๐ Introducing New ECMAScript Set Methods in TypeScript 5.5
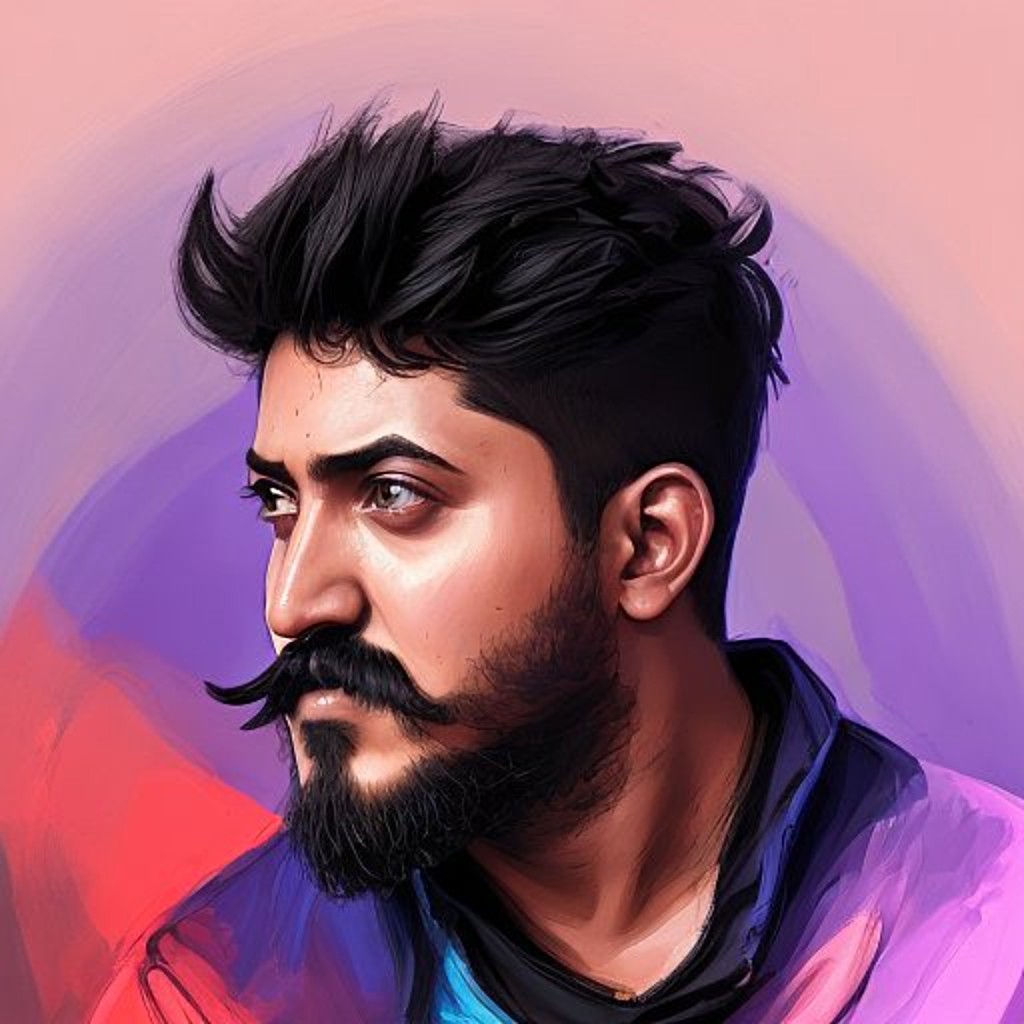
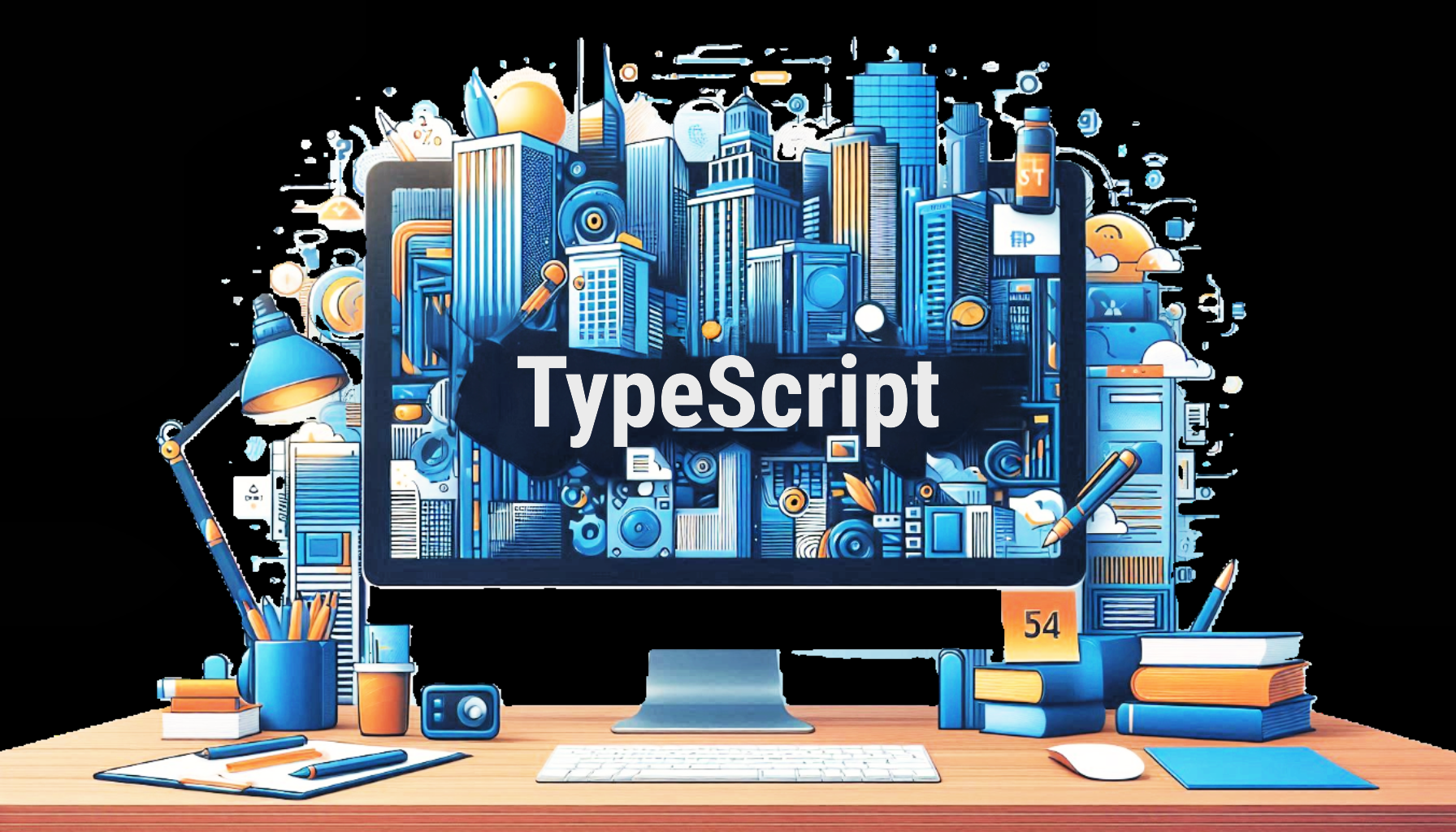
One of the standout features of TypeScript 5.5 is the New ECMAScript Set Methods. This feature introduces powerful new methods to the Set
object, aligning TypeScript with the latest ECMAScript standards and making set operations more intuitive and efficient.
Why New Set Methods Matter ๐
Sets are a fundamental part of JavaScript, providing a collection of unique values. While sets are incredibly useful, performing common operations like union, intersection, and difference required custom implementations. TypeScript 5.5 changes the game by introducing native methods for these operations, making your code cleaner and more readable.
What Are the New Set Methods?
TypeScript 5.5 introduces three new methods for Set
objects:
union
: Combines two sets, returning a new set with all unique elements from both.intersection
: Returns a new set with only the elements that are present in both sets.difference
: Returns a new set with elements from the first set that are not present in the second set.
Example Without New Set Methods ๐ซ
Before TypeScript 5.5, performing set operations required custom code:
const setA = new Set([1, 2, 3]);
const setB = new Set([3, 4, 5]);
const union = new Set([...setA, ...setB]);
const intersection = new Set([...setA].filter(x => setB.has(x)));
const difference = new Set([...setA].filter(x => !setB.has(x)));
console.log(union); // Set {1, 2, 3, 4, 5}
console.log(intersection); // Set {3}
console.log(difference); // Set {1, 2}
Example With New Set Methods ๐
With TypeScript 5.5, these operations become straightforward:
const setA = new Set([1, 2, 3]);
const setB = new Set([3, 4, 5]);
const union = setA.union(setB);
const intersection = setA.intersection(setB);
const difference = setA.difference(setB);
console.log(union); // Set {1, 2, 3, 4, 5}
console.log(intersection); // Set {3}
console.log(difference); // Set {1, 2}
Benefits of New Set Methods ๐ฏ
Simplified Operations: Perform common set operations with built-in methods, reducing the need for custom code.
Cleaner Code: Enhance code readability and maintainability by using native methods.
Consistency: Aligns with the latest ECMAScript standards, ensuring consistency across JavaScript and TypeScript.
Real-World Application ๐
Consider a scenario where you manage user roles in a web application. You might have sets representing different user permissions:
const adminPermissions = new Set(["read", "write", "delete"]);
const userPermissions = new Set(["read", "write"]);
const allPermissions = adminPermissions.union(userPermissions);
const commonPermissions = adminPermissions.intersection(userPermissions);
const adminOnlyPermissions = adminPermissions.difference(userPermissions);
console.log(allPermissions); // Set {"read", "write", "delete"}
console.log(commonPermissions); // Set {"read", "write"}
console.log(adminOnlyPermissions); // Set {"delete"}
These new methods make it easy to manage and compare user permissions, ensuring your application logic is both concise and clear.
Conclusion ๐
The new ECMAScript Set Methods in TypeScript 5.5 are a powerful addition that simplifies set operations and enhances code readability. By leveraging these built-in methods, you can write cleaner, more maintainable code while aligning with the latest standards.
Ready to Simplify Your Set Operations?
Try out these new methods in your next TypeScript project and experience the benefits firsthand! Stay tuned for more exciting updates and tips on Tech Talk by Jobin. ๐
For more detailed information, check out the TypeScript 5.5 release notes and the official TypeScript documentation.
Subscribe to my newsletter
Read articles from Jobin Mathew directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
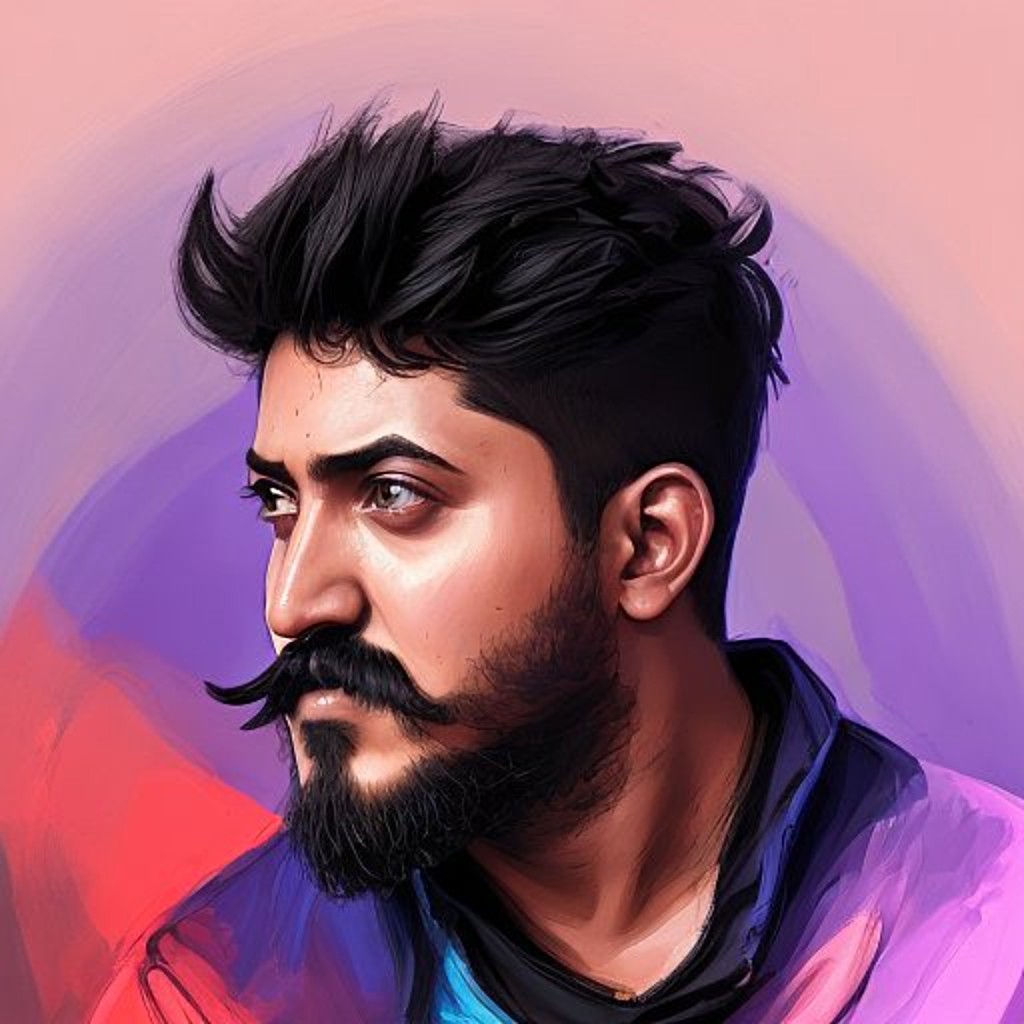
Jobin Mathew
Jobin Mathew
Hey there! I'm Jobin Mathew, a passionate software developer with a love for Node.js, AWS, SQL, and NoSQL databases. When I'm not working on exciting projects, you can find me exploring the latest in tech or sharing my coding adventures on my blog. Join me as I decode the world of software development, one line of code at a time!