๐ TypeScript 5.5: Unlocking the Power of Inferred Type Predicates
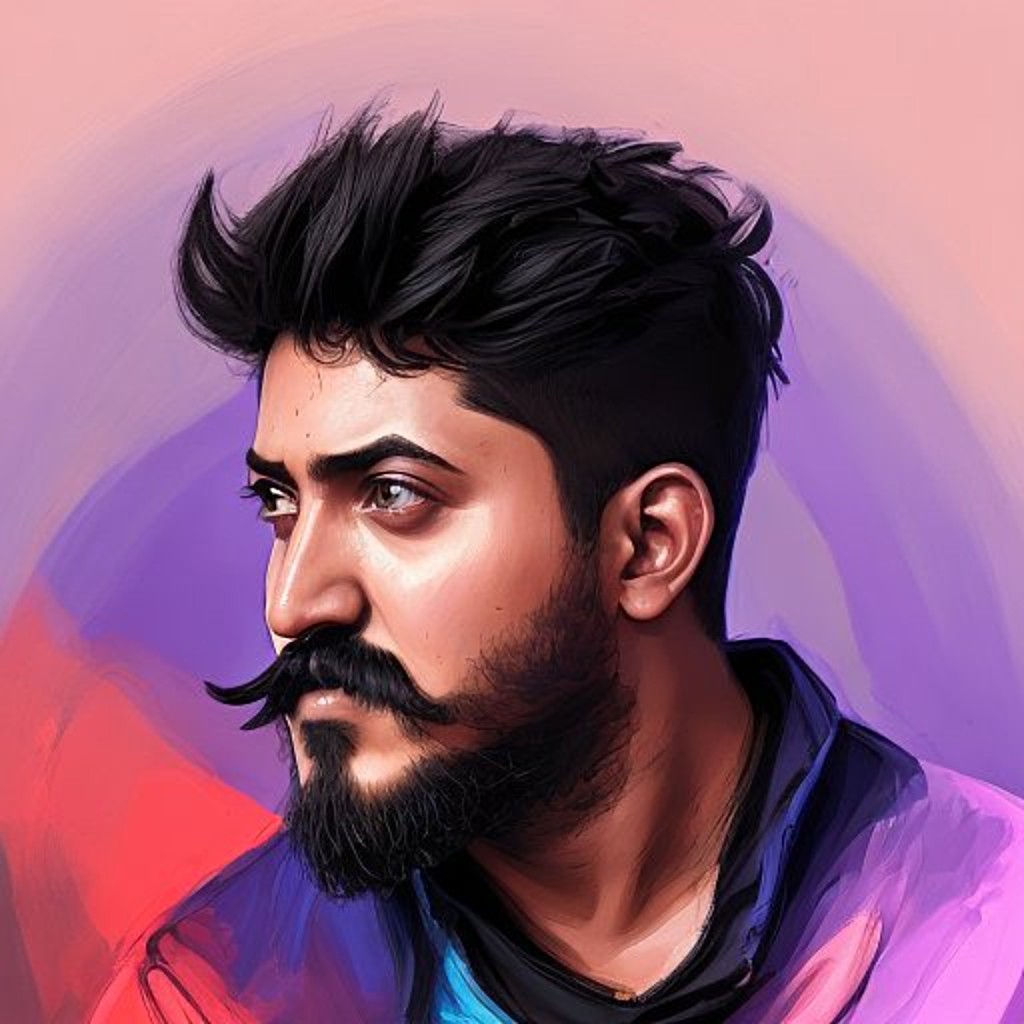
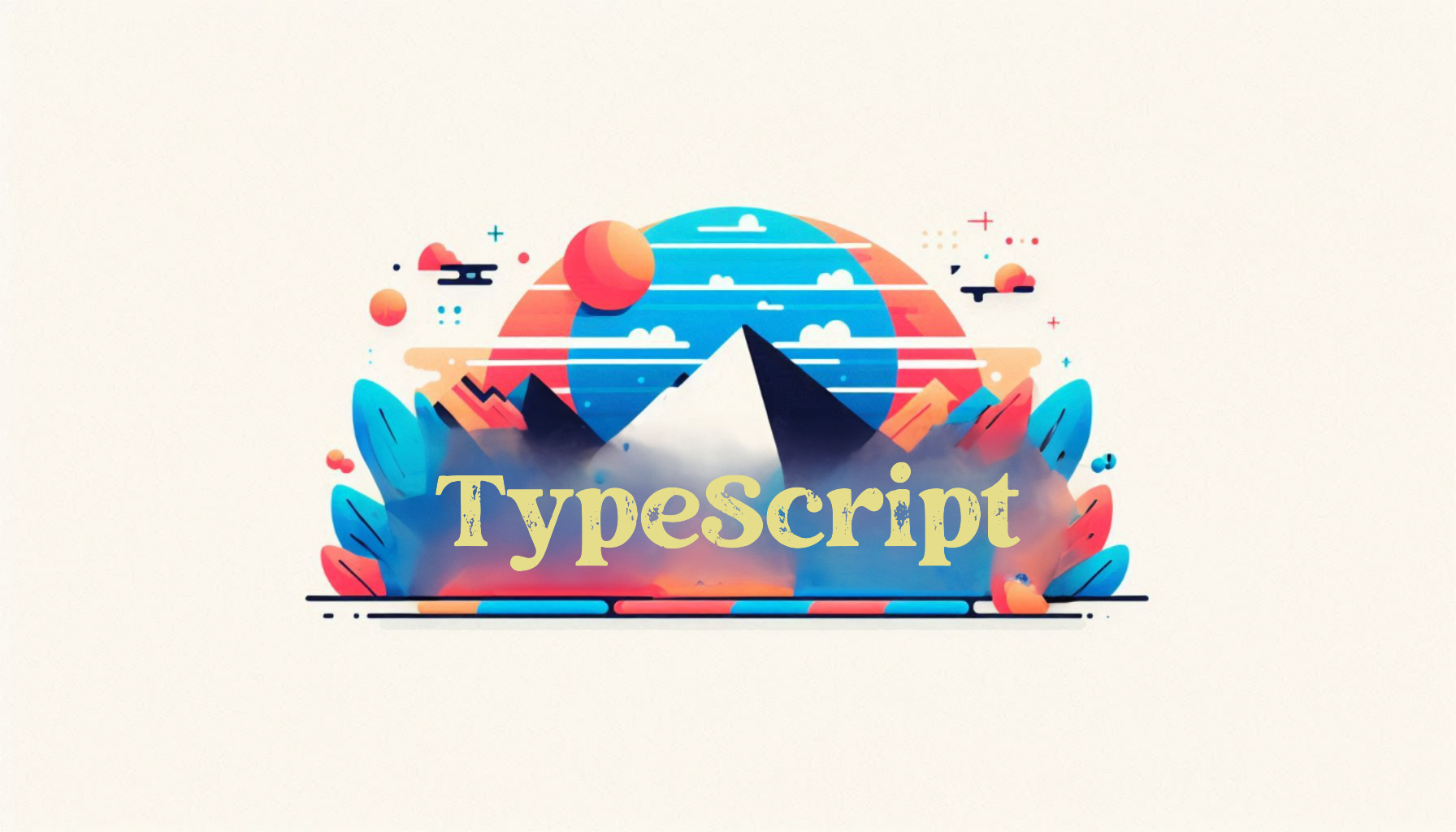
Why Inferred Type Predicates Are a Game Changer ๐
TypeScript 5.5 brings a host of new features, but one of the most exciting is the ability to use inferred type predicates. This powerful feature can simplify your code, reduce boilerplate, and improve type safety, all while making your development experience smoother and more enjoyable. Let's dive into what this means and see it in action with a practical example.
Explicit Type Guards ๐
Traditional Method: Verbose and Explicit
When working with union types, we often need to define explicit type guard functions to help TypeScript narrow down the types. While effective, this approach can be a bit verbose and requires additional boilerplate code.
interface Book {
title: string;
author: string;
pages: number;
read(): void;
}
interface DVD {
title: string;
director: string;
duration: number;
play(): void;
}
// Union type for media items
type Media = Book | DVD;
// Function to check if a media item is a Book
function isBook(media: Media): media is Book {
return (media as Book).author !== undefined;
}
// Function to check if a media item is a DVD
function isDVD(media: Media): media is DVD {
return (media as DVD).director !== undefined;
}
// Function to process media items and perform actions based on their type
function processMediaItems(mediaItems: Media[]) {
for (const media of mediaItems) {
if (isBook(media)) {
// TypeScript knows media is a Book here because of the explicit type guard function isBook
media.read();
} else if (isDVD(media)) {
// TypeScript knows media is a DVD here because of the explicit type guard function isDVD
media.play();
} else {
console.log('Unknown media type');
}
}
}
// Example data
const library: Media[] = [
{ title: "1984", author: "George Orwell", pages: 328, read() { console.log("Reading 1984"); } },
{ title: "Inception", director: "Christopher Nolan", duration: 148, play() { console.log("Playing Inception"); } },
{ title: "The Hobbit", author: "J.R.R. Tolkien", pages: 310, read() { console.log("Reading The Hobbit"); } }
];
// Process the example data
processMediaItems(library);
Pros:
Clear and explicit type checking
Reliable type safety
Cons:
Verbose and requires additional type guard functions
More boilerplate code
Inferred Type Predicates ๐
New Method: Simple and Elegant
With TypeScript 5.5, inferred type predicates let TypeScript automatically deduce the types based on properties, reducing the need for explicit type guard functions. This makes your code cleaner and more maintainable.
interface Book {
title: string;
author: string;
pages: number;
read(): void;
}
interface DVD {
title: string;
director: string;
duration: number;
play(): void;
}
// Union type for media items
type Media = Book | DVD;
// Library holding various media items
const library: Media[] = [
{ title: "1984", author: "George Orwell", pages: 328, read() { console.log("Reading 1984"); } },
{ title: "Inception", director: "Christopher Nolan", duration: 148, play() { console.log("Playing Inception"); } },
{ title: "The Hobbit", author: "J.R.R. Tolkien", pages: 310, read() { console.log("Reading The Hobbit"); } }
];
// Function to process media items and perform actions based on their type
function processMediaItems(mediaItems: Media[]) {
for (const media of mediaItems) {
if ('author' in media) {
// TypeScript infers media is a Book here
media.read();
} else if ('director' in media) {
// TypeScript infers media is a DVD here
media.play();
} else {
console.log('Unknown media type');
}
}
}
// Process the example data
processMediaItems(library);
Pros:
Cleaner and more readable code
Less boilerplate and no need for extra type guard functions
Leverages TypeScript's powerful type inference
Cons:
- May require familiarity with inferred types and their nuances
Conclusion ๐
Inferred type predicates in TypeScript 5.5 streamline your code, making it more concise and maintainable. By reducing the need for explicit type guard functions, you can focus more on your application's logic and less on boilerplate code. Embrace these new features and enhance your TypeScript development experience!
Ready to Simplify Your Code?
Try out inferred type predicates in your next TypeScript project and see the difference! ๐
This article covers the advantages of inferred type predicates over explicit type guards. For more on the latest TypeScript features, check out the official TypeScript documentation.
Subscribe to my newsletter
Read articles from Jobin Mathew directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
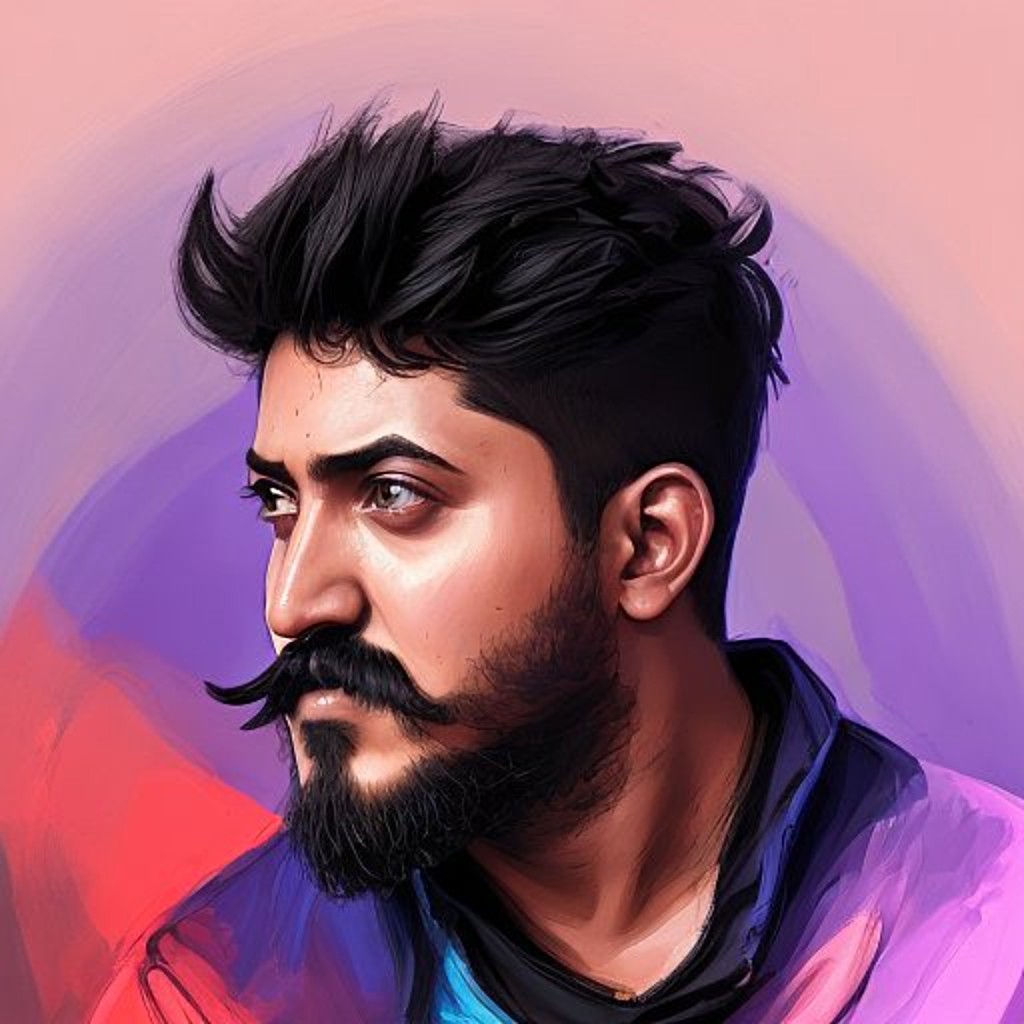
Jobin Mathew
Jobin Mathew
Hey there! I'm Jobin Mathew, a passionate software developer with a love for Node.js, AWS, SQL, and NoSQL databases. When I'm not working on exciting projects, you can find me exploring the latest in tech or sharing my coding adventures on my blog. Join me as I decode the world of software development, one line of code at a time!