Essential Tips for Optimizing PostgreSQL Semi-Joins and Anti-Joins
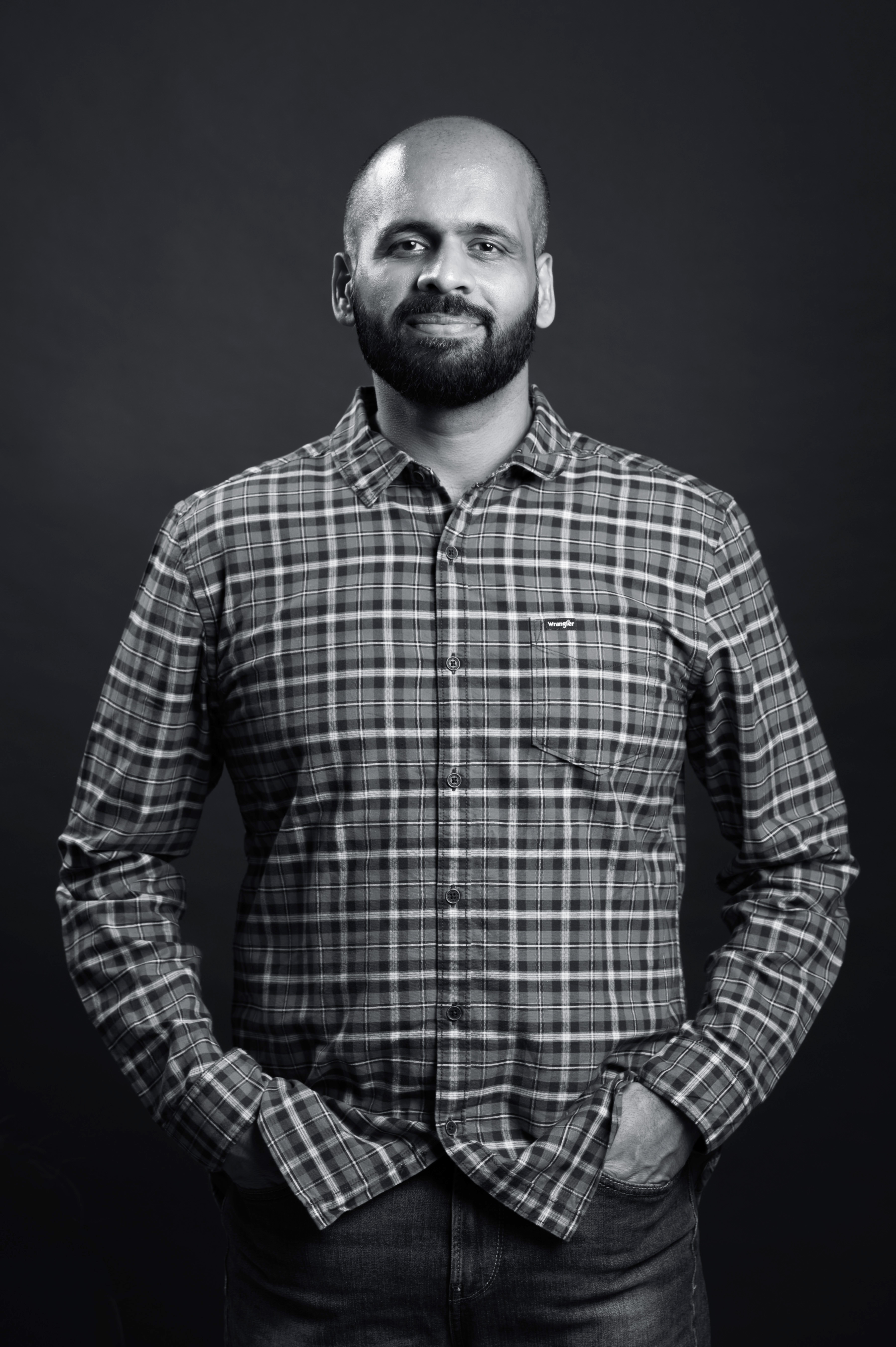
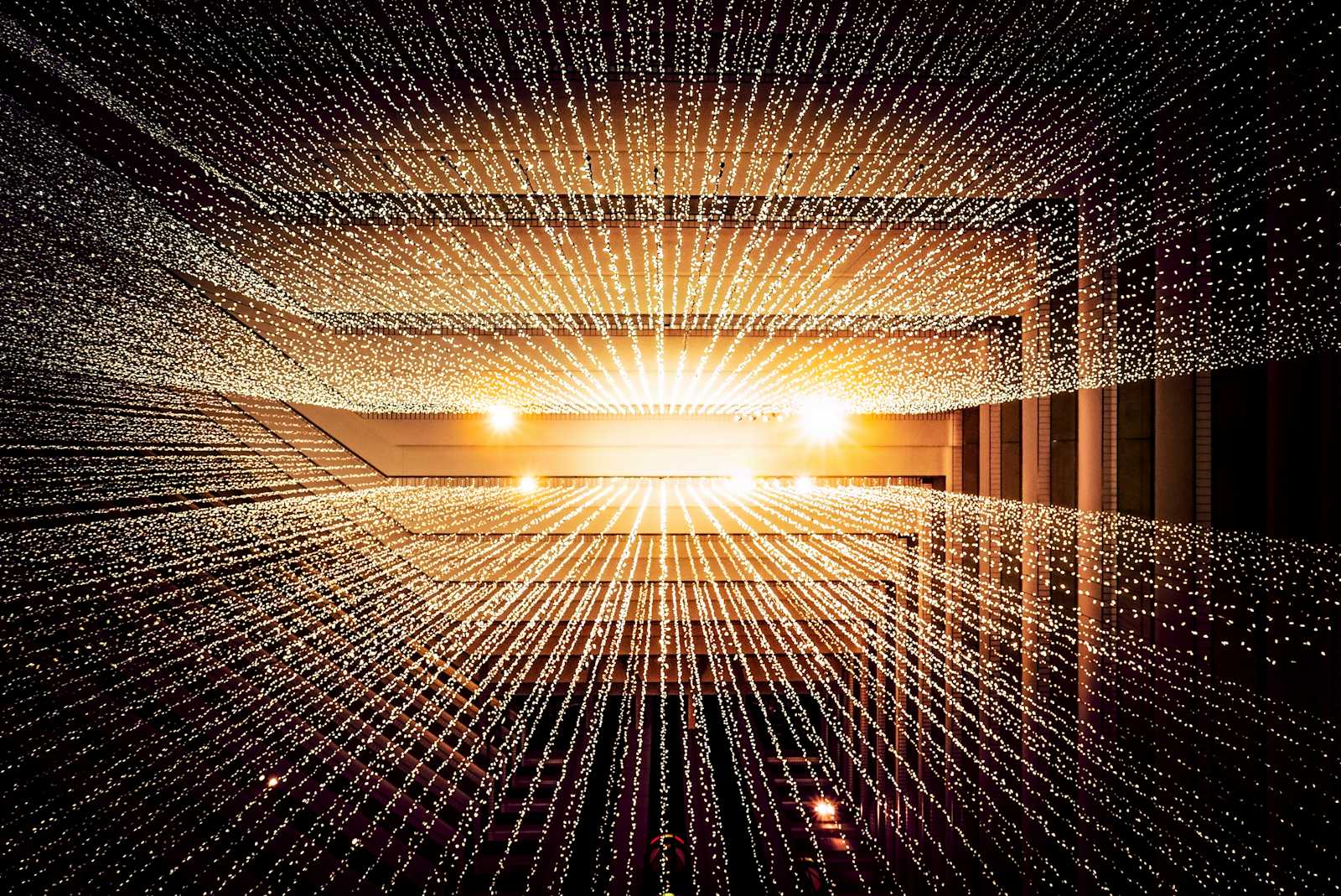
Introduction
Optimizing database queries is crucial for achieving high performance and scalability, especially when working with large datasets. In PostgreSQL, semi-joins and anti-joins are powerful techniques used to filter results based on the existence or non-existence of related data in another table. While these operations are commonly implemented using the JOIN operator, they can often be optimized further using the EXISTS and NOT EXISTS clauses. Understanding how to efficiently implement semi-joins and anti-joins can significantly enhance query performance, reduce resource consumption, and improve overall database responsiveness. This guide provides a comprehensive overview of the best practices for optimizing semi-joins and anti-joins in PostgreSQL, including detailed examples, performance tips, and practical advice for leveraging PostgreSQL's capabilities to their fullest extent.
Semi-Joins
A semi-join can be implemented using the EXISTS
clause. This approach is often more efficient than using the JOIN
operator because it stops searching as soon as it finds the first match.
Example: Semi-Join with EXISTS
SELECT t1.*
FROM table1 t1
WHERE EXISTS (
SELECT 1
FROM table2 t2
WHERE t1.id = t2.id
);
Anti-Joins
An anti-join can be implemented using the NOT EXISTS
clause. This method is typically more efficient for anti-joins compared to using LEFT JOIN
with IS NULL
.
Example: Anti-Join with NOT EXISTS
SELECT t1.*
FROM table1 t1
WHERE NOT EXISTS (
SELECT 1
FROM table2 t2
WHERE t1.id = t2.id
);
Tips and Tricks for Optimal Performance
Use Indexes:
Ensure that the columns used in the
JOIN
condition orEXISTS
subquery have indexes. Indexes on these columns can significantly speed up the search for matching rows.Example:
CREATE INDEX idx_table1_id ON table1(id); CREATE INDEX idx_table2_id ON table2(id);
Analyze and Vacuum:
Regularly run
ANALYZE
andVACUUM
on your tables to keep the statistics up-to-date. This helps the query planner make better decisions.Example:
ANALYZE table1; ANALYZE table2;
Limit the Result Set in EXISTS:
Use
SELECT 1
in theEXISTS
subquery to minimize the amount of data processed.Example:
SELECT t1.* FROM table1 t1 WHERE EXISTS ( SELECT 1 FROM table2 t2 WHERE t1.id = t2.id );
Use JOIN Hints:
In some cases, you can use join hints or change the join type to guide the planner.
Example:
SELECT /*+ LEADING(t1 t2) */ t1.* FROM table1 t1 JOIN table2 t2 ON t1.id = t2.id;
*Avoid Using SELECT :
Select only the necessary columns to reduce the amount of data processed and transferred.
Example:
SELECT t1.id, t1.name FROM table1 t1 WHERE EXISTS ( SELECT 1 FROM table2 t2 WHERE t1.id = t2.id );
Consider Subquery Filters:
Apply additional filters within the subquery to reduce the number of rows processed.
Example:
SELECT t1.* FROM table1 t1 WHERE EXISTS ( SELECT 1 FROM table2 t2 WHERE t1.id = t2.id AND t2.status = 'active' );
Use EXPLAIN to Analyze Queries:
Use
EXPLAIN
to understand the execution plan of your queries and identify potential bottlenecks.Example:
EXPLAIN ANALYZE SELECT t1.* FROM table1 t1 WHERE EXISTS ( SELECT 1 FROM table2 t2 WHERE t1.id = t2.id );
Optimize JOIN Conditions:
Ensure that the join conditions are simple and use indexed columns. Complex expressions or functions in the join condition can lead to poor performance.
Example:
SELECT t1.* FROM table1 t1 WHERE EXISTS ( SELECT 1 FROM table2 t2 WHERE t1.id = t2.id );
Conclusion
Efficiently implementing semi-joins and anti-joins in PostgreSQL is essential for optimizing query performance and ensuring smooth database operations. By utilizing the EXISTS and NOT EXISTS clauses, you can significantly improve the speed and efficiency of your queries. Key strategies include proper indexing, regular maintenance with ANALYZE and VACUUM, applying additional filters within subqueries, and using tools like EXPLAIN to analyze and refine query execution plans. By following the tips and tricks outlined in this guide, you can ensure that your PostgreSQL database handles semi-joins and anti-joins effectively, leading to faster query execution times and better overall performance. Implementing these best practices will help you maintain a high-performance, scalable database environment capable of efficiently managing complex queries and large volumes of data.
Subscribe to my newsletter
Read articles from Shiv Iyer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
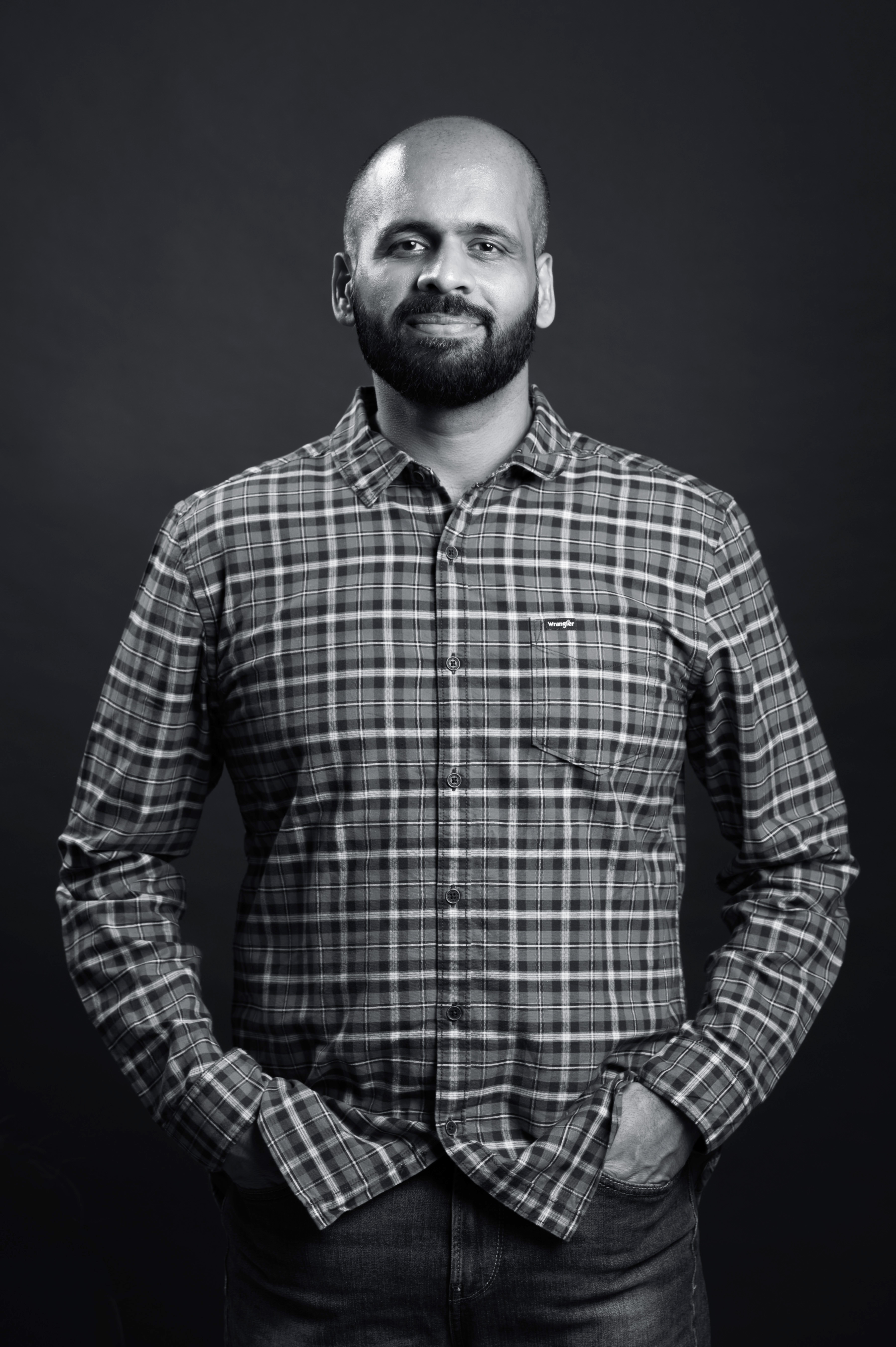
Shiv Iyer
Shiv Iyer
Over two decades of experience as a Database Architect and Database Engineer with core expertize in Database Systems Architecture/Internals, Performance Engineering, Scalability, Distributed Database Systems, SQL Tuning, Index Optimization, Cloud Database Infrastructure Optimization, Disk I/O Optimization, Data Migration and Database Security. I am the founder CEO of MinervaDB Inc. and ChistaDATA Inc.