Object.groupBy and Map.groupBy ๐
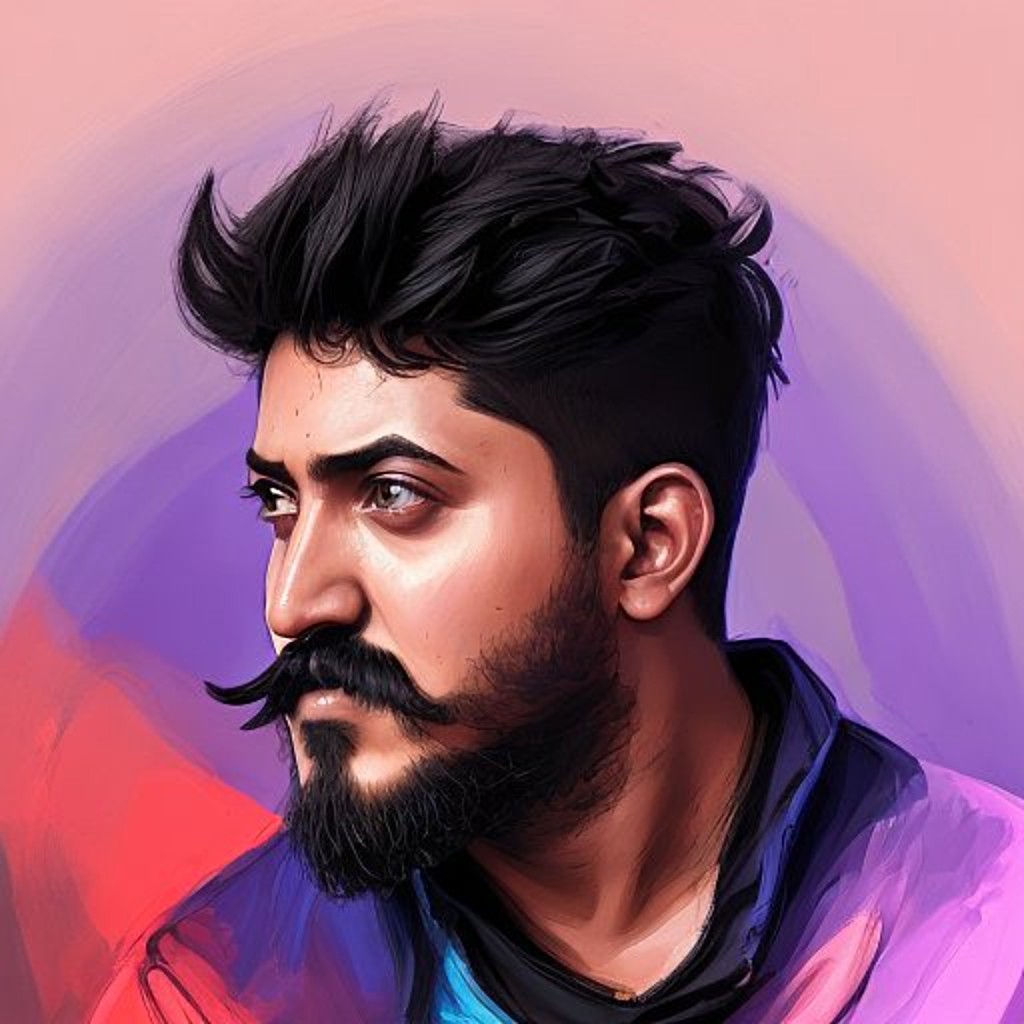
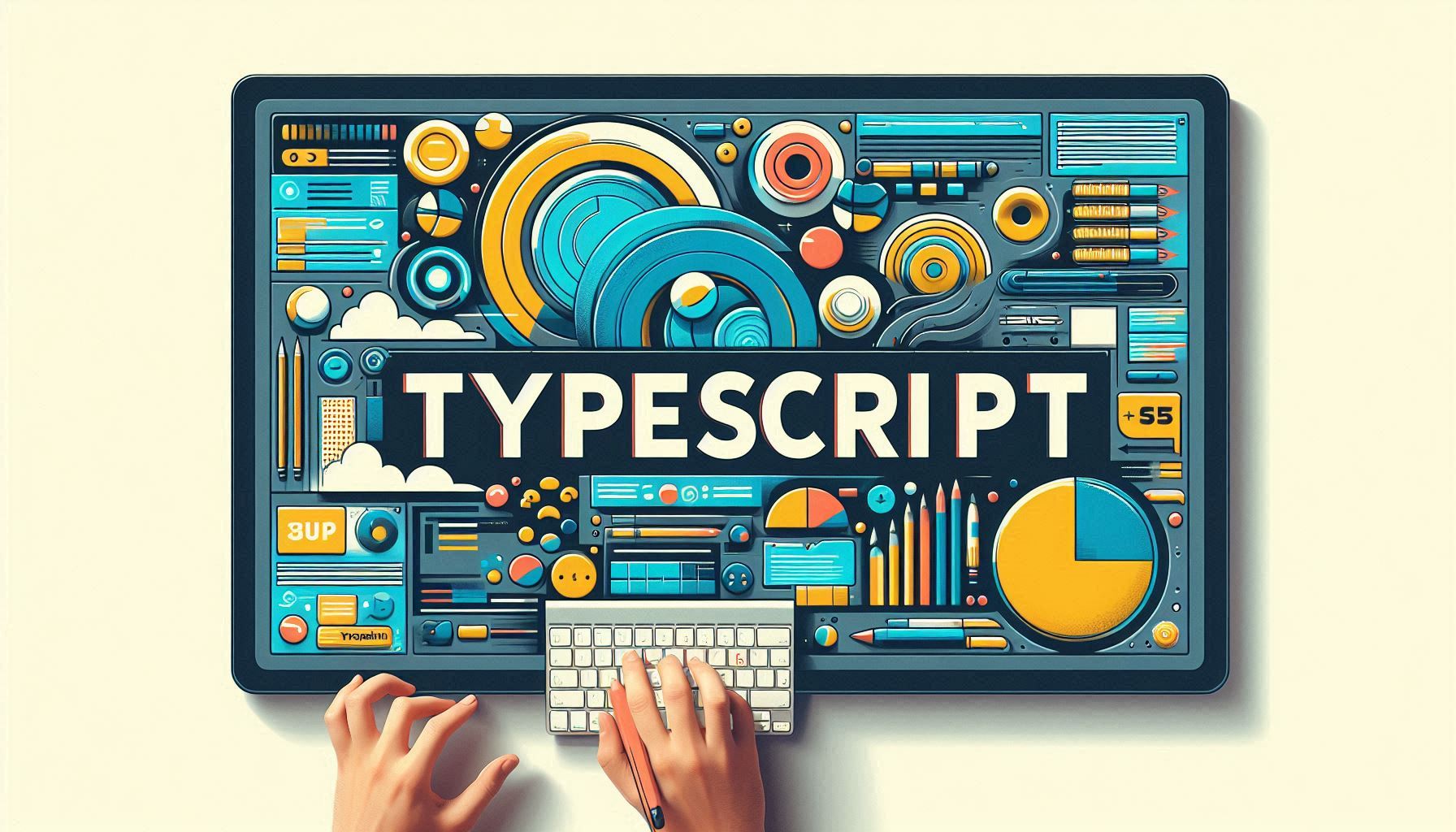
TypeScript 5.5 introduces new methods, Object.groupBy
and Map.groupBy
, which provide built-in functions for grouping elements based on a key generated by a user-defined function. These methods simplify data grouping tasks, making the code cleaner and more efficient.
Why are Object.groupBy and Map.groupBy Important? Grouping data is a common task in many applications, but it often requires custom code to implement. The introduction of Object.groupBy
and Map.groupBy
methods standardizes this process, reducing the need for custom implementations and potential errors.
Example Without groupBy Methods ๐ซ
Before TypeScript 5.5, grouping elements typically involved writing custom code:
const array = [0, 1, 2, 3, 4, 5];
const grouped = array.reduce((acc, num) => {
const key = num % 2 === 0 ? "even" : "odd";
if (!acc[key]) {
acc[key] = [];
}
acc[key].push(num);
return acc;
}, {});
console.log(grouped); // { even: [0, 2, 4], odd: [1, 3, 5] }
In this example, we use reduce
to group numbers into even and odd categories, but the code can be verbose and prone to errors.
Example With Object.groupBy and Map.groupBy ๐
With TypeScript 5.5, the process is simplified using the new groupBy
methods:
const array = [0, 1, 2, 3, 4, 5];
const groupedObject = Object.groupBy(array, num => num % 2 === 0 ? "even" : "odd");
console.log(groupedObject); // { even: [0, 2, 4], odd: [1, 3, 5] }
const groupedMap = Map.groupBy(array, num => num % 2 === 0 ? "even" : "odd");
console.log(groupedMap); // Map { "even" => [0, 2, 4], "odd" => [1, 3, 5] }
Benefits of Object.groupBy and Map.groupBy ๐ฏ
Simplified Data Grouping: Use built-in methods to group data, reducing the need for custom implementations.
Cleaner Code: Enhance code readability and maintainability by using standard methods.
Consistency: Provides a consistent way to group data across different projects and teams.
Real-World Application ๐
Consider a scenario where you have a list of transactions, and you want to group them by type:
interface Transaction {
id: number;
type: "income" | "expense";
amount: number;
}
const transactions: Transaction[] = [
{ id: 1, type: "income", amount: 100 },
{ id: 2, type: "expense", amount: 50 },
{ id: 3, type: "income", amount: 200 },
{ id: 4, type: "expense", amount: 30 }
];
const groupedTransactions = Object.groupBy(transactions, transaction => transaction.type);
console.log(groupedTransactions);
// {
// income: [
// { id: 1, type: "income", amount: 100 },
// { id: 3, type: "income", amount: 200 }
// ],
// expense: [
// { id: 2, type: "expense", amount: 50 },
// { id: 4, type: "expense", amount: 30 }
// ]
// }
Using Object.groupBy
, we can easily group transactions by their type, making the code concise and easy to understand.
Conclusion ๐
The Object.groupBy
and Map.groupBy
methods in TypeScript 5.5 are powerful additions that simplify data grouping tasks. By providing built-in functions, these methods enhance code readability, maintainability, and consistency, making development more efficient and enjoyable.
Ready to Simplify Your Data Grouping?
Try out these new methods in your next TypeScript project and experience the benefits firsthand! Stay tuned for more exciting updates and tips on Tech Talk by Jobin. ๐
For more detailed information, check out the TypeScript 5.5 release notes and the official TypeScript documentation.
Subscribe to my newsletter
Read articles from Jobin Mathew directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
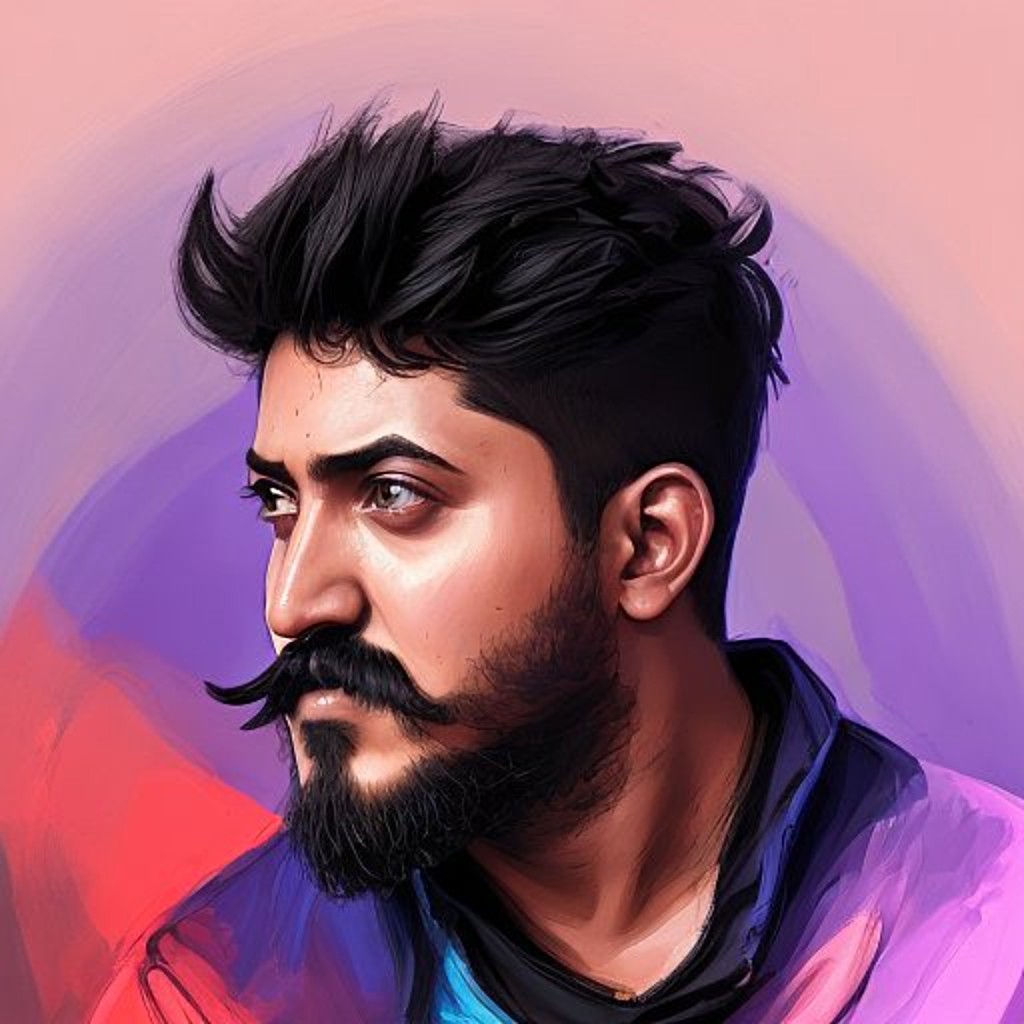
Jobin Mathew
Jobin Mathew
Hey there! I'm Jobin Mathew, a passionate software developer with a love for Node.js, AWS, SQL, and NoSQL databases. When I'm not working on exciting projects, you can find me exploring the latest in tech or sharing my coding adventures on my blog. Join me as I decode the world of software development, one line of code at a time!