Understanding String Immutability in Java: A Comprehensive Guide
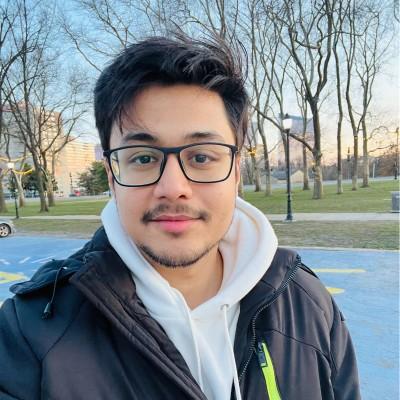
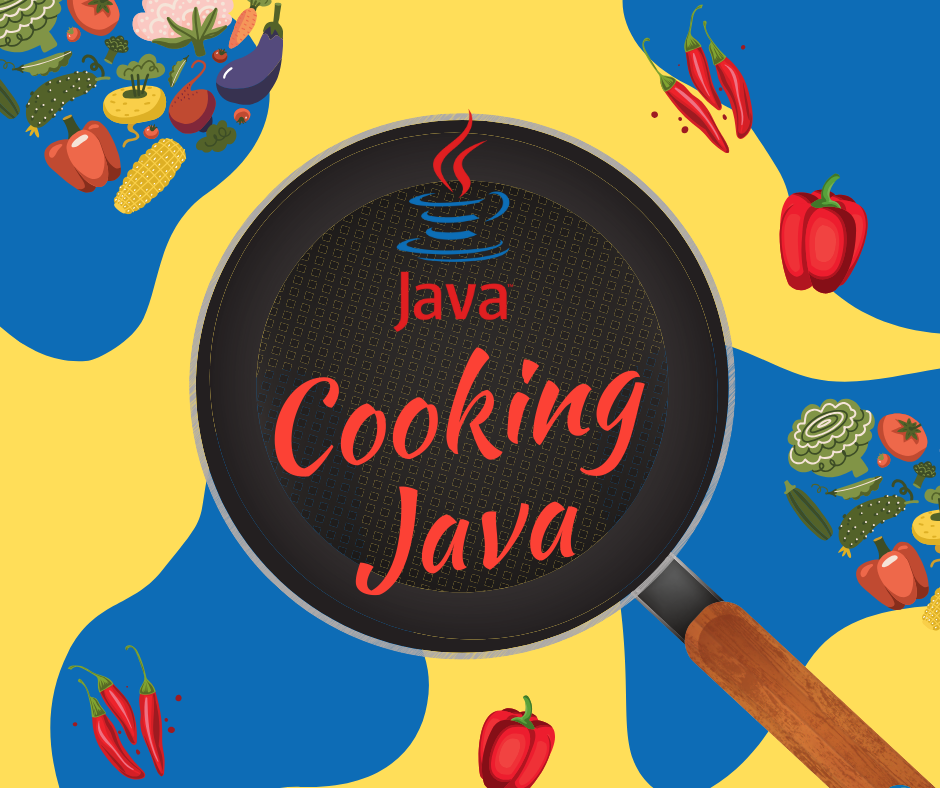
In Java, strings play a crucial role in most applications, ranging from simple text manipulation to complex data processing. One of the fundamental characteristics of strings in Java is their immutability. This blog delves into what immutability means, why strings are immutable, and how this impacts your programming.
What is String Immutability?
Immutability means that once an object is created, it cannot be changed. For strings in Java, this implies that once a String
object is instantiated, the value it holds cannot be altered. If you need to modify a string, a new String
object is created with the modified value.
Why are Strings Immutable in Java?
1. Security
Strings are widely used as parameters for network connections, file paths, and user credentials. If strings were mutable, it would be possible for a malicious actor to alter the value of the string, leading to security vulnerabilities. Immutability ensures that the value of a string remains constant throughout its lifecycle, providing a layer of security.
2. String Pooling
Java optimizes memory usage by maintaining a pool of strings. When a new string is created, the JVM checks the pool to see if the string already exists. If it does, the reference to the existing string is returned. This process is known as string interning. Immutability allows string pooling to be effective because the same string value can be safely shared across multiple references without risk of modification.
3. Thread Safety
4. Performance
While it may seem counterintuitive, the immutability of strings can lead to performance benefits. The JVM can make certain optimizations, knowing that a string’s value will not change. Additionally, operations like substring sharing and hashcode caching are facilitated by immutability.
How Does String Immutability Work?
Creating a String
When you create a string in Java, it is stored in the string pool if it is a string literal.
String str1 = "Hello";
String str2 = "Hello";
System.out.println(str1 == str2); // Output: true
In this example, both str1
and str2
refer to the same object in the string pool.
Modifying a String
If you modify a string, a new object is created.
String str1 = "Hello";
String str2 = str1.concat(" World");
System.out.println(str1); // Output: Hello
System.out.println(str2); // Output: Hello World
Here, str1
remains unchanged, and str2
is a new String
object with the modified value.
Benefits of String Immutability
1. Consistency and Predictability
With immutable strings, you can be confident that the value of a string will remain consistent throughout its lifecycle. This predictability makes it easier to reason about your code and reduces the likelihood of bugs.
2. Reduced Memory Overhead
String pooling reduces memory overhead by reusing existing string objects. This is possible only because strings are immutable, ensuring that no reference to a pooled string will be unexpectedly altered.
3. Improved Performance in Collections
Immutable objects are often used as keys in collections like HashMap
and HashSet
. Since the hashcode of an immutable object remains constant, lookups are more efficient, and the integrity of the collection is maintained.
Conclusion
Understanding string immutability is essential for effective Java programming. It enhances security, improves performance, simplifies thread safety, and optimizes memory usage through string pooling. By embracing the immutability of strings, you can write more robust and maintainable Java applications.
Happy coding!
Subscribe to my newsletter
Read articles from Pratik Upreti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
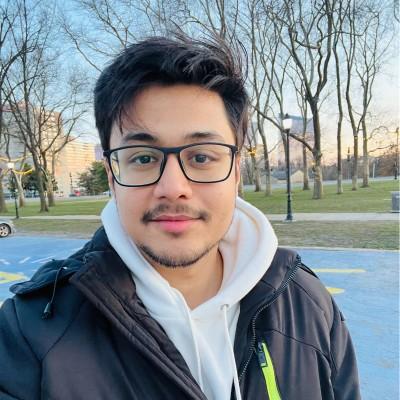
Pratik Upreti
Pratik Upreti
Hello! I’m Pratik Upreti, a seasoned software engineer with a rich background in languages like C#, Java, JavaScript, Python, SQL, and MySQL, I bring a diverse skill set to the table. I excel in backend and server technologies, including Node.js, Django, GraphQL, and Prisma ORM, and am adept in frontend development with Next.js, React.js and Tailwind CSS. Professionally, contributing at Brilliant Infotech.