Top UI Frameworks of React in 2024
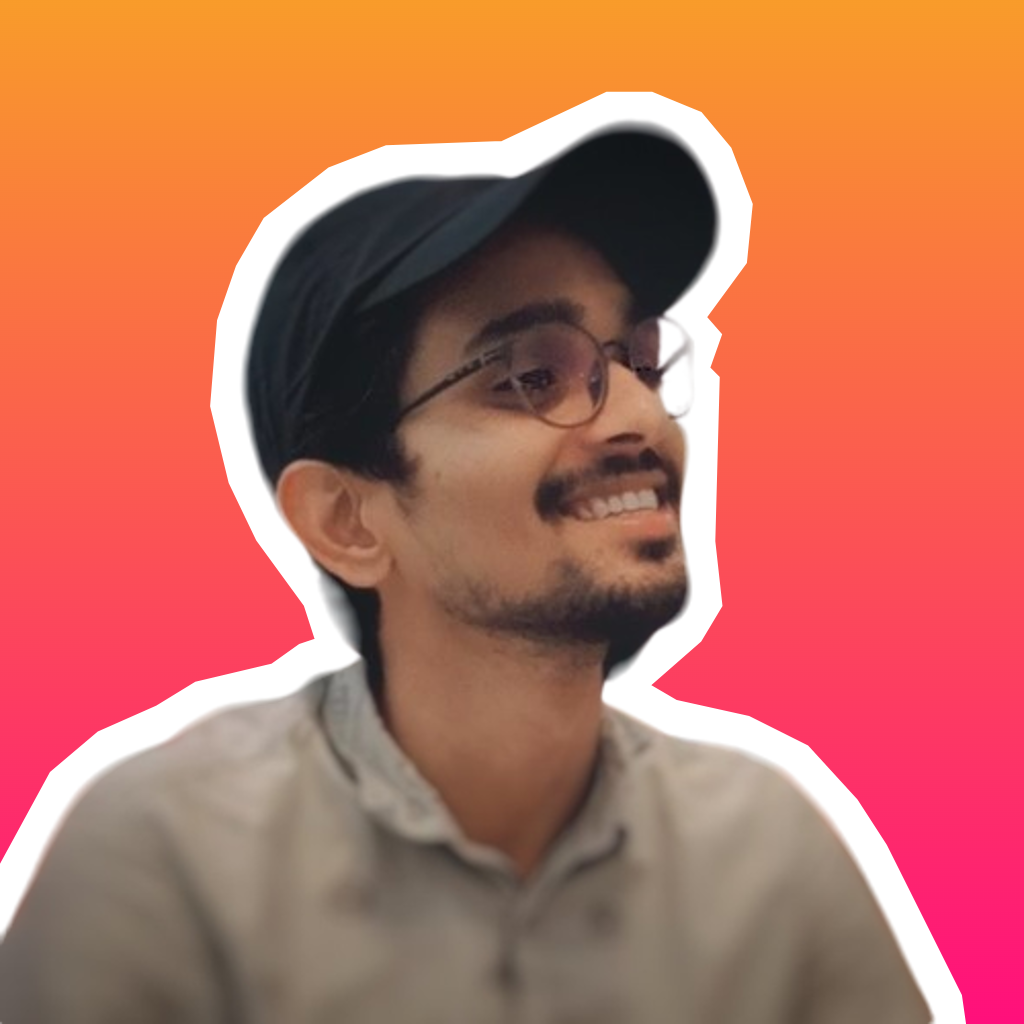
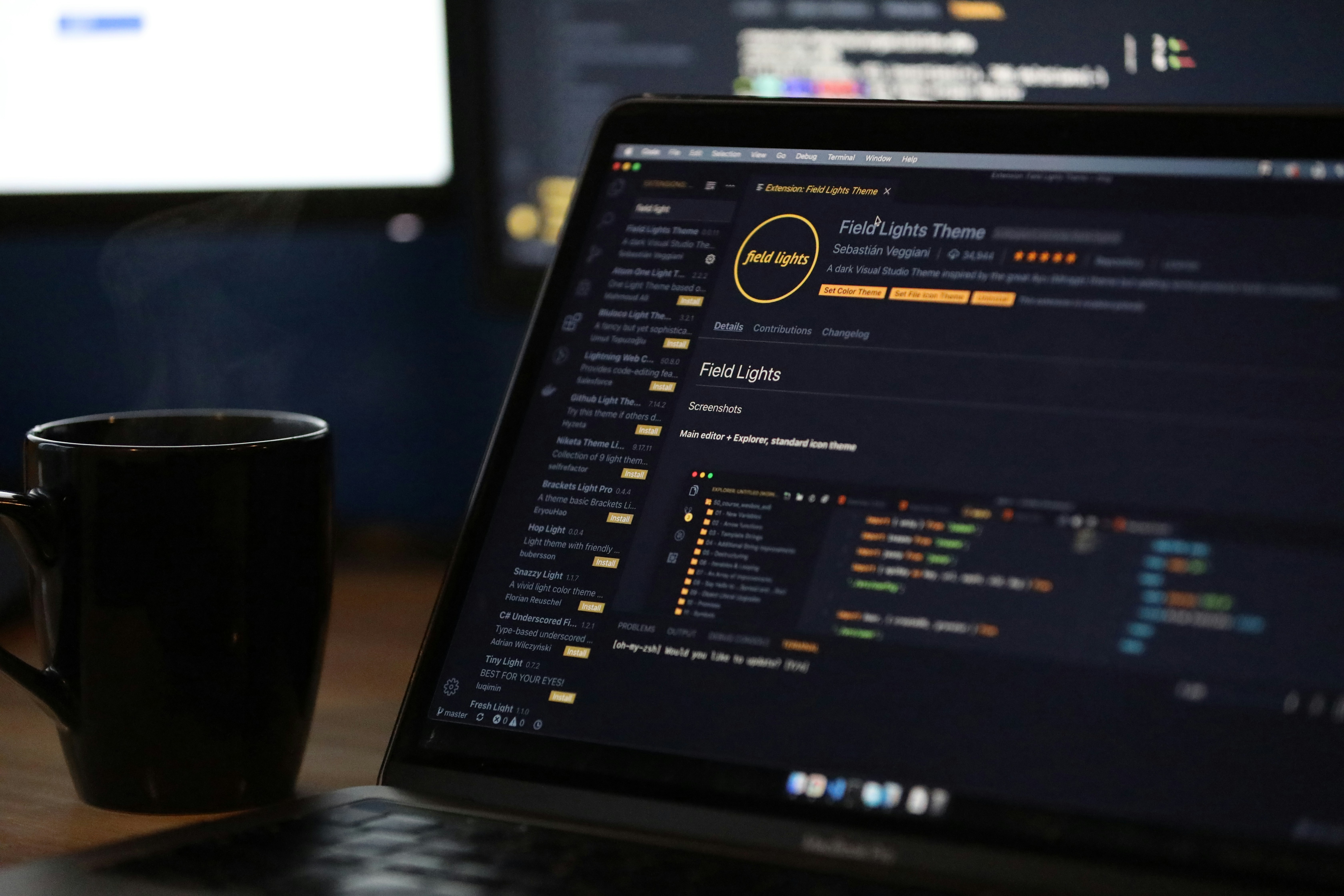
React is the most commonly used Javascript library in 2024 for front-end development. React is so popular that many UI libraries have built-in custom react components to facilitate easy integration. React UI framework provides a wide range of built in React components like buttons, cards, navigation bar, forms, tables and many more.
In this blog, we will figure out top React UI components. We will talk about their features, strengths, and suitability for React projects development.
Top UI Component libraries for React:
Chakra UI
Chakra UI has over 480k weekly downloads and 34.6k stars on GitHub.
To install Chakra UI into our project, we need to run below commands in our terminal:
npm i @chakra-ui/react @emotion/react @emotion/styled framer-motion
After installing Chakra UI into your project, you need to include ChakraProvider in root of your application.
Button components are used to trigger an event or submit for or navigate to another page.
You have to import the button from the chakra package into your React app.
Here’s an example of a button with icon:
import * as React from 'react'
// 1. import `ChakraProvider` component
import { ChakraProvider } from '@chakra-ui/react'
function App() {
// 2. Wrap ChakraProvider at the root of your app
return (
<ChakraProvider>
<Stack direction='row' spacing={4}>
<Button leftIcon={<EmailIcon />} colorScheme='teal' variant='solid'>
Email
</Button>
<Button rightIcon={<ArrowForwardIcon />} colorScheme='teal' variant='outline'>
Call us
</Button>
</Stack>
</ChakraProvider>
)
}
Output
Radix UI
Radix UI has over 13k weekly downloads on npm.
Simple and easy React based UI to customize. You can only install that particular component in your project and customize it. The vision of radix UI to create an open-source library that the community can use to build accessible design systems.
You will install Radix UI primitives for button interaction into your react app.
npm install @radix-ui/react-focus
Radix themes does not come with a built-in styling system. There’s no css
or sx
prop, and it does not use any styling libraries internally. Under the hood, it’s built with vanilla CSS.
Here's an example of Button:
import * as React from 'react';
import { Focus } from '@radix-ui/react-focus';
import { Flex, Button } from '@radix-ui/react-focus'
function MyButton() {
return (
<Focus>
<Flex align="center" gap="3">
<Button variant="classic">Edit profile</Button>
<Button variant="solid">Edit profile</Button>
<Button variant="soft">Edit profile</Button>
<Button variant="surface">Edit profile</Button>
<Button variant="outline">Edit profile</Button>
</Flex>
</Focus>
);
}
Output
Shadcn UI
It’s a collection of reusable components that we can copy and paste code directly into your projects. It is not a component library so you do not have to install it as a dependency. It is not available or distributed via NPM.
Here's an example of Button:
import { Button } from "@/components/ui/button"
export function ButtonSecondary() {
return <Button variant="secondary">Secondary</Button>
}
Ant Design
Ant design has over 1 million weekly downloads on NPM and 87k stars on GitHub
import React from 'react';
import { Button, Flex } from 'antd';
const App: React.FC = () => (
<Flex gap="small" wrap>
<Button type="primary">Primary Button</Button>
<Button>Default Button</Button>
<Button type="dashed">Dashed Button</Button>
<Button type="text">Text Button</Button>
<Button type="link">Link Button</Button>
</Flex>
);
export default App;
Output
React Bootstrap
React bootstrap is one of the popular React UI libraries. It is built on popular bootstrap framework.
React Bootstrap has over 2 million weekly download on NPM and 22k stars of GitHub
Here's an example of using different types of button components in React app.
import Button from 'react-bootstrap/Button';
function ButtonTypes() {
return (
<>
<Button variant="primary">Primary</Button>{' '}
<Button variant="secondary">Secondary</Button>{' '}
<Button variant="success">Success</Button>{' '}
<Button variant="warning">Warning</Button>{' '}
<Button variant="danger">Danger</Button>{' '}
<Button variant="info">Info</Button>{' '}
<Button variant="light">Light</Button>{' '}
<Button variant="dark">Dark</Button>
<Button variant="link">Link</Button>
</>
);
}
export default ButtonTypes;
Output
Material UI
Material UI has over 91.4K GitHub star. It is open source react library that implements Google’s material design.
It includes a comprehensive collection of prebuilt components that are ready for use in production right out of the box, and features a suite of customization options that make it easy to implement your own custom design system on top of your components.
You need to install material UI core libraries and its dependencies.
Here's an example of a button:
npm install @mui/material @emotion/react @emotion/styled
import * as React from 'react';
import Button from '@mui/material/Button';
import Stack from '@mui/material/Stack';
export default function ContainedButtons() {
return (
<Stack direction="row" spacing={2}>
<Button variant="contained">Contained</Button>
<Button variant="contained" disabled>
Disabled
</Button>
<Button variant="contained" href="#contained-buttons">
Link
</Button>
</Stack>
);
}
Output
Conclusion
In this blog, we have seen different React UI libraries with their popularity. Choosing the right UI component library which has good community support will definitely accelerate the development of your project. While deciding, weigh the pros and cons in the context of your project. For example, while one library might excel in customization, another might shine in performance. Each libraries comes with its own development benefits, so the best one for your projects largely depends on your project requirements.
References:
https://prismic.io/blog/react-component-libraries
Subscribe to my newsletter
Read articles from Ankur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
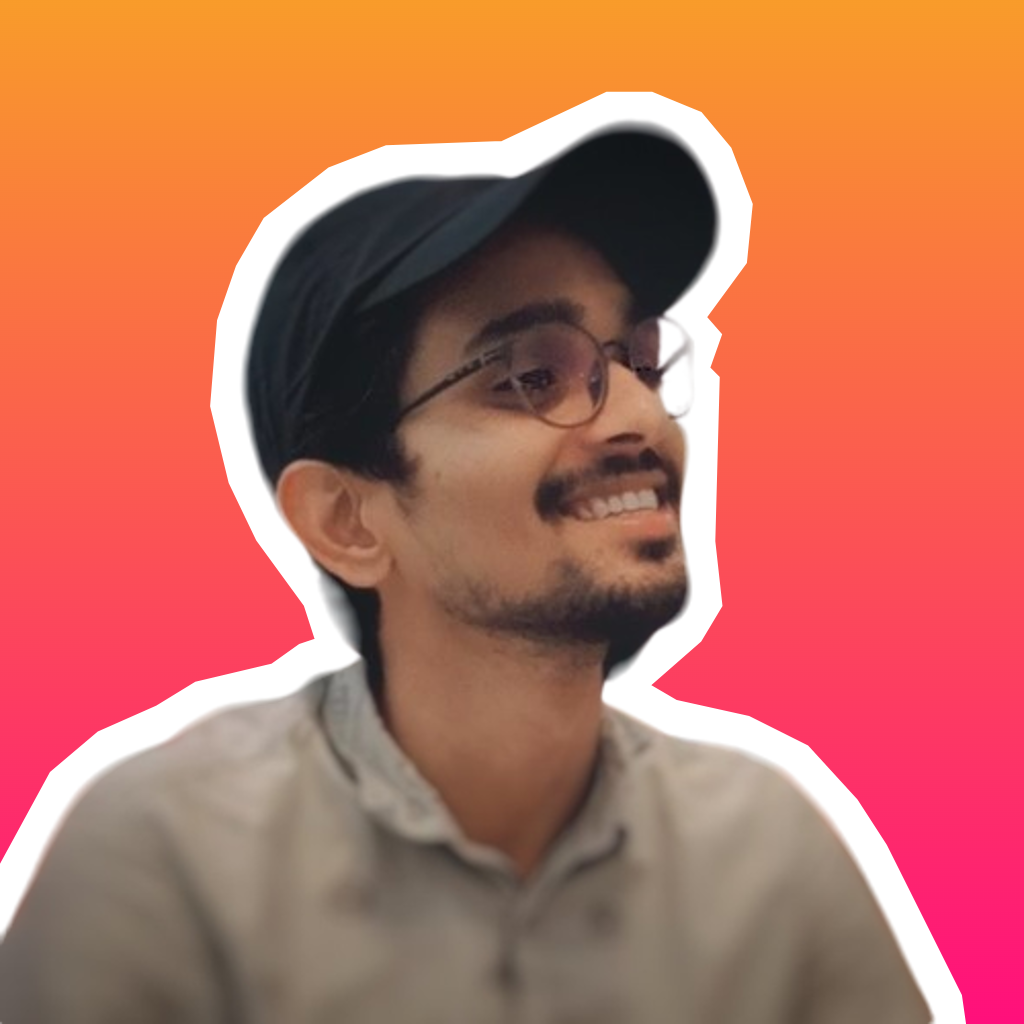