You don't have to write JavaScript for everything
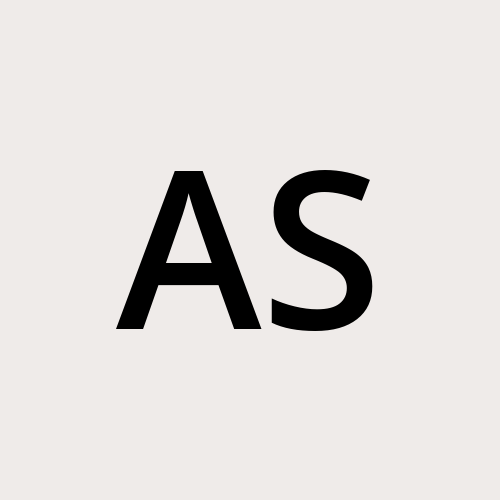
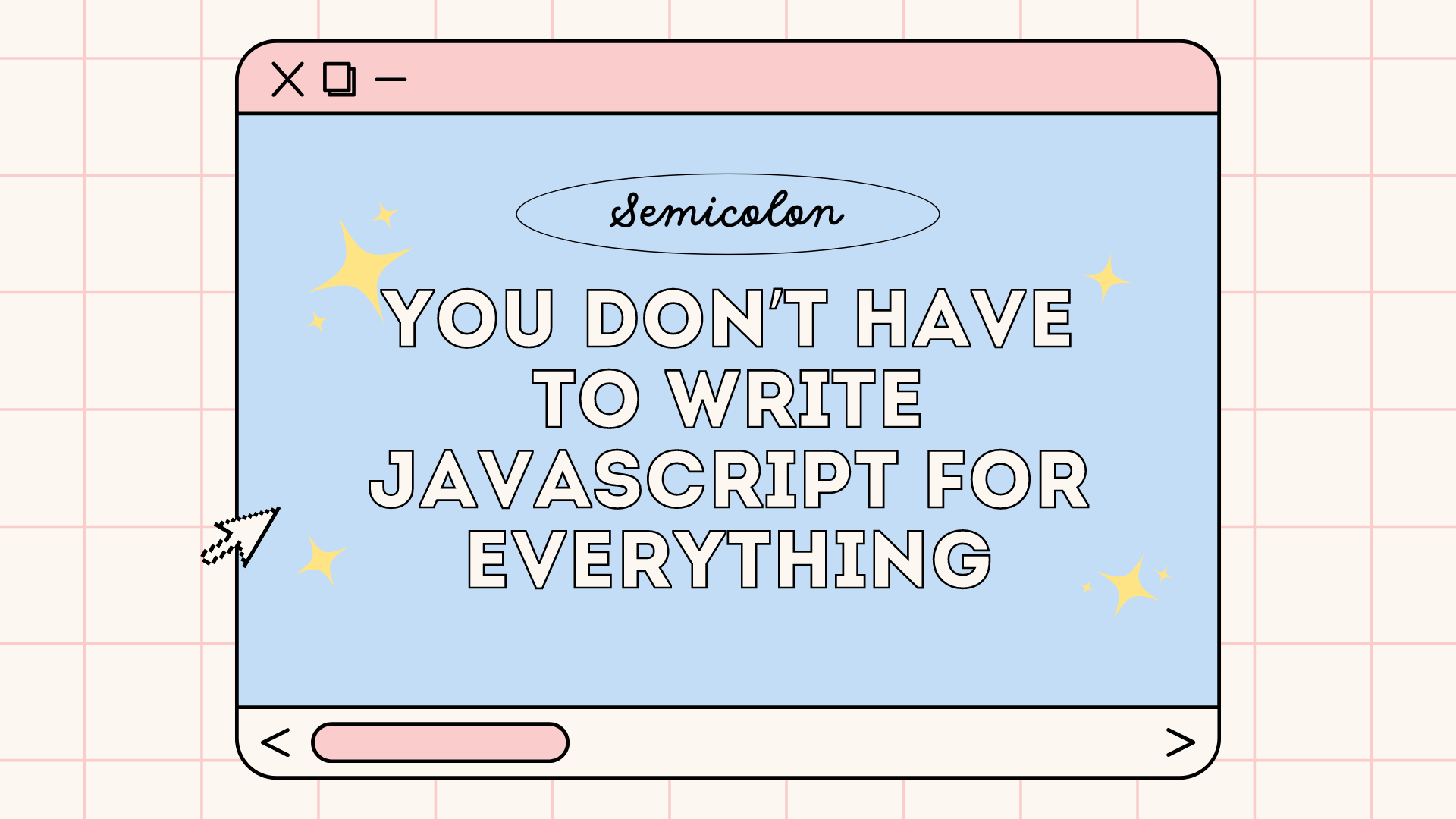
JavaScript is a very versatile language. It does everything—you can use it to build web, mobile, and desktop applications, then AI, drones, robots, games. I mean, it can even call the aliens from space. But good luck getting it to center
a div on the first try! Catching aliens seems intriguing, though a bit risky. They might abduct you just to debug their code!
Beyond the fascination with aliens, JavaScript has become ubiquitous in our web applications, powering everything from animations and modals to switches, dialogs, accordions, and auto-suggestions—the list is practically endless. You might be wondering, if these features are being built and effectively used, why am I voicing concerns about them? You're right to ask, but there's value in considering an alternative approach. When a task can be accomplished using HTML and CSS alone, it often brings benefits that are overlooked when defaulting to JavaScript.
What are the benefits of using HTML and CSS over JavaScript?
Imagine you’re about to hang a picture frame on your wall. Now, you could grab a simple nail and hammer, but nope—you decide to go all out. You whip out a power drill, a laser level, and for good measure, you call in a full construction crew. There’s a hard hat, blueprints, and someone yelling “Measure twice, cut once!” Sure, you’ll get that frame up, but let’s be honest, your neighbour now thinks you’re building an addition to your house.
This, my friend, is exactly what it’s like when you reach for JavaScript when plain old HTML and CSS could do the job just fine. Sometimes, less is more, and a little simplicity goes a long way.
You’re tasked with building a modal, dialogue, suggestion box, or accordion. The instinctive choice might be to reach for JavaScript or a related library. However, there are some important considerations to keep in mind.
Single Threaded: JavaScript operates on a single thread, meaning it runs on the main thread of your browser. When you use JavaScript—or a library that relies on it—it places additional load on this main thread. This can lead to other processes being delayed, potentially causing performance issues as the browser waits for JavaScript to complete its tasks.
Reduced Load: HTML and CSS are rendered directly by the browser, resulting in faster performance. JavaScript, on the other hand, introduces an additional layer of complexity, which can slow down the rendering process.
Fallbacks: If JavaScript is disabled or fails to load, features built with HTML and CSS will still function, ensuring a more resilient user experience.
These are just a few of the benefits of opting for HTML and CSS when possible. In the past, many features required JavaScript to implement, but advancements in HTML and CSS have made it possible to achieve these effects without the need for JavaScript. Let’s explore some of these capabilities in more detail.
Custom Switches
In your development experience, you may have encountered fancy switches or toggles built with advanced JavaScript code, which can be tricky to grasp. However, CSS has significantly evolved, allowing you to create such elements using purely CSS. Isn't it amazing? Let's go through it and understand how it's been created.
The HTML part for the switch is quite intuitive; we're just using a label tag with an input checkbox inside, and that's it. However, the magic is done by CSS.
Though the CSS part is intuitive as well, there seems to be a lot of CSS. What's important here is appearance: none
. As inputs and images are replaced content, this means that as the browser renders pages, it leaves little holes for these elements. These holes are later filled by images from the source or form controls from the corresponding Operating System. This is why form controls have historically been inconsistent across different machines. Replaced elements can't have pseudo-elements.
What appearance: none
does is tell the browser not to replace the element or send it to the OS. Instead, it leaves the element as it is, allowing us to style it with CSS.
The rest of the CSS is quite Instinctive. The ::before
pseudo-element creates the switch toggle, while the :checked
pseudo-class is used to change the appearance when the switch is toggled.
The CodePen example below demonstrates the full code of how you can create a custom switch without writing a single line of JavaScript.
Auto Suggest
This feature is used in many places in our web application as it provides a useful utility for users. Though implementing it traditionally required us to load either a complex JavaScript library or write a lot of custom code, advancements in HTML have made the implementation of auto-suggestions quite simple.
As we can see in the code snippet, we're using an input tag with the list attribute value set to the ID of the datalist. The datalist tag provides a list of predefined values to suggest to the user for this input. Whatever we type, if it's compatible with the values inside the list, will be suggested. The values provided are suggestions, not requirements: users can select from this predefined list or provide a different value if allowed. The UI of the suggestion list will be shown by the browser, so it will vary across different browsers.
The CodePen example below demonstrates the full code of how you can create a auto suggest without writing a single line of JavaScript.
Accordion
While switch bulbs might not be as iconic as accordions, they do have their own quirks. Historically, implementing them required JavaScript or other libraries to manage their open and closed states. But guess what? Now you can create them using just HTML and CSS, and the best part is, no JavaScript required!
The <details>
element creates a disclosure widget that remains hidden by default and becomes visible only when toggled to the open state. Initially, it is in the closed state, and clicking it will expand to reveal the content. By default, all content within the <details>
element is hidden except for the <summary>
, which serves as the visible part of the widget. The <details>
element also has an open state in the live DOM that can be styled as needed. However, there are currently no direct methods to animate the opening and closing transitions of the accordion.
By using open
attribute with details
element, we can specify if that accordion should be opened by default.
Another interesting aspect to mention is that the marker, which displays the open and close state of the widget, can also be customized.
The CodePen example below demonstrates the full code of how you can create an accordion without writing a single line of JavaScript.
Dialog
You might recall the famous line from The Matrix: "I'm going to show them a world where anything is possible." Similarly, if you've implemented a dialog modal in your programming journey, you know it can be quite comprehensive. Creating a dialog involves managing various elements such as the dialog content, buttons, and state. However, with the latest HTML advancements, this task has become significantly easier. Although achieving it without any JavaScript isn't entirely feasible, we can employ a few clever tricks to make it work. Consider it a little cheat code to streamline the process!
The above code snippet shows that we can create a dialog using the form
element with the dialog
method. The button click will open the dialog, though we'll need to use some JavaScript, as this functionality is only available through JavaScript. When the button is clicked, the dialog opens in the top layer
of the browser. This new concept solves the complex problem of managing z-index, where developers often had to use extremely high z-index values to keep elements on top. With the top layer
, the browser adds another layer between your page and the screen, allowing elements to be promoted to this top layer
. As a result, the dialog will always stay on top of everything else, making z-index management irrelevant. The form is used because when we submit a form with the dialog
method, it will automatically close the dialog.
We can style the dialog, add a backdrop, and incorporate animations since it's fully customizable. By using just a small amount of JavaScript, it effectively solves a complex problem.
The CodePen example below demonstrates the full code of how you can create an Dialog by writing little JavaScript.
Conclusion
As we've seen, the world of HTML and CSS has evolved to a point where things now seem more intuitive, and complex tasks that once required libraries or intricate code can now be achieved with just HTML and CSS. I've shown only a few examples, but there are many more out there, and they can be seen all over the internet. With the rapid growth of these technologies, it feels like it's not far away that almost any interactive element can be created without needing JavaScript. I would like to give a shout-out to Kilian Valkhof, as his talks and videos have been instrumental in helping me grasp these advancements.
Subscribe to my newsletter
Read articles from Asif Siddique directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
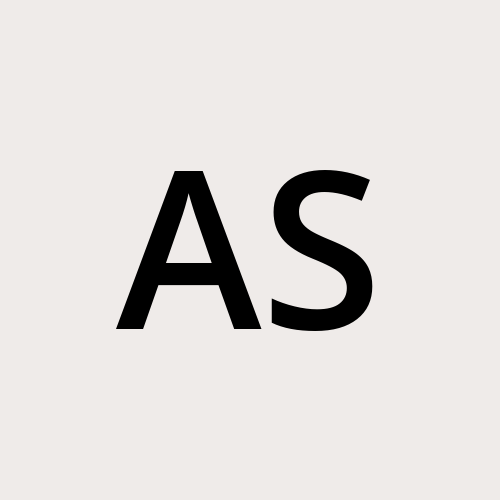