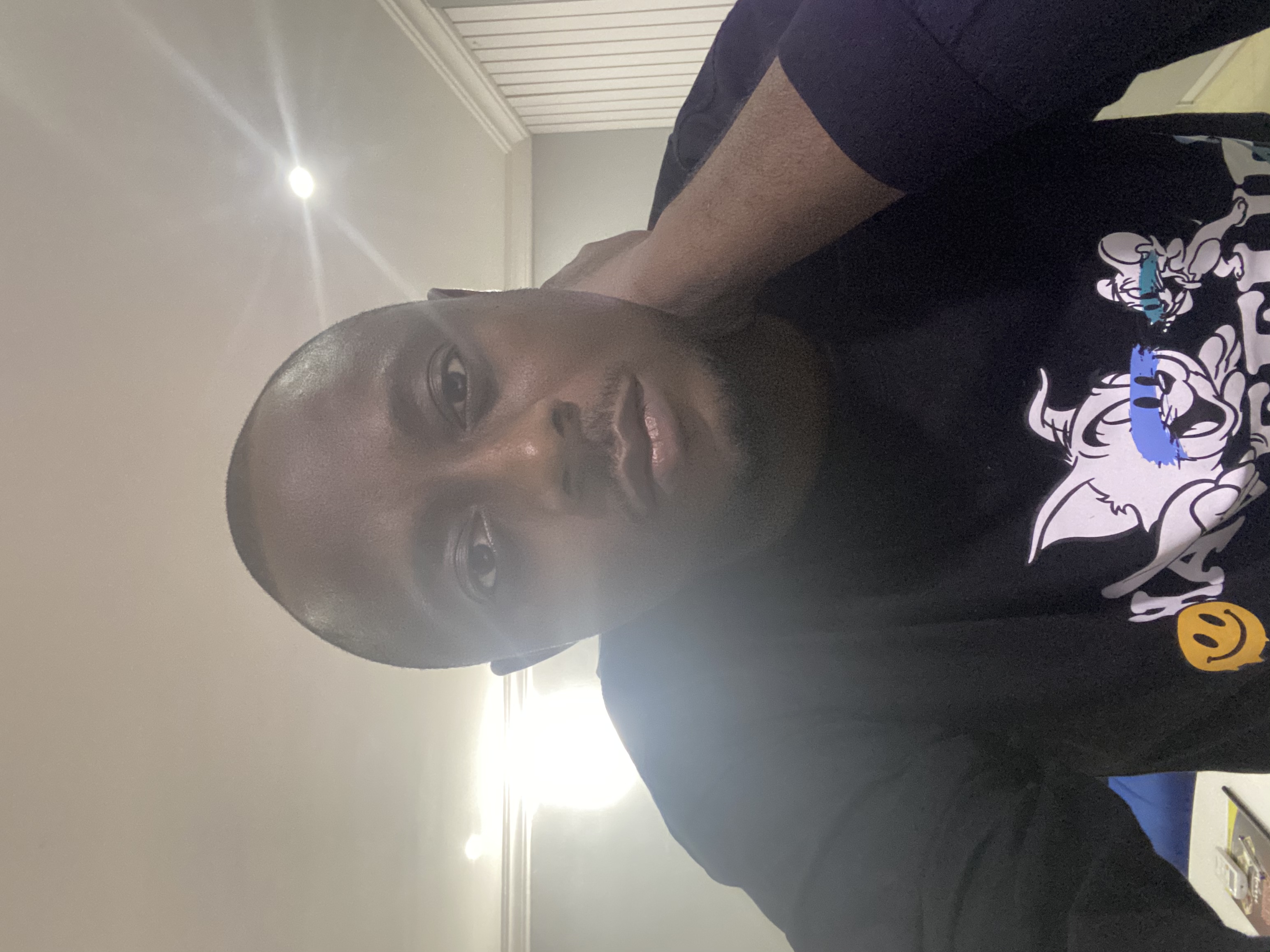
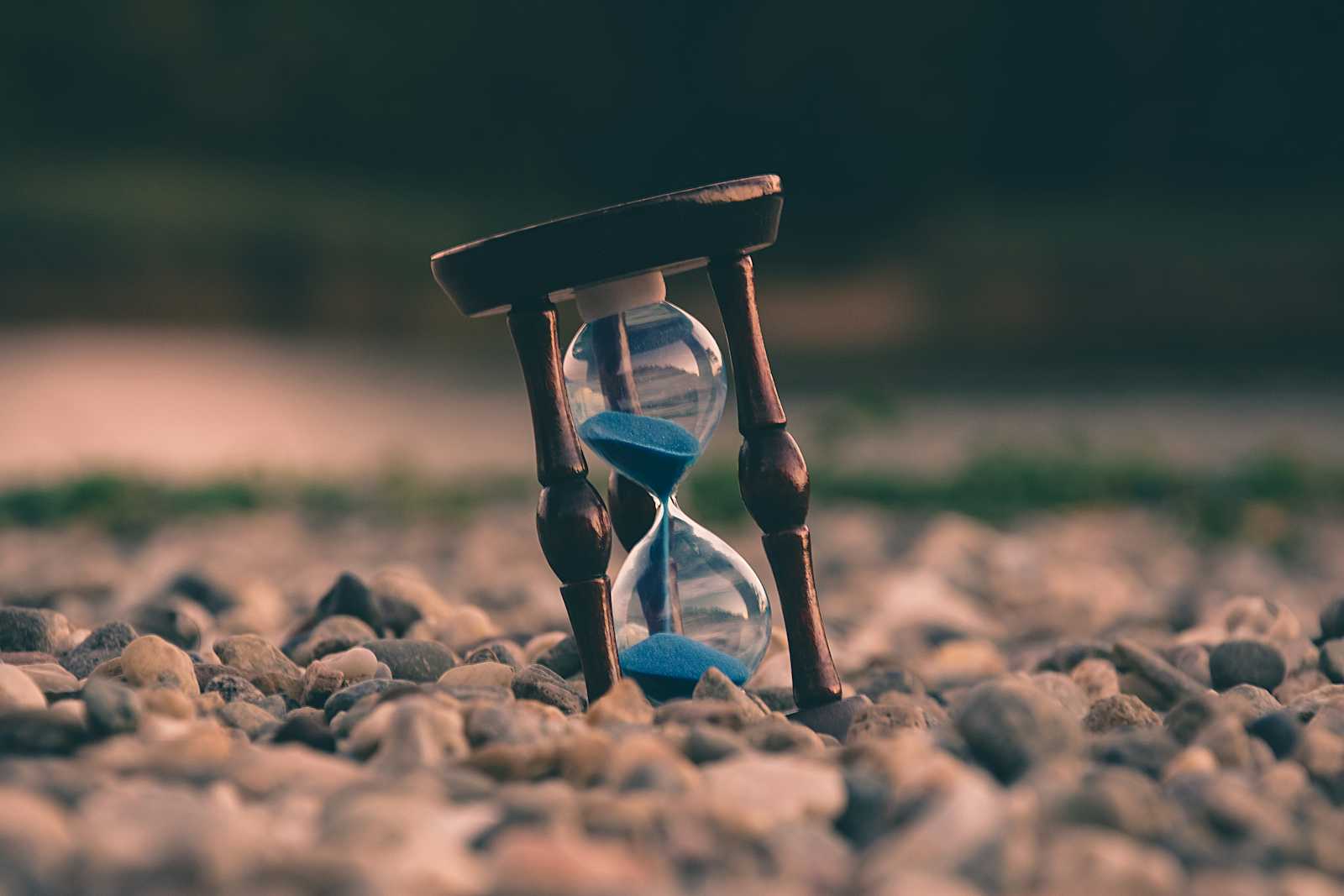
In this blog post, we will walk through the process of integrating a PHP backend with a React Native frontend to handle date range selection and fetch data accordingly. We will cover the PHP script for the backend and the React Native code for the frontend, ensuring smooth communication between the two.
Prerequisites
Basic knowledge of PHP and React Native.
A working PHP server.
A React Native development environment set up.
PHP Backend
Step 1: Database Configuration
First, ensure you have a database configuration file (config.php
) to connect to your database. Here is a sample configuration:
<?php
$servername = "your_servername";
$username = "your_username";
$password = "your_password";
$dbname = "your_dbname";
$link = new mysqli($servername, $username, $password, $dbname);
if ($link->connect_error) {
die("Connection failed: " . $link->connect_error);
}
?>
Step 2: Create the PHP Script
Next, create a PHP script (userhire.php
) to handle the POST request from the React Native application, process the date range, and fetch data from the database.
<?php
// Database connection
include("config.php");
// Check connection
if ($link->connect_error) {
die("Connection failed: " . $link->connect_error);
}
// Get POST data
$data = json_decode(file_get_contents('php://input'), true);
// Extract selected dates from POST data
$selectedDate = $data['selectedDate'];
$selectedDate1 = $data['selectedDate1'];
// Convert to Unix timestamps
$startTimestamp = strtotime($selectedDate);
$endTimestamp = strtotime($selectedDate1);
// Query to fetch data from car_selections
$query = "SELECT * FROM car_selections";
$result = $link->query($query);
$selectedOfferings = [];
$offerDetails = [];
// Check each row to see if the range overlaps
while ($row = $result->fetch_assoc()) {
$rowSelectedDate = (int)$row['selected_date'];
$rowSelectedDate1 = (int)$row['selected_date1'];
// Check if the given range overlaps with the range in the database
if (($startTimestamp <= $rowSelectedDate1) && ($endTimestamp >= $rowSelectedDate)) {
// Save the selected offering
$selectedOfferings[] = $row['selected_offering'];
// Save the selected date details for each offering
$offerDetails[] = [
'selected_offering' => $row['selected_offering'],
'selected_date' => $row['selected_date'],
'selected_date1' => $row['selected_date1'],
'rowSelectedDate' => $rowSelectedDate,
'rowSelectedDate1' => $rowSelectedDate1
];
}
}
// Convert selected offerings array to a comma-separated list for SQL IN clause
$selectedOfferingsList = "'" . implode("','", $selectedOfferings) . "'";
// Query to fetch data from hireAgent where reference is not in selected_offerings
$hireAgentQuery = "SELECT * FROM hireAgent WHERE status7=2 AND status15=1 AND reference NOT IN ($selectedOfferingsList)";
$hireAgentResult = $link->query($hireAgentQuery);
$response = [];
// Fetch data from hireAgent
while ($row = $hireAgentResult->fetch_assoc()) {
$response[] = $row;
}
// Return the data as JSON
header('Content-Type: application/json');
echo json_encode($response);
// Close the database connection
$link->close();
?>
This PHP script receives the date range from the React Native application, converts them to Unix timestamps, checks for overlapping date ranges in the database, and returns the relevant data in JSON format.
React Native Frontend
Step 1: Install Required Dependencies
Ensure you have the required dependencies installed in your React Native project:
npm install @react-navigation/native
npm install @react-navigation/native-stack
npm install @react-navigation/drawer
npm install react-native-screens react-native-safe-area-context
Step 2: Create the React Native Component
Create a React Native component that sends the date range to the PHP script and handles the response.
import React, { useState, useCallback } from 'react';
import { useFocusEffect } from '@react-navigation/native';
const YourComponent = ({ selectedDate, selectedDate1 }) => {
const [offerDetails, setOfferDetails] = useState([]);
const [filteredOfferings, setFilteredOfferings] = useState([]);
useFocusEffect(
useCallback(() => {
const fetchData = async () => {
try {
const response = await fetch('https://your-url.com/userhire.php', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({
selectedDate: new Date(selectedDate).toISOString(),
selectedDate1: new Date(selectedDate1).toISOString()
})
});
const jsonData = await response.json();
if (jsonData && jsonData.length > 0) {
setOfferDetails(jsonData);
setFilteredOfferings(jsonData); // Initialize with all data
} else {
setOfferDetails([]);
setFilteredOfferings([]);
}
} catch (error) {
console.error(error);
}
};
fetchData();
}, [selectedDate, selectedDate1])
);
return (
// Your component JSX
);
};
export default YourComponent;
This React Native component uses useFocusEffect
from @react-navigation/native
to trigger the data fetching when the component gains focus. It sends a POST request with the selected date range to the PHP script and handles the JSON response to update the state.
Conclusion
By following these steps, you can integrate a PHP backend with a React Native frontend to handle date range selection and fetch data accordingly. This approach ensures smooth communication between the frontend and backend, enabling you to build dynamic and responsive applications.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
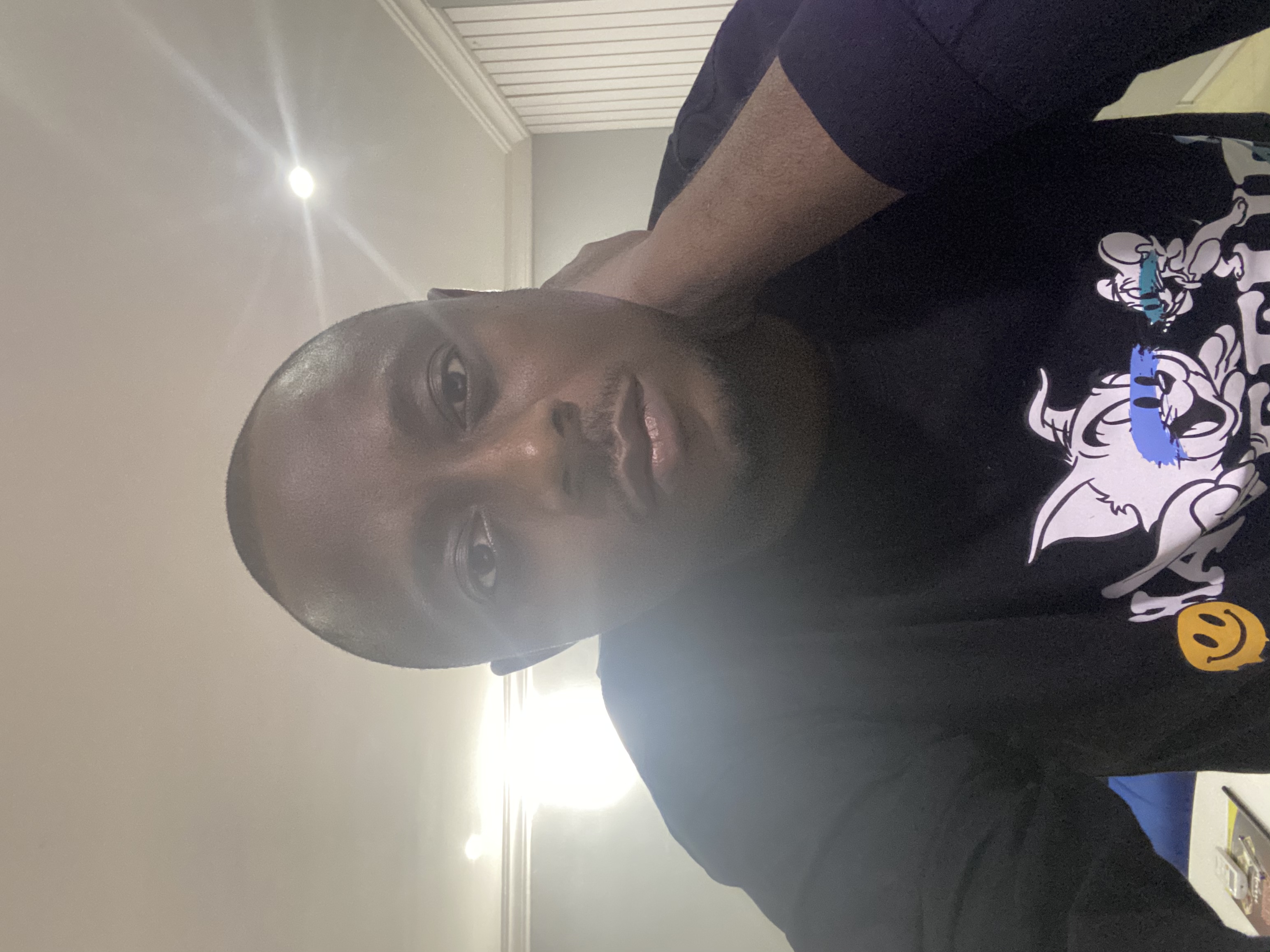
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.