Easy Guide to Sending Push Notifications in React Native via Firebase: Android

Table of contents
- Introduction
- Setting Up Push Notifications using Firebase Cloud Messaging (FCM)
- For Android
- Configure Firebase for Android:
- React Native Project
- 1. Update android/app/build.gradle
- 2. Add google-services.json file to android/app
- 3. Update android/build.gradle
- 4. Install modules
- 5. Import modules
- 6. Paste Code Snippet
- 7. Run Project
- 8. Give permission
- 9. Go to firebase console
- 10. Enter Details for Notification
- 11. Schedule campaign
- 12. Publish campaign
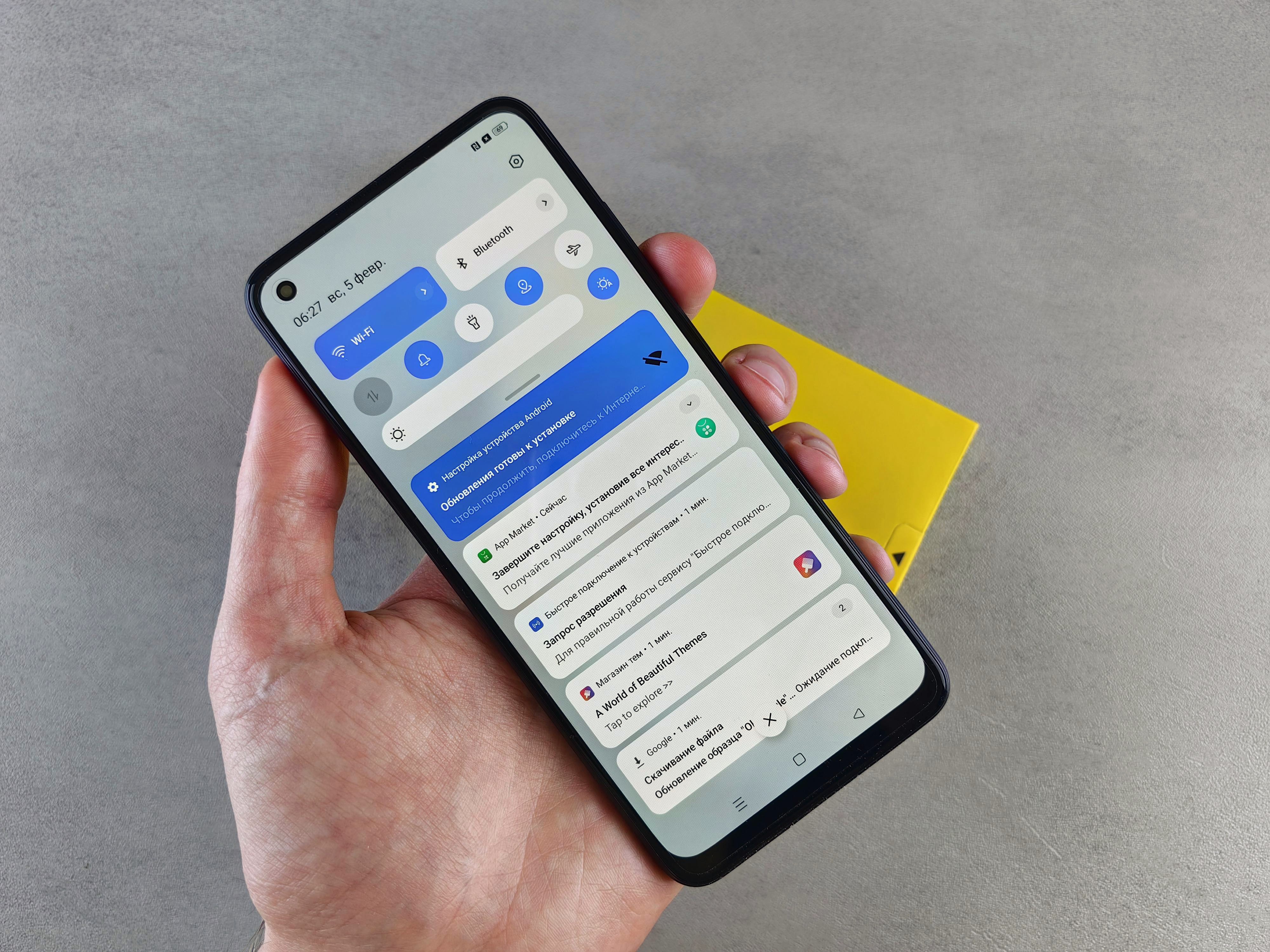
Introduction
Push notifications are a pivotal feature for mobile applications, offering developers and stakeholders a powerful tool to engage users with timely and relevant updates.
Setting Up Push Notifications using Firebase Cloud Messaging (FCM)
FCM clients need devices with Android 4.4 or higher that have the Google Play Store app installed, or an emulator running Android 4.4 with Google APIs.
For Android
Configure Firebase for Android:
Go to the Firebase Console
create a project.
Add an Android app to your Firebase project.
Get the package name
com.<DemoApp>
from the file located atandroid/app/src/main/java/com/<ProjectName>/MainApplication.kt
frompackage com.<DemoApp>
.providing the remaining required details.
Download the
google-services.json
file.
React Native Project
Note: If your project is not set up yet, start by setting up a React Native project. Refer to React Native with Expo or React Native without Framework.
1. Update android/app/build.gradle
- add the
google-services
plugin to yourandroid/app/build.gradle
file:
apply plugin: 'com.google.gms.google-services'
- add the following dependency to your
android/app/build.gradle
file:
implementation platform('com.google.firebase:firebase-bom:33.1.2')
2. Add google-services.json
file to android/app
- Add the
google-services.json
file and place it in theandroid/app
directory of your React Native project.
3. Update android/build.gradle
- add the following class path to your
android/build.gradle
file:
classpath 'com.google.gms:google-services:4.4.2'
4. Install modules
@react-native-firebase/app
@react-native-firebase/messaging
For React Native Local and Remote Notifications on iOS and Android
react-native-push-notification
npm i @react-native-firebase/app @react-native-firebase/messaging react-native-push-notification
5. Import modules
import these modules in your App.jsx file.
import messaging from '@react-native-firebase/messaging';
import { PermissionsAndroid } from 'react-native';
import PushNotification from 'react-native-push-notification';
6. Paste Code Snippet
Paste this code snippet inside your App component.
async function requestUserPermission() {
PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.POST_NOTIFICATIONS,
);
const authStatus = await messaging().requestPermission();
const enabled =
authStatus === messaging.AuthorizationStatus.AUTHORIZED ||
authStatus === messaging.AuthorizationStatus.PROVISIONAL;
if (enabled) {
console.log('Authorization status:', authStatus);
}
}
const getToken = async () => {
try {
const token = await messaging().getToken();
console.log('Token =>', token);
console.log('Use above token to start campaign');
} catch (error) {
console.error('Failed to get FCM token:', error);
}
};
useEffect(() => {
requestUserPermission();
getToken();
if (Platform.OS === 'android') {
PushNotification.createChannel(
{
channelId: '',
channelName: 'test',
channelDescription: '',
soundName: 'default',
importance: 4,
vibrate: true,
}
);
}
messaging().onMessage(async remoteMessage => {
console.log('New FCM message arrived => \n', remoteMessage);
PushNotification.localNotification({
channelId: 'default-channel-id',
title: remoteMessage.notification.title,
message: remoteMessage.notification.body,
imageUrl: remoteMessage.notification.imageUrl
});
});
}, []);
Note: If needed refer to source code.
7. Run Project
Run your React Native project on a real device or an emulator.
8. Give permission
Allow notification permission for your React Native app.
9. Go to firebase console
On the Firebase console, click the Cloud Messaging option and create your first campaign or a new campaign. Select the Notification option.
Note: copy token logged in your console to use it in identifying app if needed. Restart project if needed.
10. Enter Details for Notification
Enter the notification details like Title and Text. Then click next. Choose your target app from the dropdown.
11. Schedule campaign
Schedule your campaign. If you want to get the notification right away, choose the 'now' option from the dropdown.
12. Publish campaign
Review the campaign details and publish if everything is correct. You will receive a notification.
Subscribe to my newsletter
Read articles from Md Sohail Fiza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
