Behaviour of constructors in Testng
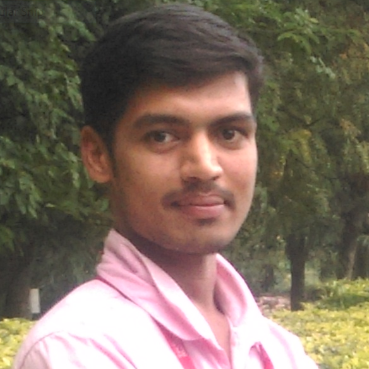
In TestNG, the behavior of constructors in relation to test groups and packages can sometimes be confusing. Here’s a breakdown of what you need to know about how constructors interact with groups and packages, and some best practices to ensure they work as expected:
Constructors and TestNG Groups
Constructor Execution: In TestNG, constructors of test classes are executed before any test methods, regardless of the groupings specified. This means that if you have a constructor in a test class, it will run before any tests in that class, regardless of which groups the tests belong to.
Group Execution: When you specify groups in TestNG, you are essentially filtering which tests should be executed based on the groupings. However, TestNG still instantiates all classes and runs their constructors if they are part of the test suite or the specified configuration.
Packages and TestNG
Package Scanning: If you use TestNG’s package scanning (e.g., through
testng.xml
or annotations), TestNG will discover and create instances of all test classes in the specified packages. Consequently, the constructors of these classes will be executed.Class Filtering: While TestNG can filter which tests to run based on groups or methods, it does not filter out the class instantiation phase. This means all classes are instantiated and their constructors run before any actual test methods are executed.
Managing Constructor Execution
To handle constructor execution effectively while using groups and packages:
Minimize Constructor Logic: Keep constructors simple and avoid placing complex setup logic in them. Ideally, constructors should be used for basic initialization that is independent of the test logic.
Use
@BeforeClass
or@BeforeMethod
: Move setup and initialization code to@BeforeClass
or@BeforeMethod
. This provides better control over when setup code is run and allows you to align it with specific test groups or methods.public class MyTest { @BeforeClass public void setUp() { // Initialization code that runs before any tests in this class } @Test(groups = { "group1" }) public void testInGroup1() { // Test code for group1 } @Test(groups = { "group2" }) public void testInGroup2() { // Test code for group2 } }
Conditional Initialization: If you need different setups for different groups, you can use conditional logic inside
@BeforeClass
or@BeforeMethod
to handle initialization based on the group or method being executed.@BeforeMethod public void setUp(Method method) { if (method.getAnnotation(Test.class).groups().length > 0) { // Perform setup based on the group } }
Example Configuration
Consider the following testng.xml
configuration for running specific groups:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Suite" verbose="1">
<test name="Test">
<groups>
<run>
<include name="group1"/>
</run>
</groups>
<classes>
<class name="com.example.MyTest"/>
</classes>
</test>
</suite>
In this configuration:
Class Instantiation: All classes under the
<classes>
tag will be instantiated, and their constructors will run.Group Filtering: Only tests belonging to the specified group (
group1
in this case) will be executed.
Conclusion
While TestNG groups and packages help in filtering and organizing tests, constructors of test classes are always executed before any test methods. To manage this effectively, keep constructor logic minimal and utilize @BeforeClass
, @BeforeMethod
, or conditional logic to handle more complex setup needs. This approach will help ensure that your test environment is correctly set up without unintended interference.
Subscribe to my newsletter
Read articles from Shyam Kunda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
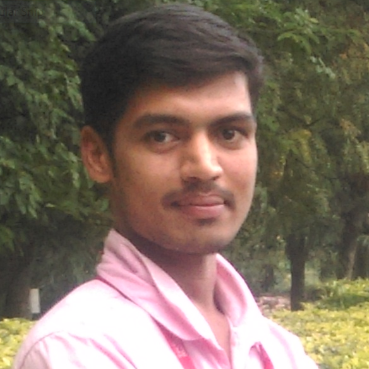