Props(Properties):

2 min read
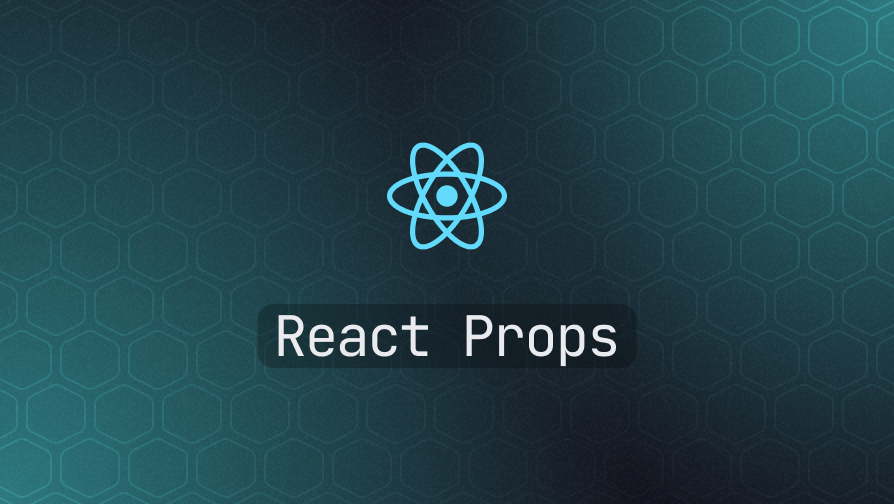
What are Props?
props are inbuilt object.
Props are used to send the data from parent components to child components.
props are immutable it means once the value is passed from parent component it can't be changed.
props are uni-directional.
Q. Write a program to send a string data type to a props:
App.jsx:
import Child from "./component/Child"
const App=()=>{
return(
<div>
<Child data="Hello"/>
</div>
)}
export default App
Child.jsx (child component):
const Child=(x)=>{
console.log(x);
return(
<div>
<h1>{x.data}</h1>
</div>
)}
export default Child
Q. WAP to send and array data Type as a props?
App.jsx:
import Child from "./component/Child"
const App=()=>{
return(
<div>
<Child data={["Hi","Hello","Bye"]}/>
</div>
)}
export default App
Child.jsx (Child component):
const Child=(x)=>{
console.log(x);
return(
<div>
{x.data[0]} //hi
{x.data[1]} //Hello
{x.data[2]} //bye
</div>
)}
export default Child
Q. Write a program to send as object data type as a props?
App.jsx:
import Child from "./component/Child"
const App=()=>{
let obj={name:"rocky", id:123}
return(
<div>
<Child data={obj}/>
</div>
)}
export default App
Child.jsx:
const Child=(x)=>{
console.log(x); //object
return(
<div>
{x.data.name} //"Rocky"
{x.data.id} //"123"
</div>
)}
export default Child
Props Drilling:
It is a process by which you can pass the data from one part of the react component tree to another by going through other parts that do not need the data but only help in passing it around.
Example:
App.jsx (Parent component)
import A from "./component/A"
const App=()=>{
return(
<div>
<A data="Hi"/>
</div>
)}
export default App
A.jsx(child component)
import B from "./component/B"
const A=(x)=>{
return(
<div>
<B data1={x.data}/>
</div>
)}
export default A
B.jsx (Grand Child Component):
const B=(x)=>{
return(
<div>
<h1>{x.data}Good morning</h1>
</div>
)}
export default B
0
Subscribe to my newsletter
Read articles from Nitin Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
