Python Lambda Functions: Beginner-Friendly Overview

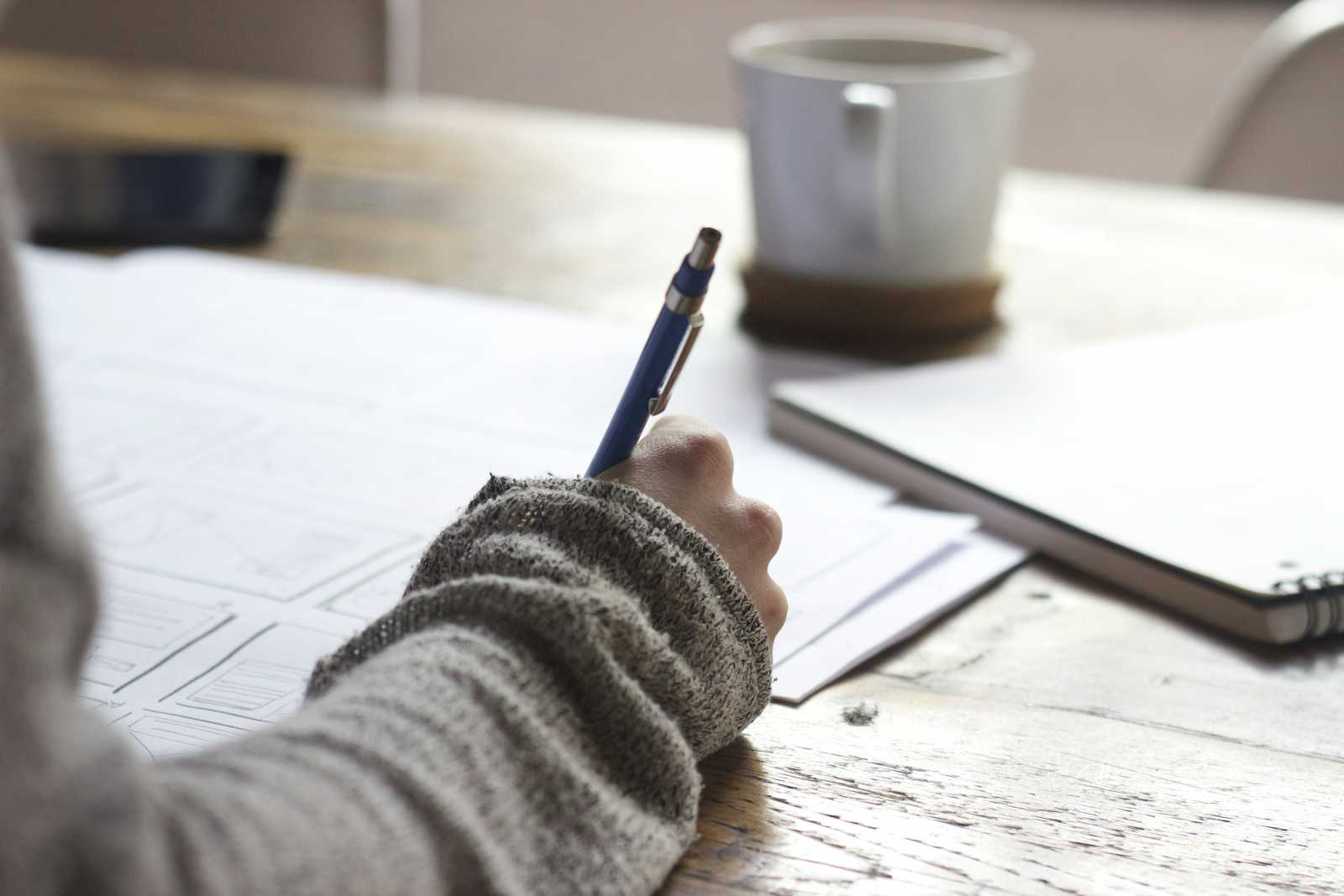
Explanation:
A lambda function in Python is a small anonymous function defined using the lambda keyword. It can take any number of arguments but can only have one expression. The expression is evaluated and returned.
Syntax:
lambda arguments: expression
Example:
# A lambda function that adds 10 to the input
add_ten = lambda x: x + 10
print(add_ten(5)) # Output: 15
Use Cases:
Short functions that are used once or a few times.
Used with higher-order functions like map(), filter(), and reduce().
Common Tricks:
- Simplifying small functions:
def add(a, b):
return a + b
# Can be simplified as:
add = lambda a, b: a + b
- Using with map():
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda x: x**2, numbers))
# squares will be [1, 4, 9, 16, 25]
- Using with filter():
numbers = [1, 2, 3, 4, 5]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
# even_numbers will be [2, 4]
- Using with reduce() from functools:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
product = reduce(lambda x, y: x * y, numbers)
# product will be 120
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!