Part 1: Getting Started with Django Tweet Project
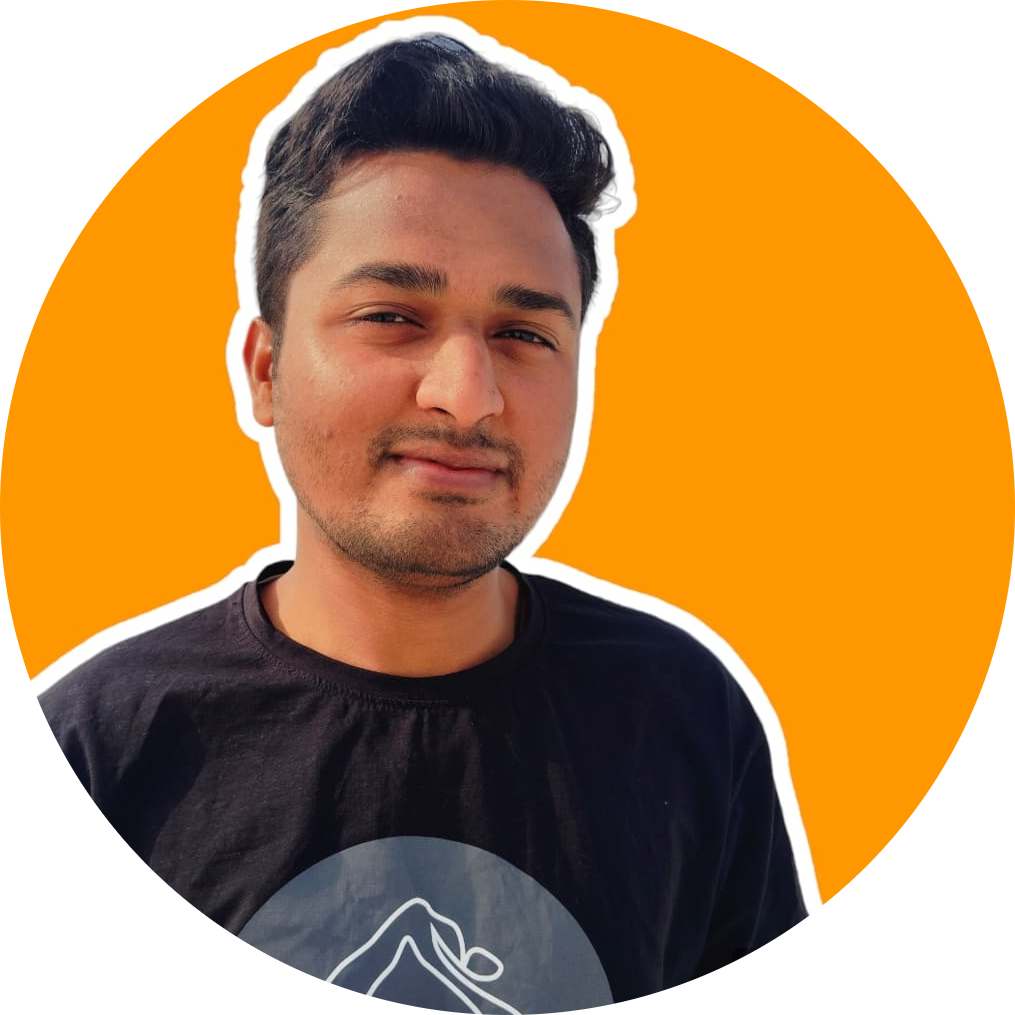
Prerequisites
Before we begin, ensure you have the following installed on your system:
Python: Python 3.6 or later.
Django: The web framework we will use to build our project.
Virtual Environment: To manage dependencies for your project.
Introduction
Hello! I'm Dhruv, and in this blog series, I'll be documenting the process of creating a Tweet Project using Django. This project aims to simulate a simplified version of a Twitter-like application where users can post tweets, view them, and interact with them. In Part 1, we will set up our development environment, create our Django project, and set up a basic structure.
Step-by-Step Guide
1. Create Project Directory
First, let's create a directory for our project. Open your terminal and navigate to the path where you want to create your project folder, then execute:
mkdir tweet-demo-project
cd tweet-demo-project
2. Set Up Virtual Environment
Next, we'll set up a virtual environment to manage our project's dependencies. Run the following commands:
python -m venv .venv
.venv\Scripts\activate # On Windows
source .venv/bin/activate # On macOS/Linux
3. Install Django
With the virtual environment activated, install Django and upgrade pip
:
pip install django
python.exe -m pip install --upgrade pip
To keep track of our project dependencies, create a requirements.txt
file:
pip freeze > requirements.txt
4. Create Django Project
Now, we'll create our Django project. Run:
django-admin startproject tweetdemo
cd tweetdemo
python manage.py runserver
You should see an output indicating the server is running. Open your browser and navigate to http://127.0.0.1:8000/
to see the default Django welcome page.
5. Make Migrations and Apply Migrations
Before applying the initial migrations, we need to create them. This sets up the database schema according to the models defined in Django's default apps. Run:
python manage.py makemigrations
Now, apply the migrations to set up the database:
python manage.py migrate
6. Create Superuser
Create a superuser to access the Django admin interface:
python manage.py createsuperuser
Enter the required information (username, email, password) when prompted.
7. Configure Static and Media Files
Open settings.py
and add the following configurations to handle static and media files:
import os
STATIC_URL = '/static/'
STATICFILES_DIRS = [os.path.join(BASE_DIR, 'static')]
MEDIA_URL = '/media/'
MEDIA_ROOT = os.path.join(BASE_DIR, 'media')
Here’s a breakdown:
STATIC_URL: This is the URL prefix for serving static files (CSS, JavaScript, images).
STATICFILES_DIRS: This tells Django where to look for additional static files.
MEDIA_URL: This is the URL prefix for serving user-uploaded files.
MEDIA_ROOT: This is the file system path to the directory that will hold user-uploaded files.
Create the necessary directories:
mkdir static
mkdir media
8. Update URLs for Media Files
In urls.py
, add the following imports and configurations to serve media files during development:
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
path('admin/', admin.site.urls),
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Here’s a breakdown:
settings: Importing settings allows us to access the
MEDIA_URL
andMEDIA_ROOT
configurations.static: The
static
function is used to serve static and media files during development.
9. Create Tweet App
Create a new Django app named tweet
:
python manage.py startapp tweet
10. Register Tweet App
Tell our tweetdemo
project about the tweet
app. In tweetdemo/
settings.py
, add 'tweet',
to the INSTALLED_APPS
list:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'tweet',
]
The INSTALLED_APPS
list contains all Django applications that are activated in this Django instance. Adding 'tweet'
allows Django to include the configurations and models of the tweet
app.
Also, update the TEMPLATES
setting to include a templates
directory:
'DIRS': [os.path.join(BASE_DIR, 'templates')],
Here’s a breakdown:
- DIRS: This is a list of directories where the
DjangoTemplates
backend will search for templates.
11. Link Tweet App URLs
In tweetdemo/
urls.py
, include the tweet
app URLs:
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('tweet/', include('tweet.urls')),
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Here’s a breakdown:
- include: The
include
function allows referencing other URL configurations. This is used to include thetweet
app's URL patterns in the main URL configuration.
12. Create a View in Tweet App
In tweet/
views.py
, create a simple index view:
from django.shortcuts import render
def index(request):
return render(request, 'index.html')
13. Set Up Tweet App URLs
Create a urls.py
file in the tweet
app and link the index view:
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
]
14. Create Template for Tweet App
Create a templates
folder within the tweet
app and add an index.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Tweet Project</title>
<style>
body {
background-color: #000;
color: #FFF;
}
</style>
</head>
<body>
<h1>TWEET INDEX TEMPLATE</h1>
</body>
</html>
15. Test the Setup
Run the development server:
python manage.py runserver
Visit http://127.0.0.1:8000/tweet/
in your browser to see the index template.
This concludes Part 1 of our Django Tweet Project. We have set up the project, created a basic structure, and ensured everything is running smoothly. Stay tuned for Part 2, where we will dive deeper into building out the Tweet functionality.
Subscribe to my newsletter
Read articles from Dhruvkumar Maisuria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
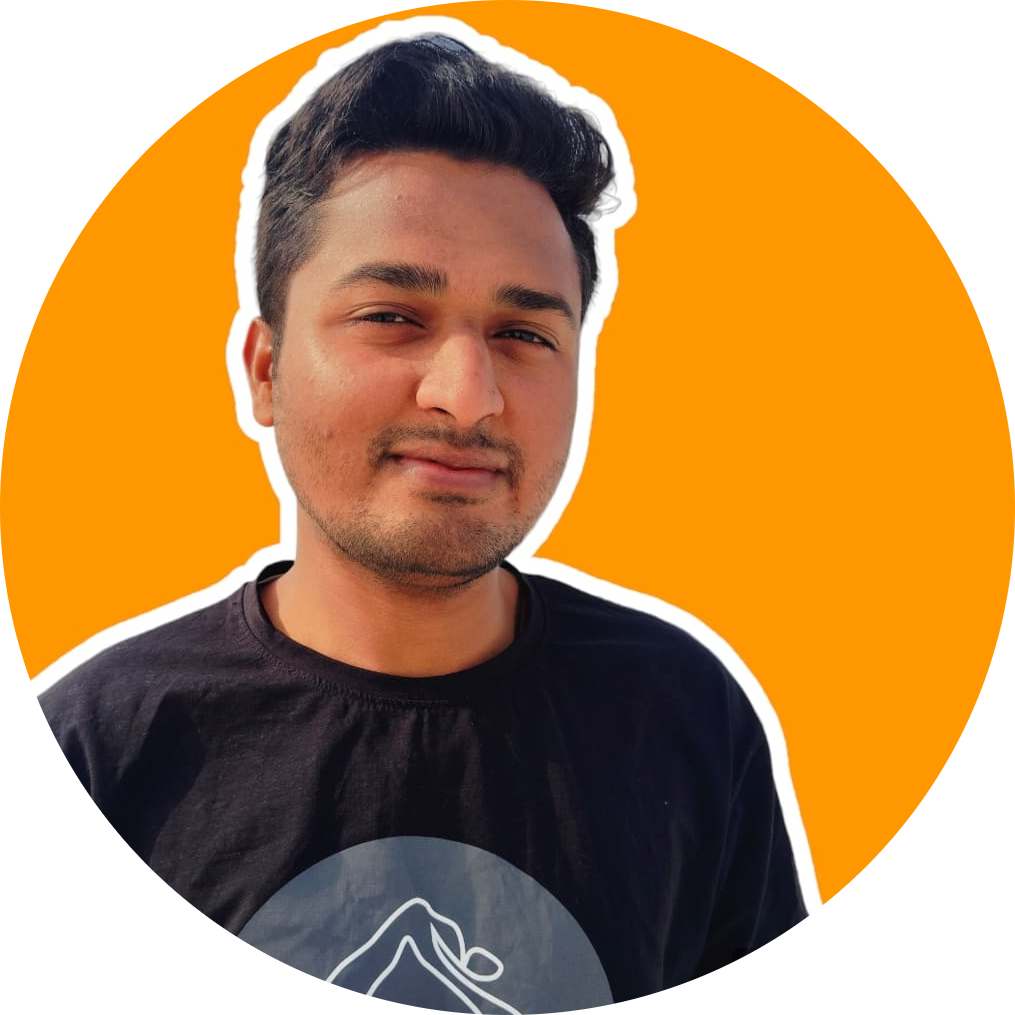
Dhruvkumar Maisuria
Dhruvkumar Maisuria
About Me As a dedicated tech enthusiast with a passion for continuous learning, I possess a robust background in software development and network engineering. My journey in the tech world is marked by an insatiable curiosity and a drive to solve complex problems through innovative solutions. I have honed my skills across a diverse array of technologies, including Python, AWS, SQL, and Golang, and have a strong foundation in web development, API testing, and cloud computing. My hands-on experience ranges from creating sophisticated applications to optimizing network performance, underscored by a commitment to excellence and a meticulous attention to detail. In addition to my technical prowess, I am an avid advocate for knowledge sharing, regularly contributing to the tech community through blogs and open-source projects. My proactive approach to professional development is demonstrated by my ongoing exploration of advanced concepts in programming and networking, ensuring that I stay at the forefront of industry trends. My professional journey is a testament to my adaptability and eagerness to embrace new challenges, making me a valuable asset in any dynamic, forward-thinking team. Whether working on a collaborative project or independently, I bring a blend of analytical thinking, creative problem-solving, and a deep-seated passion for technology to every endeavor.