Advanced Animation Techniques in Flutter: Motion, Staggered Animations, and More
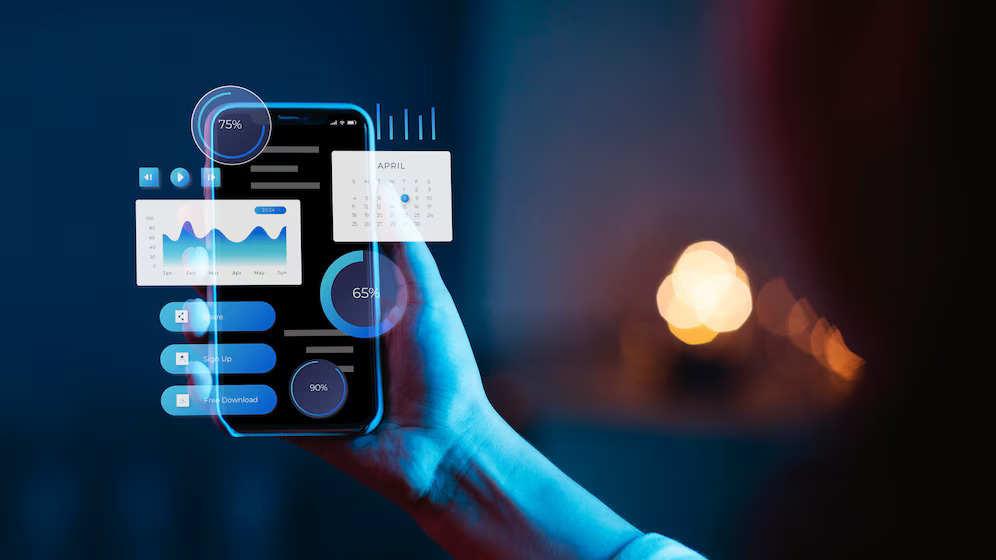
Flutter, Google's UI toolkit for building natively compiled applications, is well-known for its ability to create stunning and fluid animations. While basic animations can bring a UI to life, advanced animation techniques can elevate the user experience to a whole new level.
In this blog post, we'll explore some advanced animation techniques in Flutter, including motion animations, staggered animations, and more. If you're looking to create exceptional apps, consider hiring Flutter app developers to bring your vision to life.
1. Understanding the Basics of Animations in Flutter
Before diving into advanced techniques, it's essential to understand the basics of animations in Flutter. At its core, Flutter's animation system revolves around the Animation
and AnimationController
classes. An AnimationController
manages the animation's duration and playback, while an Animation
object defines the transition from one value to another over time.
Example: Simple Fade Animation
class FadeAnimation extends StatefulWidget
{ @override
_FadeAnimationState createState() => _FadeAnimationState();
}
class _FadeAnimationState extends State
with SingleTickerProviderStateMixin {
AnimationController _controller;
Animation _animation;
@override void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this, );
_animation = CurvedAnimation(parent: _controller, curve: Curves.easeIn);
_controller.forward();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override Widget build(BuildContext context)
{
return FadeTransition(
opacity: _animation,
child: const Text('Hello Flutter!'),
);
}
}
2. Motion Animations
Motion animations refer to animations that create the illusion of movement. These can range from simple slide-ins to complex transitions involving multiple elements. Flutter's Transform
widget and AnimatedBuilder
are powerful tools for creating motion animations.
Example: Slide Animation
class SlideAnimation extends StatelessWidget {
final Animation animation;
SlideAnimation({required this.animation});
@override
Widget build(BuildContext context) {
return SlideTransition(
position: animation, child: const Text('Sliding Text'),
);
}
}
To create a smooth slide-in effect, you can use the Tween
class to define the start and end positions of the animation.
3. Staggered Animations
Staggered animations involve multiple elements being animated in a sequence, creating a cascading effect. This can be achieved using multiple Animation
objects and AnimationController
s or by using the Interval
class to define the timing of each animation.
Example: Staggered List Animation
class StaggeredListAnimation extends StatefulWidget
{
@override
_StaggeredListAnimationState createState() => _StaggeredListAnimationState();
}
class _StaggeredListAnimationState extends State with TickerProviderStateMixin { late final List _controllers; late final List<Animation> _animations;
@override
void initState()
{ super.initState();
_controllers = List.generate(5, (index) {
return AnimationController(
duration: const Duration(milliseconds: 500),
vsync: this, );
});
_animations = _controllers.map((controller) {
return CurvedAnimation(parent: controller, curve: Curves.easeIn);
}).toList();
for (var i = 0; i < _controllers.length; i++) {
Future.delayed(Duration(milliseconds: i * 200), () {
_controllers[i].forward(); }); } }
@override
void dispose() {
for (var controller in _controllers) {
controller.dispose(); } super.dispose(); }
@override Widget build(BuildContext context) {
return Column( children: _animations.map((animation) {
return FadeTransition(opacity: animation, child: const Text('Item'));
}).toList(),
);
}
}
4. Advanced Techniques: Physics-Based Animations
Physics-based animations add a layer of realism by mimicking natural movements. Flutter provides the PhysicsSimulation
class and the SpringSimulation
class for creating physics-based animations.
Example: Spring Animation
class SpringAnimationWidget extends StatefulWidget {
@override
_SpringAnimationWidgetState createState() => _SpringAnimationWidgetState();
}
class _SpringAnimationWidgetState extends State<SpringAnimationWidget>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(
vsync: this,
duration: const Duration(milliseconds: 300),
);
}
void _runAnimation() {
_controller.animateWith(SpringSimulation(
SpringDescription(mass: 1, stiffness: 100, damping: 5),
0,
1,
0,
));
}
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: _runAnimation,
child: AnimatedBuilder(
animation: _controller,
builder: (context, child) {
return Transform.scale(
scale: 1 + _controller.value,
child: child,
);
},
child: const Icon(Icons.favorite, size: 100),
),
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
}
5. Combining Animations
Combining different animations can create complex and visually appealing effects. For instance, you can combine a fade animation with a slide animation to create a fade-in slide effect. This can be achieved by nesting different animation widgets or using the AnimatedBuilder
for more control.
Conclusion
Advanced animation techniques in Flutter allow developers to create sophisticated and engaging user experiences. From motion animations to staggered and physics-based animations, Flutter provides the tools needed to bring any design to life. By understanding and experimenting with these techniques, you can create applications that are not only functional but also visually stunning.
For businesses seeking to elevate their digital presence, leveraging Flutter app development services can be a game-changer. Whether you're a seasoned developer or just starting, don't be afraid to experiment with different animation techniques. The possibilities are endless, and with Flutter's robust animation system, you can achieve almost anything you can imagine.
Related Post
Subscribe to my newsletter
Read articles from SolGuruz LLP - Web and App Development Company directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
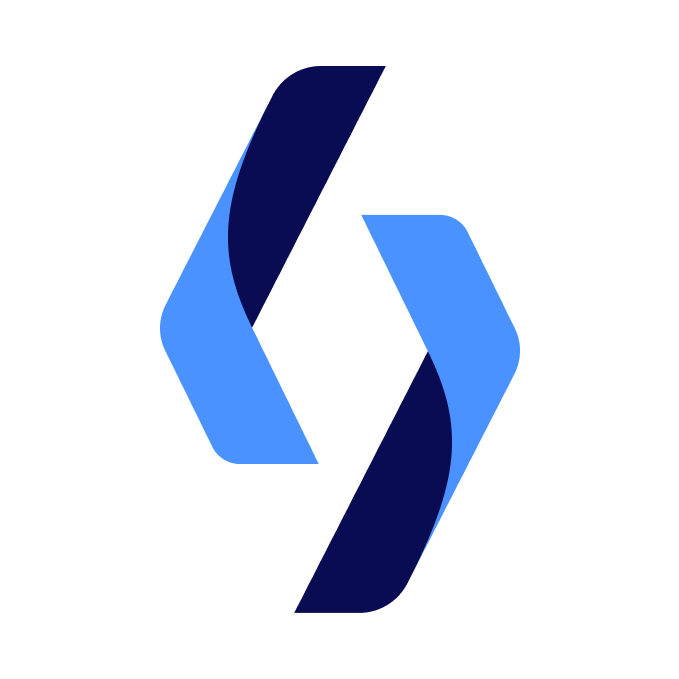
SolGuruz LLP - Web and App Development Company
SolGuruz LLP - Web and App Development Company
SolGuruz® is a custom software development company building modern, secure, and scalable solutions. our services include web application, mobile app development, and solution modernization. We work closely with clients to understand their needs and deliver software that drives business growth.