๐ค A Beginner's Guide to NLP: Building Your First Chatbot with FastAPI ๐
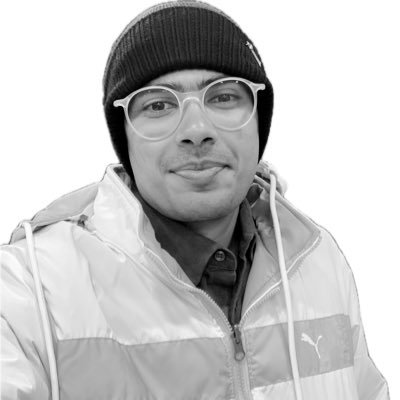
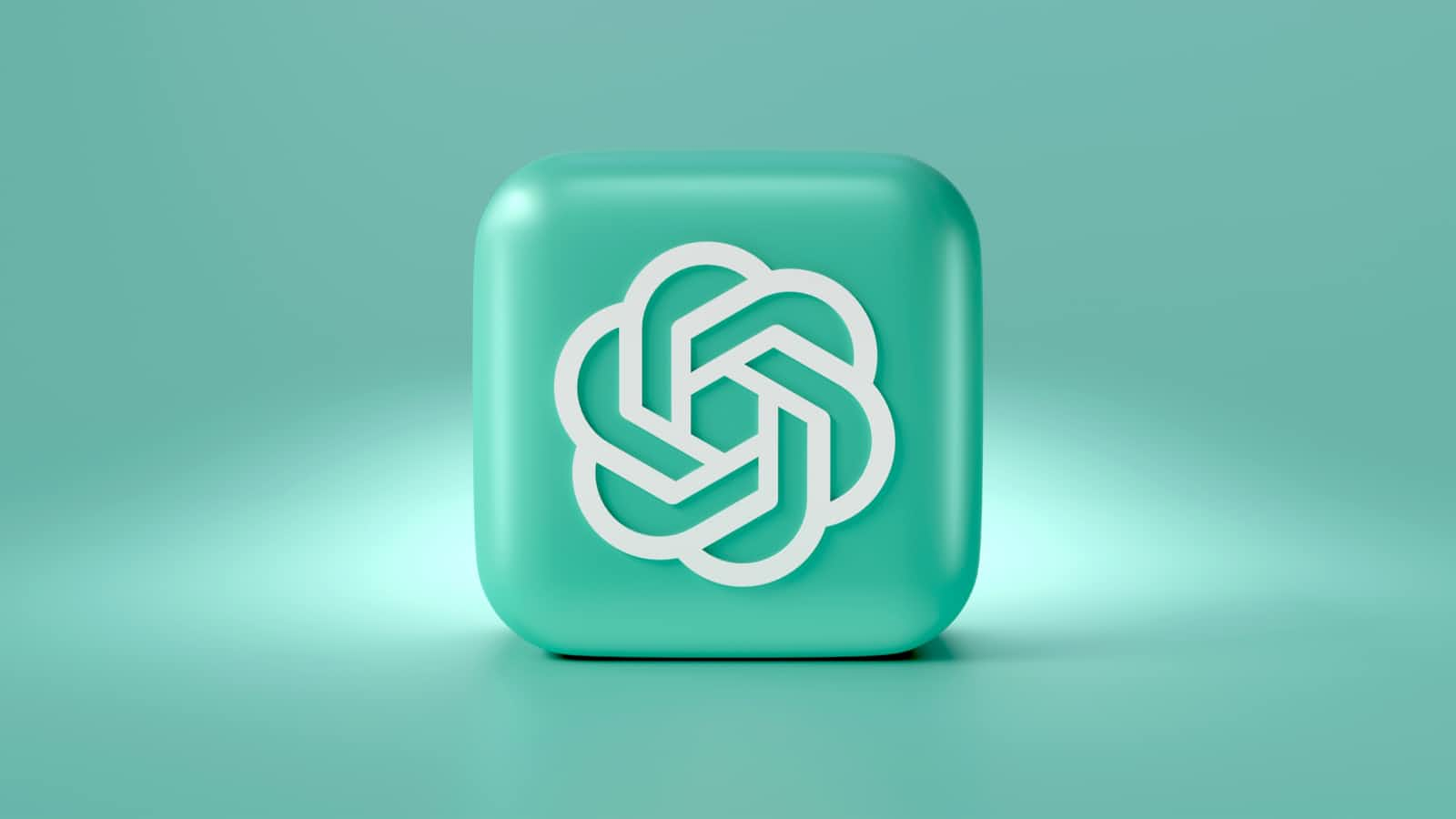
Natural Language Processing (NLP) has become a fundamental part of building intelligent applications that can understand and interact with humans in a natural way. From chatbots to virtual assistants, NLP powers a wide range of applications. In this guide, we'll walk through the process of building your first chatbot using FastAPI, a modern Python web framework, and NLP techniques.
๐ง What is Natural Language Processing?
Natural Language Processing (NLP) is a field of artificial intelligence that focuses on the interaction between computers and humans through natural language. The goal of NLP is to enable machines to understand, interpret, and generate human language in a valuable way.
Key components of NLP include:
Tokenization: Breaking text into individual words or phrases.
Sentiment Analysis: Determining the sentiment or emotion behind a piece of text.
Named Entity Recognition (NER): Identifying entities such as names, dates, and locations within text.
Text Classification: Categorizing text into predefined classes or labels.
๐ ๏ธ Setting Up Your Environment
Before we start building our chatbot, let's set up our development environment.
Prerequisites ๐
Python 3.7+: Make sure you have Python installed on your machine.
FastAPI: We'll use FastAPI for building our web application.
spaCy: A powerful NLP library for Python.
Installing Dependencies ๐ฆ
Create a new directory for your chatbot project and navigate to it in your terminal. Then, create a virtual environment and activate it:
# Create a virtual environment
python3 -m venv chatbot-env
# Activate the virtual environment
# On Windows
chatbot-env\Scripts\activate
# On macOS/Linux
source chatbot-env/bin/activate
Install the required packages using pip:
pip install fastapi uvicorn spacy
# Download the English model for spaCy
python -m spacy download en_core_web_sm
๐ค Building Your Chatbot
Now that we have our environment set up, let's start building our chatbot.
Step 1: Create a FastAPI Application ๐๏ธ
Create a new file named main.py
and import the necessary modules:
# main.py
from fastapi import FastAPI
from pydantic import BaseModel
import spacy
# Load the spaCy model
nlp = spacy.load("en_core_web_sm")
# Initialize the FastAPI app
app = FastAPI()
# Define a data model for incoming messages
class Message(BaseModel):
text: str
Step 2: Define the Chatbot Logic ๐งฉ
Next, we'll create a function to handle incoming messages and generate responses. We'll use spaCy to analyze the text and generate a simple response based on the detected entities:
# main.py
# Define a function to generate chatbot responses
def generate_response(text: str) -> str:
# Process the text with spaCy
doc = nlp(text)
# Extract entities from the text
entities = [(ent.text, ent.label_) for ent in doc.ents]
# Generate a response based on the entities
if entities:
response = f"I see you're talking about {entities[0][0]}, which is a {entities[0][1]}."
else:
response = "I'm not sure what you're talking about. Could you tell me more?"
return response
Step 3: Create an Endpoint for the Chatbot ๐ก
We'll create an endpoint that receives incoming messages and returns a chatbot response:
# main.py
# Define a POST endpoint for the chatbot
@app.post("/chat/")
async def chat(message: Message):
response = generate_response(message.text)
return {"response": response}
Step 4: Run the FastAPI Application ๐โโ๏ธ
Finally, we'll run the FastAPI application using Uvicorn:
# Run the FastAPI app
uvicorn main:app --reload
Open your browser and navigate to http://localhost:8000/docs
to access the interactive API documentation provided by FastAPI. Here, you can test your chatbot by sending messages to the /chat/
endpoint.
๐ Enhancing Your Chatbot
While our chatbot is functional, there are many ways to enhance its capabilities:
Add More Features: Implement additional NLP techniques such as sentiment analysis or text classification to provide more insightful responses.
Integrate with External APIs: Connect your chatbot to external APIs to provide dynamic information, such as weather updates or news articles.
Improve Response Generation: Use more advanced NLP models or machine learning algorithms to generate more natural and context-aware responses.
Deploy Your Chatbot: Deploy your FastAPI application to a cloud platform like AWS, Azure, or Heroku to make it accessible to users worldwide.
Congratulations! You've built your first chatbot using FastAPI and NLP techniques. ๐ This guide provides a foundation for creating more advanced AI-powered applications. As you continue to explore NLP, you'll discover new ways to enhance your chatbot's capabilities and deliver more engaging user experiences.
Happy coding! ๐
Feel free to ask questions or share your own chatbot experiences in the comments below! ๐ฌ
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
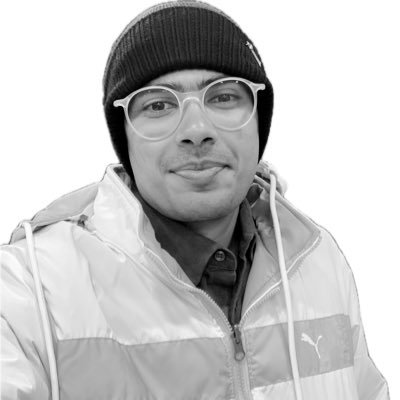
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on โจ๏ธ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix ๐จ๐ปโ๐ป