AI, ML, DL, and Data Science: An In-Depth Guide
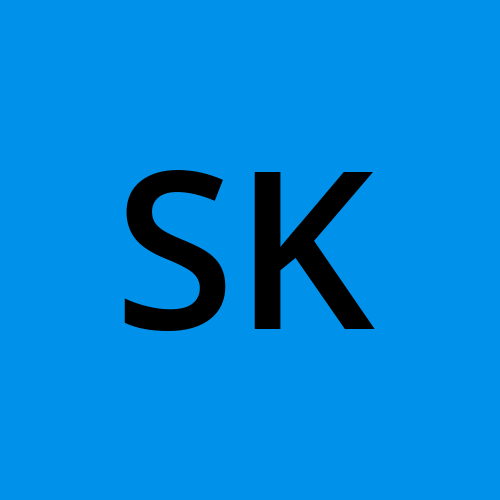
In today's data-driven world, terms like Artificial Intelligence (AI), Machine Learning (ML), Deep Learning (DL), and Data Science are often used interchangeably. However, they represent different concepts and techniques used in data analysis. This blog post aims to clarify these concepts, explain how they are interlinked, and provide practical examples to illustrate their applications. Whether you are new to technology or looking to deepen your understanding, this guide will help you navigate these fields and decide where to start your learning journey.
1. Artificial Intelligence (AI)
AI is the broadest concept among the four and refers to the simulation of human intelligence in machines. These machines are designed to think, learn, and solve problems autonomously. AI encompasses various subfields, including ML and DL.
Example: Virtual assistants like Siri or Alexa use AI to understand and respond to voice commands, perform tasks, and learn user preferences over time.
When to Use AI: AI is used when tasks require human-like decision-making, language understanding, or sensory perception. It is ideal for applications involving natural language processing, robotics, and predictive analytics.
Tools & Implementation:
Programming Languages: Python, Java, C++
Libraries: TensorFlow, PyTorch, OpenAI GPT
Example Code in Python:
import openai response = openai.Completion.create( engine="davinci", prompt="Translate the following English text to French: 'Hello, how are you?'", max_tokens=60 ) print(response.choices[0].text.strip())
2. Machine Learning (ML)
ML is a subset of AI that focuses on enabling machines to learn from data without being explicitly programmed. ML algorithms identify patterns in data and make predictions or decisions based on those patterns.
Example: A spam filter in your email uses ML to classify incoming emails as spam or not spam based on patterns learned from a large dataset of emails.
When to Use ML: Use ML when you have large datasets and need to make predictions or decisions based on historical data. It is commonly used in recommendation systems, fraud detection, and customer segmentation.
Tools & Implementation:
Programming Languages: Python, R
Libraries: Scikit-learn, Keras, XGBoost
Example Code in Python:
from sklearn.datasets import load_iris from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import accuracy_score # Load data iris = load_iris() X = iris.data y = iris.target # Split data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train model model = RandomForestClassifier(n_estimators=100) model.fit(X_train, y_train) # Predict and evaluate y_pred = model.predict(X_test) print("Accuracy:", accuracy_score(y_test, y_pred))
3. Deep Learning (DL)
DL is a specialized subset of ML that uses neural networks with many layers (hence "deep") to model complex patterns in data. DL excels in processing large amounts of unstructured data like images, audio, and text.
Example: Image recognition systems use DL to identify objects in photos, such as recognizing a cat or a dog.
When to Use DL: DL is used when the problem involves high-dimensional data, such as image or speech recognition. It requires large datasets and significant computational power.
Tools & Implementation:
Programming Languages: Python
Libraries: TensorFlow, PyTorch
Example Code in Python using TensorFlow:
import tensorflow as tf from tensorflow.keras import layers, models from tensorflow.keras.datasets import mnist # Load data (x_train, y_train), (x_test, y_test) = mnist.load_data() x_train, x_test = x_train / 255.0, x_test / 255.0 # Build model model = models.Sequential([ layers.Flatten(input_shape=(28, 28)), layers.Dense(128, activation='relu'), layers.Dropout(0.2), layers.Dense(10, activation='softmax') ]) # Compile and train model model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) model.fit(x_train, y_train, epochs=5) # Evaluate model test_loss, test_acc = model.evaluate(x_test, y_test) print('Test accuracy:', test_acc)
4. Data Science
Data Science is an interdisciplinary field that combines domain knowledge, programming skills, and knowledge of mathematics and statistics to extract meaningful insights from data. It encompasses data collection, cleaning, analysis, visualization, and interpretation.
Example: A data scientist at a retail company might analyze customer purchase data to identify trends and recommend product strategies.
When to Use Data Science: Use data science when you need to analyze complex data sets to extract actionable insights. It is essential for business intelligence, market analysis, and research.
Tools & Implementation:
Programming Languages: Python, R, SQL
Libraries: Pandas, NumPy, Matplotlib, Seaborn
Example Code in Python:
import pandas as pd import seaborn as sns import matplotlib.pyplot as plt # Load data df = sns.load_dataset('iris') # Data analysis and visualization print(df.describe()) sns.pairplot(df, hue='species') plt.show()
Interconnection and Workflow
These fields are interconnected and often used together in workflows:
Data Collection and Cleaning (Data Science): Gather and prepare data for analysis.
Exploratory Data Analysis (Data Science): Understand data patterns and relationships.
Model Building (ML/DL): Develop predictive models using ML/DL techniques.
Deployment (AI): Implement models into AI systems for real-world applications.
Choosing What to Learn First
Start with Data Science: Understand the basics of data handling and analysis.
Learn Machine Learning: Build predictive models from your data.
Explore Deep Learning: Dive into complex data like images and texts.
Understand AI: Integrate models into intelligent systems.
By following this progression, you will build a solid foundation and gradually advance to more complex topics.
Conclusion
AI, ML, DL, and Data Science are powerful tools for analyzing data and extracting insights. By understanding their differences and interconnections, you can choose the right techniques for your needs and embark on a rewarding learning journey. Remember to start with the basics of Data Science and progressively move towards ML and DL to build a comprehensive skill set.
Subscribe to my newsletter
Read articles from Sandhya Kondmare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
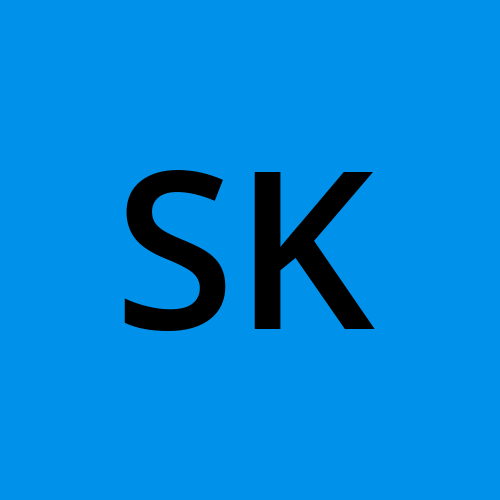
Sandhya Kondmare
Sandhya Kondmare
Aspiring DevOps Engineer with 2 years of hands-on experience in designing, implementing, and managing AWS infrastructure. Proven expertise in Terraform for infrastructure as code, automation tools, and scripting languages. Adept at collaborating with development and security teams to create scalable and secure architectures. Hands-on in AWS, GCP, Azure and Terraform best practices.