Java Class Structure: Unlocking the Secrets of Static and Instance Components
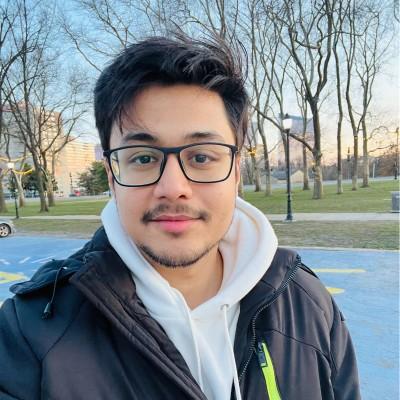
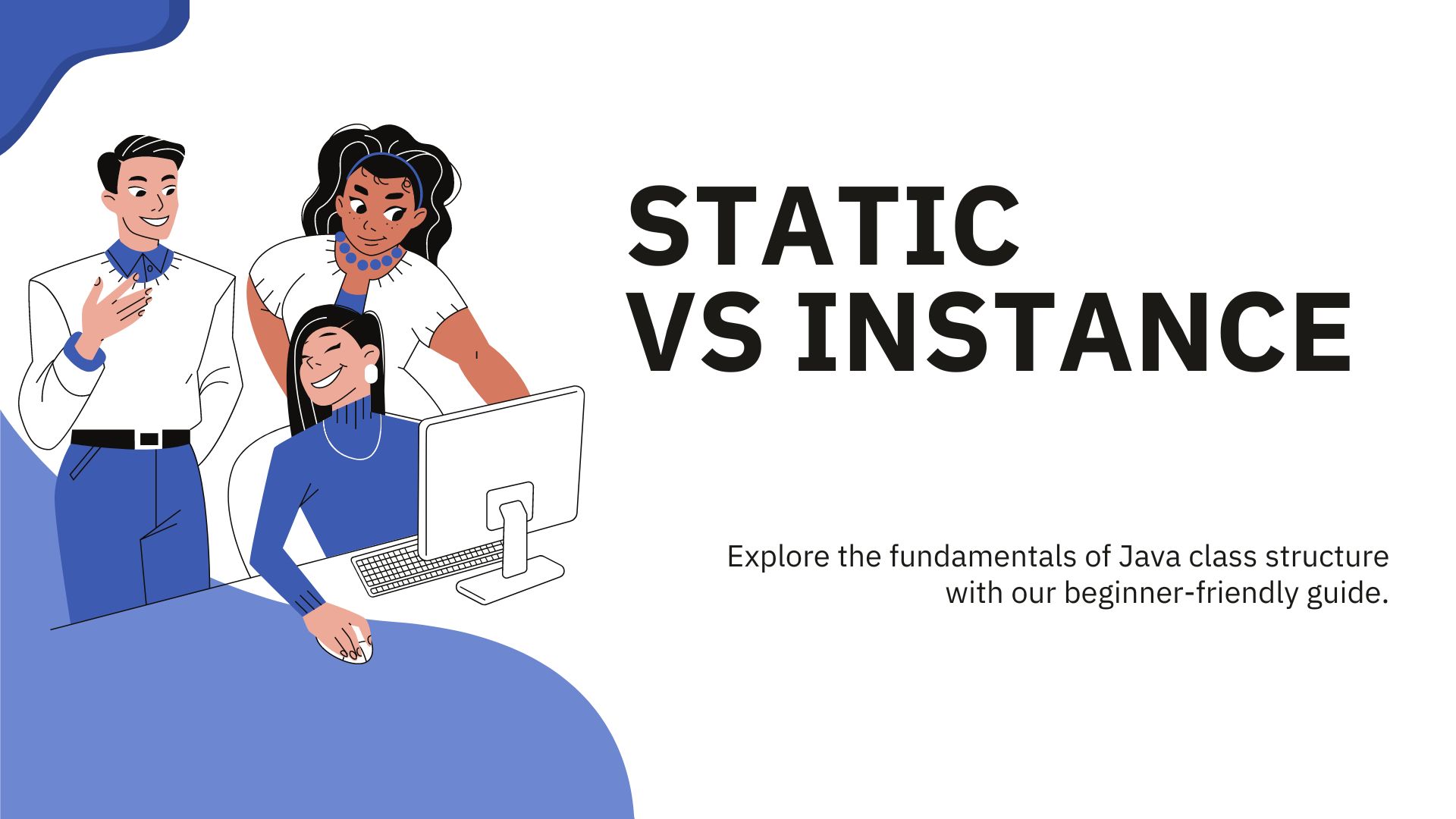
In Java, understanding the various components of a class structure is fundamental to mastering the language. Here, we'll break down a sample class, ClassStructure, to explore static variables, instance variables, static blocks, instance blocks, constructors, and methods.
/**
* ClassStructure
*/
public class ClassStructure {
// static variable
static int count;
// instance variable
int id;
// static block
// runs once when the class is loaded into memory for the first time
static {
count = 0;
}
// constructor
ClassStructure() {
// increment the static counter and assign it to the instance variable
id = ++count;
}
// static method
static void printCount() {
System.out.println("Total objects created: " + count);
}
// instance method
void printId() {
System.out.println("Object ID: " + id);
}
public static void main(String[] args) {
ClassStructure obj1 = new ClassStructure();
ClassStructure obj2 = new ClassStructure();
ClassStructure obj3 = new ClassStructure();
obj1.printId(); // Output: Object ID: 1
obj2.printId(); // Output: Object ID: 2
obj3.printId(); // Output: Object ID: 3
ClassStructure.printCount(); // Output: Total objects created: 3
}
}
Explanation:
1. Static Variable (count
)
What it is:
A static variable belongs to the class, not to any specific object.
It’s shared among all instances of the class.
Example in our class:
static int count;
- This means there is one
count
variable for the wholeClassStructure
class.
2. Instance Variable (id
)
What it is:
An instance variable belongs to an instance of the class (an object).
Each object has its own copy of the instance variable.
Example in our class:
int id;
- Each
ClassStructure
object will have its ownid
.
3. Static Block
What it is:
A static block is used to initialize static variables.
It runs once when the class is first loaded into memory.
Example in our class:
static {
count = 0;
}
- This sets the
count
variable to0
when the class is loaded.
4. Constructor
What it is:
A constructor is a special method that runs when you create a new object.
It is used to initialize instance variables.
Example in our class:
ClassStructure() {
id = ++count;
}
- Every time a new
ClassStructure
object is created, thecount
variable is incremented by 1, and theid
of the object is set to the new value ofcount
.
5. Static Method (printCount
)
What it is:
A static method belongs to the class, not to any specific object.
It can be called using the class name without creating an object.
Example in our class:
static void printCount() {
System.out.println("Total objects created: " + count);
}
- This static method prints the total number of objects created.
6. Instance Method (printId
)
What it is:
An instance method belongs to an object.
It can use instance variables.
Example in our class:
void printId() {
System.out.println("Object ID: " + id);
}
- This instance method prints the
id
of the object.
7. Main Method
What it is:
- The
main
method is the entry point of the program. It runs when you execute the program.
Example in our class:
public static void main(String[] args) {
ClassStructure obj1 = new ClassStructure();
ClassStructure obj2 = new ClassStructure();
ClassStructure obj3 = new ClassStructure();
obj1.printId(); // Output: Object ID: 1
obj2.printId(); // Output: Object ID: 2
obj3.printId(); // Output: Object ID: 3
ClassStructure.printCount(); // Output: Total objects created: 3
}
This creates three
ClassStructure
objects (obj1
,obj2
,obj3
).It calls the
printId
method on each object to print their unique IDs.It calls the
printCount
method to print the total number of objects created.
Summary:
Static variables are shared among all instances of the class.
Instance variables are unique to each object.
Static blocks initialize static variables once when the class is loaded.
Constructors initialize instance variables each time a new object is created.
The main method is the entry point of the program.
Static methods belong to the class and can be called without creating an object.
Instance methods belong to objects and can use instance variables.
This example helps in understanding how each component works and interacts within a Java class. By incrementing the id
in the constructor, each new object gets a unique id
, demonstrating practical use of static and instance variables. Happy coding!
Subscribe to my newsletter
Read articles from Pratik Upreti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
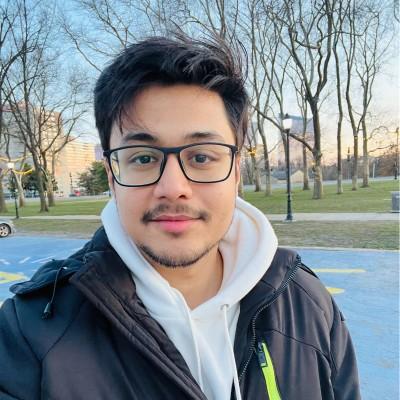
Pratik Upreti
Pratik Upreti
Hello! I’m Pratik Upreti, a seasoned software engineer with a rich background in languages like C#, Java, JavaScript, Python, SQL, and MySQL, I bring a diverse skill set to the table. I excel in backend and server technologies, including Node.js, Django, GraphQL, and Prisma ORM, and am adept in frontend development with Next.js, React.js and Tailwind CSS. Professionally, contributing at Brilliant Infotech.