understand The single-threaded event loop💫सिऺगल थेङ🤠↔😎
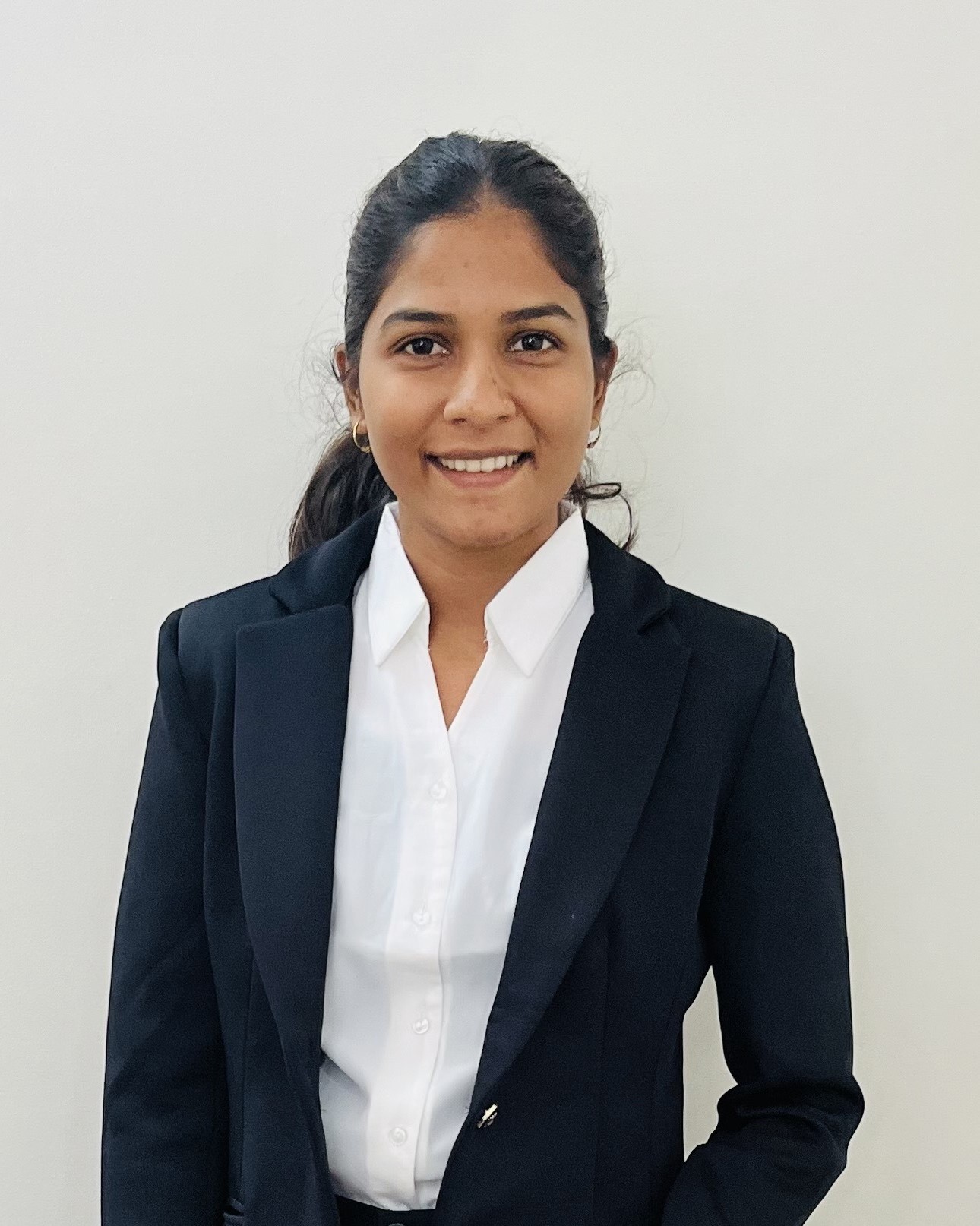
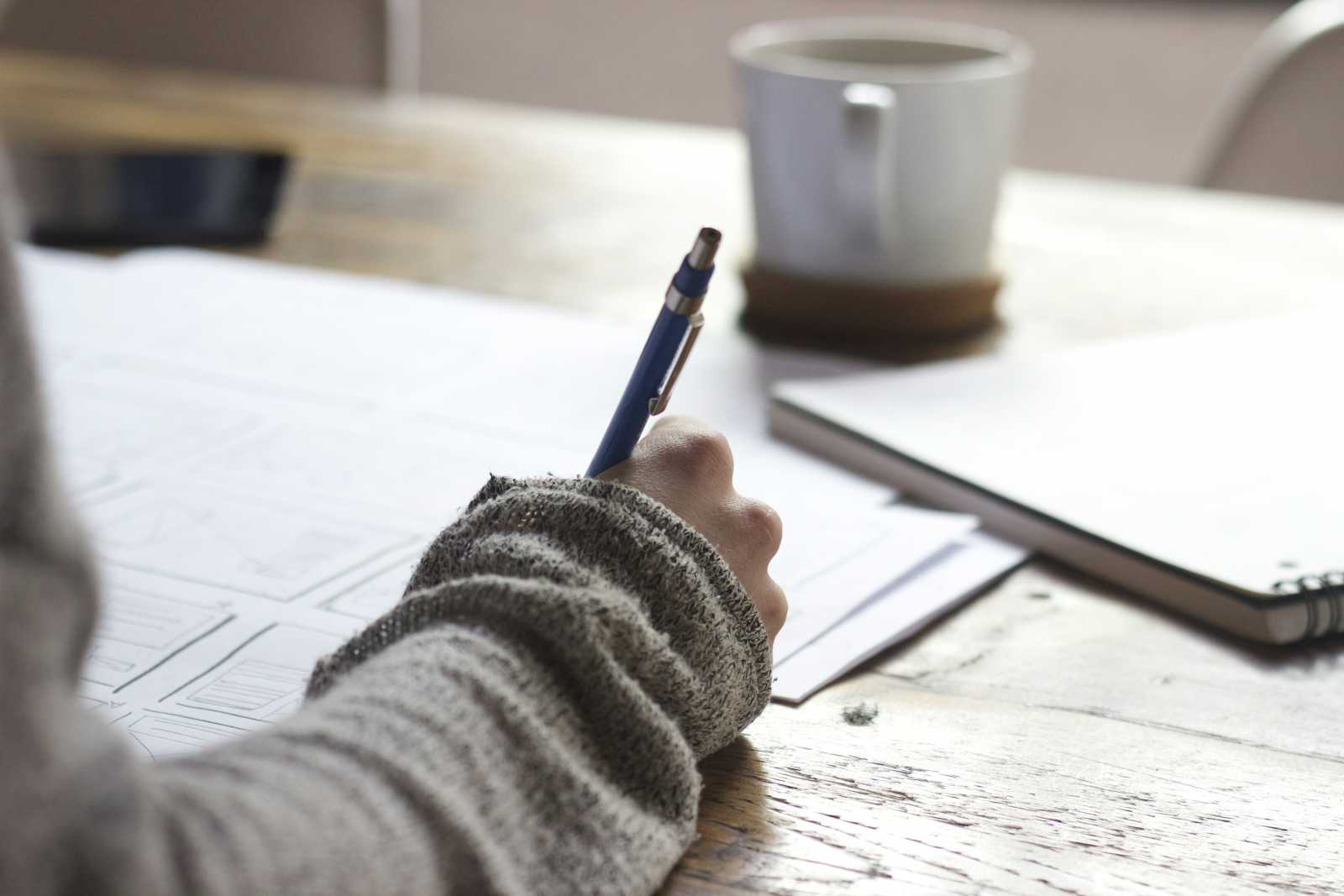
The single-threaded event loop is a core concept in JavaScript, especially relevant for environments like Node.js and browsers.
How It Works:💫
Single-Threaded Nature:
JavaScript executes code on a single thread, meaning it can only perform one task at a time.
Despite being single-threaded, JavaScript handles concurrent operations like I/O, timers, and network requests efficiently using the event loop.
Event Loop Mechanism:
Call Stack: The call stack is where functions are executed. It follows the Last-In-First-Out (LIFO) principle.
Task Queue: When an asynchronous operation (e.g., I/O, timers, promises) completes, its callback function is added to the task queue.
Event Loop: The event loop constantly checks if the call stack is empty. If it is, it pushes the first task from the task queue to the call stack for execution.
Handling Asynchronous Operations:
Callbacks: Traditional asynchronous operations use callbacks that are placed in the task queue after the operation completes.
Promises/Microtasks: Microtasks (e.g., promise callbacks) are handled with higher priority and are placed in a separate queue, which is processed before the next task from the task queue.
Timers: Timers (e.g.,
setTimeout
,setInterval
) also use the event loop to schedule tasks for future execution.
Non-Blocking I/O:
- The event loop allows JavaScript to handle I/O operations without blocking the main thread, enabling efficient handling of multiple tasks concurrently.
Example Code:💟
console.log('1: Start');
setTimeout(() => {
console.log('2: Timeout callback');
}, 0);
Promise.resolve().then(() => {
console.log('3: Promise callback');
});
console.log('4: End');
Step-by-Step Explanation:☢
Synchronous Code Execution:
The code starts executing synchronously from the top.
console.log('1: Start')
runs first and prints "1: Start".
setTimeout:
setTimeout(() => { console.log('2: Timeout callback'); }, 0);
schedules a task to run after 0 milliseconds.The callback function is added to the task queue to be executed later.
Promise:
Promise.resolve().then(() => { console.log('3: Promise callback'); });
schedules a microtask (thethen
callback) to run once the current code execution completes.Microtasks are added to the microtask queue.
Synchronous Code Continues:
console.log('4: End')
runs and prints "4: End".
Event Loop Checks:
After the synchronous code finishes, the call stack is empty.
The event loop checks the microtask queue first.
The promise callback
console.log('3: Promise callback')
runs, printing "3: Promise callback".
Task Queue Execution:
After all microtasks are processed, the event loop moves to the task queue.
The
setTimeout
callback runs, printing "2: Timeout callback".
Final Output:✅
1: Start
4: End
3: Promise callback
2: Timeout callback
Key Takeaways:
The synchronous code (
console.log
statements) runs first.The microtask queue (promises) is processed before the task queue (setTimeout).
Even with a
0ms
delay, thesetTimeout
callback is processed after the promise callback because of the event loop's prioritization of microtasks.
Why It’s Important:✔✔
- The single-threaded event loop is what allows JavaScript to be asynchronous without multithreading. It manages concurrency efficiently, making it ideal for web applications and server-side applications where non-blocking I/O is crucial.
This design helps JavaScript maintain high performance in environments where it needs to handle many tasks at once, such as web servers handling multiple requests or web browsers managing user interactions, DOM updates, and network requests simultaneously.
Subscribe to my newsletter
Read articles from priyanka chaudhari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
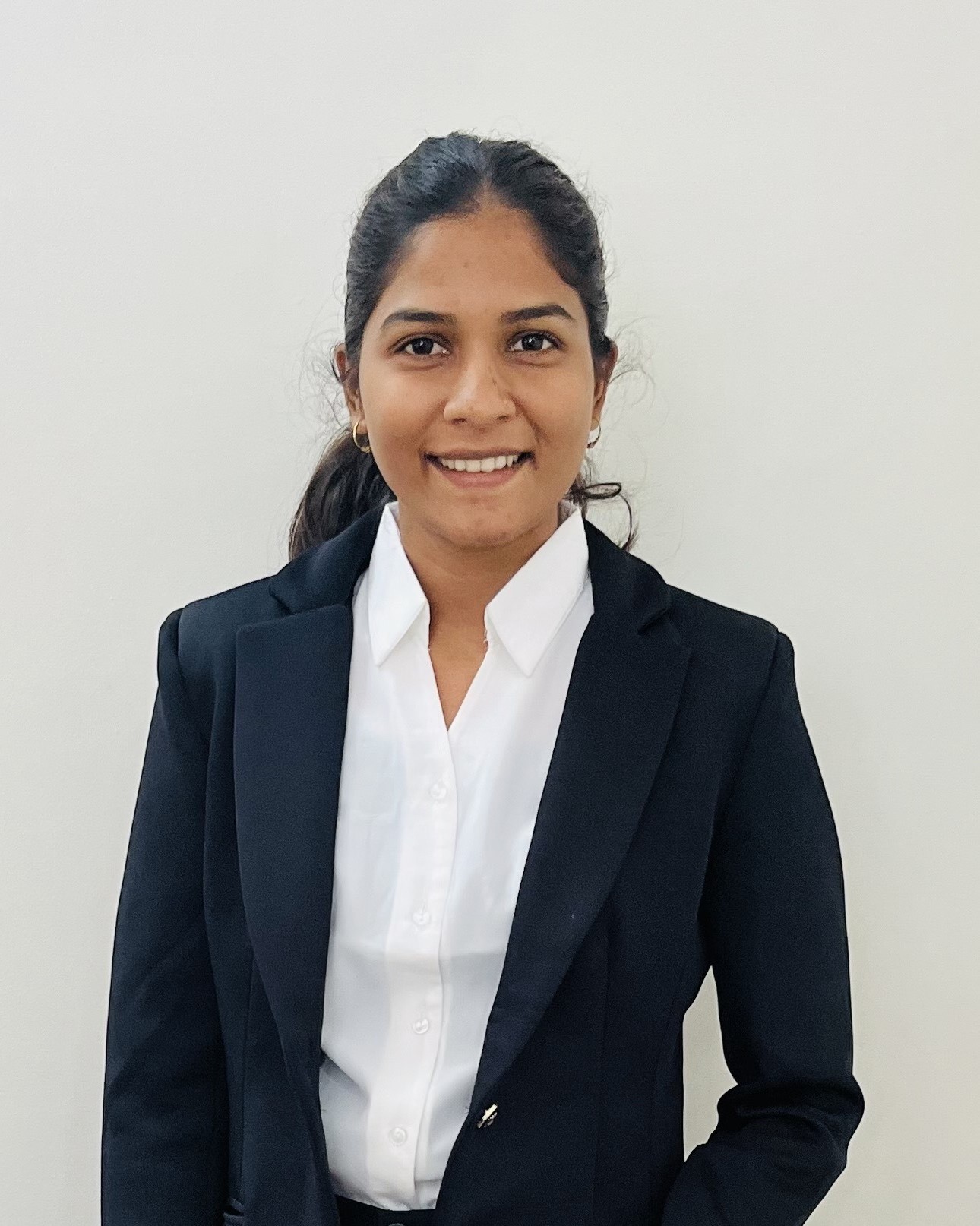
priyanka chaudhari
priyanka chaudhari
i am a full stack web developer and i write code....