Deep Dive into Data Types in C#
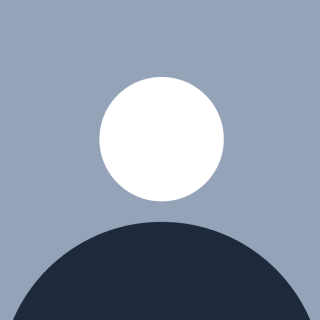
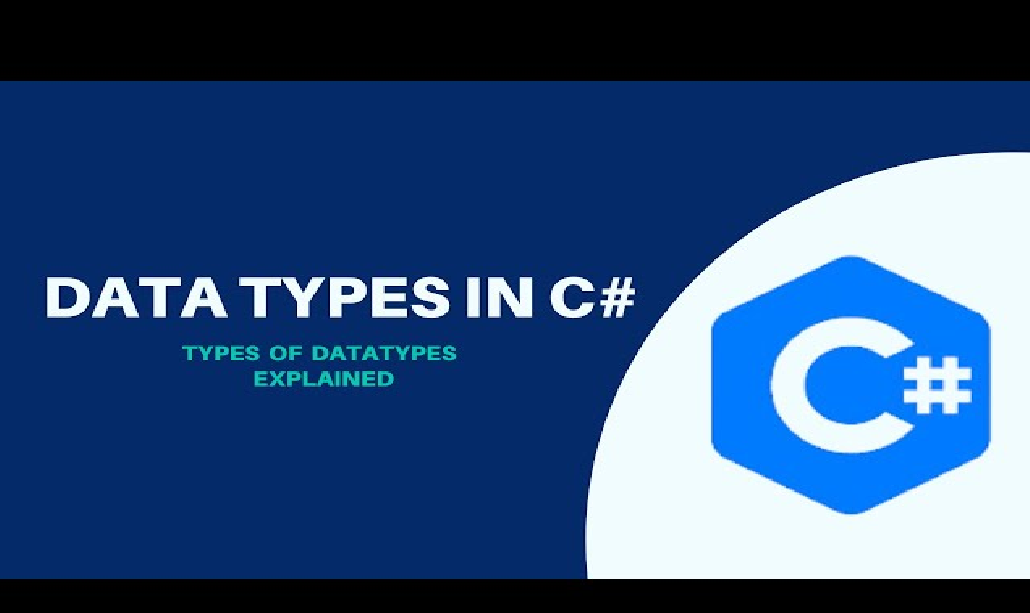
In C#, data types are fundamental building blocks that define the kind of data a variable can store and manipulate. Understanding these types is essential for efficient programming and ensuring that your application performs optimally. Let’s explore the various data types available in C# along with their memory size and range:
Basic Data Types
. int:
Memory Size: 32 bits (4 bytes) .
Range: -2,147,483,648 to 2,147,483,647 .
Description: It's used for whole numbers and is ideal for counters or simple calculations.
Example: 'int age = 30;'.
. float:
Memory Size: 32 bits (4 bytes) .
Range: Approximately ±1.5 x 10^−45 to ±3.4 x 10^38 .
Description: Represents a single.precision floating.point number. Useful for numbers with decimal points where high precision is not critical.
Example: 'float price = 19.95f;'.
. double:
Memory Size: 64 bits (8 bytes) .
Range: Approximately ±5.0 × 10^−324 to ±1.7 × 10^308 .
Description: Represents a double.precision floating.point number and offers more precision than 'float'. Ideal for scientific calculations or scenarios requiring high precision.
Example: 'double pi = 3.14159265359;'.
. char:
Memory Size: 16 bits (2 bytes) .
Range: 0 to 65,535 (Unicode characters) .
Description: Useful for storing individual characters.
Example: 'char grade = 'A';'.
. bool:
Memory Size: 8 bits (1 byte, though it may vary by implementation) .
Range: 'true' or 'false' .
Description: Represents a Boolean value used in conditional statements and logical operations.
Example: 'bool isActive = true;'.
Non.Primitive Data Types
. 'string':
Memory Size: Variable (depends on the length of the string) .
Range: Limited by available memory .
Description: Represents a sequence of characters and is used for textual data. 'string' is a reference type, holding a reference to the data rather than the data itself.
Example: 'string message = "Hello, World!";'.
. object:
Memory Size: Depends on the type of data it holds .
Range: Depends on the type of data .
Description: The base type from which all other types derive. It can hold any type of data but requires type casting for specific operations.
Example: 'object data = 100;'.
Specialized Data Types
. decima:
Memory Size: 128 bits (16 bytes) .
Range: ±1.0 × 10^−28 to ±7.9 × 10^28 (with 28.29 significant digits) . Description:It is same like float data type but it can accept large range compare to float and end of the number just give 'm'
Example: 'decimal balance = 1000.50m;'.
. DateTime:
Memory Size: 64 bits (8 bytes) .
Range: January 1, 0001 to December 31, 9999 .
Description: Represents dates and times with a high level of precision.
Example: 'DateTime today = DateTime.Now;'.
. TimeSpan:
Memory Size: 64 bits (8 bytes) .
Range: ±10675199.02 days (approximately ±29,000 years) .
Description: Represents a time interval and is useful for measuring elapsed time or differences between dates.
Example: 'TimeSpan duration = TimeSpan.FromHours(2);'.
Choosing the Right Data Type
Selecting the correct data type is crucial for several reasons:
. Memory Efficiency: Using appropriate types helps in optimizing memory usage.
For example, 'short' or 'byte' might be more memory.efficient than 'int' for certain applications.
. Performance: Different data types have varying performance characteristics. For instance, 'double' has more overhead than 'float', so choose based on the precision you need.
. Accuracy: For financial calculations, always use 'decimal' rather than 'float' or 'double' to avoid precision errors.
Conclusion
Mastering data types in C# is essential for writing clear, efficient, and effective code. By understanding the memory size and range of different data types, you can make informed decisions that enhance your applications' performance and reliability. Whether you’re dealing with simple variables or complex data structures, a solid grasp of C# data types is key to successful programming.
Feel free to reach out if you have any questions or need further clarification on C# data types!
Subscribe to my newsletter
Read articles from Suraj Pasunuri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by